React Life Cycle and State
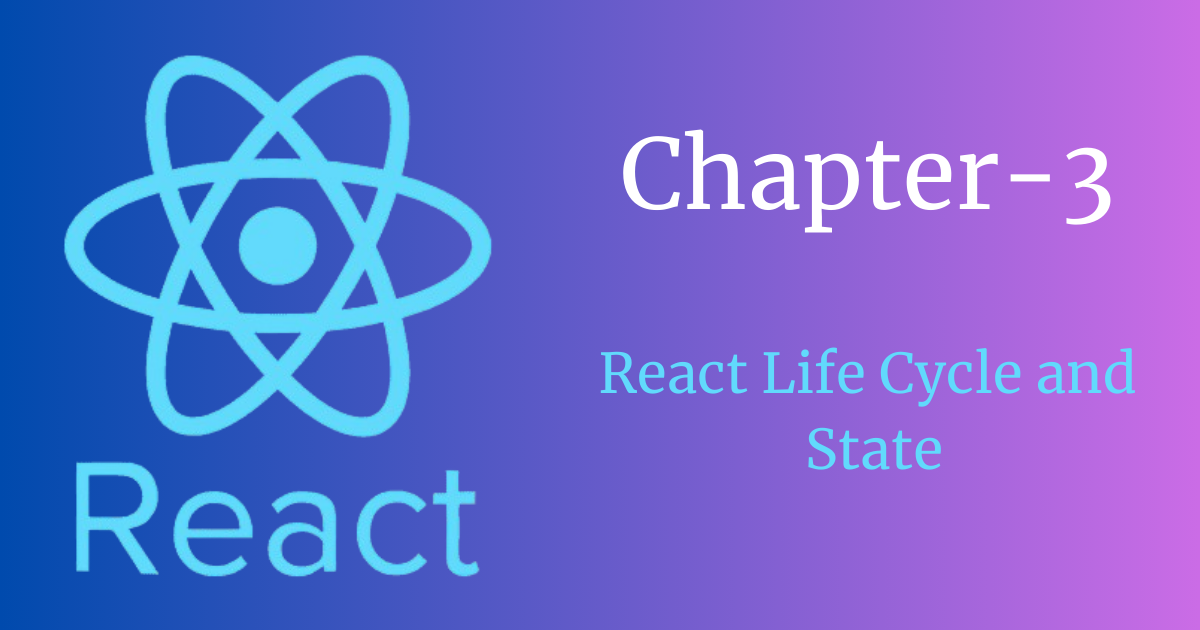
After learning what React is and how it works behind the scenes, in this blog, we will explore React's lifecycle and states.
So lets began…
What are Components?
In React, a component is an independent and reusable piece of UI. Components allow us to build complex user interfaces by composing them from smaller, isolated parts.
Types of Components in React
There are two primary types of components in React:
Functional Components
Class Components
Let's understand both types in detail.
Previously, only class components were used until a few years ago when functional components gained popularity, and now they are everywhere. The reason for this is state management in functional components, which we will learn about later in this blog. So first, let's dive into what these types of components are.
Class Components
Before React 16.8, class components were the go-to for handling state and lifecycle in a React component. Function components were seen as "stateless."
Class components are basically JavaScript classes that extend React.Component
. They were the main way to create stateful components in React before functional components and hooks became super popular.
Here’s a simple example:
// Defining a class component named 'Welcome' that extends the base 'Component' class.
// Class components are used when you need to manage state or use lifecycle methods.
class Welcome extends Component {
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h1> heading.
// The text inside the <h1> will be displayed on the screen.
return <h1>Hello, Let's learn React!</h1>;
}
}
// Exporting the 'Welcome' component as the default export of this module.
// This allows other files to import and use the 'Welcome' component.
export default Welcome;
Key Points:
Class components must extend
React.Component
orReact.PureComponent
.They must have a
render()
method that returns JSX.
Component Constructor
If your component has a constructor()
function, it gets called when the component is first created. This is where you set up the component's properties. In React, these properties are stored in an object called state
, which we'll explore more later in this tutorial.
The constructor is also where you respect the inheritance from the parent component by using the super()
statement. This runs the parent component's constructor function, giving your component access to all the functions of the parent component (React.Component
).
Example
Create a constructor function in the About component, and add a name property:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Defining a class component named 'About' that extends the base 'Component' class.
// Class components are used when you need to manage state or use lifecycle methods.
class About extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor() {
// 'super()' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component).
super();
// Initializing a property 'name' on the component instance.
// This is not state but a regular property of the class.
// Here, 'name' is an object with a key 'color' and value "Vaishnavi".
this.name = { firstName: "Vaishnavi" };
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h2> heading.
// The text inside the <h2> will be displayed on the screen.
return <h2>Let's Connect!</h2>;
}
}
// Exporting the 'About' component as the default export of this module.
// This allows other files to import and use the 'About' component.
export default About;
Props
Short form for “Properties” !
Another method for managing component properties in React is by using props
.
Unlike state, which is managed within the component, props
are external inputs that are passed into a component.
They function similarly to arguments in a function, allowing you to pass data from a parent component to a child component. This makes props
a powerful tool for creating dynamic and reusable components.
When you define a component, you can specify which props
it expects, and then you provide these props
as attributes when you use the component. This approach helps maintain a clear and organized flow of data throughout your application.
Example
Use an attribute to pass a name to the About component, and use it in the render() function:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'About' that extends the base 'Component' class.
// Class components are used when you need to manage state or use lifecycle methods.
class About extends React.Component {
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h2> heading.
// The text inside the <h2> includes a dynamic value from `this.props.name`.
// `this.props.name` is passed as a prop when the component is used.
return <h2>I am a {this.props.name}!</h2>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'About' component into the root.
// The 'name' prop is passed with the value "Vaishnavi".
// This will render the text "I am a Vaishnavi!" inside the <h2> element.
root.render(<About name="Vaishnavi" />);
Props in the Constructor
If your component has a constructor function, the props should always be passed to the constructor and also to the React.Component via the super()
method.
Example
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'About' that extends the base 'Component' class.
// Class components are used when you need to manage state or use lifecycle methods.
class About extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h2> heading.
// The text inside the <h2> includes a dynamic value from `this.props.name`.
