Day 19: JavaScript Performance Optimizationβ-βHow to Make Code Run FasterΒ π

Table of contents
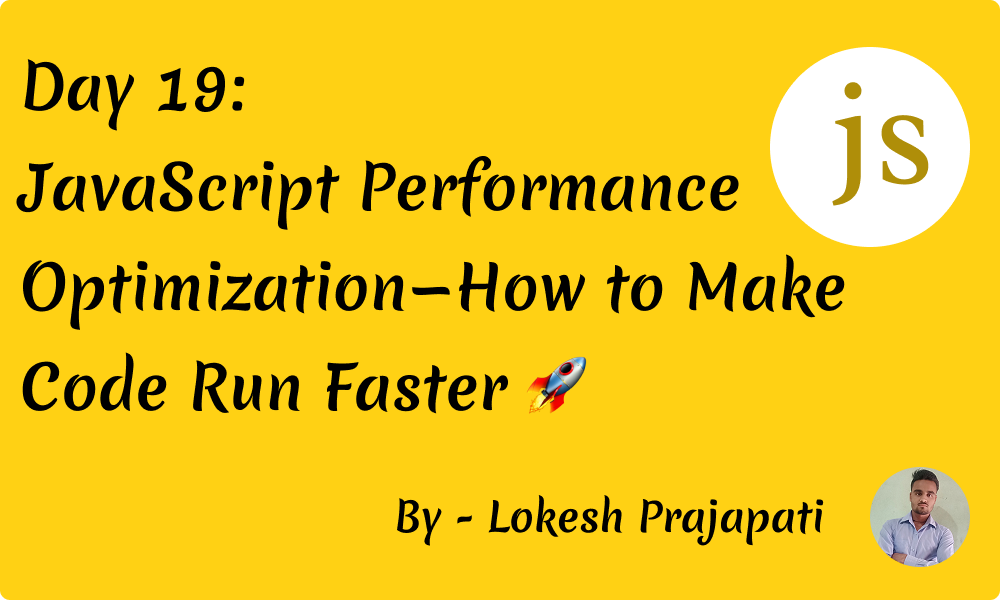
JavaScript is a powerful language, but if not written efficiently, it can lead to performance bottlenecks, slow execution, and poor user experience. In this guide, weβll cover essential JavaScript performance optimization techniques to make your code run faster and more efficiently.
1. Minimize DOM Manipulation ποΈ
DOM operations are expensive. Frequent updates to the DOM slow down rendering and impact performance.
β Bad Practice:
const list = document.getElementById("list");
for (let i = 0; i < 1000; i++) {
const item = document.createElement("li");
item.textContent = `Item ${i}`;
list.appendChild(item); // Directly modifying the DOM repeatedly
}
β Optimized Approach:
const list = document.getElementById("list");
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const item = document.createElement("li");
item.textContent = `Item ${i}`;
fragment.appendChild(item);
}
list.appendChild(fragment); // Single DOM update
Why? Using a DocumentFragment
reduces reflows and repaints, improving performance.
2. Optimize Loops and Iterations π
Avoid unnecessary loops and use efficient iteration methods.
β Inefficient Loop:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
β Optimized Approach:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(num => console.log(num)); // Faster & cleaner
Bonus: Using for...of
for (const num of numbers) {
console.log(num);
}
Why? forEach
and for...of
are optimized for iterating arrays.
3. Use Efficient Event Handling π―
Attaching too many event listeners can slow down your app.
β Bad Practice:
document.getElementById("btn").addEventListener("click", () => console.log("Clicked!"));
document.getElementById("link").addEventListener("click", () => console.log("Clicked link!"));
β Optimized Approach: Event Delegation
document.addEventListener("click", (event) => {
if (event.target.matches("#btn")) {
console.log("Clicked!");
}
if (event.target.matches("#link")) {
console.log("Clicked link!");
}
});
Why? Reducing the number of event listeners improves memory efficiency.
4. Avoid Memory Leaks π§
Memory leaks occur when objects are not properly garbage collected.
β Common Memory Leak:
let arr = [];
document.getElementById("btn").addEventListener("click", function () {
arr.push(new Array(1000000).fill("data")); // Large objects stay in memory
});
β Optimized Approach:
document.getElementById("btn").addEventListener("click", function () {
arr = []; // Reset the reference
});
Why? Removing unnecessary references allows garbage collection to free memory.
5. Optimize API Calls & Use Caching π
Fetching data multiple times unnecessarily slows performance.
β Unoptimized API Call:
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log(data));
β Optimized Approach: Caching API Response
let cache = {};
async function fetchData(url) {
if (cache[url]) {
return cache[url]; // Return cached data
}
const response = await fetch(url);
const data = await response.json();
cache[url] = data; // Store response in cache
return data;
}
fetchData("https://api.example.com/data").then(data => console.log(data));
Why? Caching prevents redundant API calls and speeds up data retrieval.
6. Optimize JavaScript Execution π
Use requestAnimationFrame()
for Animations π¬
Instead of setInterval()
or setTimeout()
, use requestAnimationFrame()
for smoother animations.
function animate() {
console.log("Animating...");
requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
Why? It ensures animations sync with the browserβs refresh rate.
7. Debounce & Throttle Expensive Functions ποΈ
Avoid running functions too frequently, especially in scroll
or resize
events.
Debouncing (Wait until user stops typing):
function debounce(func, delay) {
let timer;
return function (...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
window.addEventListener("resize", debounce(() => console.log("Resized!"), 300));
Throttling (Run function at controlled intervals):
function throttle(func, limit) {
let lastFunc, lastTime;
return function () {
const context = this, args = arguments;
if (!lastTime || Date.now() - lastTime >= limit) {
func.apply(context, args);
lastTime = Date.now();
}
};
}
window.addEventListener("scroll", throttle(() => console.log("Scrolling..."), 200));
Why? Throttling and debouncing optimize event-heavy functions.
Conclusion π
Optimizing JavaScript performance is crucial for faster load times and better user experience. By minimizing DOM manipulation, optimizing loops, handling events efficiently, and preventing memory leaks, you can significantly improve your appβs speed. π
What are your favorite JavaScript performance tips? Drop them in the comments! π
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
π JavaScript | React | Shopify Developer | Tech Blogger Hi, Iβm Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. Iβm currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Letβs learn and grow together. π‘π #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode