A Discussion on the Reduce in Javascript

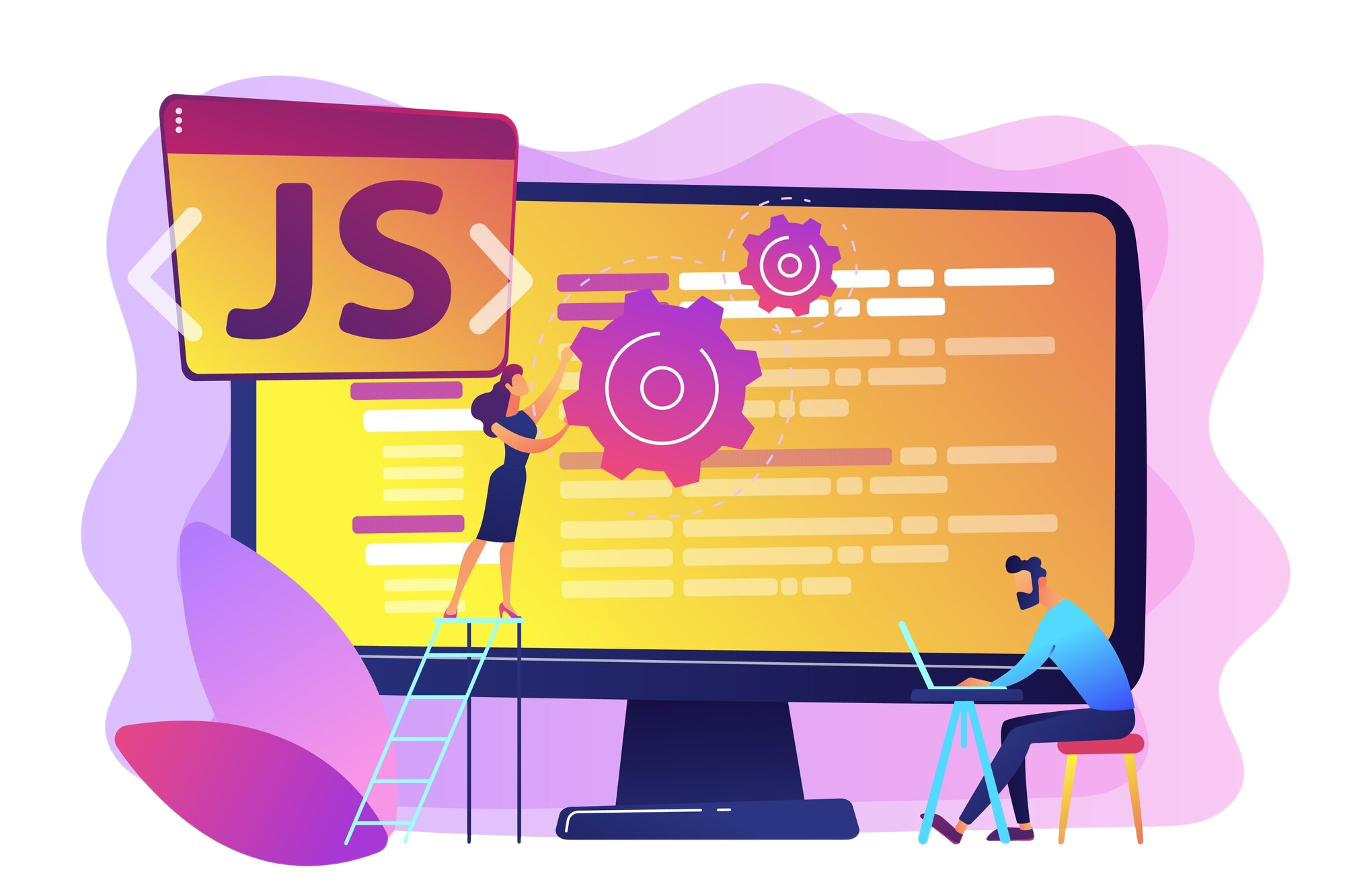
Hey there!
After diving deep into the forEach()
method, it's time to explore another powerhouse in JavaScript: the reduce()
method! If you've ever found yourself summing up an array of numbers or merging complex datasets, reduce()
is about to become your new best friend. So, grab your coffee, and let's break it down. ☕
🤔 Understanding reduce()
The reduce()
method is a higher-order function in JavaScript that allows you to process an array and reduce it into a single value—be it a sum, an object, or even another array. It iterates over each element, applying a callback function that accumulates the result step by step. Unlike forEach()
, which simply loops through elements, reduce()
builds a final output from them.
"Think of
reduce()
as a snowball rolling down a hill. With each step, it collects more snow (data) and grows into a final, well-formed result!"