// `this.props.name` is passed as a prop when the component is used.
return <h2>I am a {this.props.name}!</h2>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'About' component into the root.
// The 'name' prop is passed with the value "Vaishnavi".
// This will render the text "I am a Vaishnavi!" inside the <h2> element.
root.render(<About name="Vaishnavi" />);
Components in Components
Since components are reusable code, we can call a component within another component.
Example
Use the About component inside the Profile component:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'About' that extends the base 'Component' class.
// This component is a child component and will be used inside the 'Profile' component.
class About extends React.Component {
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h2> heading.
// The text inside the <h2> will be displayed on the screen.
return <h2>Let's Connect!</h2>;
}
}
// Defining a class component named 'Profile' that extends the base 'Component' class.
// This component is a parent component and will render the 'About' component inside it.
class Profile extends React.Component {
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which is a <div> containing:
// 1. An <h1> heading with the text "This is Profile Section".
// 2. The 'About' component, which renders its own <h2> heading.
return (
<div>
<h1>This is Profile Section</h1>
<About />
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Profile' component into the root.
// The 'Profile' component will render its own content as well as the 'About' component.
root.render(<Profile />);
React Class Component State
React class components have a built-in state
object.
The state
object is where you store property values that belong to the component. When the state
object changes, the component re-renders.
Creating the state Object
The state object is initialized in the constructor:
Example
Specify the state
object in the constructor method:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Defining a class component named 'About' that extends the base 'Component' class.
class About extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'name' with the value "Vaishnavi".
this.state = {
name: "Vaishnavi",
};
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading with the text "My Name".
// 2. A <p> element that displays the value of `this.state.name`.
return (
<div>
<h1>My Name</h1>
<p>{this.state.name}</p>
</div>
);
}
}
// Exporting the 'About' component as the default export of this module.
// This allows other files to import and use the 'About' component.
export default About;
The state object can contain as many properties as you like:
Example
Specify all the properties your component need:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Defining a class component named 'About' that extends the base 'Component' class.
class About extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has two properties: 'name' and 'age'.
this.state = {
name: "Vaishnavi",
age: "23",
};
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading with the text "Myself".
// 2. A <p> element that displays the value of `this.state.name`.
// 3. A <p> element that displays the value of `this.state.age`.
return (
<div>
<h1>Myself</h1>
<p>Name: {this.state.name}</p>
<p>Age: {this.state.age}</p>
</div>
);
}
}
// Exporting the 'About' component as the default export of this module.
// This allows other files to import and use the 'About' component.
export default About;
Changing the state
Object
To change a value in the state object, use the this.setState()
method.
When a value in the state
object changes, the component will re-render, meaning that the output will change according to the new value(s).
Example:
Add a button with an onClick
event that will change the color property:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Defining a class component named 'About' that extends the base 'Component' class.
class About extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has two properties: 'name' and 'age'.
this.state = {
name: "Vaishnavi",
age: "23",
};
}
// Method to update the 'name' in the state.
// This method is called when the button is clicked.
changeName = () => {
this.setState({ name: "Vaishnavi Dwivedi" });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.name`.
// 2. A <p> element that displays the value of `this.state.age`.
// 3. A button that, when clicked, calls the `changeName` method to update the name.
return (
<div>
<h1>My {this.state.name}</h1>
<p>And my age is {this.state.age} years.</p>
<button type="button" onClick={this.changeName}>
Change Name
</button>
</div>
);
}
}
// Exporting the 'About' component as the default export of this module.
// This allows other files to import and use the 'About' component.
export default About;
Explanation :
Constructor: The
constructor
initializes the component's state withname
set to"Vaishnavi"
andage
set to"23"
.State: The
state
is an object that holds data specific to the component. In this case, it containsname
andage
.changeName Method: The
changeName
method updates thename
property in the state usingthis.setState
. When called, it changes thename
to"Vaishnavi Dwivedi"
.Render Method:
The
render
method returns JSX that defines what the component will display.It includes:
An
<h1>
element that dynamically displays the value ofthis.state.name
.A
<p>
element that dynamically displays the value ofthis.state.age
.A button that, when clicked, calls the
changeName
method to update thename
.
Button:
The button has an
onClick
event handler that triggers thechangeName
method.The button text has been updated to
"Change Name"
for clarity.
Output:
<div>
<h1>My Vaishnavi</h1>
<p>And my age is 23 years.</p>
<button type="button">Change Name</button>
</div>
Lifecycle of Components
Each component in React has a lifecycle which you can monitor and manipulate during its three main phases.
The three phases are: Mounting, Updating, and Unmounting.
Mounting
Mounting means putting elements into the DOM.
React has four built-in methods that gets called, in this order, when mounting a component:
constructor()
getDerivedStateFromProps()
render()
componentDidMount()
The render()
method is required and will always be called, the others are optional and will be called if you define them.
constructor
The constructor()
method is the first thing that gets called when the component starts up. It's the perfect spot to set up the initial state
and any other starting values you need.
When you use the constructor()
, it comes with props
as arguments. Always begin by calling super(props)
. This kicks off the parent's constructor method and lets your component inherit methods from its parent (React.Component
).
Example:
The constructor
method is called, by React, every time you make a component:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h1> heading.
// The text inside the <h1> includes a dynamic value from `this.state.favoritecolor`.
return <h1>My Favorite Color is {this.state.favoritecolor}</h1>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
getDerivedStateFromProps
The getDerivedStateFromProps()
method is called just before rendering the element(s) in the DOM. It's the perfect spot to set up the state
object based on the initial props
.
This method takes state
as an argument and returns an object with any changes to the state
.
In the example below, we start with the favorite color as "red," but the getDerivedStateFromProps()
method updates the favorite color based on the favcol
attribute:
Example:
The getDerivedStateFromProps
method is called right before the render method:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `getDerivedStateFromProps` lifecycle method is called right before rendering.
// It allows the component to update its state based on changes in props.
// This method is static, so it does not have access to `this`.
// It takes two arguments: `props` (the updated props) and `state` (the current state).
// It returns an object to update the state or `null` to update nothing.
static getDerivedStateFromProps(props, state) {
// Updating the state with the value of the `favcol` prop.
return { favoritecolor: props.favcol };
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h1> heading.
