When AWS Lambda Meets API Gateway

Table of contents
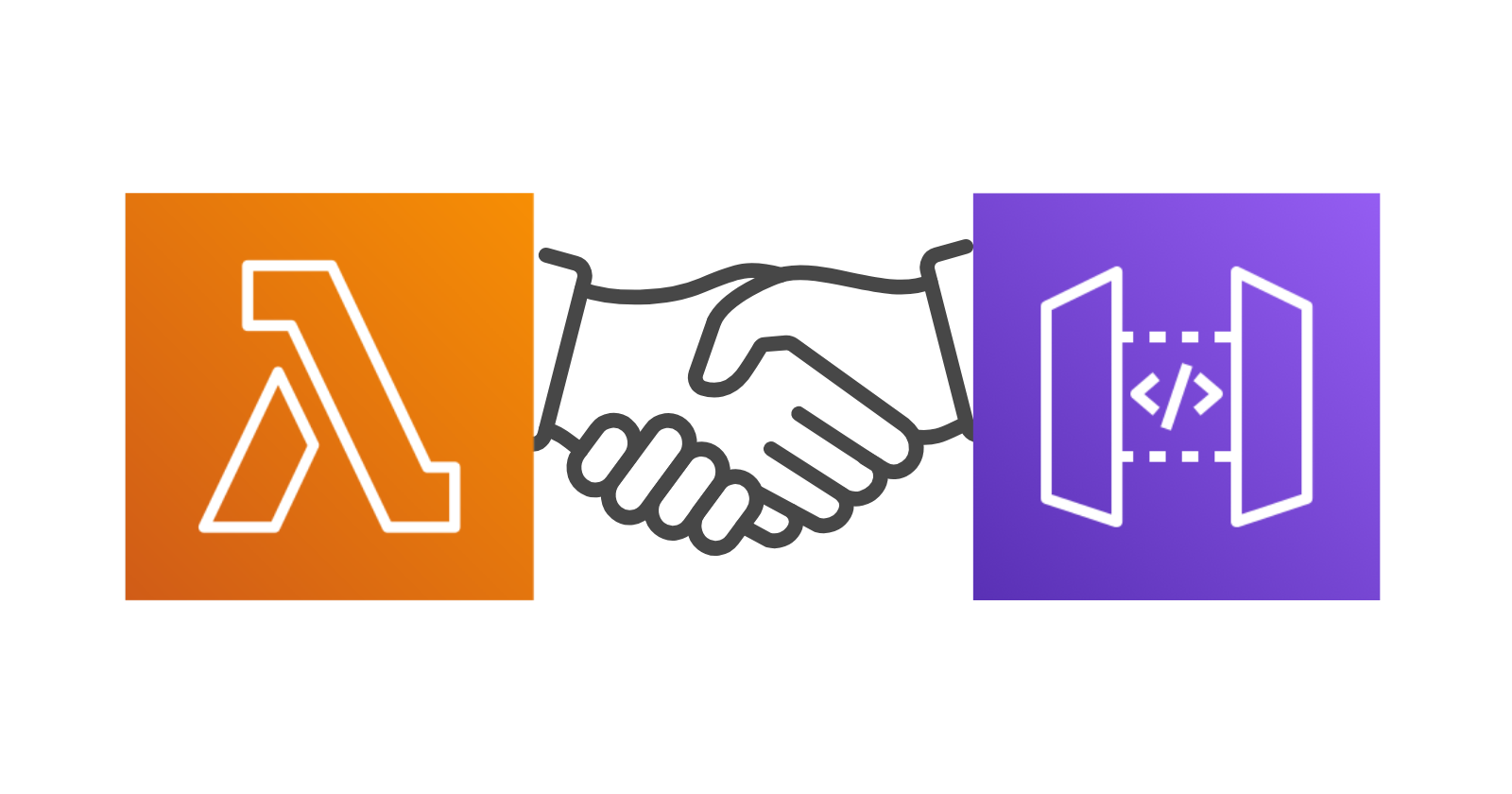
Disclaimer: This article is a continuation of the one below. If you have already read it or have a Lambda function to deploy to API Gateway, you may proceed. Otherwise, I recommend reading the previous article first.
