How to Achieve Type-Safe Integration Between Frontend and Backend

Table of contents
- Approach
- Why This Matters
- Steps to Implement
- Defining Backend Entities in Golang
- Setting Up Graphql Server & Generating GraphQL Types
- Organizing GraphQL Queries in a Maintainable Way
- Implementing the Executor Function
- Automating Query Extraction for Code Generation
- Codegen Configuration
- Benefits of This Approach
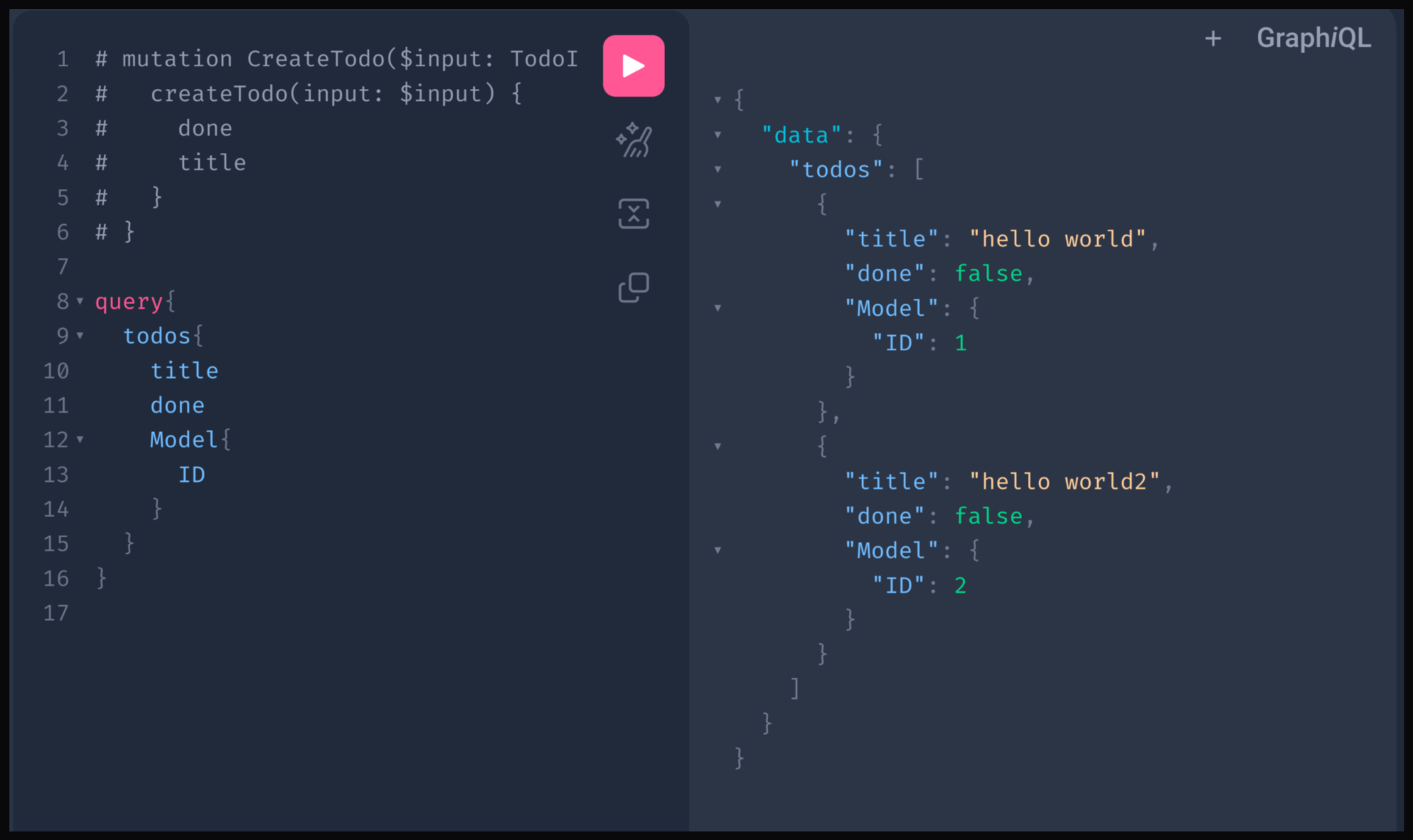
One of the major challenges in frontend-backend integration is maintaining type safety. When backend API structures change, frontend developers often struggle to detect breaking changes until runtime. To solve this, we need an automated approach that catches these issues at compile time.
A robust solution is to generate types directly from the backend API and use them in the frontend. This way, whenever there are breaking changes on the backend, the frontend can easily detect them by regenerating the types. This significantly improves developer experience and reduces integration errors.
Approach
In this article, we'll explore an approach to automatically generate TypeScript types from a backend API response and use them in a frontend application. We'll use:
Golang for the backend
Next.js with TypeScript for the frontend
GraphQL for API communication
Although this method can be implemented with REST APIs using OpenAPI Specification, GraphQL provides a more automated solution since entity types can be directly inferred from real backend entities.
Why This Matters
While working at Kloudlite, we faced this challenge with a six-member team. Manually writing types for every GraphQL query and API response was time-consuming. Automating this process saved significant development hours, allowing us to focus on building features rather than worrying about integration. With this setup, frontend developers always receive expected data, reducing the chances of runtime errors.
Steps to Implement
We'll go step by step to set up this integration:
Define backend entities in Golang
Generate GraphQL types from these entities
Expose GraphQL queries from the backend
At frontend accumulate all queries in one place and generate TypeScript types from gql SDL.
Defining Backend Entities in Golang
To demonstrate the type generation approach, let's build a simple Todo application where we fetch and manage tasks from a backend. We'll use GORM for database operations.
Defining the Todo Entity
We'll start by defining the Todo
entity using GORM:
type Todo struct {
gorm.Model `graphql:"noinput"`
Title string `json:"title"`
Done bool `json:"done"`
}
Implementing the Domain Layer
The domain layer abstracts database operations and provides a clean interface for managing todos.
Define the Domain Interface
type Domain interface {
AddTodo(ctx context.Context, todo *entities.Todo) (*entities.Todo, error)
ListTodos(ctx context.Context) ([]*entities.Todo, error)
GetTodo(ctx context.Context, id int) (*entities.Todo, error)
UpdateTodo(ctx context.Context, id int, todo *entities.Todo) (*entities.Todo, error)
DeleteTodo(ctx context.Context, id int) error
}
Implement the Domain Logic
func New(db *gorm.DB, ev *env.Env) Domain {
return &domain{
db: db,
env: ev,
}
}
type domain struct {
db *gorm.DB
env *env.Env
}
func (d *domain) AddTodo(ctx context.Context, todo *entities.Todo) (*entities.Todo, error) {
result := d.db.Create(todo)
if result.Error != nil {
return nil, result.Error
}
return todo, nil
}
func (d *domain) DeleteTodo(ctx context.Context, id int) error {
result := d.db.Delete(&entities.Todo{}, id)
if result.Error != nil {
return result.Error
}
if result.RowsAffected == 0 {
return fmt.Errorf("no todo found with id %d", id)
}
return nil
}
func (d *domain) GetTodo(ctx context.Context, id int) (*entities.Todo, error) {
var todo entities.Todo
result := d.db.First(&todo, id)
if result.Error != nil {
return nil, result.Error
}
return &todo, nil
}
func (d *domain) ListTodos(ctx context.Context) ([]*entities.Todo, error) {
var todos []*entities.Todo
result := d.db.Find(&todos)
if result.Error != nil {
return nil, result.Error
}
return todos, nil
}
func (d *domain) UpdateTodo(ctx context.Context, id int, todo *entities.Todo) (*entities.Todo, error) {
result := d.db.Save(todo)
if result.Error != nil {
