Understanding the Call Stack, setTimeout, and the Importance of bind in JavaScript

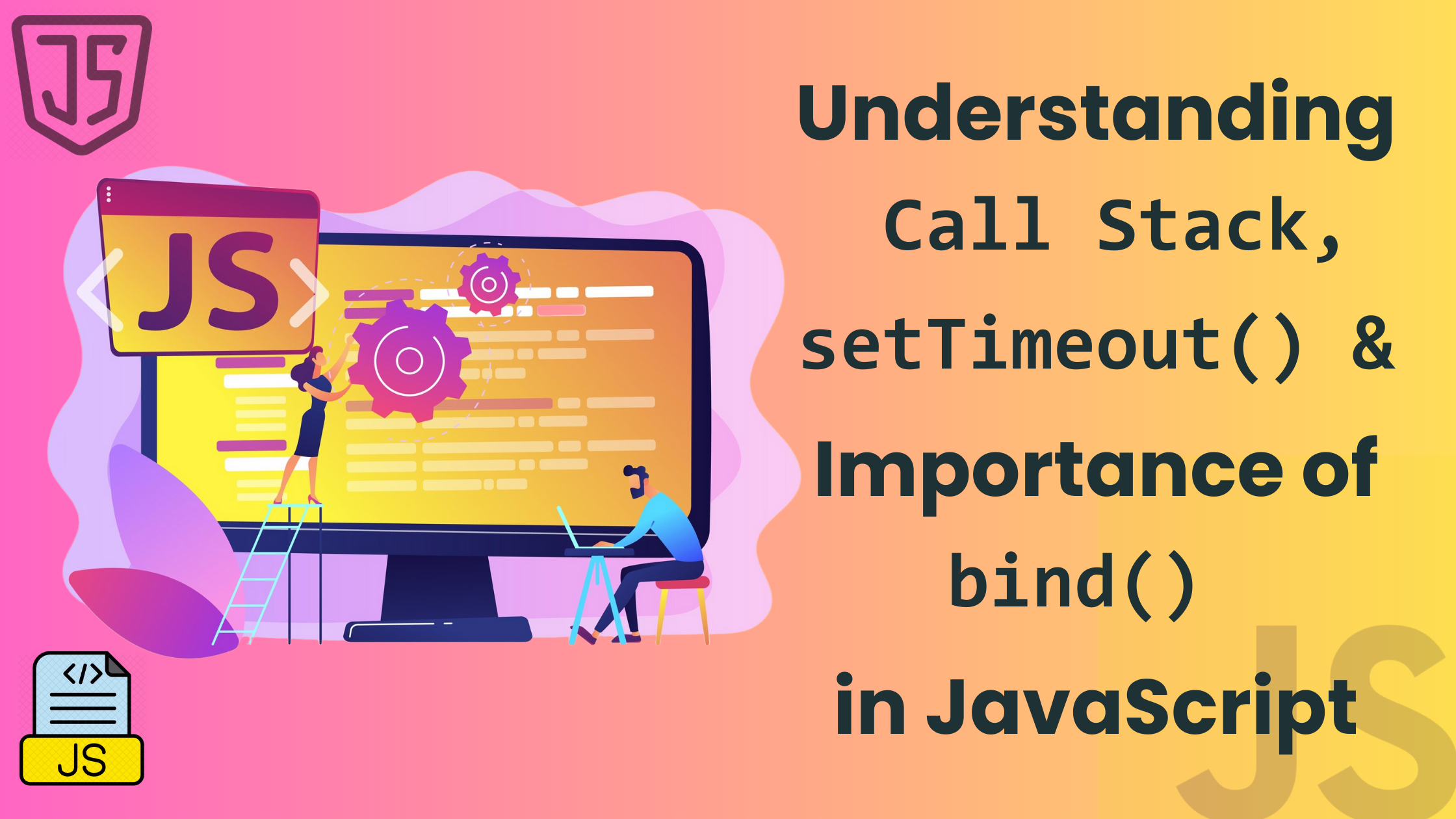
Call Stack: The Backbone of JavaScript Execution
JavaScript operates as a single-threaded language, meaning it executes one operation at a time within a single call stack. This Call Stack is a fundamental data structure that tracks function execution and follows the Last In, First Out (LIFO) principle. Whenever a function is called, it gets pushed onto the stack, and once it completes, it is popped off. This strict execution order ensures synchronous code runs predictably, but it also creates challenges when dealing with asynchronous operations, which require additional mechanisms like the Event Loop to prevent blocking and ensure responsiveness.
