React.js in a nutshell
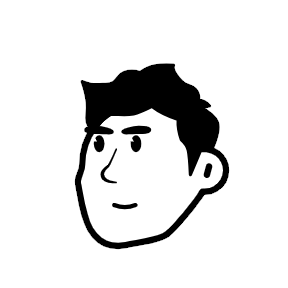
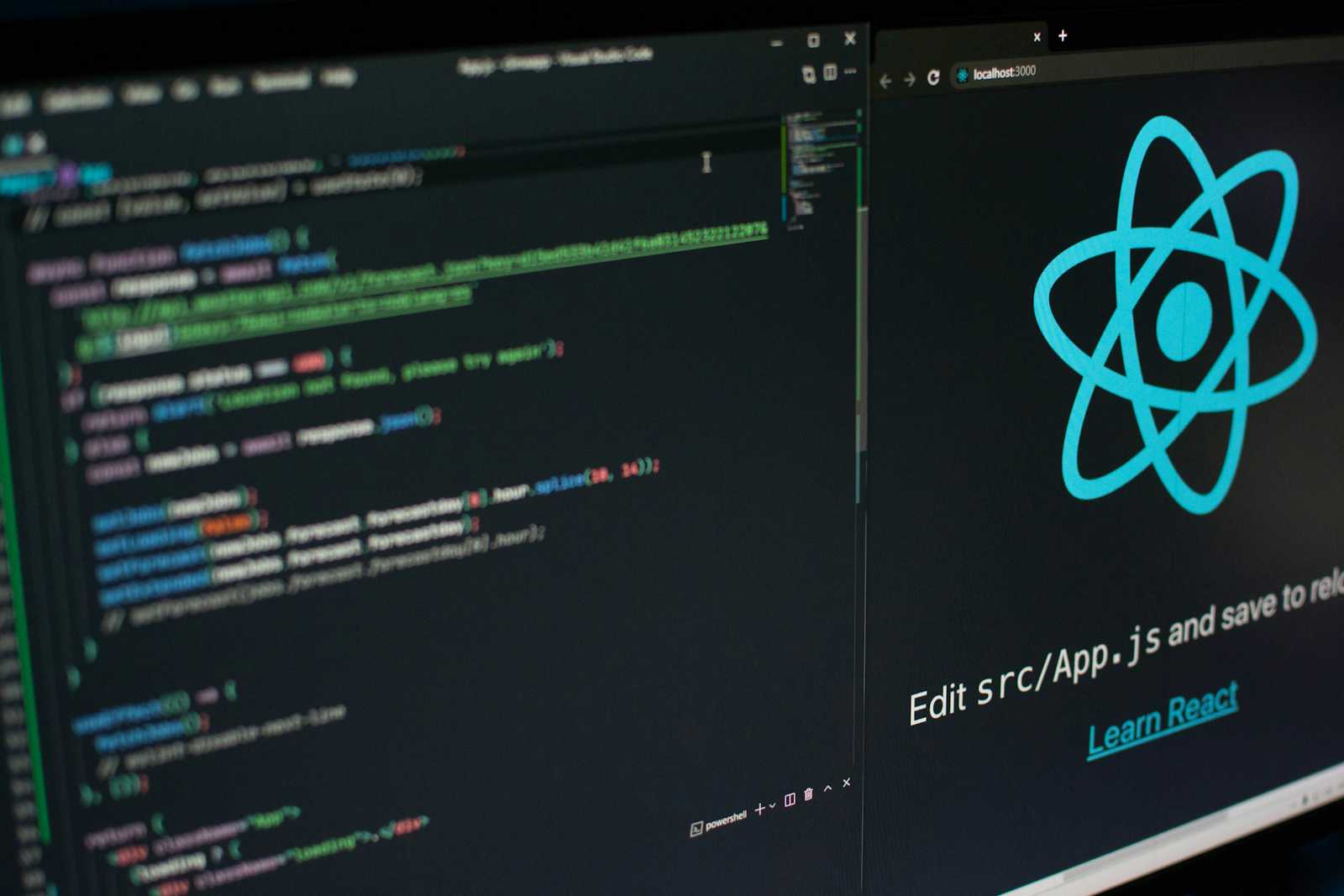
Disclaimer: This article is intended for educational purposes only.
