JavaScript Throttling Explained

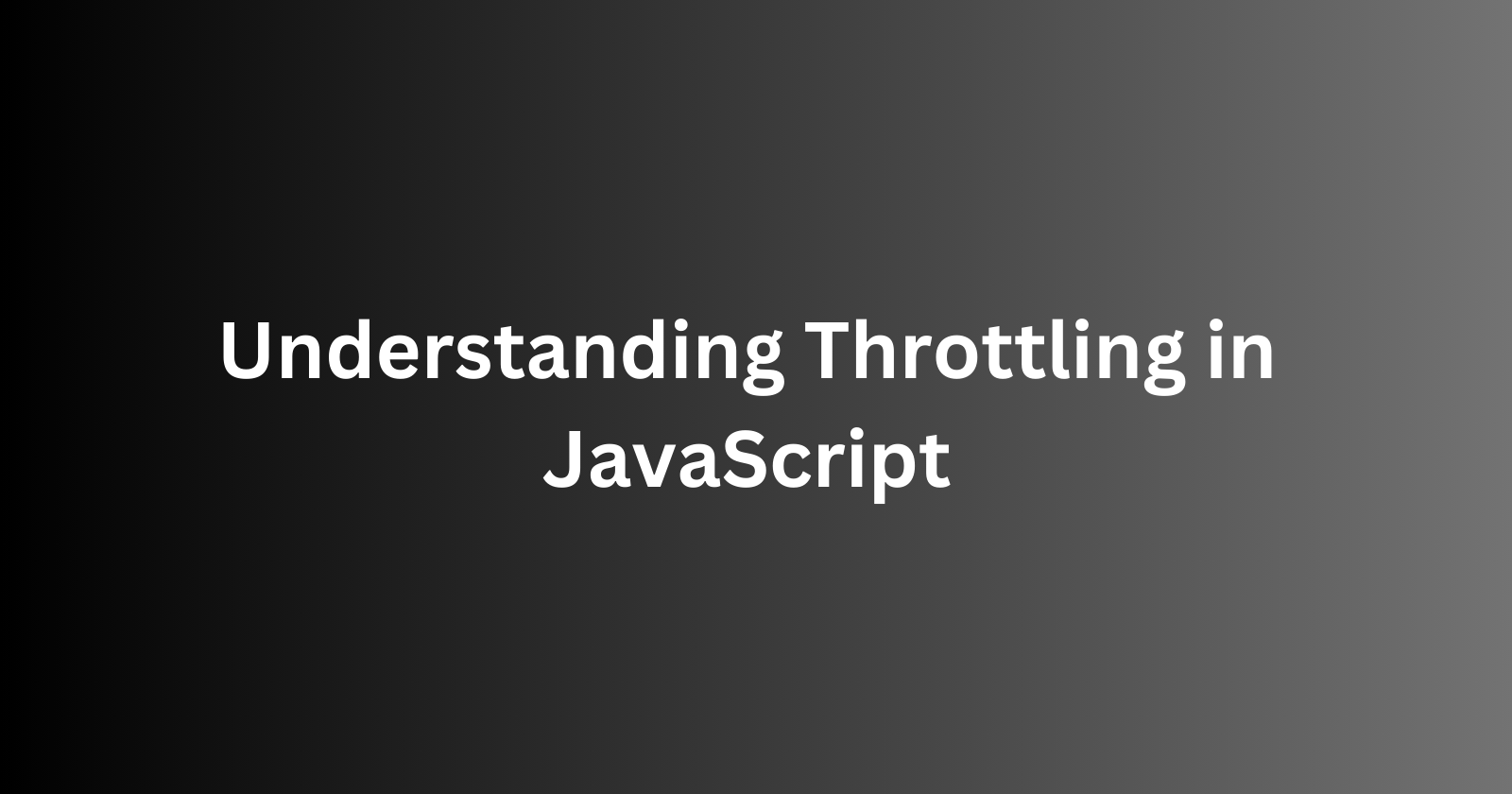
Master JavaScript Throttling: Improve Performance & Avoid Lag
What is Throttling?
Throttling is a way to limit how often a function runs. It makes sure the function runs only once in a set time, even if an event happens many times. This helps prevent unnecessary function calls and keeps things running smoothly.
Why Use Throttling?
Throttling is particularly useful for optimizing performance in scenarios where events fire repeatedly in quick succession, such as:
Scrolling events
Window resizing
Button clicks
API calls
By throttling a function, we can improve application efficiency and prevent performance issues like excessive rendering or unnecessary API requests.
Implementation of Throttling in JavaScript
// Throttle function: Ensures `fn` is called at most once every `delay` milliseconds.
function throttle(fn, delay) {
let lastCall = 0; // Tracks the last execution time
return function (...args) {
const now = Date.now(); // Get current timestamp
if (now - lastCall >= delay) { // Check if enough time has passed since last call
lastCall = now; // Update last execution time
fn(...args); // Call the original function with the provided arguments
}
};
}
// Function to simulate sending a chat message
function sendChatMessage(message) {
console.log('Sending Message:', message);
}
// Create a throttled version of `sendChatMessage` that executes at most once every 2 seconds
const sendChatMessageWithSlowMode = throttle(sendChatMessage, 2000);
// Simulating multiple calls
sendChatMessageWithSlowMode('Hi'); // Executes immediately
sendChatMessageWithSlowMode('Hello'); // Ignored (called too soon)
sendChatMessageWithSlowMode('Hello ji'); // Ignored (called too soon)
setTimeout(() => sendChatMessageWithSlowMode('Hello again'), 3000); // Executes after 3 seconds
Output
Sending Message: Hi(3 seconds delay...)
Sending Message: Hello again
Step-by-Step Execution Flow
Throttle Function Call:
throttle(sendChatMessage, 2000)
is called.The throttle function executes immediately and returns a new function, which we store in
sendChatMessageWithSlowMode
.
First Function Execution:
sendChatMessageWithSlowMode('Hi')
is called.Inside the throttled function:
now = Date.now()
stores the current timestamp.lastCall
is0
, sonow - lastCall
is greater thandelay (2000ms)
.lastCall
is updated tonow
.sendChatMessage('Hi')
is executed.
Second Function Call (Ignored):
sendChatMessageWithSlowMode('Hello')
is called immediately after.now - lastCall
is less than2000ms
, so the function call is ignored.
Third Function Call (Executed After Timeout):
setTimeout
schedulessendChatMessageWithSlowMode('Hello again')
after 3000ms.now - lastCall
is now greater than2000ms
, so:lastCall
is updated.sendChatMessage('Hello again')
executes successfully.
Key Learnings
The
throttle
function limits function execution within a specified time frame.It is useful for optimizing event-heavy scenarios like scrolling, resizing, and API requests.
Throttling ensures that function calls are controlled, preventing unnecessary execution and improving performance.
When to Use Throttling?
Use throttling when:
You want to improve UI performance (e.g., limiting button clicks).
Handling high-frequency events like scrolling or resizing.
Preventing excessive API calls to reduce server load.
Throttling is a powerful technique that helps developers create efficient and smooth web applications by controlling function execution frequency.
Thanks to Piyush Garg for the Insights
Connect to me for more info…
Subscribe to my newsletter
Read articles from Shahbaz Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
