Why Your JavaScript Is Probably Too Slow: The Async Revolution You're Missing Out On

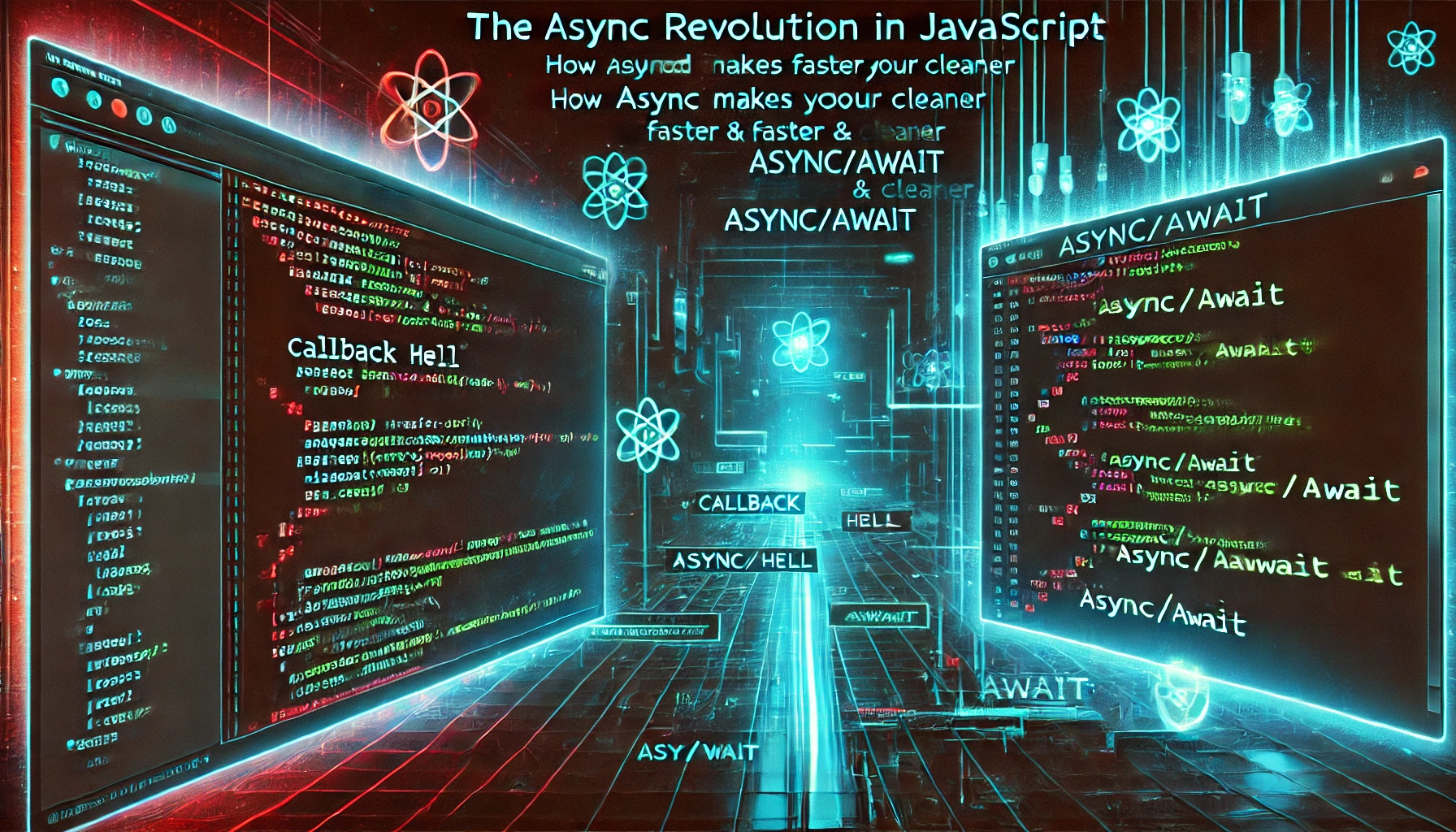
Ever been on a website that freezes when you click a button? Or watched that spinning wheel of death as your app struggles to load data? Chances are, someone didn't understand asynchronous JavaScript. And honestly, I didn't either for the longest time. The journey from callback spaghetti to the elegant async/await syntax we have today is nothing short of a programming revolution.
Let me take you on a journey through the time-bending world of asynchronous JavaScript—from the chaotic days of callback hell to the promised land of... well, Promises.