return nil, result.Error
}
if result.RowsAffected == 0 {
return nil, fmt.Errorf("no todo found with id %d", id)
}
return todo, nil
}
Setting Up Graphql Server & Generating GraphQL Types
Here’s how you can set up your GraphQL server and integrate the process of generating GraphQL types from your Golang structs, step by step:
Step 1: Set Up the GraphQL Server
We will begin by following the Apollo GraphQL Golang tutorial, which provides a simple boilerplate for setting up a GraphQL server in Golang. After implementing the boilerplate, you’ll have a server like this:
func Graphql(d domain.Domain, ev *env.Env) error {
srv := handler.New(generated.NewExecutableSchema(generated.Config{
Resolvers: &graph.Resolver{
Domain: d,
},
}))
srv.AddTransport(transport.Options{})
srv.AddTransport(transport.GET{})
srv.AddTransport(transport.POST{})
srv.SetQueryCache(lru.NewIntrospection{})
srv.Use(extension.AutomaticPersistedQuery{
Cache: lru.New ,
})
http.Handlound.Handler("GraphQL playground", "/query"))
http.Handle("/query", srv)
logging.Get().Info(fmt.Sprintf("connect to http://localhost:%d/ for GraphQL playground", ev.PORT))
return http.ListenAndServe(fmt.Sprintf(":%d", ev.PORT), nil)
}
This code does the following:
Initializes a GraphQL server using
handler.New
with the schema generated from yourResolvers
.Sets up different transports (GET, POST, and Options) for handling requests.
Uses query caching and introspection for GraphQL metadata.
Sets up the GraphQL Playground at the
/
endpoint and the query handler at/query
.Finally, it listens on the specified port and logs the URL to access the playground.
Step 2: Generate GraphQL Types From Golang Struct
Next, we’ll use the struct-to-gql tool to automatically generate GraphQL types from your Golang structs.
Steps:
Add github.com/abdheshnayak/struct-to-gql and github.com/abdheshnayak/struct-to-gql/pkg/parser to your tools.go so it will be available for use.
//go:build tools // +build tools package tools import ( _ "github.com/99designs/gqlgen" _ "github.com/abdheshnayak/struct-to-gql" _ "github.com/abdheshnayak/struct-to-gql/pkg/parser" )
generate the schemas form your struct by using script like below:
working_dir=$(mktemp -d) mkdir -p "$working_dir" - go run github.com/abdheshnayak/struct-to-gql --struct package.Entity # example: github.com/abdheshnayak/gorm-practice/app/entities.Todo > $working_dir/main.go - |+ pushd "$working_dir" go run main.go --dev --out-dir ../graph/struct-to-graphql popd - rm -rf $working_dir
In above script we created a work directory where a go file generated by the tool by executing which we can get our real GraphQL schema for the type we defined in above with struct flag.
Step 3: Use Generated GraphQL Types
Once the GraphQL types are generated, you can import the generated schema into your existing GraphQL schema. For example, in your GraphQL YAML configuration file, you can reference both the existing schema and the generated types:
schema:
- graph/*.graphqls
- graph/struct-to-graphql/*.graphqls
This configuration allows the server to merge the generated schema files from the struct-to-graphql
tool with your existing GraphQL schema. As a result, your backend GraphQL server will have access to the newly generated types and will be able to serve data using the updated schema.
Once integrated, you can directly use the generated GraphQL types in your queries and mutations. For instance:
type Query {
todos: [Todo!]!
todo(id: ID!): Todo
}
type Mutation {
createTodo(input: TodoIn!): Todo!
updateTodo(id: ID!, input: TodoIn!): Todo!
