JSON Web Tokens (JWT): Simplifying Secure Authentication and Data Exchange
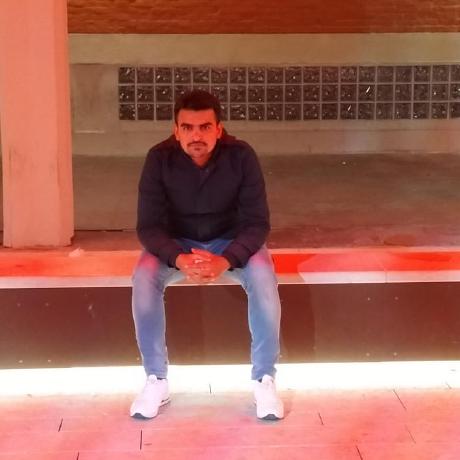
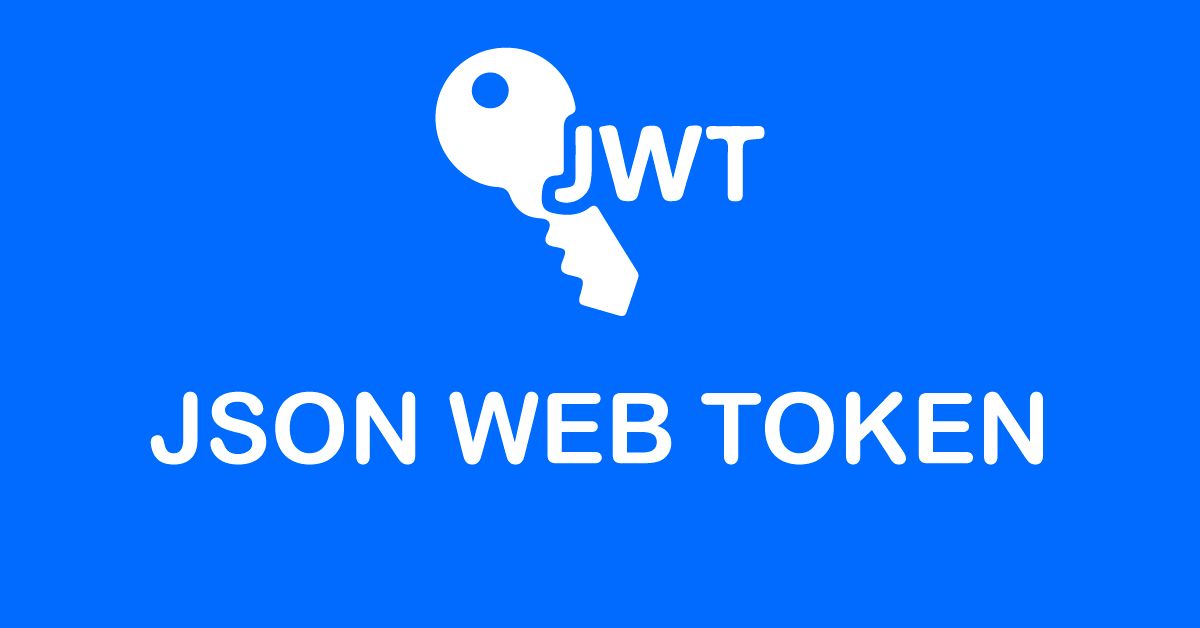
JSON Web Tokens (JWT) have become a cornerstone of modern authentication and data exchange systems due to their compactness, efficiency, and ease of use. In this article, we’ll explore the fundamentals of JWT, its use cases, and practical examples of its implementation.
What is a JSON Web Token (JWT)?
A JSON Web Token (JWT) is a compact, URL-safe token format used to securely transmit information between parties as a JSON object. The token is self-contained, meaning it includes all the necessary information for the intended purpose, eliminating the need to query a database repeatedly.
Structure of a JWT
A JWT consists of three parts:
Header: Contains metadata about the token, including the type of token (JWT) and the signing algorithm (e.g., HMAC SHA256 or RSA).
Payload: Contains the claims, which are statements about the entity (usually a user) and additional metadata.
Signature: Ensures the integrity of the token by encoding the header and payload using a secret or public/private key.