Why We Can't Just Write Code Line by Line
Here's the thing—JavaScript runs on a single thread. Yep, just one. Imagine having only one worker trying to handle everything in a busy restaurant: taking orders, cooking food, serving tables, and washing dishes. Something's gotta give.
If we tried to run everything synchronously (one task after another), we'd end up with frozen interfaces and frustrated users while our code waits for slow operations like:
Network requests that might take seconds
Database queries
File operations
User input
Let's visualize the problem:
// This is synchronous code - it blocks execution!
console.log("Starting to fetch data...");
const data = fetchDataFromServer(); // Imagine this takes 3 seconds
console.log("Got data!"); // This won't run until those 3 seconds are over
processData(data);
updateUI();
During those 3 seconds, nothing else happens. The browser freezes. Users rage-click. Chaos ensues.
Enter the Event Loop: JavaScript's Secret Sauce
Did you know JavaScript's event loop wasn't even part of the original ECMAScript specification? It was actually implemented by browsers as a way to handle user interactions and other events.
The event loop is JavaScript's elegant solution to this single-thread problem. Here's how it works:
+-------------+ +-------------+ +-----------------+
| | | | | |
| Call Stack | | Web APIs | | Callback Queue |
| | | | | |
+------+------+ +------+------+ +--------+--------+
^ | ^
| | |
| v |
+-------------------------------------------+
|
+------+------+
| Event Loop |
+-------------+
The Call Stack: Where your synchronous code runs, one function at a time
Web APIs: Browser-provided features that handle time-consuming tasks without blocking
Callback Queue: Where completed tasks wait for their turn to run
Event Loop: The bouncer that checks if the call stack is empty and moves callbacks from the queue to the stack
The beauty of this system? Your code keeps running while time-consuming operations happen in the background. When those operations finish, their callbacks join the queue and execute when the stack is free.
Callback Hell: The Problem That Haunted Developers
In the early days, we used callbacks for everything. A callback is just a function that runs after something else finishes.
// The infamous callback pattern
getData(function(data) {
processData(data, function(processedData) {
saveData(processedData, function(savedResult) {
updateUI(savedResult, function() {
notifyUser(function() {
// Dear god, make it stop
});
});
});
});
});
Ever tried reading this kind of code? It's like trying to follow a conversation where everyone is interrupting each other. We called this "callback hell" or the "pyramid of doom" - and for good reason!
Fun fact: The term "callback hell" became so infamous that it spawned an entire website (callbackhell.com) dedicated to helping developers avoid it.
Promises: A Ray of Hope
Around 2015, Promises became a native part of JavaScript. They weren't a new concept (libraries like Q and Bluebird had implemented them before), but their standardization changed everything.
A Promise is essentially an IOU - "I promise to give you a result eventually." It can be in one of three states:
Pending: Still working on it
Fulfilled: Got the result successfully
Rejected: Failed to get the result
// The same operations using promises
getData()
.then(data => processData(data))
.then(processedData => saveData(processedData))
.then(savedResult => updateUI(savedResult))
.then(() => notifyUser())
.catch(error => handleError(error));
Much cleaner, right? The linear flow makes it easier to understand what's happening in sequence.
Here's how Promises work under the hood:
+----------------+ +-----------------+ +----------------+
| | | | | |
| Create Promise +--->+ Pending +--->+ Fulfilled |
| | | | | |
+----------------+ +--------+--------+ +----------------+
|
|
v
+----------------+
| |
| Rejected |
| |
+--------+-------+
|
v
+----------------+
| |
| .then/.catch |
| |
+----------------+
But wait, we can make it even better...
Async/Await: The Promised Land
In 2017, async/await syntax landed in JavaScript (ES8), and it was like putting icing on the Promise cake. It lets us write asynchronous code that looks synchronous:
async function fetchAndProcessData() {
try {
console.log("Starting to fetch data...");
// This looks synchronous but doesn't block execution!
const data = await fetchDataFromServer();
console.log("Got data!");
const processedData = await processData(data);
const savedResult = await saveData(processedData);
await updateUI(savedResult);
await notifyUser();
return "All done!";
} catch (error) {
handleError(error);
}
}
// Don't forget, we still need to call our async function
fetchAndProcessData().then(message => console.log(message));
Here's a secret most tutorials won't tell you: async/await is just syntactic sugar over Promises. Under the hood, it's doing the same thing, but the code is far more readable.