}
And also we can implement the resolvers accordingly:
// CreateTodo is the resolver for the createTodo field.
func (r *mutationResolver) CreateTodo(ctx context.Context, input entities.Todo) (*entities.Todo, error) {
return r.Resolver.Domain.AddTodo(ctx, &input)
}
// UpdateTodo is the resolver for the updateTodo field.
func (r *mutationResolver) UpdateTodo(ctx context.Context, id int, input entities.Todo) (*entities.Todo, error) {
return r.Resolver.Domain.UpdateTodo(ctx, id, &input)
}
// Todos is the resolver for the todos field.
func (r *queryResolver) Todos(ctx context.Context) ([]*entities.Todo, error) {
return r.Resolver.Domain.ListTodos(ctx)
}
// Todo is the resolver for the todo field.
func (r *queryResolver) Todo(ctx context.Context, id int) (*entities.Todo, error) {
return r.Resolver.Domain.GetTodo(ctx, id)
}
So, we will get the GraphQL server and access our Todo application and will be able to query from our GraphQL server, as shown in the screenshot.
So we are done with our backend part, where we used a single Todo struct and gql schema from the same type. Next, we are going to proceed to generating TypeScript types from the gql SDL (Schema Definition Language) and implementing a better design pattern to consume the gql API efficiently.
Organizing GraphQL Queries in a Maintainable Way
When building a frontend application with GraphQL, it's crucial to structure queries and mutations in a way that ensures maintainability and scalability. In this article, we'll explore a centralized approach to managing GraphQL queries and mutations while using an executor
function to streamline request handling.
Structuring GraphQL Queries and Mutations
To keep our GraphQL operations well-organized, we define all queries and mutations in a single module. This approach enhances maintainability and provides a single source of truth for API interactions.
Key Features:
Centralized Queries and Mutations: All GraphQL operations are stored in one place for better organization and easy management.
Transform Function: Processes and refines API responses to return structured data.
Vars Function: Defines expected query variables, ensuring type safety and predictability.
Automated Query Extraction: Queries are extracted and stored in a
.graphql
file for use with Codegen.
Example of Centralized Queries and Mutations
import gql from "graphql-tag";
import { executor } from "./executor";
import {
MainGetTodoQuery,
MainGetTodoQueryVariables,
MainListTodosQuery,
MainListTodosQueryVariables,
} from "@/generated/types/server";
export const queries = () => ({
listTodos: executor(
gql`query ListTodos { todos { title done Model { ID } } }`,
{
transform: (data: MainListTodosQuery) => data.todos,
vars(_: MainListTodosQueryVariables) {},
}
),
getTodo: executor(
gql`query GetTodo($input: ID!) { todo(id: $input) { title } }`,
{
transform: (data: MainGetTodoQuery) => data.todo,
vars(_: MainGetTodoQueryVariables) {},
}
)
});
So in above code we can see the the imported types for the queries. these types are auto generated from the defined queries that we are going to discuss in detail.
Implementing the Executor Function
The executor
function simplifies GraphQL request execution using Axios while handling responses efficiently. It accepts a query or mutation along with transformation logic and variable definitions, ensuring a streamlined API interaction process.
Key Features:
Dynamic Query Execution: The function accommodates any GraphQL query or mutation and executes it with the provided variables.
Automatic Response Handling: Extracts relevant data from API responses, making them easier to work with.
Error Management: Captures and processes errors for better debugging and resilience.
GraphQL Query Printing: Converts
DocumentNode
into a string using theprint
function fromgraphql
.
Example of the Executor Function
import { DocumentNode, print } from "graphql";
import axios from "axios";
import { urls } from "@/config/constants";
export const executor = <T, T2, T3>(
query: DocumentNode,
options: { transform: (data: T) => T2; vars: (data: T3) => void }
) => {
const res = async (data: T3) => {
try {
const result = await axios.post(urls.api, {
query: print(query),
variables: data
}, { headers: { "Content-Type": "application/json" } });
if (result.data.errors) {
throw new Error(JSON.stringify(result.data.errors));
}
return options.transform(result.data.data);
} catch (err: any) {
throw err.response?.data?.errors || err;
}
};
res.Query = () => query;
return res;
};
Automating Query Extraction for Code Generation
To ensure type safety and maintainability, we use Codegen to generate TypeScript types for our GraphQL queries. We first extract queries and save them to a .graphql
file, which Codegen then uses to generate types.