// The text inside the <h1> includes a dynamic value from `this.state.favoritecolor`.
return <h1>My Favorite Color is {this.state.favoritecolor}</h1>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// The `favcol` prop is passed with the value "yellow".
// This will display the <h1> element with the text "My Favorite Color is yellow".
root.render(<Header favcol="yellow" />);
render
The render()
method is required, and is the method that actually outputs the HTML to the DOM.
Example:
A simple component with a simple render()
method:
class Header extends React.Component {
render() {
return (
<h1>This is the content of the Header component</h1>
);
}
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<Header />);
componentDidMount
The componentDidMount()
method gets called right after the component is rendered.
This is the perfect spot to run any code that needs the component to be in the DOM.
Example:
Initially, my favorite color is red, but just wait a moment, and it changes to yellow!
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `componentDidMount` lifecycle method is called after the component is mounted (inserted into the DOM).
// It is commonly used for side effects like fetching data, setting up subscriptions, or updating the state after a delay.
componentDidMount() {
// Using `setTimeout` to delay the execution of the code inside it by 1000 milliseconds (1 second).
setTimeout(() => {
// Updating the state using `this.setState`.
// This will change the `favoritecolor` property to "yellow".
this.setState({ favoritecolor: "yellow" });
}, 1000);
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h1> heading.
// The text inside the <h1> includes a dynamic value from `this.state.favoritecolor`.
return <h1>My Favorite Color is {this.state.favoritecolor}</h1>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
Updating
The next phase in the lifecycle is when a component gets updated.
A component updates whenever there's a change in its state
or props
.
React has five built-in methods that are called in this order when a component updates:
getDerivedStateFromProps()
shouldComponentUpdate()
render()
getSnapshotBeforeUpdate()
componentDidUpdate()
The render()
method is a must and will always be called, while the others are optional and will be called if you decide to define them.
getDerivedStateFromProps
When updates happen, the getDerivedStateFromProps
method is called first. This is the go-to spot for setting the state
object based on the initial props.
In the example below, there's a button that tries to change the favorite color to blue. But since the getDerivedStateFromProps()
method kicks in and updates the state with the color from the favoritecolor attribute, the favorite color stays yellow:
Example:
When the component updates, the getDerivedStateFromProps()
method is called:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `getDerivedStateFromProps` lifecycle method is called right before rendering.
// It allows the component to update its state based on changes in props.
// This method is static, so it does not have access to `this`.
// It takes two arguments: `props` (the updated props) and `state` (the current state).
// It returns an object to update the state or `null` to update nothing.
static getDerivedStateFromProps(props, state) {
// Updating the state with the value of the `favcol` prop.
return { favoritecolor: props.favcol };
}
// Method to update the favorite color in the state.
// This method is called when the button is clicked.
changeColor = () => {
this.setState({ favoritecolor: "blue" });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.favoritecolor`.
// 2. A button that, when clicked, calls the `changeColor` method to update the color.
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type="button" onClick={this.changeColor}>
Change color
</button>
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// The `favcol` prop is passed with the value "yellow".
// This will display the <h1> element with the text "My Favorite Color is yellow".
root.render(<Header favcol="yellow" />);
shouldComponentUpdate
In the shouldComponentUpdate()
method, you can return a Boolean value to decide if React should keep rendering or not.
By default, it's set to true
.
Check out the example below to see what happens when shouldComponentUpdate()
returns false
:
Example:
Prevent the component from rendering on any update:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `shouldComponentUpdate` lifecycle method is called before re-rendering the component.
// It determines whether the component should update or not.
// If it returns `false`, the component will not re-render, even if the state or props change.
shouldComponentUpdate() {
// Returning `false` to prevent the component from re-rendering.
return false;
}
// Method to update the favorite color in the state.
// This method is called when the button is clicked.
changeColor = () => {
// Updating the state using `this.setState`.
// This will change the `favoritecolor` property to "blue".
this.setState({ favoritecolor: "blue" });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.favoritecolor`.
// 2. A button that, when clicked, calls the `changeColor` method to update the color.
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type="button" onClick={this.changeColor}>
Change color
</button>
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
Example:
Same example as above, but this time the shouldComponentUpdate()
method returns true
instead:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `shouldComponentUpdate` lifecycle method is called before re-rendering the component.
// It determines whether the component should update or not.
// If it returns `true`, the component will re-render when the state or props change.
// If it returns `false`, the component will not re-render.
shouldComponentUpdate() {
// Returning `true` to allow the component to re-render.
return true;
}
// Method to update the favorite color in the state.
// This method is called when the button is clicked.
changeColor = () => {
// Updating the state using `this.setState`.
// This will change the `favoritecolor` property to "blue".
this.setState({ favoritecolor: "blue" });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.favoritecolor`.
// 2. A button that, when clicked, calls the `changeColor` method to update the color.
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type="button" onClick={this.changeColor}>
Change color
</button>
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
The render()
method is called whenever a component gets updated, so it can re-render the HTML to the DOM with the new changes.
In the example below, there's a button that changes the favorite color to blue:
getSnapshotBeforeUpdate
In the getSnapshotBeforeUpdate()
method, you can see the props
and state
as they were before the update. This means you can check the previous values even after the update happens.
If you use the getSnapshotBeforeUpdate()
method, make sure to include the componentDidUpdate()
method too, or you'll run into an error.
The example below might look tricky, but here's what it does:
When the component is first loaded, it shows the favorite color as "red."
Once the component is loaded, a timer kicks in and changes the state. After one second, the favorite color switches to "yellow."
This change starts the update phase. Since this component has a getSnapshotBeforeUpdate()
method, it runs and writes a message to the empty DIV1 element.
After that, the componentDidUpdate()
method runs and writes a message in the empty DIV2 element:
Example:
Try using the getSnapshotBeforeUpdate()
method to see what the state
object looked like before the update:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `componentDidMount` lifecycle method is called after the component is mounted (inserted into the DOM).
// It is commonly used for side effects like fetching data, setting up subscriptions, or updating the state after a delay.
componentDidMount() {
// Using `setTimeout` to delay the execution of the code inside it by 1000 milliseconds (1 second).
setTimeout(() => {
// Updating the state using `this.setState`.
