Understanding Asynchronous Programming in JavaScript

Table of contents
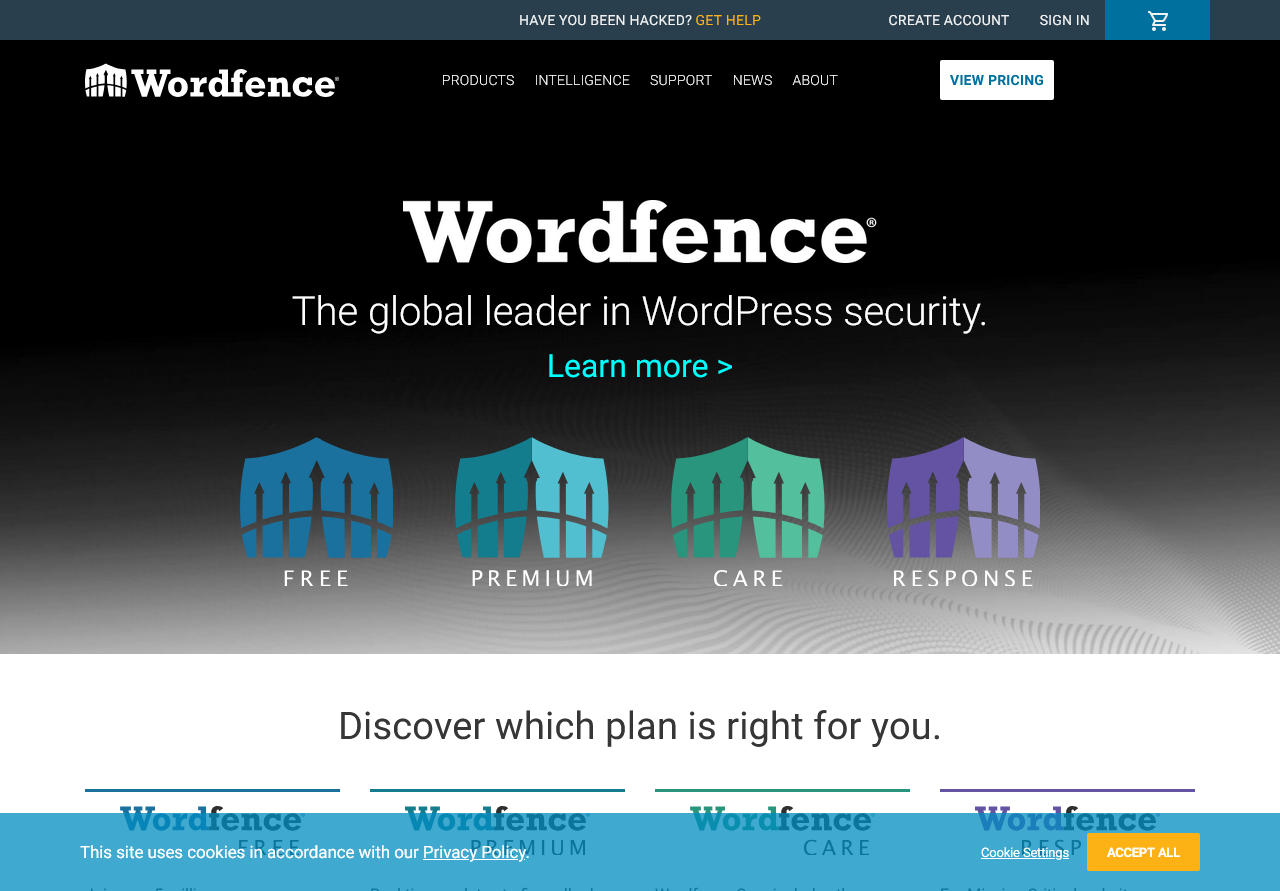
Understanding Asynchronous Programming in JavaScript
JavaScript is a powerful and versatile programming language that has become the backbone of modern web development. One of its most important features is its ability to handle asynchronous operations. Asynchronous programming allows JavaScript to perform tasks like fetching data from a server, reading files, or handling user interactions without blocking the main thread. This ensures that your web applications remain responsive and efficient.