Key Properties of Call Stack:
Functions are pushed into the stack when called.
Functions are popped out when execution is completed.
The stack waits for nothing—it executes synchronously.
Event Loop
JavaScript has a pretty smart way of handling asynchronous tasks, thanks to its Event Loop. Even though it runs on a single thread, it never feels slow or blocked—ever wondered how?
Well, the Event Loop is always keeping an eye on the Call Stack. The moment it notices the stack is empty, it jumps in and starts moving pending tasks from the Callback Queue so they can be executed in order.
This whole system is what makes JavaScript's concurrency model so efficient. It ensures tasks are scheduled properly, avoids execution bottlenecks, and keeps resources from clashing. Because of this event-driven approach, JavaScript can smoothly handle things like user interactions, I/O operations, and scheduled tasks—all without messing up the main execution thread. That’s exactly why it’s perfect for building fast, interactive web applications!
What is Callback Queue ?
Whenever an asynchronous task—like setTimeout()
, fetching data using fetch()
, or handling events like click
and keydown
—finishes, its callback doesn’t execute immediately. Instead, it gets placed in this queue, waiting for its turn.
But here’s the catch—it won’t run right away! The Event Loop makes sure it executes only when the Call Stack is completely free. This is how JavaScript smoothly handles async tasks without blocking execution, ensuring everything stays responsive.
So, whether it’s a timer, an API call, or a user event, the Callback Queue plays a crucial role in managing them all efficiently! 🚀
The Reality of setTimeout() in Browsers
Contrary to popular belief, setTimeout()
is not a feature of JavaScript itself. It is provided by the Web APIs in browsers or Node.js runtime. It acts as a scheduler that defers the execution of a function until after a specified delay. However, the actual execution time is not precise, as it depends on the current workload of the event loop. The setTimeout() specifies the only minimum delay time.
Execution Flow of setTimeout()
:
The
setTimeout()
function is invoked and registered within the Web API environment.The JavaScript engine does not pause execution but instead continues running the next synchronous code immediately.
Once the specified time delay elapses, the callback function is added to the Callback Queue, a designated area where asynchronous tasks wait to be executed. However, this does not mean that the callback runs immediately; it only becomes eligible for execution.
The Event Loop continuously monitors the Call Stack. When the stack is empty, it transfers the pending callback from the Callback Queue into the stack, ensuring orderly execution.
Only when the event loop identifies an empty call stack does it move the scheduled callback into execution.
Since JavaScript runs in a single-threaded environment, if the main thread is busy executing other tasks, the setTimeout()
callback may experience additional delays beyond the specified timeout.
Dry Run of Code Execution
Consider the following code:
console.log("hello JS");
setTimeout(() => console.log("A B C"), 0);
console.log("Bye bye");
Step-by-Step Execution:
console.log("hello JS")
is added to the call stack and executed. Output:hello JS
setTimeout()
is encountered, and the browser starts a timer (with 0 ms delay) but does not execute the callback immediately. It movessetTimeout
to the Web API environment.console.log("Bye bye")
is added to the stack and executed. Output:Bye bye
The call stack is now empty, so the event loop checks the Callback Queue and moves
console.log("A B C")
to the stack.console.log("A B C")
executes. Final output:hello JS Bye bye A B C
Why Doesn't A B C
Print First?
Even though the delay is 0 ms
, setTimeout
does not execute immediately—it waits until the stack is clear, following the event loop mechanism that has been discussed previously.
How Long Does setTimeout() Actually Wait?
console.log("hello JS");
setTimeout(() => console.log("A B C"), 1000 * 10);
console.log("Bye bye");
// Assume this runs 100 million times
for (let i = 0; i < 1e8; i++) {
console.log("Bye bye");
}
What Happens?
console.log("hello JS")
executes.setTimeout()
registers a 10-second timer.console.log("Bye bye")
executes millions of times, blocking the main thread.After 10 seconds,
A B C
is ready but must wait until the call stack is empty.Since the for-loop runs for ~3 hours,
A B C
is delayed.
Expected Output:
hello JS
Bye bye
Bye bye // (Repeated ~100 million times)
A B C // Appears after 3 hours, not 10 seconds!
The Role of bind
in setTimeout()
What Happens Without bind
?
