Django method decorator
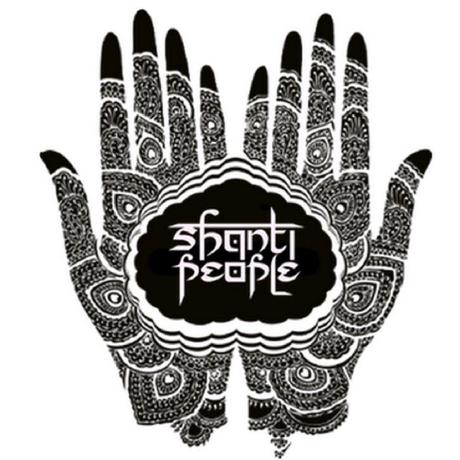
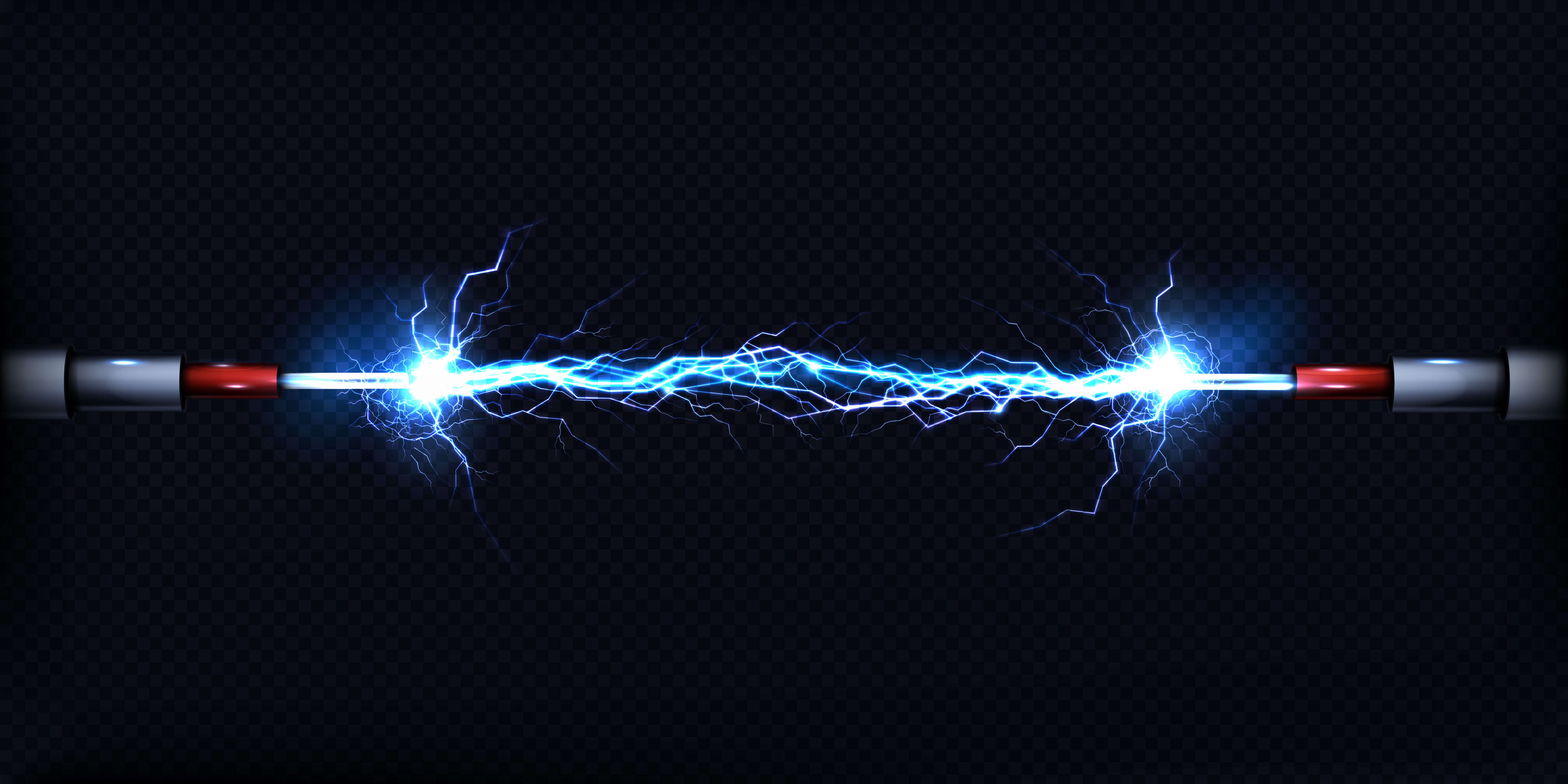
Recently I found some interesting issue when switching from Django 4.x to Django 5.x. I’ve been working with a legacy software and I had found some interesting case when core framework (Django) has been updated to one of the latest versions.
In the class view we can add lots of different decorators to methods. One of the main decorator I’ve been using for ling time in my django code was the one that I wrote to catch and log view calls, ie.
def my_log(*args):
def log_func(function):
def wrapper(request, *args, **kwargs):
# inject request API payload for airbrake
if log.isEnabledFor(logging.DEBUG):
function_name = "{}.{}".format(type(function).__name__, function.__name__)
log.debug(
"{} called with data: {}, args:{}, kwargs:{}".format(function_name, request.data, args, kwargs)
)
responder = function(request, *args, **kwargs)
try:
log.debug("{} responds with: {}".format(function_name, responder.content))
except Exception as ex:
log.error("{} failed to log response: {}".format(function_name, ex))
return responder
else:
return function(request, *args, **kwargs)
return wrapper
return log_func
Once I have custom decorator I can apply it to my view as shown in the following example.
class MyRestAPIView(GenericAPIView):
authentication_classes = ()
authentication_classes = (authentication.TokenAuthentication,)
permission_classes = (permissions.IsAuthenticated, )
@my_log
def post(self, request):
""""magic happens here"""
return Response(response.data, status=http_status.HTTP_201_CREATED)
That’s been working just fine until Django version 5. Once upgraded to this I realized that my decorator does not work anymore. The easiest fix is to move it under method decorator call like in proceeding example.
from django.utils.decorators import method_decorator
class MyRestAPIView(GenericAPIView):
@method_decorator((my_log())
def post(self, request):
""""magic happens here"""
return Response(response.data, status=http_status.HTTP_201_CREATED)
That’s it! Happy coding:)
Subscribe to my newsletter
Read articles from Hubert Piotrowski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
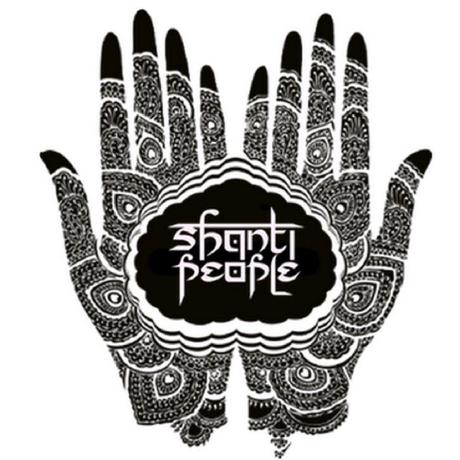