Find and Replace Text in PowerPoint with Python Like an Expert

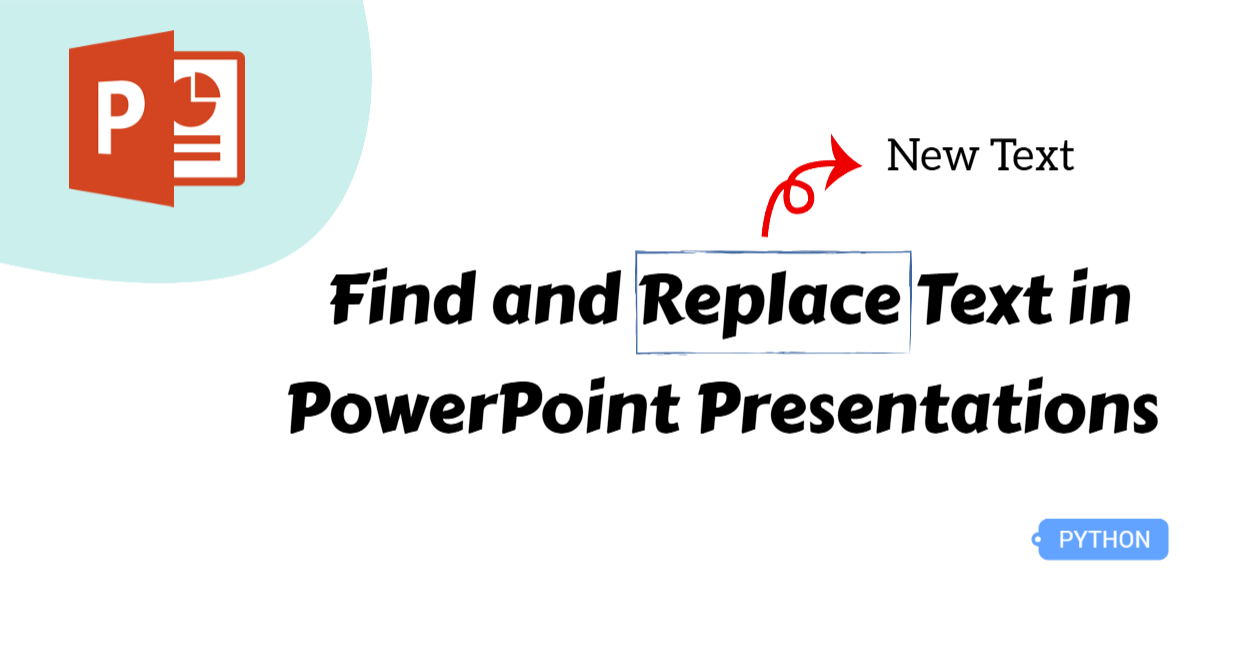
Manually searching for and updating text in PowerPoint presentations can be time-consuming, especially when working with lengthy slideshows. While PowerPoint offers a built-in Find and Replace feature, it still requires opening each file and making edits manually, which isn't ideal for bulk changes. A more efficient solution is to automate the process with Python. By using Python, you can find and replace text in PowerPoint across multiple slides or presentations instantly—without even opening PowerPoint. In this article, we’ll explore how to achieve seamless text replacement with Python in just one click.
How to Find and Replace Text in PowerPoint: First Occurrence
When updating text in PowerPoint, you may not always need to replace every occurrence of a word or phrase. Sometimes, updating only the first match is enough. While PowerPoint allows manual control over replacements, automating this task with Python requires the right tools.
One efficient way to achieve this is by using Spire.Presentation for Python, a powerful and feature-rich library that enables PowerPoint processing without requiring MS Office. It provides an intuitive API for handling slides, text, images, and more, making text replacement straightforward.
To get started, install the library using the following pip command: pip install Spire.Presentation
.
Let’s go through how to use Spire.Presentation to find and replace text in PowerPoint.
Steps to find and replace the first occurrence of a text in PowerPoint presentations:
Create a Presentation class.
Read a source PowerPoint file using the Presentation.LoadFromFile() method.
Loop through all slides in the PowerPoint presentation.