// This will change the `favoritecolor` property to "yellow".
this.setState({ favoritecolor: "yellow" });
}, 1000);
}
// The `getSnapshotBeforeUpdate` lifecycle method is called right before the most recently rendered output is committed to the DOM.
// It allows the component to capture some information from the DOM before it is potentially changed.
// The return value of this method is passed as a parameter to `componentDidUpdate`.
getSnapshotBeforeUpdate(prevProps, prevState) {
// Updating the content of the div with ID "div1" to show the previous favorite color.
document.getElementById("div1").innerHTML =
"Before the update, the favorite was " + prevState.favoritecolor;
// Returning `null` because we don't need to use the snapshot in this example.
return null;
}
// The `componentDidUpdate` lifecycle method is called after the component is re-rendered.
// It is commonly used to perform side effects after the DOM has been updated.
componentDidUpdate() {
// Updating the content of the div with ID "div2" to show the updated favorite color.
document.getElementById("div2").innerHTML =
"The updated favorite is " + this.state.favoritecolor;
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.favoritecolor`.
// 2. A <div> with ID "div1" to display the previous favorite color.
// 3. A <div> with ID "div2" to display the updated favorite color.
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<div id="div1"></div>
<div id="div2"></div>
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
componentDidUpdate
The componentDidUpdate
method kicks in after the component updates in the DOM.
The example below might look a bit complex, but here's what happens:
When the component is mounting, it starts with the favorite color "red".
Once the component is mounted, a timer changes the state, turning the color to "yellow".
This change triggers the update phase, and because this component has a componentDidUpdate
method, it runs and writes a message in the empty DIV element:
Example:
The componentDidUpdate
method is called after the update is shown in the DOM:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Header' that extends the base 'Component' class.
class Header extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'favoritecolor' with the value "red".
this.state = {
favoritecolor: "red",
};
}
// The `componentDidMount` lifecycle method is called after the component is mounted (inserted into the DOM).
// It is commonly used for side effects like fetching data, setting up subscriptions, or updating the state after a delay.
componentDidMount() {
// Using `setTimeout` to delay the execution of the code inside it by 1000 milliseconds (1 second).
setTimeout(() => {
// Updating the state using `this.setState`.
// This will change the `favoritecolor` property to "yellow".
this.setState({ favoritecolor: "yellow" });
}, 1000);
}
// The `componentDidUpdate` lifecycle method is called after the component is re-rendered.
// It is commonly used to perform side effects after the DOM has been updated.
componentDidUpdate() {
// Updating the content of the div with ID "mydiv" to show the updated favorite color.
document.getElementById("mydiv").innerHTML =
"The updated favorite is " + this.state.favoritecolor;
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. An <h1> heading that displays the value of `this.state.favoritecolor`.
// 2. A <div> with ID "mydiv" to display the updated favorite color.
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<div id="mydiv"></div>
</div>
);
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Header' component into the root.
// This will display the <h1> element with the text "My Favorite Color is red".
root.render(<Header />);
Unmounting
The next phase in the lifecycle is when a component is taken out of the DOM, or as React calls it, unmounting.
React provides just one built-in method for when a component is unmounted: componentWillUnmount()
componentWillUnmount
The componentWillUnmount
method is called right before the component is removed from the DOM.
Example:
Click the button to remove the header:
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Importing ReactDOM to render the component into the DOM.
import ReactDOM from 'react-dom/client';
// Defining a class component named 'Container' that extends the base 'Component' class.
class Container extends React.Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'show' with the value `true`.
this.state = {
show: true,
};
}
// Method to update the 'show' property in the state.
// This method is called when the button is clicked.
delHeader = () => {
// Updating the state using `this.setState`.
// This will change the `show` property to `false`.
this.setState({ show: false });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Declaring a variable `myheader` to conditionally render the `Child` component.
let myheader;
if (this.state.show) {
// If `this.state.show` is `true`, assign the `Child` component to `myheader`.
myheader = <Child />;
}
// Returning a JSX element, which in this case is a <div> containing:
// 1. The `myheader` variable, which conditionally renders the `Child` component.
// 2. A button that, when clicked, calls the `delHeader` method to hide the `Child` component.
return (
<div>
{myheader}
<button type="button" onClick={this.delHeader}>
Delete Header
</button>
</div>
);
}
}
// Defining a class component named 'Child' that extends the base 'Component' class.
class Child extends React.Component {
// The `componentWillUnmount` lifecycle method is called just before the component is unmounted (removed from the DOM).
// It is commonly used for cleanup tasks like canceling network requests or invalidating timers.
componentWillUnmount() {
// Displaying an alert to indicate that the component is about to be unmounted.
alert('The component named Header is about to be unmounted.');
}
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is an <h1> heading.
return <h1>Hello World!</h1>;
}
}
// Creating a root for the React application using ReactDOM.createRoot.
// The root is attached to the DOM element with the ID 'root'.
const root = ReactDOM.createRoot(document.getElementById('root'));
// Rendering the 'Container' component into the root.
// This will display the `Child` component and a button to delete it.
root.render(<Container />);
State in Class Components
State is a crucial concept in React. It allows components to manage data internally.
// Importing React and Component from the 'react' library.
// React is necessary to write JSX, and Component is the base class for class components.
import React, { Component } from 'react';
// Defining a class component named 'Counter' that extends the base 'Component' class.
class Counter extends Component {
// The constructor method is called when an instance of the component is created.
// It is used to initialize state or bind methods.
constructor(props) {
// 'super(props)' must be called before accessing 'this' in the constructor.
// It calls the constructor of the parent class (Component) and passes the props.
super(props);
// Initializing the component's state.
// The state is an object that holds data specific to this component.
// Here, the state has a property 'count' with the initial value `0`.
this.state = {
count: 0,
};
}
// Method to increment the count in the state.
// This method is called when the button is clicked.
increment = () => {
// Updating the state using `this.setState`.
// This will increment the `count` property by 1.
this.setState({ count: this.state.count + 1 });
};
// The 'render' method is a required method in class components.
// It returns the JSX that will be rendered to the DOM.
render() {
// Returning a JSX element, which in this case is a <div> containing:
// 1. A <p> element that displays the value of `this.state.count`.
