How To Use dotenv (.env) In Node.js?
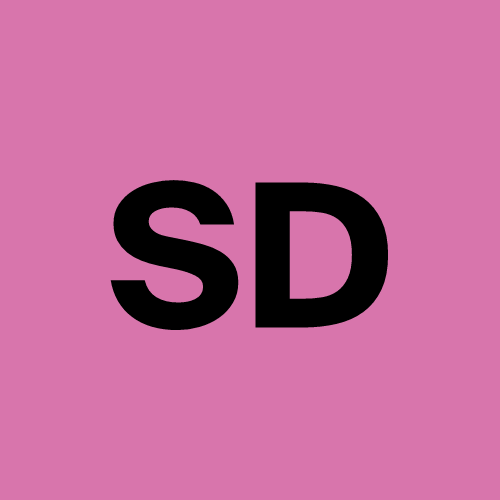
When working on a Node.js project, you often need to manage sensitive data like API keys, database credentials, and other environment-specific configurations. Instead of hardcoding these values directly into your code, a better practice is to store them in a .env
file. This allows you to keep your configuration separate from your codebase and ensures that sensitive information is not exposed in your source code, especially when pushing it to version control systems like Git.
What Is dotenv?
dotenv
is a zero-dependency Node.js module that loads environment variables from a .env
file into process.env
. The .env
file should be placed in the root directory of your project. This file contains key-value pairs of environment variables that can be used within your application.
Steps to use dotenv
Open up any terminal inside your project work space and install
dotenv
.npm install dotenv
Create a
.env
file in the root directory of your project.my-node-app/ ├── node_modules/ ├── other_folder/ ├── .env ├── index.js ├── package.json └── package-lock.json
Add your environment variable in
.env
file as key value pairs .DB_URL=mongodb+srv://username:password@cluster0.pahbl.mongodb.net DB_NAME=mydb PORT=5000
Import and configure the
dotenv
package at the top of your main JavaScript file (e.g.,index.js
).const dotenv = require('dotenv') dotenv.config() //or require('dotenv').config();
Load the environment variable from .env file.
const dbUrl = process.env.DB_URL; const dbName = process.env.DB_NAME;
Default Values: You can also set default values directly in your code in case an environment variable is not defined in the
.env
file. For example, you can use the following syntax for thePORT
variable:const port = process.env.PORT || 3000;
Important Notes
Never Commit Your .env
File to Version Control: Since the .env
file contains sensitive information (like API keys and database passwords), you should never commit it to version control (Git). To prevent this, add the .env
file to your .gitignore
:
# .gitignore
.env
Conclusion
Using the dotenv
package in Node.js is a simple and effective way to manage environment variables. It helps keep sensitive data like API keys and database credentials safe, and it makes your code more maintainable by separating configuration from code logic.
By following the steps outlined in this article, you can easily manage different configurations for different environments and ensure that your application behaves consistently across different stages of development.
Happy coding!
Subscribe to my newsletter
Read articles from Surajit Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
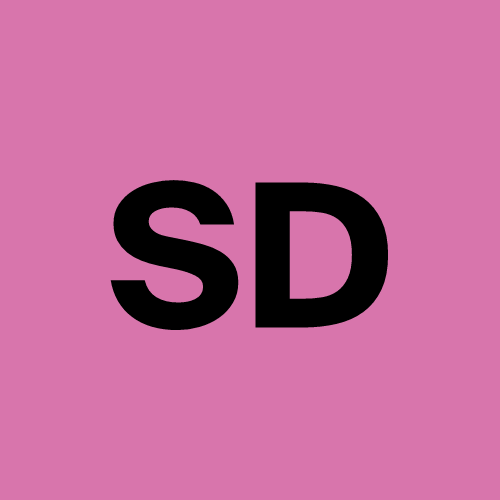