Find and replace the first instance of the matching text through the ISlide.ReplaceFirstText() method.
Save the updated PowerPoint file with the Presentation.SaveToFile() method.
Here is a Python example showing how to replace the first occurrence of “AI-Generated Art” in a PowerPoint file:
from spire.presentation.common import *
from spire.presentation import *
# Create an object of the Presentation class
ppt = Presentation()
# Load a PowerPoint document
ppt.LoadFromFile("/AI-Generated Art.pptx")
# Loop through all slides in the document
for slide in ppt.Slides:
# Replace the first occurrence of "Spire.Presentation for Python" with "E-iceblue Product"
slide.ReplaceFirstText("AI-Generated Art", "New Text", False)
break
# Save the result document
ppt.SaveToFile("/ReplaceFirstTextOccurrence.pptx", FileFormat.Pptx2013)
ppt.Dispose()
Find and Replace Text in PowerPoint: All Occurrences
If a PowerPoint document contains errors or outdated information, updating it efficiently is crucial. In cases where the same text appears multiple times—such as product names or other key details—replacing all occurrences at once is the best approach.
With Spire.Presentation for Python, you can automate this process using the ISlide.ReplaceAllText() method. This allows you to update all matching text in just one step, ensuring consistency across the presentation.
Next, let’s go through the specific steps to achieve this.
Steps to find and replace all occurrences of a text in PowerPoint:
Create an object of the Presentation class.
Read a PowerPoint presentation from files with the Presentation.LoadFromFile() method.
Loop through each slide in the PowerPoint presentation.
Find and replace all instances of the matching text through the ISlide.ReplaceAllText() method.
Save the updated PowerPoint file as a new one.
Below is an example of replacing all occurrences of “AI-Generated Art” with “New Text” in a PowerPoint file:
from spire.presentation.common import *
from spire.presentation import *
# Create an object of the Presentation class
ppt = Presentation()
# Load a PowerPoint document
ppt.LoadFromFile("/AI-Generated Art.pptx")
# Loop through all slides in the document
for slide in ppt.Slides:
# Replace all occurrences of "Spire.Presentation for Python" with "E-iceblue Product"
slide.ReplaceAllText("AI-Generated Art", "New Text", False)
# Save the result document
ppt.SaveToFile("/ReplaceAllTextOccurrences.pptx", FileFormat.Pptx2013)
ppt.Dispose()
How to Find and Replace Text in PowerPoint Using Regular Expression
Sometimes, the text you need to replace isn’t identical but follows a specific pattern, such as dates, phone numbers, or other structured text. In such cases, manually searching for each variation can be time-consuming. This is where regular expressions come in handy. They allow you to efficiently find and replace text based on patterns rather than exact matches.
Let’s explore how to use regular expressions in Python to locate and replace text in a PowerPoint slide.
Steps to find and replace text in PowerPoint presentations using regular expression:
Create an instance of the Presentation class.
Load a PowerPoint presentation through the Presentation.LoadFromFile() method.
Iterate through all slides in the presentation.
Loop through all shapes on each slide.
Replace text that matches a regular expression pattern using IShape.ReplaceTextWithRegex() method.
Save the modified PowerPoint presentation.
The code example below shows how to replace matching text with regular expressions:
from spire.presentation.common import *
from spire.presentation import *
# Create an object of the Presentation class
ppt = Presentation()
# Load a PowerPoint document
ppt.LoadFromFile("/test ppt.pptx")
# Loop through all slides in the document
for slide in ppt.Slides:
# Loop through all shapes on each slide
for shape in slide.Shapes:
# Replace text starting with # on the slide to "Monitor"
shape.ReplaceTextWithRegex(Regex("#\w+"), "Monitor")
# Save the result document
ppt.SaveToFile("/ReplaceTextUsingRegex.pptx", FileFormat.Pptx2013)
ppt.Dispose()
The Conclusion
Mastering how to find and replace text in PowerPoint efficiently can significantly enhance your workflow. Whether you need to update the first occurrence of a word, replace all instances across slides, or leverage regular expressions for advanced text manipulation, these techniques provide flexibility and precision. By incorporating these methods, you can streamline presentations, ensure consistency, and save valuable time in document editing.
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
