Supabase + Next.js: Full-Stack Workflow

Table of contents
- Now, let's dive into it step by step!
- Why Supabase?
- Step-by-Step: Supabase on NextJS
- Build your very own Supabase’d NextJS Application in minutes by following these 5 steps.
- Step 1: Create Supabase Project
- Step 2: Create Next.js App with Supabase
- Step 3: Declare Supabase Environment Variables
- Step 4: Query Data from Supabase in Next.js App
- Step 5: Start Next.js Application
- Next Steps
- Value of Using Supabase with Next.js
- Congrats and Happy Coding!
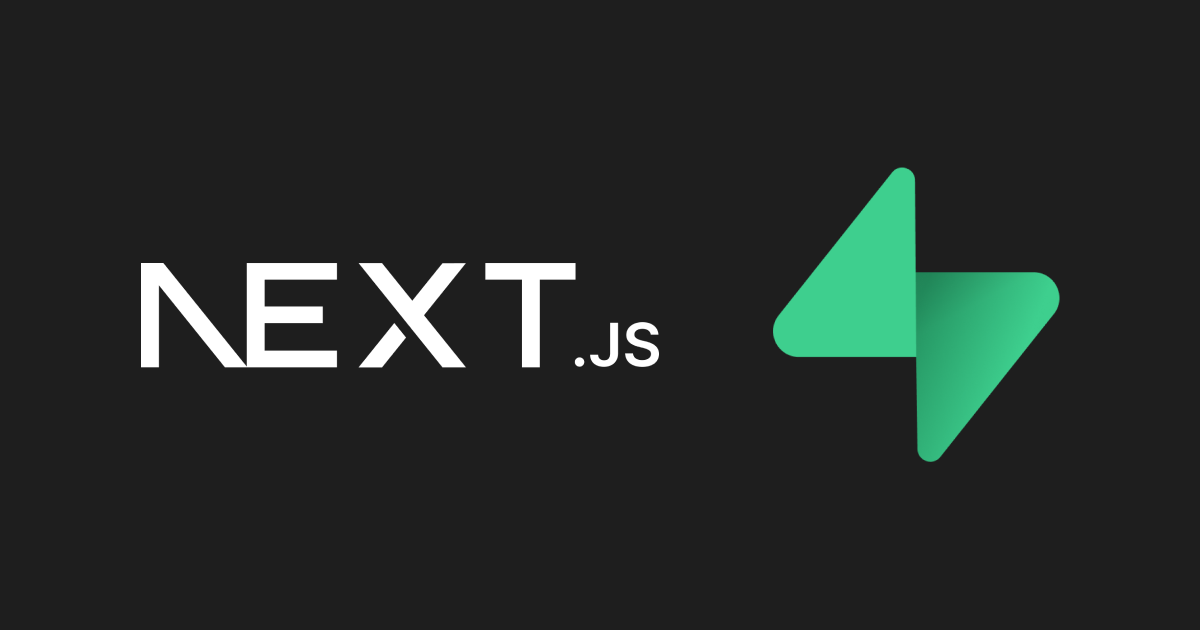
This tutorial will guide you through integrating Supabase with a Next.js application. You will learn how to set up a database with sample data and perform efficient queries.
Supabase is an open-source backend as a service (BaaS) that offers developers robust tools such as real-time databases, authentication, file storage, and more, all without the need for a separate backend server.
Establish and configure a Supabase project.
Set up a Next.js application pre-configured with Supabase.
Configure environment variables and perform queries on your database within your application.
Now, let's dive into it step by step!
Why Supabase?
Supabase empowers developers by simplifying backend processes with its multitude of features, such as:
PostgreSQL-backed database management: Add, query, and manage relational data.
Serverless functionality: Powered by SQL and APIs, no backend coding required.
Real-time updates: Reflect changes instantly across connected clients.
Authentication and Authorization: Built-in user management tools for seamless security.
Storage options: Manage file uploads and delivery.
Whether you're building a new application or enhancing an existing project, Supabase reduces development time while ensuring scalable backend functionality.
Step-by-Step: Supabase on NextJS
Build your very own Supabase’d NextJS Application in minutes by following these 5 steps.
Step 1: Create Supabase Project
Get started with Supabase by navigating to database.new and creating a new project. Once your project is running, navigate to the Table Editor inside the Supabase dashboard to create a new table. You can also run some SQL commands to set up a table with sample data.
SQL script to create an instruments
table with sample data
-- Create the 'instruments' table
create table instruments (
id bigint primary key generated always as identity,
name text not null
);
-- Insert sample data into the table
insert into instruments (name)
values
('violin'),
('viola'),
('cello');
-- Enable Row-Level Security for the table
alter table instruments enable row level security;
-- Make the table publicly readable
create policy "public can read instruments"
on public.instruments
for select
to anon
using (true);
Creates a table named
instruments
withid
andname
columns.Inserts three sample entries: violin, viola, and cello.
Sets up row-level security so only authenticated users have access.
Adds a policy allowing public “read-only” access to the instrument data.
Step 2: Create Next.js App with Supabase
Next, let’s create a Next.js app. You can use the Supabase template to set up a pre-configured project with TypeScript, Tailwind CSS, and cookie-based auth. Run the following command in your terminal:
npx create-next-app -e with-supabase
Step 3: Declare Supabase Environment Variables
Once your project is ready, you need to add Supabase configuration to your app, then simply rename the .env.example
file to .env.local
in your project root directory | cp .env.example .env
cp .env.example .env.local
Populate .env.local
with your Supabase Project URL and Anon Key found in your Supabase dashboard.