Here's a visual comparison of how the different approaches work:
CALLBACK HELL | PROMISES | ASYNC/AWAIT
--------------------- | --------------------- | ---------------------
getData() { | getData() | async function() {
processData() { | .then(process) | const data = await getData()
saveData() { | .then(save) | await processData(data)
updateUI() { | .then(update) | await saveData()
notify() | .then(notify) | try/catch for errors
} | .catch(error) | }
} | |
} | |
} | |
Real-World Async: Fetching Data from an API
Let's put all this theory into practice. Here's a real example of fetching weather data:
// The old way (callbacks)
function getWeatherData(city, callback) {
const xhr = new XMLHttpRequest();
xhr.open('GET', `https://api.weather.com/v1/${city}`);
xhr.onload = function() {
if (xhr.status === 200) {
callback(null, JSON.parse(xhr.responseText));
} else {
callback(new Error('Request failed'));
}
};
xhr.onerror = function() {
callback(new Error('Network error'));
};
xhr.send();
}
getWeatherData('london', function(error, data) {
if (error) {
console.error('Could not get weather:', error);
return;
}
console.log('Weather data:', data);
});
Now, let's see the same operation with Promises:
// The better way (Promises)
function getWeatherData(city) {
return fetch(`https://api.weather.com/v1/${city}`)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
});
}
getWeatherData('london')
.then(data => console.log('Weather data:', data))
.catch(error => console.error('Could not get weather:', error));
And finally, with async/await:
// The best way (async/await)
async function getWeatherData(city) {
try {
const response = await fetch(`https://api.weather.com/v1/${city}`);
if (!response.ok) {
throw new Error('Network response was not ok');
}
return await response.json();
} catch (error) {
console.error('Could not get weather:', error);
throw error; // Re-throw to let caller handle it if needed
}
}
// Using the function
async function displayWeather() {
try {
const data = await getWeatherData('london');
console.log('Weather data:', data);
// Update UI with weather data
} catch (error) {
// Show error message to user
}
}
displayWeather();
Did you notice how each version gets progressively more readable? The async/await version practically reads like synchronous code but without any of the blocking behavior.
Error Handling: The Unsung Hero of Async JavaScript
One of the most underappreciated aspects of modern asynchronous JavaScript is proper error handling. In the callback days, we had to manually check for errors in each callback. With Promises, we got the .catch()
method. With async/await, we can use familiar try/catch blocks.
Here's a pro tip most tutorials miss: always handle your errors at the appropriate level. Sometimes that means catching and logging them locally, other times it means letting them bubble up to a central error handler.
// A practical error handling pattern
async function fetchUserData(userId) {
try {
const response = await fetch(`/api/users/${userId}`);
// Check for HTTP errors (which don't trigger catch blocks by default)
if (!response.ok) {
// Create a custom error with status information
const error = new Error(`API error: ${response.status}`);
error.status = response.status;
throw error;
}
return await response.json();
} catch (error) {
// You could handle specific errors differently
if (error.status === 404) {
console.log(`User ${userId} not found`);
return null; // Return a safe default
}
// For other errors, re-throw to be handled by caller
throw error;
}
}
The Future of Async JavaScript
JavaScript's async journey isn't over. New patterns and APIs continue to emerge. Have you heard about the Observable pattern? It's like Promises but for handling multiple values over time rather than just one. Think of them as "streams of data" rather than single results.
Or what about the newer AbortController API for cancelling fetch requests? Ever had a user navigate away from a page while data was still loading? That's where cancellation becomes crucial.
const controller = new AbortController();
const signal = controller.signal;
fetch('/api/large-data', { signal })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => {
if (error.name === 'AbortError') {
console.log('Fetch was cancelled');
} else {
console.error('Error:', error);
}
});
// Cancel the fetch if it takes too long
setTimeout(() => controller.abort(), 5000);
Final Thoughts: Embracing the Async Mindset
Asynchronous programming isn't just a technique – it's a mindset. Once you start thinking in terms of operations that can run independently of your main code flow, you'll write more responsive, efficient applications.
Remember these key takeaways:
JavaScript's single-threaded nature makes async programming essential
Callbacks worked but led to messy, hard-to-maintain code
Promises brought structure and chainability to async operations
Async/await gives us the best of both worlds: clean, synchronous-looking code with asynchronous benefits
Error handling is just as important as the happy path
The next time you're building a web application, ask yourself: "Could this operation block my UI?" If the answer is yes, reach for async patterns. Your users will thank you, even if they never know why your app feels so much smoother than the others.
What's your biggest async JavaScript challenge? Drop a comment below—I'd love to help troubleshoot some real-world problems!
Subscribe to my newsletter
Read articles from Jatin Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
