All You Need to Know About the useCallback Hook

Table of contents
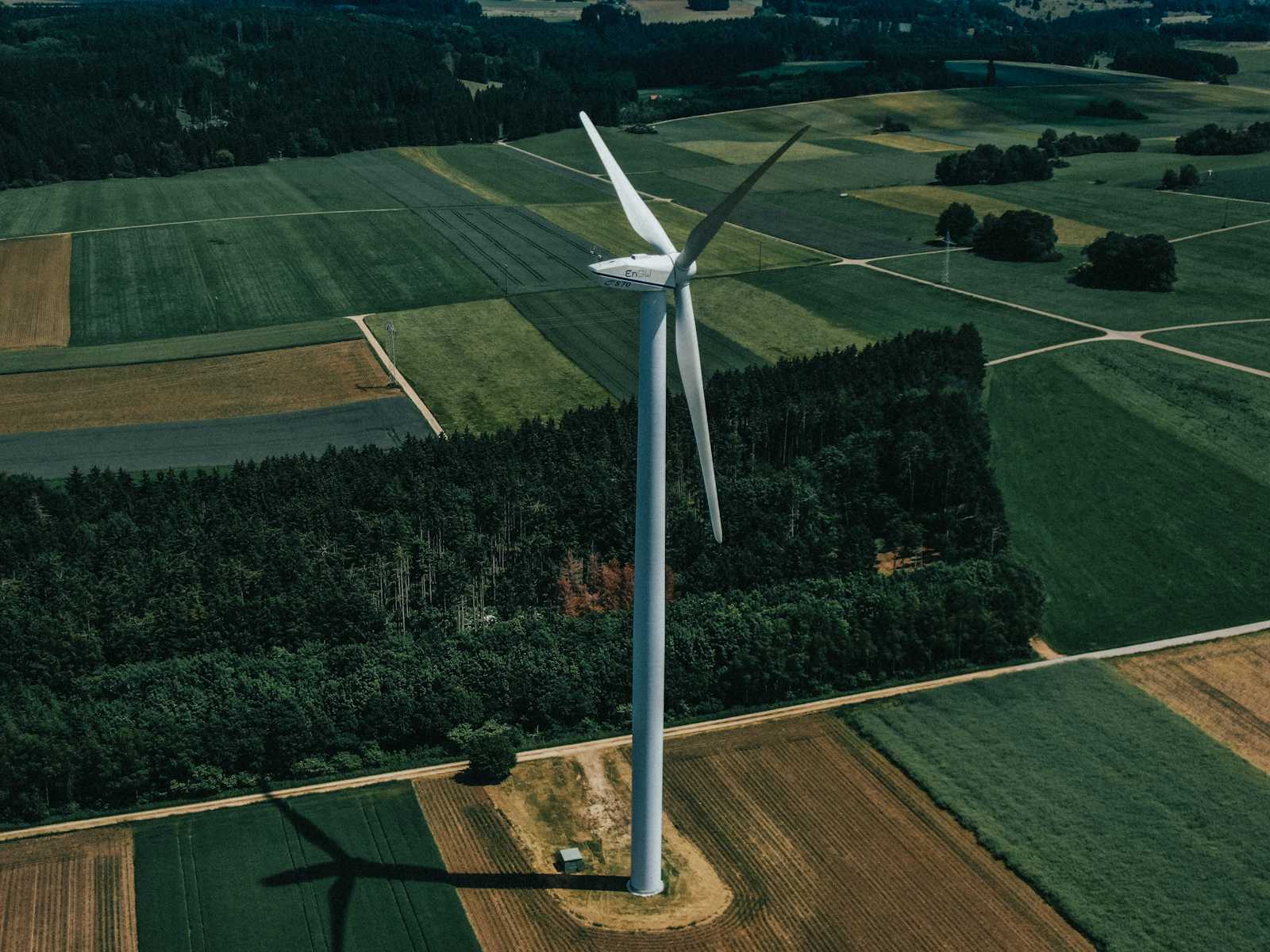
At present, when I am writing this article, React 19 is already out there, where useCallback
is not necessary because of the new React Compiler. Since the new React compiler optimizes performance, there is no real need for using useCallback
hook. However, it is not obsolete and many applications have been built on React 18. So, it’s crucial to understand how useCallback
works.
In this article, I will primarily explore what the recreation of functions in components is, how useCallback
hook prevents them from being recreated and how this leads to performance optimization.
What is Function Recreation in React Components?
