How to Create a REST API with Node.js and Express Using MVC
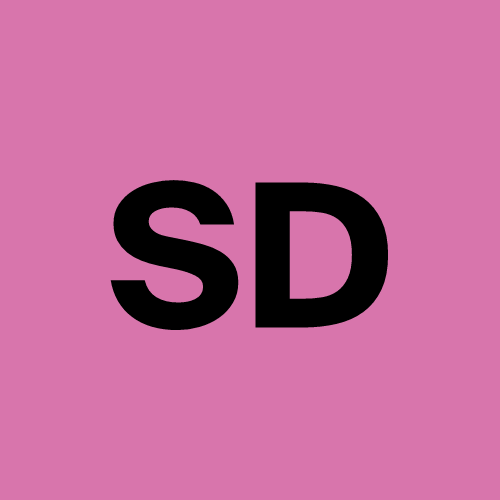
Creating a REST API is a common task for web developers, and using Node.js with Express is a popular choice due to its simplicity and efficiency. In this article, we will walk through the steps to create a REST API for a user CRUD (Create, Read, Update, Delete) operation while following the MVC (Model-View-Controller) architecture. We will also use MongoDB as our database.
Prerequisites
Before we begin, ensure you have the following installed on your machine:
Node.js
npm (Node Package Manager)
MongoDB (either locally or using a service like MongoDB Atlas)
Steps
First, create a new folder for your project and navigate into it:
mkdir user-crud-api cd user-crud-api
Next, initialize a new Node.js project using npm. Open terminal and execute -
npm init -y
Now, install the necessary packages for our project:
npm install express mongoose
Install
nodemon
as dev dependency.npm install -D nodemon
Open
package.json
and under thescripts
add a key value pair to start the server with nodemon."server": "nodemon index.js"
Create the following folder structure:
user-crud-api/ │ ├── controllers/ │ └── user.controller.js │ ├── models/ │ └── user.model.js │ ├── routers/ │ └── user.router.js │ ├── utils/ │ └── db.js │ └── index.js
Open up
index.js
file and write necessary code to start the server with a basic health check end-point.// index.js // import express const express = require('express') // define port, where server will start const PORT = 5000; //initialize the express server const app = express() // health-check route app.get("/",(req, res)=>{ res.send("Hello World") }) // run server app.listen(PORT, function(){ console.log(`Server started at port ${PORT}`) })
Run the server and check the
localhost:5000/
endpoint.npm run server
If everything is working fine, time to add database configuration and connect to database. Copy the connection string from the MongoDB Atlas or use your local MongoDB path. If you are using MongoDB Atlas you connection string may look like the following. You can trim out the remaining. Replace the
username
andpassword
with your username and password.mongodb+srv://username:password@cluster0.pfose.mongodb.net
In the
utils/db.js
file, set up the MongoDB connection:// utils/db.js const mongoose = require('mongoose'); const connectDB = async () => { const DB_URL="mongodb+srv://username:password@cluster0.pfose.mongodb.net" const DB_NAME="user-crud" try { await mongoose.connect(`${DB_URL}/${DB_NAME}`) console.log('MongoDB connected'); } catch (error) { console.error('MongoDB connection error:'); throw error } }; module.exports = connectDB;
Import the
connectDB
function inindex.js
file check if the database is getting connected or not.const express = require('express') //add this const connectDB = require('./utils/db.js') // import the file const PORT = 5000; const app = express() app.get("/",(req, res)=>{ res.send("Welcome to Event Portal") }) app.listen(PORT, function(){ console.log(`Server started at port ${PORT}`) //add this connectDB(); //call the function })
In the
models/user.model.js
file, define the User schema:// models/user.model.js const mongoose = require('mongoose'); const userSchema = new mongoose.Schema({ name: { type: String, required: true, }, email: { type: String, required: true, unique: true, }, age: { type: Number, required: true, }, }); const User = mongoose.model('User', userSchema); module.exports = User;
In the
controllers/user.controller.js
file, add function to create user and export it:// controllers/user.controller.js const User = require('./../models/user.model.js'); // Create a new user const createUser = async (req, res) => { try { const user = new User(req.body); await user.save(); res.status(201).json(user); } catch (error) { res.status(400).json({ message: error.message }); } }; module.exports = { createUser }
In the
routers/user.router.js
