Debouncing and Throttling

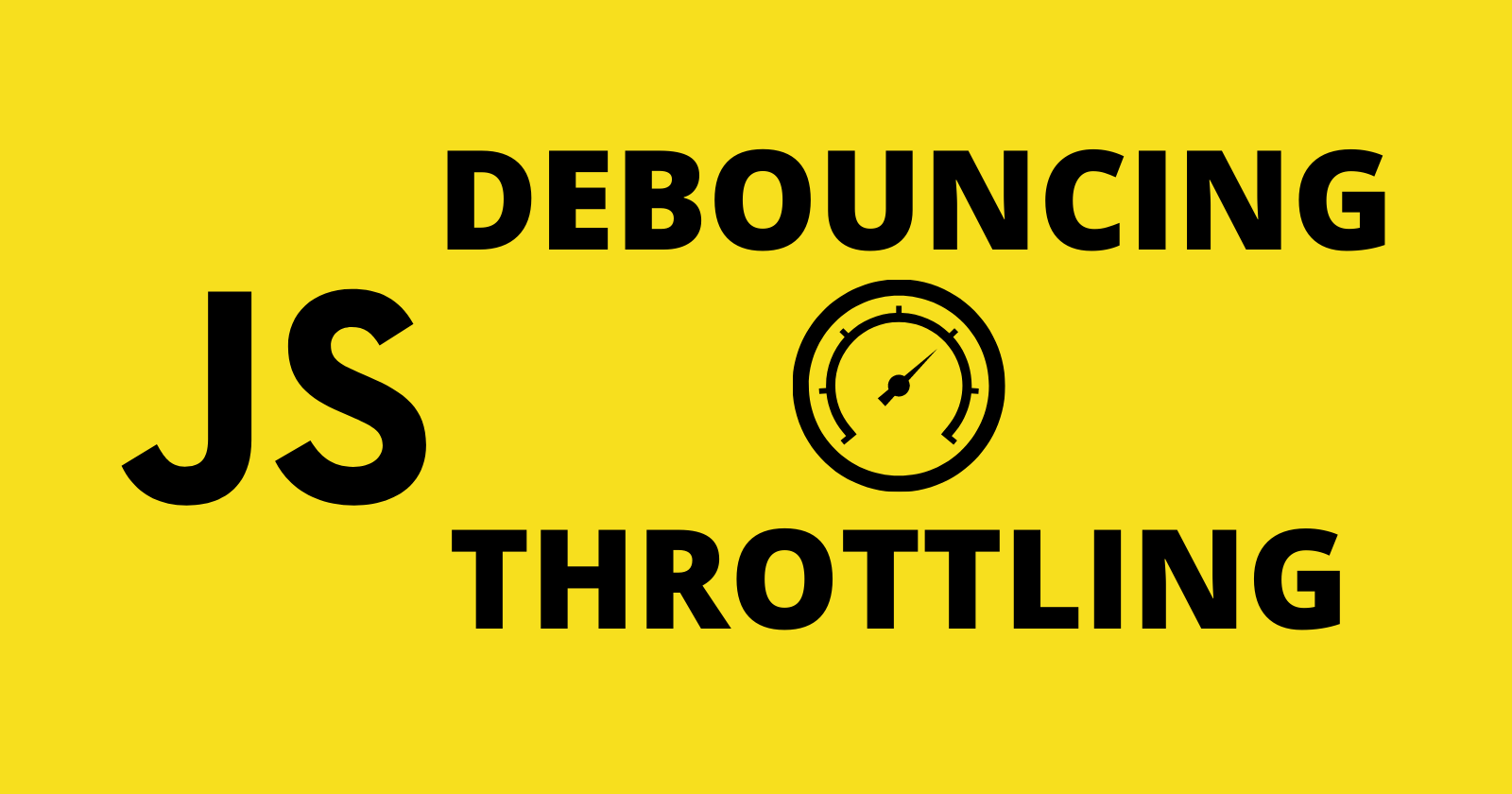
These are techniques used to control how frequently a function executes, particularly with events that can fire rapidly (like scrolling, resizing, or typing). Both help optimize performance and prevent excessive function calls, but they work differently:
Debouncing
Debouncing ensures a function runs only after a certain period of inactivity.
It's like waiting until someone completely stops talking before you respond.
How it works:
User triggers an event (e.g., types in a search box)
A timer starts
If another event occurs before the timer ends, the timer resets
The function only executes after the timer completes without interruption
Common use cases:
Events where you only care about the final state, like:
Search input (wait until user stops typing)
Window resizing (update layout only after resizing stops)
Form validation as users type
Simple JavaScript implementation
function debounce(func, wait) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => {
func.apply(this, args);
}, wait);
};
}
// Usage
const debouncedSearch = debounce(searchFunction, 500);
searchInput.addEventListener('input', debouncedSearch);
Throttling
Throttling limits how often a function can execute, allowing it to run at most once within a specified time interval.
It's like speaking once every 30 seconds regardless of how much information you have.
Throttling creates an intentional bottleneck for function execution, deliberately limiting how frequently a function can run regardless of how often it's triggered.
Like a bottleneck in a physical pipe that restricts flow to a controlled rate, throttling restricts the execution flow of functions to a predetermined frequency.
How it works:
First event triggers the function immediately
Subsequent events within the time interval are ignored
After the interval passes, the next event will trigger the function again
Common use cases:
Regular updates during continuous events: Likewise,
Scrolling (update UI elements while scrolling)
Game input (process movement at consistent intervals)
Tracking mouse position
Simple JavaScript implementation
function throttle(func, limit) {
let inThrottle = false;
return function(...args) {
if (!inThrottle) {
func.apply(this, args);
inThrottle = true;
setTimeout(() => {
inThrottle = false;
}, limit);
}
};
}
// Usage
const throttledScroll = throttle(handleScroll, 300);
window.addEventListener('scroll', throttledScroll);
Comparing Debounce vs. Throttle
Debounce: Waits until activity stops before executing (good for "completion" events)
Throttle: Executes regularly during continuous activity (good for "during" events)
To visualize: If you rapidly press a button 10 times:
A debounced function might execute just once (after you stop)
A throttled function might execute 3-4 times (at regular intervals)
That’s all for now!
Subscribe to my newsletter
Read articles from Shivam Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivam Verma
Shivam Verma
Hello, I am Shivam Verma a software builder and fullstack developer with a passion for converting complex ideas into robust applications