NEXT_PUBLIC_SUPABASE_URL=<YOUR_SUPABASE_PROJECT_URL>
NEXT_PUBLIC_SUPABASE_ANON_KEY=<YOUR_SUPABASE_ANON_KEY>
Step 4: Query Data from Supabase in Next.js App
Create file at app/instruments/page.tsx
and add the following code to fetch data from the instruments
table using Supabase's JavaScript SDK and render it on the page:
import { createClient } from '@/utils/supabase/server';
export default async function Instruments() {
// Initialize Supabase client
const supabase = createClient();
// Fetch data from the instruments table
const { data: instruments } = await supabase.from("instruments").select();
// Render the data
return <pre>{JSON.stringify(instruments, null, 2)}</pre>;
}
instruments
table and displays fetched JSON data. Style it or pass into components.Step 5: Start Next.js Application
yarn && # install dependencies
yarn dev # start dev server on localhost
Navigate to http://localhost:3000/instruments
in your browser. You should see the list of instruments (violin
, viola
, cello
) rendered on the page.
Next Steps
Now that you’ve successfully set up Supabase and integrated it into your Next.js app, here are some suggestions for what to explore next:
Authentication: Add user signup/login functionality to secure different parts of your app.
Data Management: Insert, update, and delete rows directly from your Next.js app.
File Storage: Use Supabase Storage to upload and serve assets like images, videos, or documents.
Custom APIs: Extend functionality using Supabase Edge Functions or integrate with third-party tools.
Deployment: We recommend Vercel to deploy your app online.
Log in to Vercel: connect your repository, and select your project.
Deploy to Vercel: review and adjust settings if needed, then click “Deploy”.
Vercel will build and deploy your app, giving you a live URL to share or test.
Benefits include: database integrations, the ability to seamlessly connect to custom domains, and serverless functions to enhance your development workflow.
Value of Using Supabase with Next.js
Rapid Prototyping
Supabase significantly streamlines the backend setup process, allowing developers to concentrate more on crafting the front end of their applications. This combination of Supabase with Next.js provides a robust and efficient foundation for developing web applications. It eliminates the need to deal with the complexities typically associated with backend development, enabling quicker iterations and faster time-to-market for new features and updates.
Flexibility and Scalability
Supabase is built on top of PostgreSQL, one of the most reliable and powerful relational database systems available. This ensures that your application benefits from robust data integrity and high efficiency. Moreover, as your application grows, Supabase can easily scale to accommodate increased data loads and user demands, providing a seamless experience without compromising performance.
Full-stack Capabilities
When paired with Next.js, Supabase offers a comprehensive full-stack solution that supports modern web development practices. This includes seamless integration of server-side rendering, which enhances performance and SEO, as well as efficient file storage solutions for managing assets like images and videos. Additionally, Supabase provides powerful APIs and robust authentication mechanisms, all of which are optimized to require minimal effort from developers, allowing them to focus on creating exceptional user experiences.
Open Source Platform
Supabase is an open-source platform, which means you have the freedom to customize and adapt every aspect of the stack to suit your specific needs. This transparency allows developers to inspect the underlying code, ensuring that they have complete control over their application's architecture.
By building on Supabase, you can leverage the benefits of open-source development, such as community support and continuous improvements, while maintaining the flexibility to tailor the platform to your unique requirements.
Congrats and Happy Coding!
You have successfully completed several important steps in your development journey!
Initialized and configured a Supabase project as the foundation for your backend services.
- Involves creating a project in Supabase, setting up your database, and configuring the necessary authentication and API settings to ensure your application can securely interact with your data.
Set up Next.js app and integrated it with Supabase to fetch and display data.
This includes creating a new Next.js project, connecting it to your Supabase instance, and writing the necessary code to retrieve data from your database and render it in your application.
This step is crucial for creating dynamic, data-driven web applications.
Explored capabilities of Supabase, which support the development of robust full-stack applications.
This exploration might include experimenting with Supabase's real-time features, using its built-in authentication system, or leveraging its storage capabilities to manage files.
By understanding these features, you can build powerful applications that are both scalable and maintainable.
By completing these steps, you have laid a strong foundation for developing modern web applications that are both efficient and scalable.
Keep exploring and building on this knowledge to create even more sophisticated applications in the future!
Are you ready to start building? Visit the Supabase Documentation for more advanced examples and use cases, or delve deeper into Next.js to create user-friendly interfaces.
Happy Coding <3
About the Author — Val Alexander
Full-Stack AI + Blockchain • DeDevs Founder • Developer Relations Engineer (DRE) @ Chainlink Labs
Valentina Alexander | 0xBuns.com
CoFounder of DeDevs Club
Developer Relations Engineer @ Chainlink Labs
Join our Online Community for Blockchain and AI Developers
Read More | Why Join DeDevs
Subscribe to my newsletter
Read articles from Valentina Alexander directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Valentina Alexander
Valentina Alexander
Cofounder of DeDevs, which serves as a hub for cutting-edge development, knowledge sharing, and collaboration on groundbreaking projects. Our community specifically focuses on the intersection of blockchain and AI technologies, providing a unique space where experts from both fields can come together to innovate and learn from each other.