Improve Your App's Performance: 5 Effective Tips
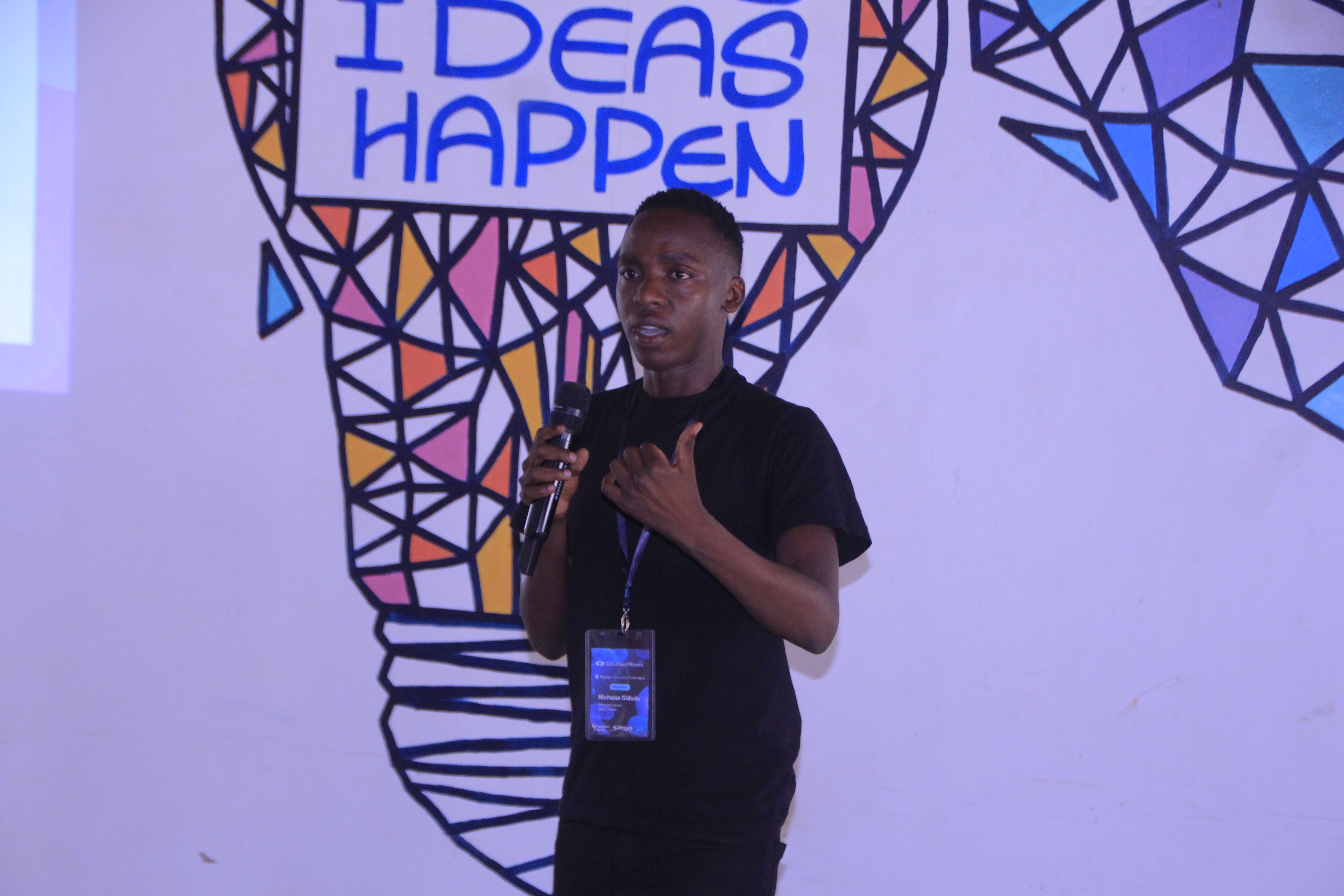
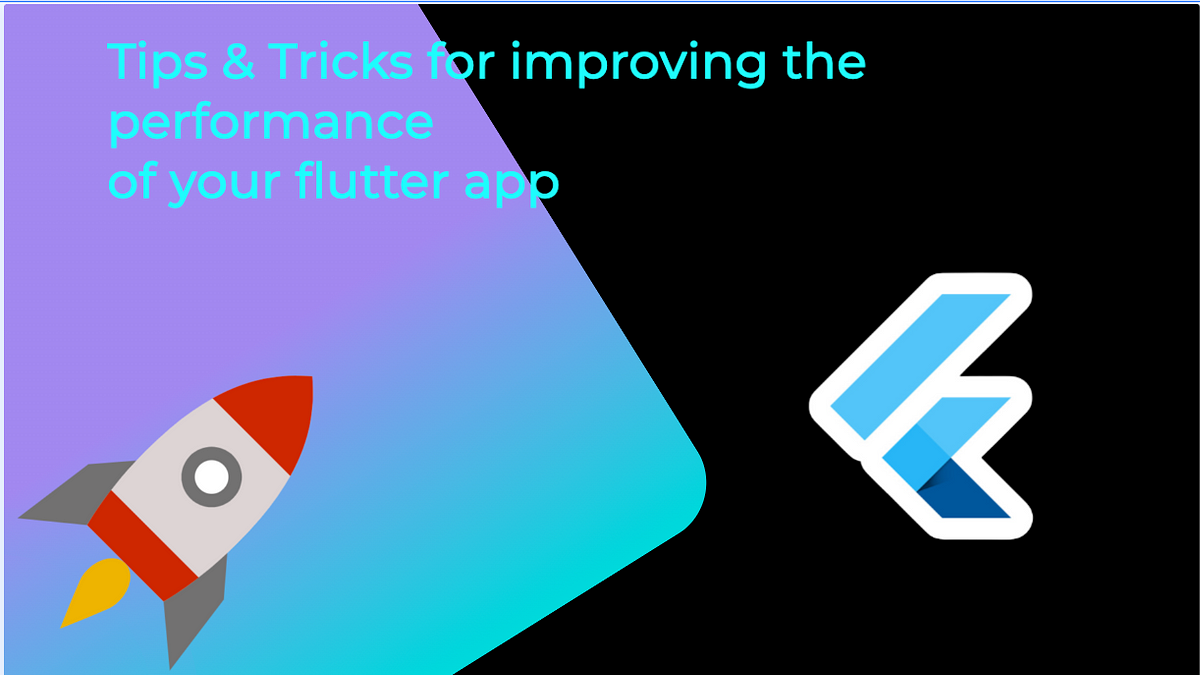
If you’re looking to make your Flutter app faster and smoother, you’re in the right place. Improving performance isn’t just about fancy tech—it’s about giving your users the best experience possible. Let’s walk through five practical tips that can make a difference in your app performance.
1. Use const Constructors & Embrace Immutable Widgets
What’s the deal?
Using const
constructors means Flutter can set up parts of your app at compile time instead of doing all the work when your app runs. Plus, immutable widgets (ones that don’t change) mean fewer unnecessary rebuilds, saving time and memory.
How to make it happen:
Mark any widget that doesn’t change with
const
.Stick to immutable data patterns for widget properties.
Example:
// Before:
Widget build(BuildContext context) {
return Text('Hello, Nico walter!');
}
// After:
Widget build(BuildContext context) {
return const Text('Hello, Nico walter!');
}
2. Keep Widget Rebuilds in Check with Smart State Management
Why care?
When too many parts of your app rebuild unnecessarily, it can slow things down. By managing state wisely, you ensure only the pieces that need to update do so, keeping your app running smooth.
Easy steps to try:
Consider state management solutions like Provider, Riverpod, or Bloc.
Use widgets like
Consumer
orSelector
so only the parts that need updating can rebuild.
Remember:
