TanStack Query Firebase v1.0.7 for React: What’s New and How to Use It

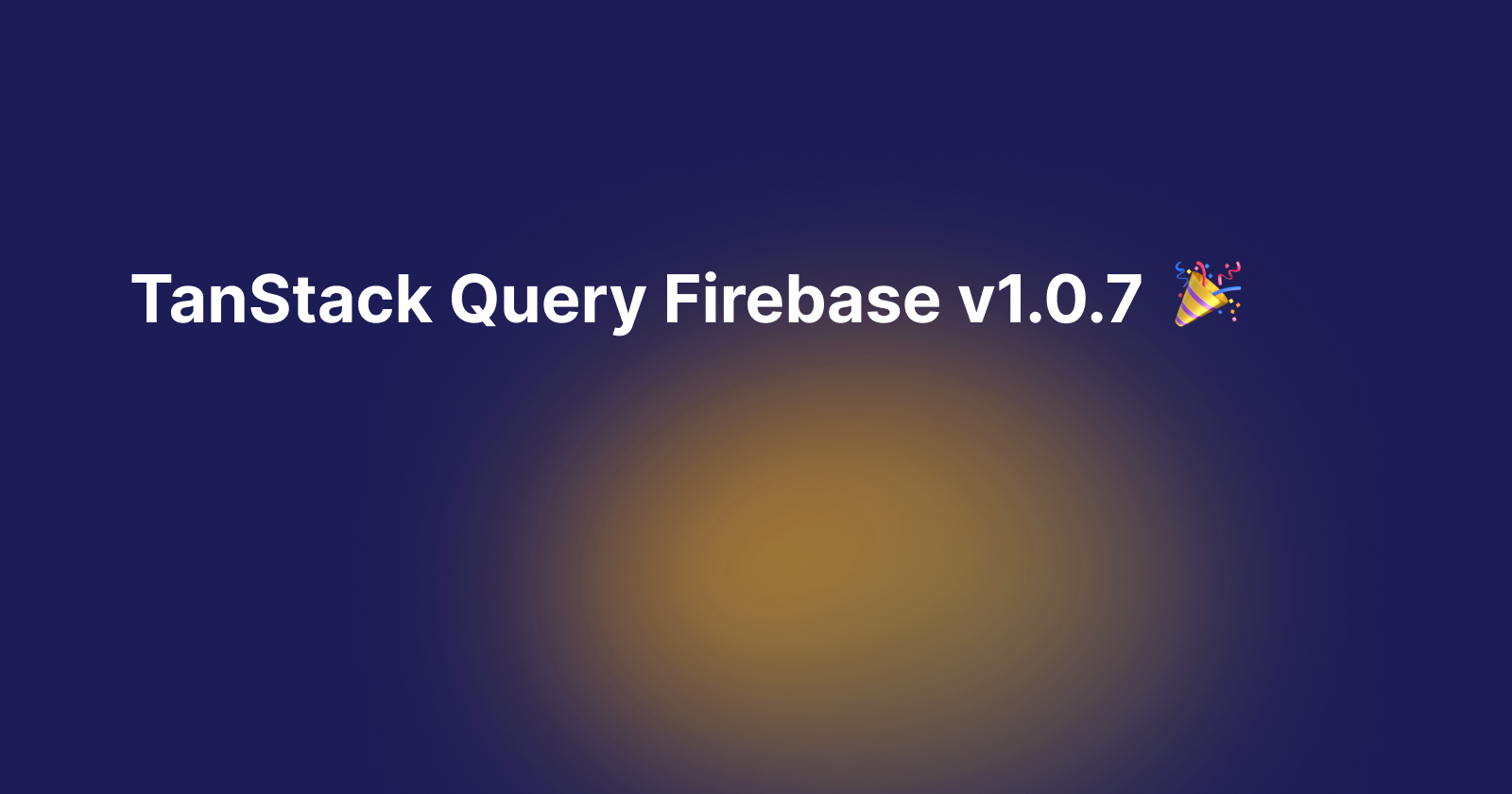
TanStack Query Firebase is a powerful library that provides a set of hooks for handling asynchronous tasks with Firebase in your applications. This library simplifies real-time data fetching, caching, and syncing data in your applications.