Extracting Queries
import fs from "fs";
import { DocumentNode, print } from "graphql";
export const loader = (handler: () => { [key: string]: { Query(): DocumentNode } }, prefix: string) => {
const gqlQueries = handler();
const updatedQueries: { [name: string]: DocumentNode } = {};
Object.entries(gqlQueries).forEach(([key, query]) => {
// @ts-ignore
query.Query().definitions[0].name.value = `${prefix}${key.charAt(0).toUpperCase() + key.slice(1)}`;
updatedQueries[key] = query.Query();
});
return Object.values(updatedQueries).map(print).join("\n\n");
};
export const ensureDirectoryExistence = (dirPath: string) => {
if (!fs.existsSync(dirPath)) fs.mkdirSync(dirPath, { recursive: true });
};
export const docPath = "generated/gql";
Writing Extracted Queries to a File
import fs from "fs";
import { docPath, ensureDirectoryExistence, loader } from "./loader";
import { queries } from "@/lib/queries";
const init = () => {
ensureDirectoryExistence(docPath);
const results = [loader(queries, "main")];
const info = `# This file is auto-generated. Do not modify it manually.\n# Generated by pnpm gql:parse`;
fs.writeFileSync(`${docPath}/queries.graphql`, `${info}\n\n${results.join("\n\n")}`);
};
init();
Codegen Configuration
Now we can feed our generated queries file to Codegen to generate TypeScript types that can be used within our application.
overwrite: true
schema: "http://localhost:8080/query"
generates:
generated/types/server.ts:
documents:
- generated/gql/*.graphql
plugins:
- "typescript"
- typescript-operations
config:
onlyOperationTypes: true
skipTypename: true
ignoreEnumValuesFromSchema: true
enumsAsTypes: true
maybeValue: 'T'
hooks:
afterOneFileWrite:
- 'eslint --fix'
So with above steps we can generate the types for our our graphql queries automatically when any changes is pushed to the backend entity.
Using the query in our react component
Here is how we can use the query in our react component, i am considering the client side react component but you can use the same with other fronend frameworks as well.
export default function App() {
const [todos, setTodos] = useState<MainListTodosQuery["todos"]>([]);
useEffect(() => {
(async () => {
const todos = await queries().listTodos({});
setTodos(todos);
})();
}, []);
return (
<Table aria-label="Example table with dynamic content">
<TableHeader columns={columns}>
{(column) => <TableColumn key={column.key}>{column.label}</TableColumn>}
</TableHeader>
<TableBody
items={todos.map((todo) => ({
...todo,
done: todo.done ? "done" : "pending",
}))}
>
{(item) => (
<TableRow key={item.Model.ID}>
{(columnKey) => (
<TableCell>{getKeyValue(item, columnKey)}</TableCell>
)}
</TableRow>
)}
</TableBody>
</Table>
);
}
So the response of the listTodos call we will get the typed response like this:
And finally we listed our todos items on frontend:
Benefits of This Approach
By structuring GraphQL queries in a centralized manner and leveraging an executor function, we achieve:
Improved Code Organization: All API interactions are neatly structured in a single place.
Enhanced Maintainability: Queries and mutations are easy to update and modify.
Type Safety: Using TypeScript ensures queries return the expected data types, reducing runtime errors.
Efficient API Calls: The executor function streamlines request handling and error management, ensuring a smooth development experience.
All of the above implementation available in the given repository.
Subscribe to my newsletter
Read articles from Abdhesh Nayak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