ဒီ article မှာ တော့ React.js နဲ့ပါတ်သက်တဲ့ concept တချို့ကိုလေ့လာသွားပါမယ်။
React.js ဆိုတာ UI user interface တွေကို ရေးဆွဲတဲ့နေရာမှာသုံးတဲ့ JavaScript front-end library တခုပဲဖြစ်ပါတယ်။
Facebook/Meta team ကနေဖန်တီးခဲ့တာဖြစ်ပြီး လက်ရှိမှာတော့ Facebook နဲ့ open-source community ကနေ maintain လုပ်နေပါတယ်။
React.js library ကို လေ့လာဖို့အတွက် JavaScript ကိုသိထားဖို့လိုပါတယ်။
Basic syntax
Es6+ features
Arrow functions
Template literals
JavaScript perequisites
Array method
Object property shorthand
Destructuring
Spread operator
Promises
Async/Await syntax
Import and export syntax
ဒါတွေကိုသိထားပြီဆိုရင် React.js ကိုလေ့လာဖို့အခြေခံကတော့
File & Folder structure
Components
JSX
Props
State
Events
Styling
Conditional Rendering
တို့ဖြစ်ပါတယ်။
နောက်ထပ်လေ့လာဖို့လိုအပ်တာတွေကတော့
useState - for managing state.
useEffect - for handling side effects like data fetching, subscriptions, or manually changing the DOM.
useContext - for sharing data across components without prop drilling.
useRef - for accessing DOM elements directly or persisting values across renders without re-rendering.
useMemo - for memoizing expensive computations to improve performance and avoid unnecessary recalculations.
useReducer - for managing complex state logic, similar to
useState
, but more powerful for handling state transitions.useCallback - for optimizing callback functions by memorizing them to prevent unnecessary re-creations.
ဆိုတဲ့ React.js hooks တွေပဲဖြစ်ပါတယ်။
React.js Concepts
Components
React.js က components တွေကိုအခြေခံထားတဲ့ front-end library တခုပဲဖြစ်ပါတယ်။ ဆိုလိုချင်တာက Web Application တခုမှာပါဝင်ဖွဲ့စည်းထားတဲ့ အစိတ်အပိုင်းတွေကို သေးငယ်တဲ့ components တွေအဖြစ်နဲ့ ခွဲခြားတည်ဆောက်ထားတယ်လို့မြင်ကြည့်လို့ရပါတယ်။
Component ဆိုတာ UI မှာမြင်ရတဲ့ပြန်လည်အသုံးပြုလို့ရတဲ့ သေးငယ်တဲ့အစိတ်ပိုင်း တွေ ဖြစ်ပါတယ်။ React.js ကိုသုံးပြီးဖန်တီးထားတဲ့ application ကိုတော့အဲ့ components တွေနဲ့ပေါင်းစပ်ပြီးတည်ဆောက်ထားတာ ဖြစ်ပါတယ်။ React ကို အသုံးပြုပြီး ရေးဆွဲထားတဲ့ Web Application ရဲ့ UI မှာ မြင်ရတဲ့ Sidebar, Search bar စတာ တွေကတော့ components တွေဖြစ်ပါတယ်။
Types of components
React မှာ components အမျိုးစား ၂ ခုရှိပါတယ်။
Functional Components
Class Components
ဖြစ်ပါတယ်။
Functional Components
import React, { useState } from "react";
const TaskList = () => {
const [tasks, setTasks] = useState(["Read Atomic Habits", "Study CISSP"]);
return (
<div>
<h2>My Tasks</h2>
<ul>
{tasks.map((task, index) => (
<li key={index}>{task}</li>
))}
</ul>
</div>
);
};
export default TaskList;
Class Component
import React, { Component } from "react";
class TaskList extends Component {
constructor(props) {
super(props);
this.state = {
tasks: ["Read Atomic Habits", "Study CISSP"],
};
}
render() {
return (
<div>
<h2>My Tasks</h2>
<ul>
{this.state.tasks.map((task, index) => (
<li key={index}>{task}</li>
))}
</ul>
</div>
);
}
}
export default TaskList;
JSX - JavaScript XML
JSX ကတော့ JavaScript မှာပေါင်းထည့်ထား တဲ့ syntax extension တခုပဲဖြစ်ပါတယ်။ React မှာ UI ကို ဘယ်လိုပုံစံတခုနဲ့ ပြသရမယ်ဆိုတာကိုဖော်ပြဖို့အသုံးပြုပါတယ်။ JSX ဟာ template language တစ်ခုလိုမျိုးထင်မြင်ရ ပေမယ့် javaScript အတိုင်းပဲ လုပ်ဆောင်နိုင်ပါတယ်။ JSX ဟာ React element တွေကိုထုတ်လုပ်ဖို့အတွက် အသုံးပြုတာဖြစ်ပြီးတော့ React ရဲ့ အခြေခံ syntax တစ်ခုလည်းဖြစ်ပါတယ်။
JSX နဲ့ HTML ဟာအတော်များများတူညီမှုရှိပေမယ့် တချို့နေရာတွေမှာ ကွဲလွဲပါသေးတယ်။ class
(HTML) အစား className
(JSX) လို့သုံးပါတယ်။ class
က JavaScript မှာ reserved keyword ဖြစ်နေလို့ပါ။
Basic JSX Example
import React from "react";
const Greeting = () => {
const name = "John";
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
နောက်ထပ်တခုကတာ့ <label>
element အတွက်သုံးတဲ့ ‘for
’ ဆိုတဲ့ attribute ပဲဖြစ်ပါတယ်။
HTML
<label for=””>
JSX
<label htmlfor=””>
ဆိုပြီးသုံးပါတယ်။
နောက်ထပ်မတူတဲ့ ကြီးမားတဲ့ ကွာခြားချက်တခုက တော့ div အစား ‘React Fragments’ ကိုအသုံးပြုနိုင်တာဖြစ်ပါတယ်။
Using <div>
(Standard Approach)
import React from "react";
const TaskList = () => {
return (
<div>
<h2>My Tasks</h2>
<p>Here are your daily tasks:</p>
</div>
);
};
export default TaskList;
Using React Fragments (<>...</>
)
import React from "react";
const TaskList = () => {
return (
<>
<h2>My Tasks</h2>
<p>Here are your daily tasks:</p>
</>
);
};
export default TaskList;
JSX မှာ JavaScript ကိုတိုက်ရိုက်ခေါ်ပြီးအသုံးပြုနိုင်ပါတယ်။ JavaScript ရဲ့ expression တွေကို {}
မှာထည့်ပြီးသုံးနိုင်ပါတယ်။ HTML မှာဆိုရင်တာ့ JavaScript ကိုခေါ်ပြီးသုံးမယ်ဆိုရင် script tag ဒါမှမဟုတ် JavaScript file ကိုခေါ်သုံးဖို့လိုပါတယ်။
What are props?
Components မှာ မတူညီတဲ့ Data တွေကိုလက်ခံ ပေးနိုင်ဖို့အတွက် props or properties ကိုအသုံးပြုနိုင်ပါတယ်။ Props ဆိုတာ တနည်းအားဖြင့် React component မှအသုံးပြုနိုင်တဲ့ argument တခုဖြစ်ပါတယ်။ React မှာ component တခုကနေတခုကို Data တွေ pass လုပ်ပေးချင်တယ်ဆို props ကိုသုံးပါတယ်။
Parent Component —> Child Component
Parent Component (Passing Props)
import React from "react";
import TaskItem from "./TaskItem"; // Import child component
const TaskList = () => {
return (
<div>
<h2>My Tasks</h2>
<TaskItem task="Read Atomic Habits" time="9AM - 10AM" />
<TaskItem task="Study CISSP" time="1PM - 2PM" />
</div>
);
};
export default TaskList;
Child Component (Receiving Props)
import React from "react";
const TaskItem = ({ task, time }) => {
return (
<p>
📌 {task} - <strong>{time}</strong>
</p>
);
};
export default TaskItem;
အပေါ်က component 2 ခုအလုပ်လုပ်သွားတဲ့ပုံစံကို လေ့လာကြည့်ပါမယ်။
Parent (TaskList) က (task and time) ဆိုတဲ့ props တွေကို child(TaskItem) ကို pass လုပ်ပေးပါတယ်။child component က props တွေကို access လုပ်ဖို့နဲ့ ပြန်လည်ပြသဖို့ destructuring({ task, time}) ကိုသုံးပါတယ်။
Output က ဒီလိုဖြစ်သွားမှာပါ..
