Mastering the find Method in JavaScript: Efficient Array Searching
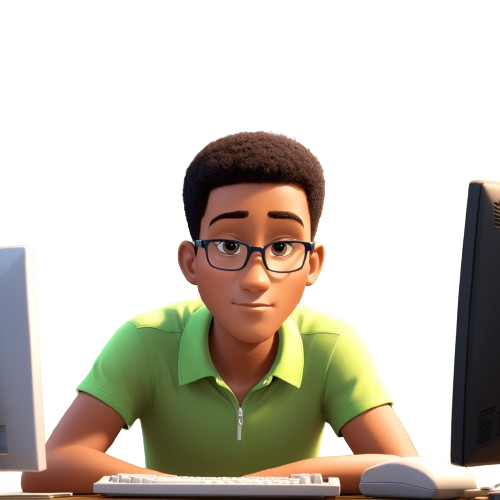
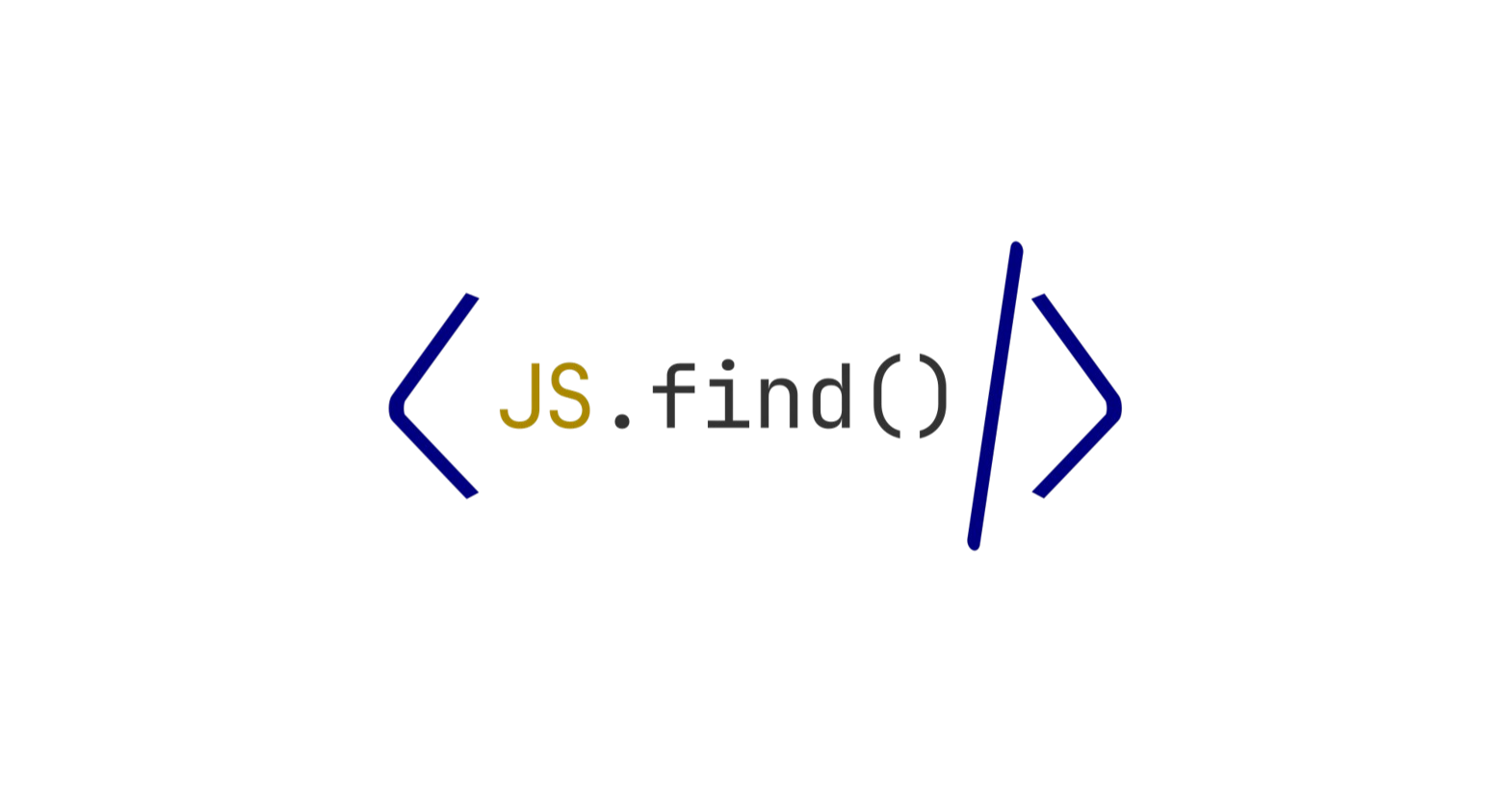
Introduction
Searching for a specific element in an array is a common task in programming, and JavaScript provides an elegant solution for this—the find
method. In this article, we'll explore the find
method, breaking down its functionality through practical examples and interactive exercises.
