Design A Low-Cost Staycation with AI Agents & Free Tools
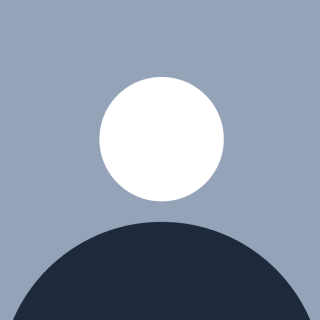
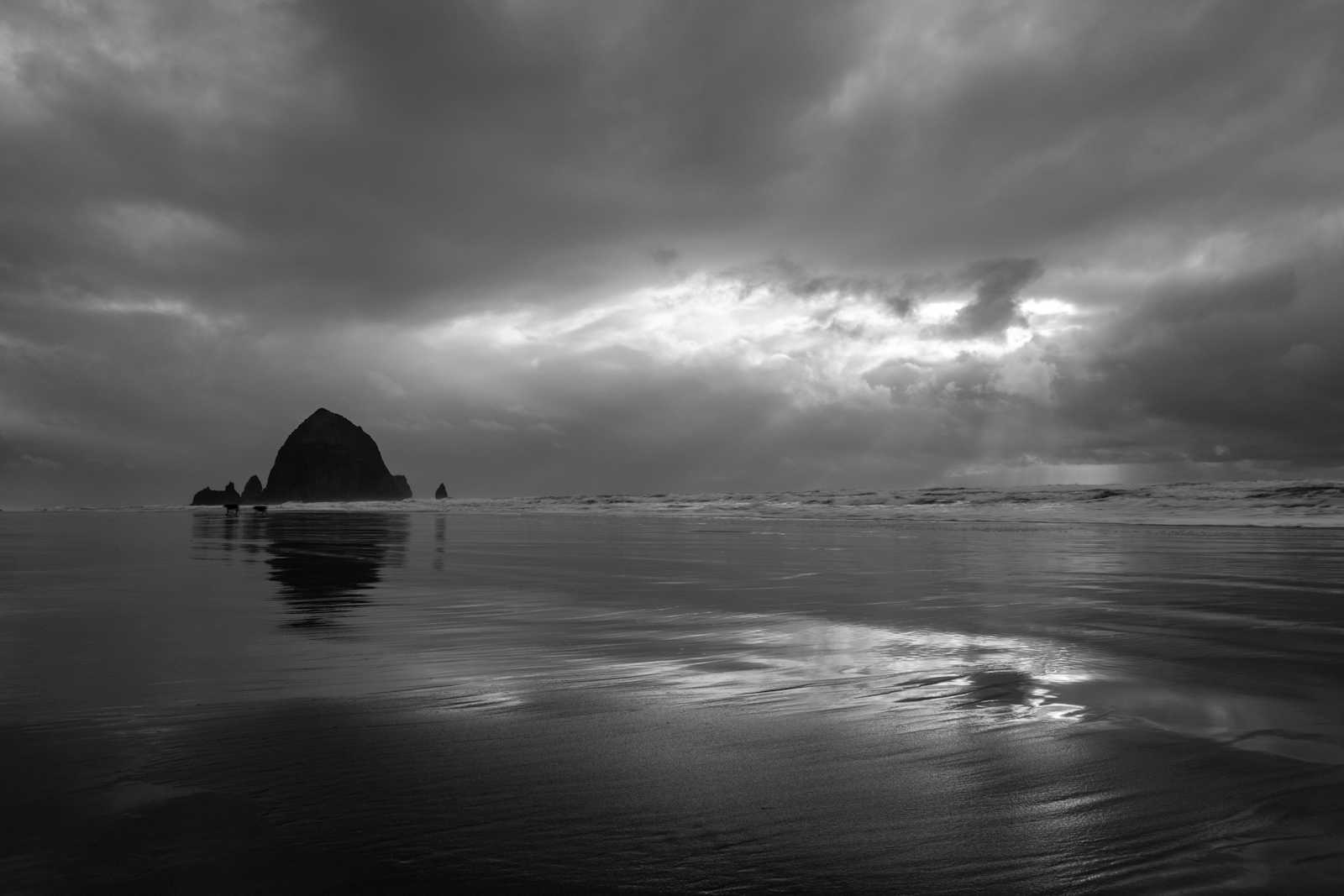
Since it is almost Spring Break, I was curious to see if I could build a little AI agentic travel agency to take a vacation to a beautiful beach, not to some tropical Hawaiian island, but to a cold, subtropical, cheap and mostly rainy Oregon coast.
