A Beginner's Guide to the Builder Design Pattern
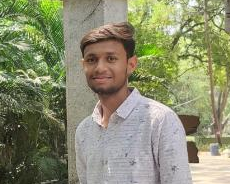
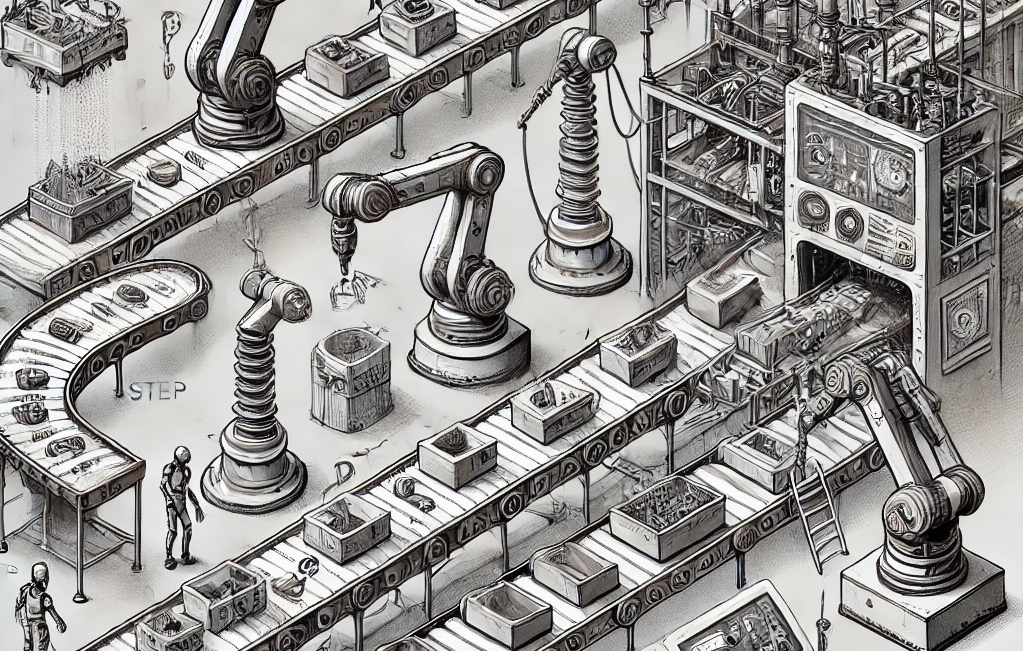
Introduction
The Builder Design Pattern is a way to create complex objects step by step. Instead of using a big constructor with many parameters, the Builder pattern offers a clear and easy-to-read method to build an object, keeping it organized.
Problems Solved by the Builder Pattern
When an object requires multiple parameters to be initialized, managing constructors becomes cumbersome. Some problems include:
Constructor Overloading: When a class has multiple constructors with different parameters, it can lead to confusion and complexity. Developers might struggle to remember which constructor to use in various situations, especially if the parameters are similar in type or number. This can result in errors or the need for extensive documentation to clarify the purpose of each constructor. The Builder pattern addresses this issue by providing a more intuitive and flexible way to create objects. It allows developers to set only the parameters they need, in a clear and step-by-step manner, reducing the likelihood of mistakes and making the codebase easier to maintain and understand.
Readability Issues: Too many parameters in a constructor reduce code clarity.
Immutability: Once an object is created, changing it can be challenging. This is because immutable objects are designed to remain unchanged after their initial creation. Any modification would require creating a new instance of the object with the desired changes. This means that developers need to carefully plan and manage how objects are constructed and used, as any change necessitates the creation of a new object.
Unnecessary Dependencies: Some object fields might be optional, leading to unnecessary initialization. For example, imagine a
Car
class with fields likeengineType
,color
,sunroof
, andGPS
. If a user wants to create a basic car without a sunroof or GPS, initializing these fields (even if it is NULL) would be unnecessary. This can lead to complex code. By using a pattern like the Builder, developers can choose to set only the fields they need, avoiding the initialization of optional features that aren't required for every instance.Code Maintainability: Hardcoded object creation logic can be difficult to manage and modify.