file, set up the routes for the user operations:// routers/user.router.js const express = require('express'); const { createUser } = require('../controllers/user.controller'); const userRouter = express.Router(); // Define routes userRouter.post('/', createUser ); module.exports = userRouter ;
In the
index.js
file, import the router and required middleware.// index.js const express = require('express'); const connectDB = require('./utils/db'); // add this const userRouter = require('./routers/user.router'); const app = express(); const PORT = 5000; // add these // Middleware app.use(express.json()) app.use(express.urlencoded({extended: true})) // add this // Routes app.use('/users', userRouter); // Start the server app.listen(PORT, function(){ console.log(`Server started at port ${PORT}`) connectDB(); })
Check the console, if the server is still running or not. If running open up and API client like Postman, make a POST request to
http://localhost:5000/users
and try to add user.{ "name": "John Doe", "email": "john@example.com", "age": 30 }
In the
controllers/user.controller.js
file, add rest of the functions to complete the CRUD operations:// controllers/user.controller.js const User = require('./../models/user.model.js'); // Create a new user const createUser = async (req, res) => { try { const user = new User(req.body); await user.save(); res.status(201).json(user); } catch (error) { res.status(400).json({ message: error.message }); } }; // Get all users const getAllUsers = async (req, res) => { try { const users = await User.find(); res.status(200).json(users); } catch (error) { res.status(500).json({ message: error.message }); } }; // Get a user by ID const getUserById = async (req, res) => { try { let { id } = req.params const user = await User.findOne({_id: id}); if (!user) { return res.status(404).json({ message: 'User not found' }); } res.status(200).json(user); } catch (error) { res.status(500).json({ message: error.message }); } }; // Update a user const updateUser = async (req, res) => { try { let { id } = req.params let user = req.body user = await User.findOneAndUpdate({_id: id}, user, {new: true}); if (!user) return res.status(404).json({ message: 'User not found' }); res.status(200).json(user); } catch (error) { res.status(500).json({ message: error.message }); } }; // Delete a user const deleteUser = async (req, res) => { try { let { id } = req.params let user = await User.findOneAndDelete({_id: id}) if (!user) return res.status(404).json({ message: 'User not found' }); res.status(200).send(user); } catch (error) { res.status(500).json({ message: error.message }); } }; module.exports = { createUser, getAllUsers, getUserById, updateUser, deleteUser }
In the
routers/user.router.js
file, link rest of the controller functions:// routers/user.router.js const express = require('express'); const { createUser, getAllUsers, getUserById, updateUser, deleteUser } = require('../controllers/user.controller'); const userRouter = express.Router(); // Define routes userRouter.post('/', createUser ); userRouter.get('/', getAllUsers); userRouter.get('/:id', getUserById); userRouter.put('/:id', updateUser ); userRouter.delete('/:id', deleteUser ); module.exports = userRouter ;
Look for any errors in the console, test the end points otherwise.
Conclusion
In this article, we walked through the steps to create a REST API using Node.js and Express while following the MVC architecture. We set up a user CRUD application with MongoDB as the database. This structure not only helps in organizing your code but also makes it easier to maintain and scale your application in the future.
Feel free to expand upon this basic structure by adding features such as authentication, validation, and error handling to make your API more robust.
Happy coding!
Subscribe to my newsletter
Read articles from Surajit Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
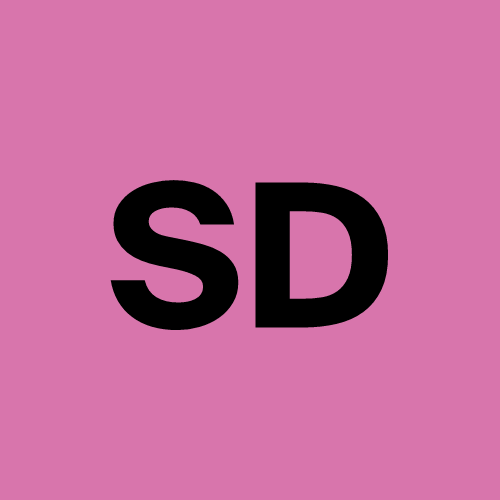