Because there is nothing to make you feel more alive than having cold wind whipping surf, sand and rain in your face.
Ahah, the natural exfoliation — It’s a 2 for 1 deal, an inexpensive getaway plus an invigorating, free spa treatment! Good times!
I've been wanting to get into machine learning for a while. So when I came across a video on YouTube about how to create AI agents and how to extend a LLM by giving them tools (aka Python libraries) to do things that it could otherwise not do, for example: scrape a website, do math better or search the internet for you.
I decided to look into DeepLearning.ai and finished the CrewAI course last weekend.
Some folks do a Netflix binge on the weekend, while other weirdos binge on learning how to create AI agents to make them do things they don’t want or don’t have time to do — and yes, I’m part of the latter.
Basically, what you do is that you let the AI agents solve more complex problems by allowing them to run in a loop. You give them a task, a backstory, and some tools to work with that allows each to make decisions on how to act and which tools to use to solve their particular task, then you let them decide how to use the tools.
And if you have more than one AI agent, you can have them work together to accomplish a set of complex tasks — an agentic crew!
Most tutorials and courses use OpenAI as the LLM and this one was no expectation. But since I really can’t afford to waste money on the many calls I will be making just to test this out, I decided to use Llama 3.2 for my LLM.
Llama is open-source (aka free) and I can run it locally. I also used Jupyter Notebook, because I wanted to step through the code, test and make changes as I needed to.
Libraries
Here are the libraries I used:
from crewai import Agent, Crew, Task, LLM
import os
from crewai.tools import BaseTool
from langchain_community.tools import DuckDuckGoSearchRun
from crewai_tools import ScrapeWebsiteTool
from pydantic import BaseModel
from IPython.display import Markdown
import json
from pprint import pprint
LLM
I used Ollama to bring in the Llama language model and ran it locally:
#LLM Object from crewai package
ollama_llm=LLM(model="ollama/llama3.2", base_url="http://localhost:11434")
Tools
The course used Serper, a Google search API, while it does a give great search results and a lot of free credits to work with before you have to pay, I wanted to avoid burning through all my Serper free credits.
I decided to hack a custom search tool that uses DuckDuckgo instead and is provided by LangChain — Plue it’s free!
class MyCustomDuckDuckGoTool(BaseTool):
name: str = "DuckDuckGo Search Tool"
description: str = "Search the web for a given query."
def _run(self, query: str) -> str:
# Ensure the DuckDuckGoSearchRun is invoked properly.
duckduckgo_tool = DuckDuckGoSearchRun()
response = duckduckgo_tool.invoke(query)
return response
def _get_tool(self):
# Create an instance of the tool when needed
return MyCustomDuckDuckGoTool()
Then, initialize both the native CrewAI and custom tools:
# Initialize the tools
search_tool = MyCustomDuckDuckGoTool()
scrape_tool = ScrapeWebsiteTool()
Create the Agents
I have 3 AI agents for my vacation planning crew:
Travel Advisor
Fueling Guru
Tour Guide
# Agent 1: Travel advisor
travel_advisor = Agent(
role="Travel Advisor",
goal="Use search to find lodging under budget at destination.",
tools=[search_tool, scrape_tool],
verbose=True,
llm=ollama_llm,
max_iterations=8,
max_time=240,
backstory=(
"Proven experience finding lodging"
"and for organizing travel for personal use."
"With a keen sense of finding lodging"
"on the cheap, you are very resourceful "
"and skilled in finding and securing "
"the perfect lodging that fits within budget constraints."
)
)
# Agent 2: Fueling Agent
gas_guru = Agent(
role='Fueling Agent',
goal=(
"Identify the cheapest gas and charging stations "
"along the driving route"
),
tools=[search_tool, scrape_tool],
llm=ollama_llm,
max_iterations=8,
max_time=240,
verbose=True,
backstory=(
"Uses the location "
"to define the route from origin to destination. "
"Searches for cheapest gas price and charging sources "
"Provides 2-3 gas and charging stop options with location, "
"price per gallon, and estimated cost."