// 2. A button that, when clicked, calls the `increment` method to increment the count.
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
// Exporting the 'Counter' component as the default export of this module.
// This allows other files to import and use the 'Counter' component.
export default Counter;
Key Points:
State is an object declared in the constructor.
this.setState()
is used to update state and trigger re-renders.
Lifecycle Methods
Class components have lifecycle methods that let you hook into different stages of the component’s life.
Lifecycle Method | Phase | Purpose |
constructor() | Mounting | Initialize state, bind methods |
getDerivedStateFromProps() | Mounting & Updating | Sync state with props |
render() | Mounting & Updating | Returns JSX to be displayed |
componentDidMount() | Mounting | Run side effects (API calls) |
shouldComponentUpdate() | Updating | Control re-rendering |
getSnapshotBeforeUpdate() | Updating | Capture values before update |
componentDidUpdate() | Updating | Run side effects after the update |
componentWillUnmount() | Unmounting | Cleanup before unmounting |
Handling Events
Class components handle events using this
binding.
class Clicker extends Component {
handleClick = () => {
alert('Button clicked!');
};
render() {
return <button onClick={this.handleClick}>Click Me</button>;
}
}
Functional Components
A functional component is simply a JavaScript function that returns JSX (JavaScript XML) to render UI elements. Unlike class components, functional components do not have their own this
context and rely on hooks for managing state and lifecycle methods.
Basic Syntax of a Functional Component
function Greeting() {
return <h1>Hello, World!</h1>;
}
or using an arrow function:
const Greeting = () => <h1>Hello, World!</h1>;
Rendering a Functional Component
You can use this component inside another component:
function App() {
return (
<div>
<Greeting />
</div>
);
}
Features | Class Components | Functional Components |
State Initialization | constructor() | useState() |
Lifecycle Methods | componentDidMount(), shouldComponentUpdate(), componentDidUpdate(), componentWillUnmount() | useEffect() handles mounting, updating, and unmounting |
Handling Updates | shouldComponentUpdate(), componentDidUpdate() | useEffect() with dependency array |
Cleanup | componentWillUnmount() | Return cleanup function in useEffect() |
Functionality | Component methods tied to lifecycle phases | Hooks like useState, useEffect, useCallback, useMemo |
Advantages of Functional Components:
Simpler and more readable.
Easier to test and debug.
Better performance since they do not involve the overhead of a class instance.
Can use React Hooks like
useState
anduseEffect
.
How Functional Components Work
Let’s break down how a functional component works step by step.
Receiving Props
Props (short for properties) are the inputs passed to a functional component. They are immutable and provide data that a component needs to render itself.
How props work:
Props are passed as attributes when calling the component.
They are received as an object in the component function.
We can destructure them for cleaner access.
Example:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default function App() {
return <Greeting name="Vaishnavi" />;
}
How this works:
<Greeting name="Vaishnavi" />
passesname
as a prop.props
is an object:{ name: "Vaishnavi" }
.The component accesses
props.name
and renders it.Output: "Hello, Vaishnavi!".
Returning JSX
Functional components return JSX, which looks like HTML but is actually JavaScript. React uses this JSX to describe what the UI should look like. JSX gets transpiled into JavaScript using Babel behind the scenes.
Example with JSX:
function UserProfile(props) {
return (
<div>
<h2>{props.username}</h2>
<p>Age: {props.age}</p>
</div>
);
}
export default function App() {
return <UserProfile username="Vaishnavi" age={25} />;
}
How JSX works:
JSX expressions:
{}
allow embedding JavaScript within the markup.JSX elements are converted into React.createElement() calls by Babel.
React builds a virtual DOM tree based on this structure.
Before React Hooks, only class components could manage state. But with useState
, functional components can now manage local state efficiently. Let’s break down how state management works.
What is Hook?
A React Hook is a handy function from the React library that lets developers use state and other cool React features in functional components.
Before hooks came along, functional components couldn't manage state or use lifecycle methods, which made them less capable than class components. But now, with hooks, you can manage state and use lifecycle features right inside functional components, making them much more powerful and flexible.
What does a Hook do?
A React Hook is a special function that lets you tap into React features and lifecycle methods right inside functional components. With hooks, you can use state, handle side effects, access context, and do other tasks that used to be possible only with class components.
Why Hooks were introduced?
Hooks were added in React 16.8 to solve some problems and make functional components better.
Before Hooks, functional components couldn't have their own state or handle side effects, which made them less useful. Developers often had to change functional components into class components to use state or lifecycle methods. Hooks fixed this by letting functional components have state and manage side effects, making them more powerful and flexible.
Also, sharing stateful logic between class components was tricky and often required complex patterns like render props and higher-order components (HOCs), which made the code harder to read.
Hooks make it easier to reuse and organize code by allowing stateful logic to be put into reusable functions. They also make the code simpler and cleaner, removing the complexity of class components and the need for the this
keyword.
By keeping related logic together in custom hooks, Hooks make the codebase easier to maintain and understand. Plus, Hooks are backwards-compatible, meaning you can start using them gradually. Existing class components can stay the same, while new components can be built with Hooks, making the transition smoother.
Now…
State Management in Functional Component
The useState
Hook
useState
lets functional components store and update state.
// Importing React and the `useState` hook from the 'react' library.
// `useState` is a hook that allows functional components to manage state.
import React, { useState } from 'react';
// Defining a functional component named 'Counter'.
function Counter() {
// Using the `useState` hook to create a state variable `count` and its setter function `setCount`.
// The initial value of `count` is set to `0`.
const [count, setCount] = useState(0);
// Function to increment the `count` state.
// This function is called when the button is clicked.
const increment = () => {
// Updating the `count` state using the `setCount` function.
// This will increment the `count` by 1.
setCount(count + 1);
};
// The return statement defines the JSX that will be rendered to the DOM.
return (
<div>
{/* Displaying the current value of `count` inside a <p> element. */}
<p>Count: {count}</p>
{/* A button that, when clicked, calls the `increment` function to update the `count`. */}
<button onClick={increment}>Increment</button>
</div>
);
}
// Exporting the `Counter` component as the default export of this module.
// This allows other files to import and use the `Counter` component.
export default Counter;
How useState
works:
useState
initializescount
to0
.setCount
updatescount
and triggers a re-render.The new
count
value gets reflected in the UI.