In this article, we’ll dive deep into asynchronous programming in JavaScript, exploring its core concepts, techniques, and best practices. Whether you're a beginner or an experienced developer, understanding asynchronous programming is crucial for building high-performance applications. And if you're looking to monetize your programming skills, platforms like MillionFormula offer a great way to make money online—completely free and without the need for credit or debit cards.
What is Asynchronous Programming?
In synchronous programming, code is executed line by line, and each operation must complete before the next one begins. While this approach is straightforward, it can lead to performance bottlenecks, especially when dealing with time-consuming tasks like network requests or file I/O.
Asynchronous programming, on the other hand, allows multiple operations to run concurrently. This means that while one task is being processed, the program can continue executing other tasks. In JavaScript, this is achieved using mechanisms like callbacks, Promises, and async/await.
Callbacks: The Foundation of Asynchronous JavaScript
Callbacks are the simplest way to handle asynchronous operations in JavaScript. A callback is a function that is passed as an argument to another function and is executed once the parent function completes its task.
Here’s an example of a callback in action:
javascript
Copy
function fetchData(callback) {
setTimeout(() => {
const data = "This is the fetched data";
callback(data);
}, 2000); // Simulate a 2-second delay
}
fetchData((data) => {
console.log(data); // Output: "This is the fetched data" after 2 seconds
});
While callbacks are easy to understand, they can lead to callback hell—a situation where nested callbacks make the code difficult to read and maintain.
Promises: A Better Way to Handle Asynchronous Code
Promises were introduced in ES6 to address the shortcomings of callbacks. A Promise represents a value that may be available now, in the future, or never. It has three states: pending, fulfilled, and rejected.
Here’s how you can use a Promise:
javascript
Copy
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = "This is the fetched data";
resolve(data);
}, 2000);
});
}
fetchData()
.then((data) => {
console.log(data); // Output: "This is the fetched data" after 2 seconds
})
.catch((error) => {
console.error(error);
});
Promises provide a cleaner and more structured way to handle asynchronous operations. They also allow you to chain multiple asynchronous tasks using .then()
.
Async/Await: The Modern Approach
Introduced in ES8, async/await
is syntactic sugar built on top of Promises. It allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to read and debug.
Here’s an example of using async/await
:
javascript
Copy
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
const data = "This is the fetched data";
resolve(data);
}, 2000);
});
}
async function main() {
try {
const data = await fetchData();
console.log(data); // Output: "This is the fetched data" after 2 seconds
} catch (error) {
console.error(error);
}
}
main();
With async/await
, you can handle errors using try/catch
blocks, making your code more robust and maintainable.
Real-World Use Cases of Asynchronous Programming
Fetching Data from APIs: Asynchronous programming is essential when working with APIs. For example, you can use the
fetch
API to retrieve data from a server: javascript Copyasync function fetchUserData() { const response = await fetch('https://api.example.com/users'); const data = await response.json(); console.log(data); } fetchUserData();
Handling User Input: Asynchronous programming is also useful for handling user interactions, such as form submissions or button clicks, without blocking the UI.
File Operations: When working with files in Node.js, asynchronous methods like
fs.readFile
ensure that your application remains responsive.
Best Practices for Asynchronous Programming
Avoid Callback Hell: Use Promises or
async/await
instead of deeply nested callbacks.Error Handling: Always handle errors using
.catch()
with Promises ortry/catch
withasync/await
.Use
Promise.all
for Parallel Execution: If you need to run multiple asynchronous tasks concurrently, usePromise.all
: javascript Copyasync function fetchMultipleData() { const [data1, data2] = await Promise.all([fetchData1(), fetchData2()]); console.log(data1, data2); }
Keep Your Code Clean: Use meaningful variable names and modularize your code to improve readability.
Monetize Your JavaScript Skills
If you’ve mastered asynchronous programming in JavaScript, you’re already equipped with a valuable skill that can help you make money online. Platforms like MillionFormula provide opportunities to monetize your programming expertise without any upfront costs or the need for credit or debit cards. Whether you're building web applications, contributing to open-source projects, or freelancing, your skills can open doors to countless opportunities.
Conclusion
Asynchronous programming is a cornerstone of modern JavaScript development. By mastering concepts like callbacks, Promises, and async/await
, you can build efficient and responsive applications. Remember to follow best practices and keep your code clean and maintainable. And if you're ready to take your skills to the next level, explore platforms like MillionFormula to start earning online today.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