)
)
# Agent 3: Tour Guide Agent
tour_guide = Agent(
role="Tour Guide",
goal="Curate a list of free sights and activities "
"near the selected lodging location."
"building the trip’s core plan.",
tools=[search_tool, scrape_tool],
llm=ollama_llm,
max_iterations=8,
max_time=240,
verbose=True,
backstory=(
"Creative and communicative, "
"you craft activities that are zero-cost "
"to keep the budget intact."
"Uses the shared location and "
"gathers details via web search "
"to find 3-5 free sights or events "
"near lodging location."
)
)
Build a Pydantic Object
I created a class LodgingDetails
using a Pydantic BaseModel, because I wanted to see if the Travel Agent would create a JSON output of the lodging information that it recommended.
from pydantic import BaseModel
# Define a Pydantic model for venue details
# (demonstrating Output as Pydantic)
class LodgingDetails(BaseModel):
lodging: str
lodging_url: str
city: str
price: int
Create the Crew’s Tasks
The crew has to output either JSON or Markdown files upon completion of their tasks.
lodging_task = Task(
description="Find {lodging} in {destination_city}, {destination_state} "
"that meets criteria for under {budget} per night.",
expected_output="All the details of a specifically chosen "
"lodging you found to accommodate the client. "
"Choose the location with the cheapest lodging you can find.",
# human_input=True,
output_json=LodgingDetails,
output_file="lodging_details.json", # Outputs the lodging details as a JSON file
agent=travel_advisor
)
logistics_task = Task(
description="Coordinate traveling and "
"find charging or gas stations between "
"{origin_city}, {origin_state} and "
"{destination_city}, {destination_state} "
"with {budget} in mind "
"on {tentative_date} of travel.",
expected_output="Report route options, gas stations"
"and charging stations locations along route "
"formatted as markdown.",
# async_execution=True,
context=[lodging_task],
output_file="fueling_details.md", # Outputs the lodging details as a markdown file
agent=gas_guru
tour_task = Task(
description="Find activities, points of interest "
"or free events to attend for {event_topic} "
"during stay at {destination_city}, {destination_state}.",
expected_output="Report on points of interest, free activites "
"and events formatted as markdown.",
async_execution=True,
output_file="points_interest_report.md", # Outputs free activities as a markdown file
context=[lodging_task, logistics_task],
agent=tour_guide
)
Run the Crew
I set the inputs for the crew to work from:
event_details = {
'event_topic': "Staycation",
'event_details':"A quick family vacation "
"roadtrip to the Oregon Coast.",
'lodging':"hotel",
'origin_city': "Portland",
'origin_state': "Oregon",
'destination_city': "Cannon Beach",
'destination_state': "Oregon",
'tentative_date': "2025-03-15",
'expected_traveler_count': 3,
'budget': 200
}
And then let them do the work:
result = travel_agency_crew.kickoff(inputs=event_details)
Display Generated Files
For outputting the JSON file:
with open('lodging_details.json') as f:
data = json.load(f)
pprint(data)
Output:
{
'city': 'Cannon Beach, Oregon',
'lodging': 'Tolovana Inn',
'lodging_url': 'https://www.tolovanainn.com/',
'price': 192
}
For the Markdown files:
Markdown("fueling_details.md")
**Route Recommendations from Portland, Oregon to Cannon Beach, Oregon**
The most scenic drive from Portland to Cannon Beach is through bike. However, for a more convenient option, we can consider taking the daily bus service or train.
1. **Bus Service**: The daily bus service from Portland to Cannon Beach takes 2 hours and costs $10-$20.
- Bus Station: Portland (Various locations)
- Departure Time: 6am-8pm
- Arrival Time: 8am-10am
2. **Train Service**: The train ride from Portland to Cannon Beach takes 12-13 hours and costs $50-$100.
- Train Station: Portland (Union Station)
- Departure Time: Various times throughout the day
- Arrival Time: Various times throughout the day
3. **Driving**: The driving route is through Highway 26 West to 101 North or if traffic is bad, take I-5 North to Longview, Washington and crossing the bridge back over to Oregon's Highway 30 West.