Understanding the Find Method
Just like filter
and map
, the find
method operates on arrays, enabling the retrieval of an element based on a given condition. Unlike filter
, which returns an array of matching elements, find
returns only the first element that satisfies the specified condition. If no element meets the condition, it returns undefined
.
How It Works ?
Consider the following array:
const numbers = [5, 12, 8, 130, 44];
Suppose we want to find the first number greater than 10. We can use the find
method with a callback function that specifies this condition:
const found = numbers.find(number => number > 10);
console.log(found); // 12
The callback function receives each element of the array in sequence. When it finds an element that satisfies the condition (number > 10
), it immediately stops and returns that element. If no such element exists, the result would be undefined
.
Finding Objects in an Array
The find
method is especially useful when working with arrays of objects. Consider the following example:
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
We can search for a user by their ID:
const user = users.find(user => user.id === 2);
console.log(user); // { id: 2, name: 'Bob' }
Here, find
efficiently retrieves the first object where id
equals 2
, making it a powerful tool for searching in complex data structures.
Handling Edge Cases
When using find
, it's important to handle cases where no element meets the condition. For example:
const result = users.find(user => user.id === 10);
console.log(result); // undefined
Since no user has an id
of 10
, the method returns undefined
. To prevent errors, you can implement a fallback:
const user = users.find(user => user.id === 10) || { message: 'User not found' };
console.log(user); // { message: 'User not found' }
Practical Challenges
Now, it's time to put your skills to the test! Consider the following array of products:
const products = [
{ name: 'Laptop', price: 1200 },
{ name: 'Phone', price: 800 },
{ name: 'Tablet', price: 500 }
];
Your task is to find the first product with a price greater than 1000. Implement the find
method to achieve this goal.
Conclusion
The find
method in JavaScript provides a simple yet effective way to search for elements within an array. Whether dealing with numbers, strings, or complex objects, find
enhances code readability and efficiency.
Stay tuned for more JavaScript insights, and happy coding!
Subscribe to my newsletter
Read articles from Rayane TOKO directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
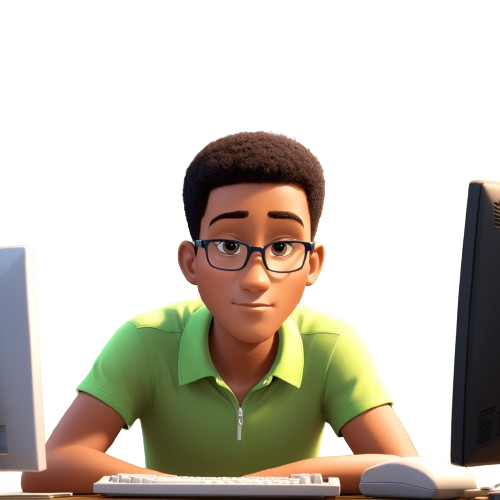
Rayane TOKO
Rayane TOKO
👨💻 Computer Scientist | 🌌 Physicist Enthusiast 💡 Always exploring new technologies and seeking to bridge the gap between science and technology.