Let’s look at this example:
const obj = {
personName: "Akash",
greet: function(){
console.log(`Hello, ${this.personName}`);
},
};
console.log("Hi");
setTimeout(obj.greet, 5 * 1000);
console.log("bye");
Expected Output:
Hi
bye
Hello, undefined // `this` is lost in setTimeout
Why Does this
Become undefined
?
When we do setTimeout(obj.greet, 5000);
, JavaScript doesn’t execute greet
immediately. Instead, it takes the function reference and schedules it. But when greet
runs later, it’s not attached to obj
anymore—it’s called as a regular function.
So, when
greet
executes later, it is not called onobj
but as a regular function.In non-strict mode,
this
in a regular function call defaults to the global object (window
in browsers,global
in Node.js).In strict mode,
this
becomesundefined
instead of referring to the global object.
Fixing It with bind
To ensure this
stays linked to obj
, we can explicitly bind it:
setTimeout(obj.greet.bind(obj), 5 * 1000);
New Expected Output:
Hi
bye
Hello, Akash // `bind` preserves `this`
How Does bind
Work Here?
.bind(obj)
returns a new function where this
is permanently set to obj
. So when setTimeout
calls this new function, this
remains obj
, and we get the expected behavior.
This trick is super handy whenever you need to keep this
intact in delayed function executions! 🚀
Still Have a doubt ?
That’s a great question ! The reason greet
is no longer attached to obj
when passed to setTimeout
is that JavaScript treats functions as first-class citizens, meaning functions can be assigned to variables, passed as arguments, and executed independently of their original context.
Here’s what happens step by step:
Function Reference Extraction
setTimeout(obj.greet, 5000);
Here, we’re passing only the function reference
obj.greet
tosetTimeout
, not calling it immediately.JavaScript essentially does something like this under the hood:
const func = obj.greet; // Function reference is copied setTimeout(func, 5000); // `func` is now detached from `obj`
Loss of
this
ContextWhen
setTimeout
executesfunc
after 5 seconds, it’s now a standalone function call.Since it's no longer called as
obj.greet()
, JavaScript doesn’t associatethis
withobj
.In non-strict mode,
this
falls back to the global object (window
in browsers,global
in Node.js).In strict mode,
this
becomesundefined
.
How to Preserve
this
?To prevent
this
from getting lost, we use.bind(obj)
, which creates a new function withthis
permanently set toobj
:setTimeout(obj.greet.bind(obj), 5000);
Now, even though
setTimeout
calls the function later,this
still refers toobj
, ensuring the correct behavior.
Summary
JavaScript’s Call Stack executes functions synchronously, without waiting for any asynchronous operations.
setTimeout() does not run precisely after the specified delay—it depends on the Event Loop and Call Stack availability.
The Callback Queue temporarily holds delayed functions until the Event Loop moves them to execution.
bind()
ensures thatthis
remains correctly linked to its object, preventing unexpected behavior in asynchronous calls likesetTimeout()
.
Understanding these concepts will help you write more efficient and bug-free JavaScript code!
Bonus Section: A Real World Analogy
Scenario Without bind
(Losing this
)
Imagine you’re at a restaurant, and a waiter (your function greet
) takes your order.
You (the object
obj
) tell the waiter:"I want a Pizza."
Now, instead of bringing the order immediately, the waiter forgets to write down your table number and just hands the order (the function reference) to another waiter (setTimeout).
setTimeout(obj.greet, 5000);
After 5 minutes, the new waiter brings the order but doesn’t know whose order it was!
He randomly asks, "Who ordered this?"
Since there’s no table assigned, the system defaults to "undefined".
That’s why, when setTimeout
executes, you get:
Hello, undefined
because the function forgot who it belonged to!
Scenario With bind
(Keeping this
)
Now, what if the first waiter writes down your table number (binds this
to obj
) before handing the order over?
setTimeout(obj.greet.bind(obj), 5000);
Now, after 5 minutes, the second waiter knows exactly where to take the order—straight to your table!
So when setTimeout
executes, the output is:
Hello, Akash
because the function remembers who it belongs to.
Without
bind
, the function (waiter) forgets who it belongs to, leading toundefined
.With
bind
, the function is permanently attached to the original object (table), so it always works correctly.
Subscribe to my newsletter
Read articles from Santwan Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santwan Pathak
Santwan Pathak
"A beginner in tech with big aspirations. Passionate about web development, AI, and creating impactful solutions. Always learning, always growing."