- Distance: 95 miles
- Drive Time: 2 hours
**Gas Stations along Route**
| Station Name | Location | Price per Gallon |
| --- | --- | --- |
| Chevron | Portland, OR (1221 NW 22nd Ave) | $3.77 |
| Chevron | Portland, OR (1141 SW Broadway) | $3.73 |
| Shell | Cannon Beach, OR (1110 Hemlock St) | $3.83 |
| Mobil | Seaside, OR (1700 S Pacific Way) | $3.67 |
| Costco Gasoline | Clackamas, OR (13130 SE 84th Ave) | $3.62 |
**Charging Stations along Route**
| Station Name | Location | Type | Cost per Hour |
| --- | --- | --- | --- |
| Electrify America | Portland, OR (2000 NE Martin Luther King Jr Blvd) | DC Fast Charging | $0.25 |
| EVgo | Cannon Beach, OR (1111 Hemlock St) | Level 2 Charging | $0.15 |
| ChargePoint | Seaside, OR (1700 S Pacific Way) | Level 2 Charging | $0.12 |
**Hotel Recommendation**
* Tolovana Inn at Cannon Beach: Starting at $170 per night
Note: Prices are subject to change and may vary depending on the time of year and availability.
Markdown("points_interest_report.md")
### Free Activities and Events in Cannon Beach, Oregon
#### Highly Rated Attractions
* **Hike Cape Falcon Trail**: A 4.5-mile out & back trail with a moderate difficulty level and an elevation gain of 580 feet.
* **Oswald West State Park**: A park featuring hiking trails, scenic views, and historic sites.
* **Arcadia Beach State Recreation Site**: A beach with tide pools, picnic areas, and scenic views.
* **Haystack Rock**: A iconic sea stack with stunning views and a popular spot for photography.
#### Free Events
* **Cannon Beach Sandcastle Contest** (annual event): A contest where participants build sandcastles on the beach.
* **4th of July Celebration** (annual event): A celebration with fireworks, live music, and food vendors.
* **Arts Festivals**: Various festivals throughout the year celebrating local art and culture.
* **Writers Read Celebration**: A free event featuring poetry readings and performances.
* **Line Dancing Night at Ashtown Brewing**: A fun night of line dancing with a 70s, 80s, and 90s band.
#### Other Activities
* **Build a bonfire by Haystack Rock**
* **Spot starfish & other critters in the tide pools**
* **Spend an afternoon at Arcadia Beach**
* **Enjoy a morning coffee**
* **Drive up to Seaside**
Conclusion
The agents took about 5 minutes or so to output their results, but I did come across some interesting issues.
I originally tried to have the travel agent find a city on the Oregon Coast for me and give me an option to choose from, but I couldn’t get the option human_input=True
to work in VS Code, so I decided to just hard code the destination. Sometimes the agents would hallucinate and had to remind themselves to use the tools that I had given them.
I also had to limit their max_iterations
and give them a max_time
to complete the task, which wasn’t apart of the course. I had to create some limits, so this could have also affected their performance. I could have had a better results if I had a better laptop (I did this on a MacBook Air), access to a GPU (🤑) or had used OpenAI’s API ($20/month 😬), instead of my free, local Lllama LLM.
Despite the issues I had with getting the results I wanted from the agents, their outputs were promising after a few iterations.
It it very different working with fuzzy inputs and fuzzy outputs than just regular programming, but it really comes down to how you prompt, giving the agents clear tasks and a good backstory, so I will be diving into the Prompt Engineering guide to get better at it.
I think next time, I would give them a template for Markdown output to reference from and, of course, work on my prompting, because the Travel Advisor agent had the JSON result down by the 2nd iteration or so while the other two would sometimes output only a couple of sentences.
This experiment was inspiring and I can see how helpful it can be to create an agent crew to do work if given the the tools and clear direction to do so — being able to extend an LLMs functionality is absolutely mind-blowing. 🤯
And now it’s on to what I really want to do with AI agents… build a beach bonfire and roast some marshmallows for me!
Subscribe to my newsletter
Read articles from Shani Rivers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shani Rivers
Shani Rivers
I'm a data enthusiast with web development and graphic design experience. I know how to build a website from scratch, but I sometimes work with entrepreneurs and small businesses with their Wix or Squarespace sites, so basically I help them to establish their online presence. When I'm not doing that, I'm studying and blogging about data engineering, data science or web development. Oh, and I'm wife and a mom to a wee tot.