In JavaScript, functions are objects, meaning they are reference types. In React, when a component re-renders, every function declared inside it gets re-created (a new reference is assigned) unless it's memorized.
import { useState } from "react"
const Counter = () => {
const [count, setCount] = useState(0)
// This function is re-created on every render
const increment = () => {
setCount(count + 1)
}
console.log("Counter re-rendered!") // Logs on every render
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
)
}
export default Counter
What Happens Here?
The component renders for the first time.
The
increment
function is created.When the button is clicked,
setCount
updates the state.The component re-renders, and a new version of
increment
is created in memory.The process repeats on every render.
This means every render creates a brand new function, even though the logic inside it remains the same.
Why is Function Recreation a Problem?
Function recreation itself is not a problem in simple cases. But in more complex scenarios, it can cause unnecessary re-renders or break optimizations in React.
Problem 1: Function Recreation Causes Unnecessary Child Re-renders
Whenever a function is passed as a prop to a child component, if that function gets re-created on every render, the child component will re-render unnecessarily even when its props haven’t changed.
import React, { useState } from "react"
// Child component that only re-renders when its props change
const Button = React.memo(({ onClick }: { onClick: () => void }) => {
console.log("Button re-rendered!")
return <button onClick={onClick}>Click Me</button>
})
const Parent = () => {
const [count, setCount] = useState(0)
const [otherState, setOtherState] = useState(false)
// Function gets re-created on every render
const increment = () => {
setCount(prev => prev + 1)
}
console.log("Parent re-rendered!")
return (
<div>
<p>Count: {count}</p>
<Button onClick={increment} />
<button onClick={() => setOtherState(prev => !prev)}>Toggle</button>
</div>
)
}
export default Parent
What's Wrong Here?
Whenever
setOtherState
updates state, the parent re-renders.The
increment
function gets re-created because it's defined inside the parent component.Even though
Button
is wrapped withReact.memo
, it still re-renders because it receives a new function reference (onClick
).This defeats the purpose of
React.memo
, which is supposed to prevent re-renders when props don’t change.
Problem 2: Function Recreation Causes Unnecessary useEffect
Runs
If a function is inside the dependency array of useEffect
, and it gets re-created on every render, it will trigger the effect every time.
import React, { useState, useEffect } from "react"
const FetchData = () => {
const [data, setData] = useState<string | null>(null)
const fetchData = async () => {
const response = await fetch("https://jsonplaceholder.typicode.com/todos/1")
const result = await response.json()
setData(result.title)
}
useEffect(() => {
fetchData() // Function reference changes every render, causing unnecessary API calls!
}, [fetchData])
return <div>Data: {data}</div>
}
export default FetchData
What's Wrong Here?
fetchData
is inside the component, so it gets re-created on every render.Since it's in the
useEffect
dependency array, React thinks the function has changed and runs the effect again.This leads to unnecessary API calls on every render.
How useCallback
Solves Function Recreation
useCallback
is a React hook that memoizes a function, preventing it from being re-created on every render unless its dependencies change.
If dependencies remain the same, React returns the same function reference, preventing unnecessary re-renders.
import React, { useState, useEffect, useCallback } from "react"
const FetchData = () => {
const [data, setData] = useState<string | null>(null)
// Function is now memoized and will only change if setData changes
const fetchData = useCallback(async () => {
const response = await fetch("https://jsonplaceholder.typicode.com/todos/1")
const result = await response.json()
setData(result.title)
}, [])
useEffect(() => {
fetchData() // Now this only runs when needed
}, [fetchData])
return <div>Data: {data}</div>
}
export default FetchData
The API would have been called unnecessarily on every render because fetchData
gets re-created in every render without the useCallback
wrapper. Now, the API call runs only once because fetchData
reference doesn’t change on re-renders.
When It Needs to Re-create Functions
A function in a React component needs to be recreated when it depends on values that change over time. This ensures that the function always has access to the latest state or props.
const Counter = () => {
const [count, setCount] = useState(0)
const logCount = useCallback(() => {
console.log("Current count:", count) // Needs to be recreated to use the latest count
}, [count])
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(prev => prev + 1)}>Increment</button>
<button onClick={logCount}>Log Count</button>
</div>
)
}
If logCount
didn't update, it would log an old count value. Adding [count]
as a dependency ensures it re-creates when count
updates.
Subscribe to my newsletter
Read articles from Abeer Abdul Ahad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abeer Abdul Ahad
Abeer Abdul Ahad
I am a Full stack developer. Currently focusing on Next.js and Backend.