It’s best not to wrap your whole widget tree in one stateful widget. Instead, break it down so that only the affected areas refresh when something changes.
3. Get to Know Your App with Flutter DevTools
Why it matters:
Sometimes, performance hiccups aren’t obvious. Flutter DevTools is like a health check-up for your app—it helps you see what’s slowing things down, from heavy rebuilds to layout issues.
What to do:
Use the performance profiler to track frame rendering times.
Check out the widget rebuild stats and look out for janky frames.
Use the timeline view to spot any slow operations.
Tip:
Keep performance benchmarks handy so you can measure improvements after each tweak.
4. Streamline Your Assets and Animations
What’s up?
Large images or overly complicated animations can bog down your app, especially on less powerful devices. Optimizing your assets means your app will be leaner and faster.
Simple fixes include:
Compress and resize images before bundling them with your app.
Use the
FadeInImage
widget for smooth image transitions.Offload heavy tasks from the UI thread—try using the
compute
function for complex operations.
Extra tip:
Stick to Flutter’s built-in animation tools like AnimatedBuilder
for smooth, hardware-accelerated animations without the extra hassle.
5. Simplify Your Widget Tree and Cut Down on Overdraw
What’s the benefit?
A super deep or overly complex widget tree can slow down how quickly your app updates its UI. Keeping things simple helps Flutter render your app faster.
How to simplify:
Flatten your widget hierarchy whenever you can.
Avoid unnecessary nesting or redundant layouts.