Lifecycle of a Functional Component
In class components, lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
manage component behavior. Functional components don’t have lifecycle methods, but React Hooks like useEffect
replicate their behavior.
Functional Component Lifecycle with Hooks
Class Component Lifecycle | Equivalent Hook (useEffect ) | When it Runs |
componentDidMount | useEffect(() => {}, []) | After the initial render |
componentDidUpdate | useEffect(() => {}, [state]) | After state/props change |
componentWillUnmount | useEffect(() => { return () => {} }, []) | Cleanup on component unmount |
Example:
// Import React and necessary hooks (useState and useEffect) from the 'react' library
import React, { useState, useEffect } from 'react';
// Define a functional component named Timer
function Timer() {
// Declare a state variable `time` and a function `setTime` to update it
// Initialize `time` with a default value of 0
const [time, setTime] = useState(0);
// useEffect hook to handle side effects (e.g., setting up a timer)
// The empty dependency array `[]` means this effect runs only once, when the component mounts
useEffect(() => {
// Log a message to the console when the component mounts
console.log("Component mounted");
// Set up an interval that increments the `time` state by 1 every second (1000ms)
const interval = setInterval(() => {
// Use the functional form of `setTime` to ensure the latest state value is used
setTime((prev) => prev + 1);
}, 1000);
// Return a cleanup function that runs when the component unmounts
return () => {
// Log a message to the console when the component unmounts
console.log("Component unmounted");
// Clear the interval to prevent memory leaks and stop the timer
clearInterval(interval); // Cleanup
};
}, []); // Empty dependency array ensures this effect runs only on mount and unmount
// Another useEffect hook to log the updated `time` value whenever it changes
// The dependency array `[time]` means this effect runs whenever `time` is updated
useEffect(() => {
// Log the current value of `time` to the console
console.log("Time updated:", time);
}, [time]); // Dependency array with `time` ensures this effect runs on `time` changes
// Render the component's UI
return <h1>Time: {time}s</h1>;
}
// Export the Timer component as the default export of this module
export default Timer;
How this works:
useEffect(() => {}, [])
: Logs when the component mounts.setInterval()
: Updatestime
every second.return () => {}
: Cleanup function clears the interval when the component unmounts.useEffect(() => {}, [time])
: Logs whentime
changes.
Explanation of each step:
useState
:Used to declare and manage state in functional components.
time
is the state variable, andsetTime
is the function to update it.The initial value of
time
is set to0
.
useEffect
:Used to perform side effects in functional components.
The first
useEffect
sets up a timer when the component mounts and cleans it up when the component unmounts.The second
useEffect
logs the updatedtime
value whenever it changes.
Dependency Array (
[]
or[time]
):An empty array
[]
means the effect runs only once (on mount and unmount).An array with
[time]
means the effect runs whenevertime
changes.
Cleanup Function:
- Returned by the first
useEffect
to clear the interval and prevent memory leaks when the component unmounts.
- Returned by the first
Functional Update in
setTime
:setTime((prev) => prev + 1)
ensures the latest state value is used, avoiding potential issues with stale state.
Example Output in Console:
When the component mounts:
Component mounted
Every second:
Time updated: 1 Time updated: 2 Time updated: 3 ...
When the component unmounts:
Component unmounted
Component Mounts
|
v
useEffect (Mount) -> Set Interval -> Update `time` every second
|
v
useEffect (Update) -> Log `time` on every change
|
v
Component Unmounts -> Cleanup Interval
Re-Rendering in Functional Components
A re-render happens when:
State changes (
setState
)Props change
A parent component re-renders
How React minimizes re-rendering:
Diffing algorithm: Only changed elements are updated in the real DOM.
Component memoization:
React.memo()
prevents unnecessary re-renders.
Complete Lifecycle Flow in Functional Components
Let’s summarize the full lifecycle flow:
1 Component renders with initial props and state
️2 Virtual DOM created and compared with real DOM
3 Real DOM updated efficiently (only changed parts)
4 useEffect runs for side effects (like fetching data)
5 State changes → triggers re-render
6 Virtual DOM updated → diffing → real DOM update
7 Cleanup on unmount (like clearing intervals or subscriptions)
Pure Components
Though after the popularity of functional components, the class component lost its value, so did the pure component, but let's cover it to have a better understanding of React components.
When working with React, performance optimization is often crucial, especially as your application grows. One of the simplest and most effective tools for improving performance is the React Pure Component.
What is a React Pure Component?
A React Pure Component is a special kind of component that helps avoid unnecessary re-renders. It works by doing a quick check on the component’s state and props. If nothing has changed, the component won’t re-render, which saves time and resources.
To put it simply, a Pure Component only re-renders when the data it relies on actually changes.
Here’s how you create a Pure Component:
import React, { PureComponent } from 'react';
class MyPureComponent extends PureComponent {
render() {
console.log('MyPureComponent rendered');
return <div>{this.props.message}</div>;
}
}
export default MyPureComponent;
In this example, MyPureComponent
will only re-render if props.message
changes. If the same value is passed again, it won’t render.
Difference Between React Component and Pure Component
Feature | Component | PureComponent |
Re-rendering | Always re-renders | Only re-renders when state or props change |
Comparison Type | No comparison | Shallow comparison |
Performance | May cause unnecessary re-renders | Optimized by skipping identical updates |
A shallow comparison checks if the references of objects or primitives have changed—not their contents. This makes Pure Components efficient but also means they may not detect deep changes in objects or arrays.
When to Use Pure Components (Though not in use anymore)
Stateless components with simple props: If your component’s state and props change predictably, a Pure Component can prevent wasteful renders.
Performance-sensitive parts of your app: Use them for frequently updated, simple components.
Avoid with deep objects: Since shallow comparisons don’t detect deep changes, avoid Pure Components when working with deeply nested objects unless you manage changes carefully.
Potential Pitfalls
Mutability issues: Pure Components rely on shallow comparison, so if you mutate objects directly, the component won’t see a change and won’t re-render.
Overhead with complex data structures: Shallow comparison might miss nested changes, so be cautious when using arrays or objects.