📌 Syntax
array.reduce((accumulator, currentValue, currentIndex, array) => {
// logic here
}, initialValue);
Parameters:
accumulator
→ The variable that stores the progressively accumulated result as the callback function executes. Think of it as your storage box, where each iteration adds something new.currentValue
→ The element currently being processed in the array. This is what gets added, subtracted, merged, or transformed.currentIndex
(optional) → The index ofcurrentValue
within the array. Useful when you need positional awareness.array
(optional) → The array on whichreduce()
is called. Rarely used but can be handy.initialValue
(optional) → The starting value assigned toaccumulator
before iteration begins. If not provided, the first element of the array is used
"Think of
accumulator
as a piggy bank. Each time you go through the loop, you drop a coin (currentValue
) into it. By the end, you get the total amount!"
🔍 Understanding the Flow of reduce()
with a Dry Run
To truly grasp how reduce()
works, let’s step through a dry run with a simple example. This will help you visualize how the accumulator evolves with each iteration.
const numbers = [5, 10, 15];
const total = numbers.reduce((acc, num) => acc + num, 0);
console.log(total); // Output: 30
Step-by-Step Execution
1️⃣ Initialization: acc = 0
(initial value), num = 5
acc + num → 0 + 5 = 5
2️⃣ Next iteration:acc = 5
,num = 10
acc + num → 5 + 10 = 15
3️⃣ Final iteration:acc = 15
,num = 15
acc + num → 15 + 15 = 30
This step-by-step breakdown illustrates how reduce()
accumulates values over iterations to produce a single result.
🛠 Examples with Different Data Structures
1️⃣ Summing Up an Array of Numbers
const numbers = [10, 20, 30, 40];
const total = numbers.reduce((sum, num) => sum + num, 0);
console.log(total); // Output: 100
This is the most common use case: summing up values efficiently.
2️⃣ Flattening an Array of Arrays
const nestedArray = [[1, 2], [3, 4], [5, 6]];
const flatArray = nestedArray.reduce((acc, val) => acc.concat(val), []);
console.log(flatArray); // Output: [1, 2, 3, 4, 5, 6]
Here, reduce()
helps merge multiple arrays into a single one.
3️⃣ Transforming an Array of Objects
const tasks = [
{ task: "Write report", completed: false },
{ task: "Send email", completed: true },
{ task: "Prepare PPT", completed: false }
];
const incompleteTasks = tasks.reduce((acc, task) => {
if (!task.completed) acc.push(task.task);
return acc;
}, []);
console.log(incompleteTasks); // Output: ["Write report", "Prepare PPT"]
🌍 Real-World Use Cases
🛒 1. Calculating Cart Total (E-Commerce)
Imagine you're shopping online, and you want to see the total price of all items in your cart. Instead of looping through the array manually, reduce()
helps sum up all the prices efficiently.
const cart = [
{ product: "Laptop", price: 800 },
{ product: "Mouse", price: 50 },
{ product: "Keyboard", price: 100 }
];
const totalPrice = cart.reduce((total, item) => total + item.price, 0);
console.log(totalPrice); // Output: 950
See how reduce()
makes calculating totals in an online shopping cart seamless? Now, no more manual loops!
📊 2. Aggregating Survey Results
Let's say you conduct a survey, and now you need to count how many people answered 'yes' or 'no'. Instead of manually iterating through responses, reduce()
can efficiently tally the results.
const survey = ["yes", "no", "yes", "yes", "no", "no", "yes"];
const result = survey.reduce((acc, answer) => {
acc[answer] = (acc[answer] || 0) + 1;
return acc;
}, {});
console.log(result); // Output: { yes: 4, no: 3 }
This approach simplifies counting occurrences in an array—useful for surveys, votes, and more!
📁 3. Grouping Data Dynamically
When working with user data, you often need to categorize people by roles, departments, or preferences. reduce()
makes this grouping effortless.
const users = [
{ name: "Amit", role: "admin" },
{ name: "Priya", role: "editor" },
{ name: "Rahul", role: "admin" },
];
const groupedUsers = users.reduce((acc, user) => {
(acc[user.role] = acc[user.role] || []).push(user.name);
return acc;
}, {});
console.log(groupedUsers);
// Output: { admin: ["Amit", "Rahul"], editor: ["Priya"] }
Data organization becomes a breeze with reduce()
. Not only does it allow you to streamline data processing, but it also helps in making your code more efficient and readable. Whether you're categorizing users, aggregating statistics, or restructuring objects, reduce()
gives you the power to transform data effortlessly.
🎯 Wrapping It Up
So far so, We’ve taken a good knowledge aboutreduce()
, breaking down its syntax, logic, and real-world applications. By now, you should have a clear understanding of how it transforms arrays into meaningful results—whether it's summing numbers, grouping data, or restructuring objects.
The key takeaway? Think of reduce()
as a powerful tool for aggregation and transformation. Once you get comfortable with it, you'll find yourself reaching for it more often in your JavaScript journey.
So, the next time you need to process an array efficiently, ask yourself—can reduce()
do the job? Chances are, it can! 💡
Practice is key! Try rewriting some of your existing loops using reduce()
and see the magic unfold.
Subscribe to my newsletter
Read articles from Santwan Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santwan Pathak
Santwan Pathak
"A beginner in tech with big aspirations. Passionate about web development, AI, and creating impactful solutions. Always learning, always growing."