Mastering Debouncing and Throttling in React: Optimize Performance Like a Pro

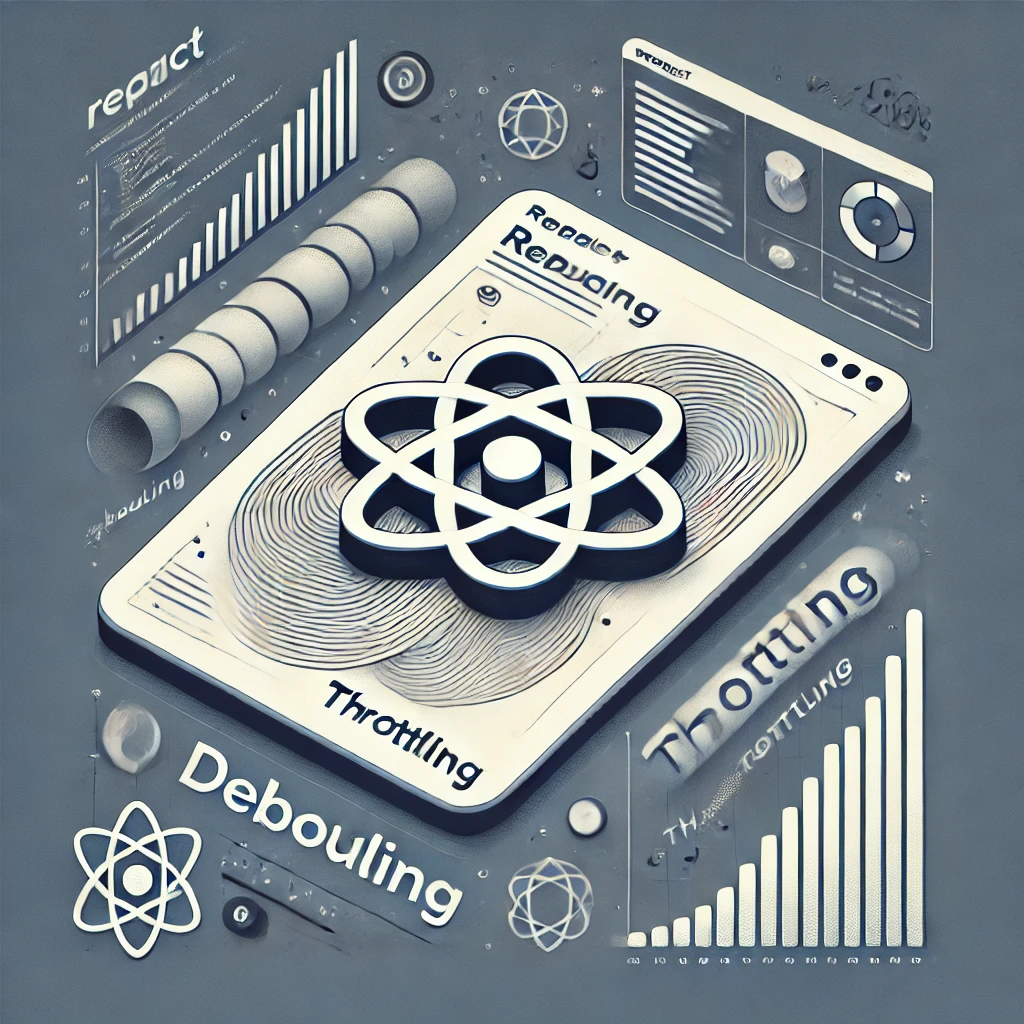
Performance optimization is a critical aspect of building smooth and efficient React applications. Two essential techniques for handling frequent function executions are debouncing and throttling. These techniques help manage performance by limiting how often expensive operations (like API calls or event handlers) are executed.
In this article, we'll dive into the differences between debouncing and throttling, when to use each, and how to implement them effectively in React applications.
🚀 What is Debouncing?
Debouncing is a technique that ensures a function is only executed after a certain delay has passed since the last time it was invoked. This is especially useful for input fields, search bars, or resizing events where rapid consecutive changes can lead to unnecessary computations.
✅ Example: Debouncing a Search Input
When implementing a search feature, debouncing prevents excessive API requests by delaying execution until the user stops typing for a specific duration.
Using Lodash’s debounce
:
import React, { useState, useEffect, useCallback } from "react";
import debounce from "lodash.debounce";
const SearchComponent = () => {
const [query, setQuery] = useState("");
const fetchResults = (searchTerm) => {
console.log("Fetching results for:", searchTerm);
// Call an API or perform an expensive operation here
};
const debouncedFetchResults = useCallback(
debounce((searchTerm) => fetchResults(searchTerm), 500),
[]
);
useEffect(() => {
if (query) {
debouncedFetchResults(query);
}
}, [query, debouncedFetchResults]);
return (
<input
type="text"
placeholder="Search..."
value={query}
onChange={(e) => setQuery(e.target.value)}
/>
);
};
export default SearchComponent;
🛠 How It Works
The
debouncedFetchResults
function ensures that the API call is only triggered 500ms after the user stops typing.useCallback
ensures the function reference remains stable across renders.
⚡ What is Throttling?
Throttling limits the execution of a function to once per specified time interval, even if the event is continuously triggered. This is particularly useful for performance optimization when handling scroll, resize, or mouse move events.
✅ Example: Throttling a Scroll Event
Imagine you have a feature that tracks user scrolling. Instead of firing the event on every pixel change, you can throttle it to execute at most once every 300ms.
Using Lodash’s throttle
:
import React, { useEffect } from "react";
import throttle from "lodash.throttle";
const ScrollTracker = () => {
useEffect(() => {
const handleScroll = throttle(() => {
console.log("User scrolled! Current position:", window.scrollY);
}, 300);
window.addEventListener("scroll", handleScroll);
return () => window.removeEventListener("scroll", handleScroll);
}, []);
return <div style={{ height: "200vh", padding: "20px" }}>Scroll Down</div>;
};
export default ScrollTracker;
🛠 How It Works
The
handleScroll
function is throttled to execute once every 300ms, even if the user continuously scrolls.This prevents excessive calculations and improves performance.
🔥 Debouncing vs. Throttling: When to Use What?
Feature | Debouncing | Throttling |
Definition | Delays function execution until after a pause in activity. | Ensures function runs at most once per specified interval. |
Best Use Cases | User input handling (e.g., search bar, form validation) | Scroll events, resizing, rate-limiting API calls |
Example | API search requests after typing | Handling window scroll events |
✅ Best Practices
Use Lodash: Lodash provides optimized
debounce
andthrottle
functions that are easy to use.Use
useCallback
anduseEffect
: These hooks ensure that the debounced/throttled function persists correctly across renders.Choose wisely: Use debouncing for user input and throttling for scroll/mouse events to optimize performance.
Cleanup event listeners: Always remove event listeners when using throttling to prevent memory leaks.
🎯 Conclusion
Debouncing and throttling are essential techniques for optimizing React applications. Using these wisely will enhance performance, reduce unnecessary re-renders, and improve the overall user experience. Whether handling API requests, scroll events, or UI interactions, implementing these techniques correctly ensures your app runs smoothly.
📌 What’s Next?
Try using React’s built-in
useDeferredValue
for similar optimizations.Experiment with custom hooks to encapsulate debounce and throttle logic.
Implement server-side debouncing to further reduce API load.
🚀 What’s your favorite use case for debouncing or throttling in React? Let me know in the comments!
Subscribe to my newsletter
Read articles from Youssef El naggar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