Analogy and Example
Imagine ordering a custom-built Car at a dealership. Instead of choosing a pre-built car, you specify each component: the type of engine, brand, model, color, etc. The dealer follows your instructions step by step and assembles the car accordingly.
Similarly, in software development, when creating a complex object with multiple optional attributes, the Builder pattern allows us to assemble the object incrementally and customize it as needed.
Implementation of Builder Pattern in Golang
Step 1: Define the Product
package main
import "fmt"
// Product we want to build
type Car struct {
Brand string
Model string
Year int
Color string
EngineCC int
}
Step 2: Create the Builder Interface
// CarBuilder interface defines the steps to build a car
type CarBuilder interface {
SetBrand(brand string) CarBuilder
SetModel(model string) CarBuilder
SetYear(year int) CarBuilder
SetColor(color string) CarBuilder
SetEngineCC(cc int) CarBuilder
Build() Car
}
Step 3: Implement a Concrete Builder
type CarBuilderImpl struct {
car Car
}
func NewCarBuilder() CarBuilder {
return &CarBuilderImpl{}
}
func (b *CarBuilderImpl) SetBrand(brand string) CarBuilder {
b.car.Brand = brand
return b
}
func (b *CarBuilderImpl) SetModel(model string) CarBuilder {
b.car.Model = model
return b
}
func (b *CarBuilderImpl) SetYear(year int) CarBuilder {
b.car.Year = year
return b
}
func (b *CarBuilderImpl) SetColor(color string) CarBuilder {
b.car.Color = color
return b
}
func (b *CarBuilderImpl) SetEngineCC(cc int) CarBuilder {
b.car.EngineCC = cc
return b
}
func (b *CarBuilderImpl) Build() Car {
return b.car
}
Step 4: Introducing the Director Class
The Director class is optional in the Builder pattern. Its role is to manage the building process and ensure objects are created in a specific order. The Director helps keep the building steps separate from the code that uses the final product. In our case, we can have different types of directors, such as one that builds a sports car, another that builds a luxury car, and so on.
Buyers can build cars using the existing directors or using the builder themselves.
Example Implementation:
type CarDirector struct {
builder CarBuilder
}
func NewCarDirector(builder CarBuilder) *CarDirector {
return &CarDirector{builder: builder}
}
func (d *CarDirector) ConstructSportsCar() Car {
return d.builder.SetBrand("Ferrari").SetModel("F8").SetYear(2023).SetColor("Red").SetEngineCC(3900).Build()
}
Step 5: Using the Builder and Director
func main() {
builder := NewCarBuilder()
director := NewCarDirector(builder)
sportsCar := director.ConstructSportsCar()
fmt.Printf("Sports Car Built: %+v\n", sportsCar)
}
Usability
The Builder pattern is useful in the following scenarios:
When an object has too many parameters, especially optional ones.
When an object requires step-by-step initialization.
When multiple variations of an object are needed.
When improving code maintainability by separating object creation from its representation.
When a consistent object construction process is needed (using a Director).
Summary: Pros and Cons
Pros:
✅ Improves Code Readability: The step-by-step construction makes the object creation process more readable.
✅ Flexible Object Creation: Supports different configurations of the same object.
✅ Reduces Constructor Overloading: Avoids large and confusing constructors.
✅ Encapsulates Construction Logic: Helps keep object creation logic separate from business logic.
✅ Director Ensures Consistency: Provides a standard way to create predefined objects.
Cons:
❌ More Code: Requires additional classes/interfaces, which can add complexity.
❌ Not Always Necessary: For simple objects, a builder might be overkill.
❌ Less Flexible with Director: If used, the Director can enforce a strict object construction process that may not fit all use cases.
Conclusion
The Builder pattern helps in constructing complex objects in a structured and readable way. We can ensure better maintainability and avoid cumbersome constructors. It is widely used in real-world applications, especially in designing APIs, UI components, and assembling complex objects in system design.
Subscribe to my newsletter
Read articles from Darsh Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
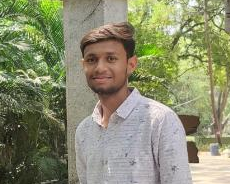