📌 Read Atomic Habits - 9AM - 10AM
📌 Study CISSP - 1PM - 2PM
Child Component ကနေတော့ Parent Component ကို Data pass မလုပ်နိုင်တာတော့သတိပြုရပါမယ်။
Props ကနေရလာတဲ့ Data က read-only ပဲဖြစ်ပြီးတာ့ လက်ခံရရှိတဲ့ component အနေနဲ့ ပြုပြင် ပြောင်းလဲလို့မရပါဘူး။
What is state?
State ဆိုတာကတော့ React မှာ component ရဲ့လက်ရှိအခြေအနေကို ဖော်ပြတဲ့ အချက်အလက်တခုကို ရည်ညွှန်းတဲ့ plain JavaScript object တခုဖြစ်ပါတယ်။ Function တခုမှာကြေငြာထားတဲ့ variable လိုပဲ Component မှာ state ကိုမြင်ကြည့်လို့ရပါတယ်။ State Object ဟာ component အလိုက်သက်ဆိုင်ရာ property value များကို သိမ်းဆည်းရာ နေရာဖြစ်ပါတယ်။ State object ပြောင်းလဲသွားရင် component ကပြန်လည် render လုပ်ပါတယ်။ State Data ကိုသက်ဆိုင်ရာ component ကပဲပြုပြင် ပြောင်းလဲမှုလုပ်နိုင်ပါတယ်။
What is event?
Event ဆိုတာကတော့ User ရဲ့လုပ်ဆောင်ချက် ကရလာတဲ့ ရလဒ် ဒါမှမဟုတ် system က generate လုပ်လိုက်တဲ့ အဖြစ်အပျက် ပေါ်ကို လိုက်ပြီး ဖြစ်ပေါ်လာတဲ့ လုပ်ဆောင်ချက်တခုပဲဖြစ်ပါတယ်။
ဥပမာ - mouse click, pressing a key နဲ့ other interaction တွေက event တွေဖြစ်ပါတယ်။
React element တွေမှာ event တွေကိုလုပ်ဆောင်တဲ့ပုံစံဟာ DOM element မှာ event တွေကိုလုပ်ဆောင်တဲ့ပုံစံနဲ့တူပါတယ်။ Syntax ရေးသားပုံ တွေမှာတော့ကွာခြားချက်တွေရှိပါတယ်။
React event တွေကိုရေးသားရာမှာ lowercase အစား camelCase ကိုသုံးပါတယ်။
JSX မှာ string အစား function ကို event handler အနေနဲ့ pass လုပ်ပေးပါတယ်။
What are Hooks?
Hooks ဟာ React version 16မှာအသစ်ထပ်ပါလာတာဖြစ်ပြီးတော့ state နဲ့ lifecycle method လိုမျိုး အခြားသော react features တွေကိုလည်းအသုံးပြုလို့ရပါတယ်။ Hook တွေဟာ class တွေအတွင်းမှာ အလုပ်လုပ်မှာမဟုတ်ပါဘူး။ Classes တွေမပါပဲနဲ့ React ကိုအသုံးပြုစေနိုင်မှာဖြစ်ပါတယ်။ Hook တွေဟာ အမြဲတမ်း function တွေကို သုံးစေနိုင်မှာဖြစ်ပြီး Function, classes, high-order component, render component တွေကြားအမြဲတမ်းလဲလှယ် နေဖို့ လိုမှာမဟုတ်တော့ပါဘူး။
useState
Hook (Managing State)
import React, { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<h2>Count: {count}</h2>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
};
export default Counter;
Conclusion
ဒီ article မှာ တော့
Components
JSX
Props
State
Event
တွေအကြောင်းလေ့လာသွားတာဖြစ်ပါတယ်။
Subscribe to my newsletter
Read articles from Wai Yan Pyae Sone directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
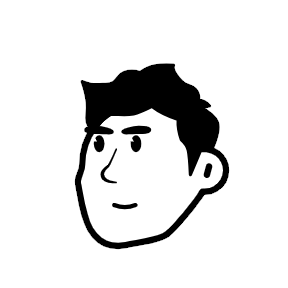