Use the Flutter Inspector to get a clear look at your widget tree and spot areas to simplify.
Quick tip:
Reuse custom widgets and combine similar ones to keep your tree neat and efficient.
Wrapping Up
Taking your Flutter app’s performance to the next level is all about making smart tweaks. Whether it’s using const
constructors, managing state better, getting familiar with DevTools, optimizing your assets, or simplifying your widget tree, every little bit helps. Tackle these improvements one step at a time, and you’ll soon notice a smoother, faster user experience that sets your app apart.
Happy coding!
Subscribe to my newsletter
Read articles from Nicholas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
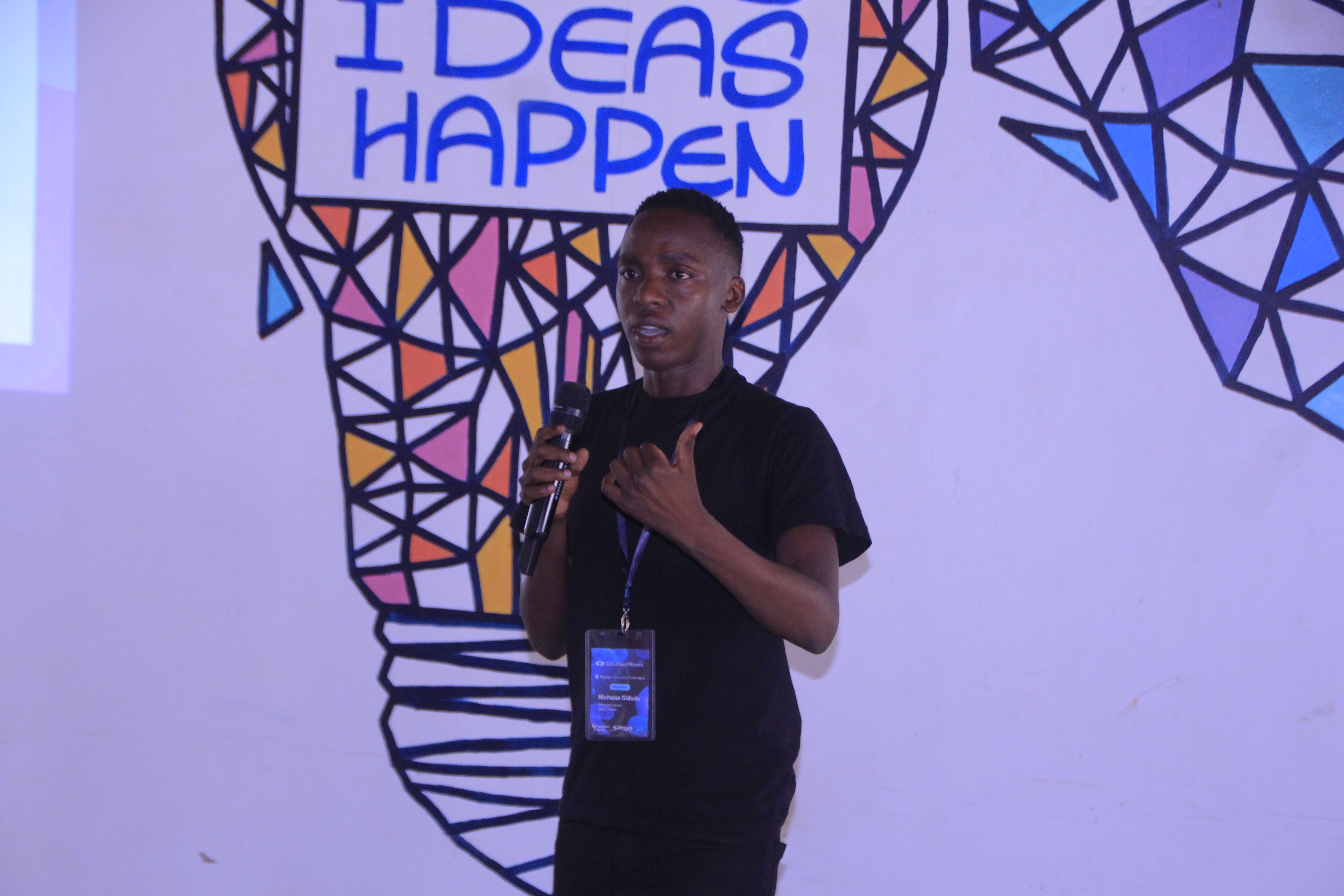
Nicholas
Nicholas
Hello, I am a senior Flutter developer with vast experience in crafting mobile applications. I am a seasoned community organizer with vast experience in launching and building Google Developer communities under GDG Bugiri Uganda and Flutter Kampala.