A typical JWT looks like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9
.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ
.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
Key Use Cases for JWT
1. User Authentication
JWTs are widely used for authenticating users in modern web and mobile applications. After a user successfully logs in, a server generates a JWT and sends it to the client. The client includes the token in the header of subsequent requests, enabling stateless authentication.
Example:
A user logs into a Single Page Application (SPA).
The server validates the credentials and issues a JWT.
The client stores the token (e.g., in localStorage or cookies) and attaches it to every API request in the
Authorization
header as a Bearer token.
2. Information Exchange
JWTs facilitate secure and compact data exchange. Because they are signed, the recipient can verify the data's integrity and authenticity without requiring access to the sender’s database.
Example:
Two microservices exchanging user data via an API.
The originating service generates a JWT containing the user's ID and roles.
The receiving service validates the token and processes the request accordingly.
3. API Authorization
APIs often use JWTs to enforce access control. By embedding user roles or permissions within the token, servers can restrict access to specific endpoints or resources without additional database lookups.
Example:
A JWT issued to a user contains a claim indicating
role: "admin"
.The API checks the JWT to confirm that the user has administrative privileges before granting access to sensitive endpoints.
4. Single Sign-On (SSO)
JWTs are integral to SSO implementations, allowing users to authenticate across multiple applications using a single login.
Example:
A user logs into an identity provider (IdP).
The IdP issues a JWT containing user identity claims.
The token is used to access multiple affiliated applications without requiring separate logins.
Practical Examples
Example 1: Implementing JWT Authentication in Node.js
const jwt = require('jsonwebtoken');
// Secret key
const SECRET_KEY = "your_secret_key";
// Generate JWT
function generateToken(user) {
const payload = {
id: user.id,
username: user.username,
role: user.role,
};
return jwt.sign(payload, SECRET_KEY, { expiresIn: '1h' });
}
// Middleware to verify JWT
function authenticateToken(req, res, next) {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.sendStatus(401);
jwt.verify(token, SECRET_KEY, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
In this example:
The
generateToken
function issues a JWT containing user data.The
authenticateToken
middleware validates the token and attaches the decoded payload to thereq.user
object.
Example 2: Using JWT in a React Application
In a React application, you can manage authentication using JWTs as follows:
Login and Store the Token:
fetch('/api/login', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ username, password }), }) .then(response => response.json()) .then(data => localStorage.setItem('token', data.token));
Attach the Token to Requests:
const token = localStorage.getItem('token'); fetch('/api/protected', { method: 'GET', headers: { 'Authorization': `Bearer ${token}`, }, }) .then(response => { if (response.status === 401) { // Handle unauthorized access } return response.json(); });
Best Practices When Using JWT
Use Secure Storage: Store tokens in secure locations like HTTP-only cookies to protect against XSS attacks.
Implement Token Expiry: Set an appropriate expiration time to mitigate the risk of token misuse.
Use HTTPS: Always transmit tokens over secure HTTPS connections to prevent interception.
Validate Tokens Properly: Verify both the signature and claims (e.g., expiration) on the server.
Rotate Secrets Periodically: Update signing keys regularly to enhance security.
Conclusion
JWTs are a powerful tool for building secure and scalable systems. Their ability to streamline authentication, authorization, and data exchange makes them invaluable in modern software architecture. By adhering to best practices and leveraging JWTs effectively, developers can create robust and user-friendly applications that stand the test of time.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
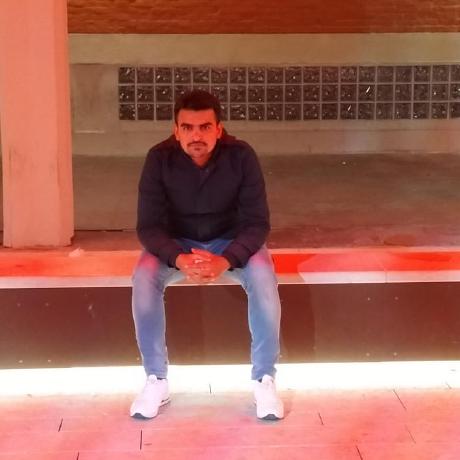
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.