Introduction
Now that you’ve successfully deployed an AWS Lambda function to calculate Fibonacci numbers, it's time to expose it as a REST API using AWS API Gateway.
Without API Gateway, your Lambda function can only be invoked through the AWS Console, CLI, or AWS SDKs. However, by integrating it with API Gateway, we can:
Make Lambda accessible via HTTP requests.
Enable frontend and mobile apps to communicate with it.
Enhance scalability and security.
Easily control request authentication and authorisation.
In this guide, we will connect your existing AWS Lambda function (which calculates Fibonacci numbers using an npm
package) to AWS API Gateway and transform it into a fully functional API endpoint. By the end of this tutorial, you’ll have a working API that can be called via any browser, Postman, or curl request.
This tutorial will help you understand how to connect AWS Lambda to API Gateway.
Why Should You Integrate API Gateway with AWS Lambda?
AWS Lambda alone is powerful, but combining it with API Gateway unlocks new possibilities for modern web and mobile applications.
1. Exposing AWS Lambda as a REST API: API Gateway allows us to make Lambda functions callable via HTTP endpoints. This means your function can be accessed using a simple URL instead of relying on AWS CLI or SDKs. (Don’t worry, we will create our own shortly.)
2. Security and Access Control: With API Gateway, we can secure our Lambda function using:
IAM roles (AWS identity-based access control)
API keys (restrict access to authorised users)
CORS (Cross-Origin Resource Sharing) settings
3. Request & Response Transformation: API Gateway lets us modify the request before passing it to Lambda and format the response before sending it back to the client.
4. Rate Limiting & Caching: You can control API usage by setting rate limits and improve performance using caching mechanisms.
5. Cost Optimisation: By integrating Lambda with API Gateway, you get a server-less, scalable, and cost-efficient API, where you only pay for execution time.
Now that you know some good reasons to integrate, let's jump directly to the AWS Console and create the API.
Step 1: Open API Gateway in AWS Console
Go to the AWS Management Console.
Search for API Gateway and open it.
Click "Create API".
At this point, you’ll see several API types to choose from:
REST API (Recommended) → Best for full-featured APIs.
HTTP API (Cheaper & Faster) → Ideal for simple integrations.
WebSocket API → Used for real-time, event-driven applications.
REST API Private → REST API that is only accessible from within a VPC.
Choose "REST API" since we need fine-grained control. Click Build to proceed.
Step 2: Create a New API
Now, configure the new API:
Choose "New API"
Give it a name (
FibonacciAPI
)Set Endpoint Type
Regional (default): Best for most applications
Edge-Optimised: Used for low-latency global APIs
Private: For internal AWS network use
Select "Regional" (best for standard use cases). Click Create API.
Step 3: Create a New Resource
Now, we need to create an API resource that will serve as an endpoint for our function:
Go to "Resources" (Left Sidebar)
Click "Create Resource"
Enter Resource Name →
fibonacci
Enable "CORS"
Click Create Resource
Step 4: Create a Method and Connect It to Lambda
Now, let's create a POST method that will send data to our Lambda function.
Select the
/fibonacci
resource.Click "Create Method".
Choose POST method from method type dropdown.
Under Integration Type, select Lambda Function.
Select your Lambda function from the dropdown from the specific region.
Click Save and OK when prompted to give permissions.
Your API is now linked to Lambda!
Other Settings You May Explore
1. Authorisation: Specifies how API Gateway should handle authentication for this method.
Options:
None: No authentication is required (public API).
AWS IAM: Uses AWS Identity and Access Management (IAM) permissions.
When to use it?
Use None for public APIs.
Use IAM for internal AWS services.
2. Request Validator: Determines whether API Gateway should validate request parameters before invoking the backend.
Options:
None: No validation.
Validate only parameters & headers: Checks query parameters and headers.
Validate body: Validates request body based on a schema.
Validate body, parameters, and headers: Validates all incoming request data.
When to use it?
Use validation when you want to enforce required query parameters, headers, or body structure before invoking Lambda.
Helps prevent invalid requests from reaching backend services.
3. API Key Required: Specifies whether a client must provide an API key in the request.
When to use it?
Use it when you want to track usage and limit access to specific clients via API keys.
Ideal for rate-limiting, monetisation, or private API access.
4. Operation Name (Optional): A label for the operation, helpful for logging and API documentation.
When to use it?
When you want to identify API operations easily in logs or API definitions.
Example: Naming it GetPets for a method that fetches pet data.
Other expandable sections:
URL Query String Parameters: Defines required/optional query parameters.
HTTP Request Headers: Specifies required headers (e.g.,
Content-Type
).Request Body: Defines body structure for methods like
POST
andPUT
.
These settings help configure how requests are handled before reaching the backend, improving security, validation, and access control in your API.
Step 5: Deploy the API
Before testing, we need to deploy the API:
Click "Deploy API".
Create a New Stage (let’s say,
prod
).Click Deploy.
AWS will generate a public URL for your API. Copy this URL—we’ll use it for testing!
https://4kabch7a4m.execute-api.ap-south-1.amazonaws.com/prod
Step 6: Test the API
Now, let's send a POST request with input data:
You can use Curl or Postman for testing the endpoint:
curl --location 'https://4kabch7a4m.execute-api.ap-south-1.amazonaws.com/prod/fibonacci' \
--header 'Content-Type: application/json' \
--data '{
"number": 10
}'
You should get a response like this:
{
"number": 10,
"result": 55
}
Congratulations! You’ve successfully:
Connected AWS Lambda to API Gateway
Created a fully functional API
Why Use API Gateway Beyond AWS Lambda?
While integrating AWS Lambda with API Gateway is a common practice, API Gateway's capabilities extend far beyond serving as a front-end for Lambda functions. It offers a robust set of features that enhance the management, security, and performance of APIs across various architectures.
1. Centralised API Management: API Gateway acts as a unified entry point for multiple backend services, simplifying the process of monitoring, versioning, and maintaining APIs. This centralised approach streamlines operations and ensures consistent API behaviour across different services.
2. Enhanced Security Features: With built-in support for authentication mechanisms such as AWS Identity and Access Management (IAM) policies, Lambda authoriser functions, and Amazon Cognito user pools, API Gateway ensures secure access to APIs. It also integrates with AWS Web Application Firewall (WAF) to protect APIs from common web exploits.
3. Traffic Management and Scalability: API Gateway efficiently handles traffic management tasks, including request throttling and burst rate limits, to protect backend services from being overwhelmed. It automatically scales to accommodate varying levels of API traffic, ensuring consistent performance during peak usage periods.
4. Protocol Flexibility: Beyond RESTful APIs, API Gateway supports WebSocket APIs, enabling real-time two-way communication applications such as chat apps and streaming dashboards. This versatility allows developers to choose the most appropriate protocol for their application's needs.
5. Cost Efficiency: As a fully managed service, API Gateway eliminates the need for provisioning and managing infrastructure for API hosting. Its pay-as-you-go pricing model ensures that organisations only pay for the API calls they receive and the amount of data transferred out, optimising cost management.
By leveraging these features, organisations can build secure, scalable, and high-performance APIs that cater to a wide range of use cases, independent of AWS Lambda.
Conclusion
Integrating AWS Lambda with API Gateway transforms server-less functions into robust, scalable APIs accessible over standard HTTP protocols. This integration not only simplifies the deployment of micro-services but also enhances security, performance, and monitoring capabilities. By following the steps outlined in this guide, developers can effectively expose Lambda functions via API Gateway, unlocking the full potential of server-less architectures.
If you ever need help or just want to chat, DM me on Twitter / X or LinkedIn.
Subscribe to my newsletter
Read articles from Kartik Mehta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kartik Mehta
Kartik Mehta
A code-dependent life form.