I know I started by saying there are just two types of components, and here we are learning about a fourth type, but just like Pure components, let's cover HOC or High Order Components for a basic understanding of React.
Higher-Order Components (HOCs)
A Higher-Order Component (HOC) is a function that takes a component and returns a new component. It’s a pattern for reusing component logic in a clean and composable way. HOCs are not part of the React API but are a pattern that leverages React’s compositional model.
For functional components, a HOC is simply a function that wraps a component and returns a new functional component.
Why Use HOCs with Functional Components?
a. Code Reusability : HOCs allow you to extract shared logic (e.g., data fetching, authentication, logging) into a single place. This reduces duplication and makes your codebase easier to maintain.
b. Separation of Concerns : By separating logic (e.g., state management, side effects) from presentation, HOCs make your components more focused and easier to test.
c. Cross-Cutting Concerns
HOCs are ideal for handling cross-cutting concerns like:
Authentication
Logging
Error handling
Performance tracking
d. Props Manipulation
HOCs can:
Inject additional props into the wrapped component.
Modify or filter existing props.
Conditionally render components based on certain conditions.
How to Create a HOC for Functional Components
Creating a HOC for functional components is straightforward. Here’s the basic structure:
import React from 'react';
const withHOC = (WrappedComponent) => {
return (props) => {
// Add logic here
return <WrappedComponent {...props} />;
};
};
Example: Basic HOC
Here’s a simple HOC that logs the component’s name when it mounts:
const withLogger = (WrappedComponent) => {
return (props) => {
React.useEffect(() => {
console.log(`Component ${WrappedComponent.name} mounted`);
}, []);
return <WrappedComponent {...props} />;
};
};
// Usage
const MyComponent = ({ message }) => {
return <div>{message}</div>;
};
const EnhancedComponent = withLogger(MyComponent);
const App = () => {
return <EnhancedComponent message="Hello, HOC!" />;
};
export default App;
Practical Examples of HOCs for Functional Components
a. Authentication HOC
Protect routes or components that require authentication.
const withAuth = (WrappedComponent) => {
return (props) => {
const [isAuthenticated, setIsAuthenticated] = React.useState(false);
React.useEffect(() => {
const checkAuth = async () => {
const authStatus = await checkAuthStatus(); // Assume this checks authentication
setIsAuthenticated(authStatus);
};
checkAuth();
}, []);
if (!isAuthenticated) {
return <div>Please log in to view this page.</div>;
}
return <WrappedComponent {...props} />;
};
};
// Usage
const ProtectedComponent = withAuth(MyComponent);
b. Loading State HOC
Handle loading states for components that fetch data.
const withLoading = (WrappedComponent) => {
return (props) => {
const [isLoading, setIsLoading] = React.useState(true);
const [data, setData] = React.useState(null);
React.useEffect(() => {
const fetchData = async () => {
const result = await fetchDataFromAPI(); // Assume this fetches data
setData(result);
setIsLoading(false);
};
fetchData();
}, []);
if (isLoading) {
return <div>Loading...</div>;
}
return <WrappedComponent data={data} {...props} />;
};
};
// Usage
const DataComponent = withLoading(MyComponent);
c. Styling HOC
Inject styles or class names into a component.
const withStyles = (WrappedComponent, styles) => {
return (props) => {
return <WrappedComponent {...props} style={styles} />;
};
};
// Usage
const StyledComponent = withStyles(MyComponent, { color: 'red' });
d. Error Boundary HOC
Catch errors in the component tree and display a fallback UI.
const withErrorBoundary = (WrappedComponent) => {
return (props) => {
const [hasError, setHasError] = React.useState(false);
React.useEffect(() => {
const errorHandler = (error) => {
console.error("Error caught by HOC:", error);
setHasError(true);
};
window.addEventListener('error', errorHandler);
return () => window.removeEventListener('error', errorHandler);
}, []);
if (hasError) {
return <div>Something went wrong.</div>;
}
return <WrappedComponent {...props} />;
};
};
// Usage
const SafeComponent = withErrorBoundary(MyComponent);
Best Practices for Using HOCs with Functional Components
a. Don’t Mutate the Wrapped Component
Always return a new component instead of modifying the original component. This ensures that the original component remains pure and reusable.
b. Pass Unrelated Props Through
Use the spread operator ({...props}
) to pass all unrelated props to the wrapped component. This ensures that the HOC doesn’t interfere with the component’s expected behavior.
c. Use Display Names for Debugging
Set a display name for the enhanced component to make debugging easier.
const withLogger = (WrappedComponent) => {
const WithLogger = (props) => {
React.useEffect(() => {
console.log(`Component ${WrappedComponent.name} mounted`);
}, []);
return <WrappedComponent {...props} />;
};
WithLogger.displayName = `WithLogger(${WrappedComponent.name})`;
return WithLogger;
};
d. Avoid Using HOCs Inside Render
Creating HOCs inside the render
method can lead to performance issues and unexpected behavior. Always define HOCs outside of components.
Alternatives to HOCs: Custom Hooks
With the introduction of React Hooks, many use cases for HOCs can now be handled more elegantly using custom hooks. Custom hooks allow you to encapsulate reusable logic in a function.
Example: Custom Hook for Data Fetching
const useDataFetching = (url) => {
const [data, setData] = React.useState(null);
const [isLoading, setIsLoading] = React.useState(true);
React.useEffect(() => {
const fetchData = async () => {
const result = await fetch(url).then((response) => response.json());
setData(result);
setIsLoading(false);
};
fetchData();
}, [url]);
return { data, isLoading };
};
// Usage
const MyComponent = ({ url }) => {
const { data, isLoading } = useDataFetching(url);
if (isLoading) return <div>Loading...</div>;
return <div>{JSON.stringify(data)}</div>;
};
Now, here ends the section on components. Let's dive deep into props. We already covered some basics of props above, but now let's dive deeper. I will use functional components only, as these are the only ones used.
Let's begin…
Understanding Props
Props (short for properties) are read-only attributes that are passed from parent to child components. They allow components to be reusable and dynamic.
Example of Passing Props:
function Welcome(props) {
return <h1>Welcome, {props.username}!</h1>;
}
export default function App() {
return <Welcome username="Vaishnavi" />;
}
Key Characteristics of Props:
Immutable: Props cannot be modified inside the component.