On 7th March, 2025, a new release, v1.0.7 was published. With the release of v1.0.7, the library introduces new react hooks. In this post, we'll explore what's new in v1.0.7, how to set up the library in a React project, and practical usage examples.
What’s New in v1.0.7?
We've added more hooks for react, making it easier to manage data insertions, updates and deletion operations to Cloud Firestore. The new hooks include:
useAddDocumentMutation
: Add a new document to specified CollectionReference with the given data, assigning it a document ID automatically.useUpdateDocumentMutation
: Updates fields in the document referred to by the specified DocumentReference. The update will fail if applied to a document that does not exist.useSetDocumentMutation
: Writes to the document referred to by this DocumentReference. If the document does not yet exist, it will be created.
Getting Started with TanStack Query Firebase in React
1. Install Dependencies
To use TanStack Query Firebase, install the required packages:
npm install @tanstack/react-query firebase @tanstack/query-firebase/react
2. Setup Firebase and TanStack Query
Configure Firebase in your project:
import { getApps, initializeApp } from "firebase/app";
import { getFirestore } from "firebase/firestore";
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID",
};
const firebaseApp = getApps().length === 0 ? initializeApp(firebaseConfig) : getApps()[0];
export const db = getFirestore(firebaseApp);
Now, wrap your app with QueryClientProvider:
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
const queryClient = new QueryClient();
function App() {
return (
<QueryClientProvider client={queryClient}>
<MyComponent />
</QueryClientProvider>
);
}
export default App;
Using TanStack Query Firebase v1.0.7 in React
Mutating Firestore Data
import { useSetDocumentMutation } from "@tanstack-query-firebase/react/firestore";
import { collection, doc } from "firebase/firestore";
import { db } from "./firebase";
import { useState } from "react";
type Expense = {
amount: number;
category: string;
date: string;
description: string;
};
export default function CreateExpense() {
const createExpenseMutation = useSetDocumentMutation(doc(collection(db, "expenses")));
const [expense, setExpense] = useState<Expense>({
amount: 0,
category: "",
date: "",
description: "",
});
function handleChange(e: React.ChangeEvent<HTMLInputElement | HTMLSelectElement>) {
setExpense({ ...expense, [e.target.name]: e.target.value });
}
async function handleSubmit(e: React.FormEvent) {
e.preventDefault();
try {
await createExpenseMutation.mutate(expense);
setExpense({
amount: 0,
category: "",
date: "",
description: "",
});
} catch (error) {
console.error("Error adding expense:", error);
}
}
return (
<div>
<form onSubmit={handleSubmit}>
<div>
<input
name="amount"
value={expense.amount}
onChange={handleChange}
type="number"
required
/>
</div>
<div>
<select
value={expense.category}
onChange={handleChange}
name="category"
required
>
<option value={""}>Select Category</option>
<option value={"food"}>Food</option>
<option value={"transport"}>Transport</option>
<option value={"internet"}>Internet</option>
</select>
</div>
<div>
<input
name="date"
value={expense.date}
onChange={handleChange}
type="date"
required
/>
</div>
<div>
<input
name="description"
value={expense.description}
onChange={handleChange}
type="text"
required
/>
</div>
<button type="submit">
{createExpenseMutation.isPending ? "Submitting" : "Submit"}
</button>
</form>
</div>
);
};
Conclusion
TanStack Query Firebase v1.0.7 brings react hooks to super charge your Firebase applications.
By leveraging TanStack Query Firebase, you get a declarative, efficient, and scalable way to manage real-time data fetching and mutations in your frontend apps.
🚀 Give it a try and upgrade your Firebase queries and mutations with TanStack Query Firebase!
References
Subscribe to my newsletter
Read articles from Hassan Bahati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