Parent-to-Child Communication: Data is passed from a parent component to a child component.
Reusable: Props help in making components reusable.
Default Props
Sometimes, you may want to provide default values for props in case they are not passed by the parent component. You can do this using the defaultProps
property.
Example:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Greeting.defaultProps = {
name: "Guest",
};
function App() {
return (
<div>
<Greeting /> {/* Renders "Hello, Guest!" */}
<Greeting name="Alice" /> {/* Renders "Hello, Alice!" */}
</div>
);
}
Prop Validation with prop-types
Prop validation ensures that the props passed to a component are of the expected type and shape. React provides a library called prop-types
for this purpose.
Installation:
npm install prop-types
Basic Usage:
import PropTypes from 'prop-types';
function UserProfile(props) {
return (
<div>
<h1>{props.name}</h1>
<p>Age: {props.age}</p>
<p>Occupation: {props.occupation}</p>
</div>
);
}
UserProfile.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
occupation: PropTypes.string,
};
function App() {
return (
<UserProfile name="John Doe" age={30} occupation="Software Engineer" />
);
}
Common Prop Types:
PropTypes.string
PropTypes.number
PropTypes.bool
PropTypes.array
PropTypes.object
PropTypes.func
PropTypes.node
(anything that can be rendered)PropTypes.element
(a React element)
Custom Validators:
You can define custom validation functions for props.
UserProfile.propTypes = {
age: function(props, propName, componentName) {
if (props[propName] < 18) {
return new Error(
`Invalid prop ${propName} supplied to ${componentName}. Age must be at least 18.`
);
}
},
};
Shape Validation:
UserProfile.propTypes = {
user: PropTypes.shape({
name: PropTypes.string,
age: PropTypes.number,
occupation: PropTypes.string,
}),
};
Array of Specific Types:
UserProfile.propTypes = {
hobbies: PropTypes.arrayOf(PropTypes.string),
};
TypeScript for Prop Validation
TypeScript provides static type checking, which can be used for prop validation in React components. This is a more robust and scalable solution compared to prop-types
.
Example:
// Define an interface `UserProfileProps` to describe the shape of the props
// that the `UserProfile` component expects.
interface UserProfileProps {
name: string; // `name` is a required string prop
age: number; // `age` is a required number prop
occupation?: string; // `occupation` is an optional string prop (denoted by `?`)
}
// Define the `UserProfile` functional component.
// It uses object destructuring to extract `name`, `age`, and `occupation` from the `props` object.
// The `UserProfileProps` interface ensures that the component receives the correct types for its props.
function UserProfile({ name, age, occupation }: UserProfileProps) {
return (
<div>
{/* Render the `name` prop in an <h1> tag */}
<h1>{name}</h1>
{/* Render the `age` prop in a <p> tag */}
<p>Age: {age}</p>
{/* Render the `occupation` prop in a <p> tag.
If `occupation` is not provided, display a default value of 'Not specified'. */}
<p>Occupation: {occupation || 'Not specified'}</p>
</div>
);
}
// Define the `App` component, which is the root component of the application.
function App() {
return (
// Render the `UserProfile` component and pass the required props.
<UserProfile
name="John Doe" // Pass a string value for `name`
age={30} // Pass a number value for `age`
occupation="Software Engineer" // Pass a string value for `occupation`
/>
);
}
Explanation of Key Concepts
1. TypeScript Interface (UserProfileProps
):
An interface in TypeScript is used to define the shape of an object. In this case, it describes the props that the
UserProfile
component expects.name: string
: Thename
prop is required and must be a string.age: number
: Theage
prop is required and must be a number.occupation?: string
: Theoccupation
prop is optional (denoted by?
) and must be a string if provided.
2. Functional Component (UserProfile
):
The
UserProfile
component is a functional component that accepts props of typeUserProfileProps
.Destructuring Props: The props are destructured directly in the function signature for cleaner and more readable code.
tsx
Copy
function UserProfile({ name, age, occupation }: UserProfileProps)
Default Value for Optional Prop: The
occupation
prop is optional, so a default value ('Not specified'
) is provided using the||
operator.
3. Rendering the Component:
The component renders the
name
,age
, andoccupation
props in a structured way using JSX.If
occupation
is not provided, it defaults to'Not specified'
.
4. Parent Component (App
):
The
App
component is the root component that renders theUserProfile
component.Props are passed to
UserProfile
as attributes in JSX:tsx
Copy
<UserProfile name="John Doe" age={30} occupation="Software Engineer" />
Benefits of Using TypeScript for Props
Type Safety:
- TypeScript ensures that the correct types are passed as props. For example, if you try to pass a string for
age
, TypeScript will throw an error at compile time.
- TypeScript ensures that the correct types are passed as props. For example, if you try to pass a string for
Autocompletion and Intellisense:
- When using TypeScript, your IDE (e.g., VSCode) will provide autocompletion and suggestions for the props, making development faster and less error-prone.
Optional Props:
- TypeScript allows you to define optional props (e.g.,
occupation?: string
), making your components more flexible.
- TypeScript allows you to define optional props (e.g.,
Documentation:
- The interface serves as self-documenting code, making it clear what props the component expects and their types.
Example Output
When the App
component is rendered, the output will look like this:
<div>
<h1>John Doe</h1>
<p>Age: 30</p>
<p>Occupation: Software Engineer</p>
</div>
If the occupation
prop is not provided:
<UserProfile name="Jane Doe" age={25} />
The output will be:
<div>
<h1>Jane Doe</h1>
<p>Age: 25</p>
<p>Occupation: Not specified</p>
</div>
Diagram: Component and Props Flow
App Component
|
v
Pass Props -> UserProfile Component
|
v
Props Validation (TypeScript Interface)
|
v
Render JSX with Props
Best Practices for Props
Always Validate Props: Use
prop-types
or TypeScript to validate props and catch errors early.Use Default Props: Provide default values for optional props to make your component more robust.
Keep Props Read-Only: Never modify props directly in the child component.
Document Props: Use comments or tools like Storybook to document the expected props for your components.
Use TypeScript for Large Projects: For larger projects, consider using TypeScript for better type safety and scalability.
Subscribe to my newsletter
Read articles from Vaishnavi Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by