Detailed steps to create and modify EC2 instance using Terraform
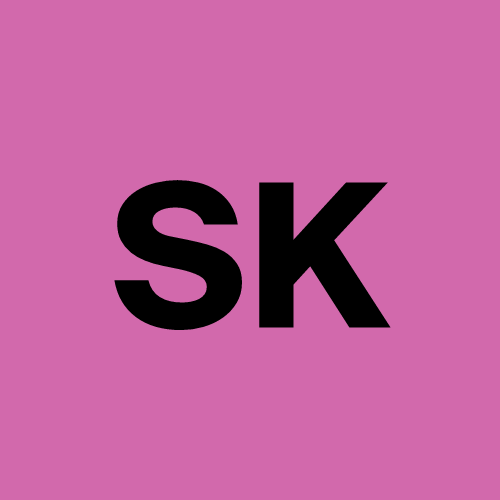
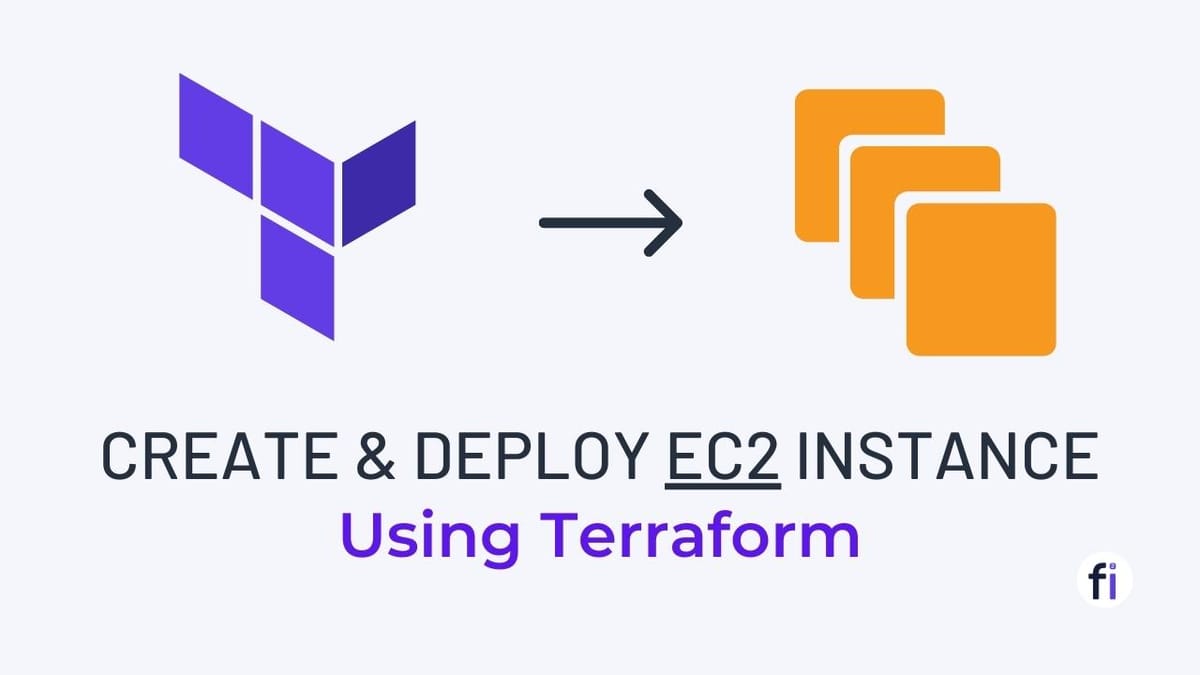
EC2 instance
Amazon Ec2(Elastic Compute Cloud) is a web service provided by AWS(Amazon Web Services) that allows us to rent virtual servers (known as instances) on which we can run our applications. EC2 instances offer a scalable computing capacity in the cloud, allowing users to easily scale up or down based on demand.
Instead of having the whole hardware for our Servers in on-premises, which costs so much and difficult to maintain and to face many challenges to provide security to our Servers, we can just have our Virtual Server (EC2 instance) with few clicks within no time.
Terraform
Terraform is an IAC(Infrastructure As Code) tool that helps us to automate the creation and management of cloud resources like servers, databases and networks.
Instead of manually creating resources in our cloud, we can write Terraform code based on how our infrastructure requirements.
The main advantage of Terraform is we can create our infrastructure in any cloud like AWS, Azure, Google Cloud, Kubernetes and also in on-premises.
Other IAC tool in AWS
We have CloudFormation service in AWS, using this we can create our infrastructure in AWS.
The main disadvantage of using CloudFormation is, we can create resources only in AWS.
So, when we are 100% sure that we use only AWS for infrastructure, then we can go with AWS CloudFormation. Otherwise, it is better to create infrastructure through Terraform, as we can create some resources in one cloud provider and some other in another cloud providers and some resources in On-premises. Terraform is very flexible to deploy resources wherever we want .
Step1: How to Download and Install Terraform (Step-by-Step Guide) in Windows
There are different ways to install Terraform on Windows, macOS and Linux.
Now we see, how to download Terraform in Windows using Binary.
i) Download Terraform Binary
Go to the official Terraform website: https://developer.hashicorp.com/terraform/downloads
Download the Windows version.
ii) Extract and move Binary to System Path
Extract the downloaded ZIP file to a folder (e.g.,
C:\terraform
).Add the folder to the system
PATH
environment variable:Open Control Panel → System → Advanced system settings.
Click Environment Variables → Under System Variables, find Path → Edit → Add
C:\terraform
.
Now we can use Terraform from any folder in our System, as we added path in Environment Variables.
Step2: Where to write the code
We can write Terraform code even in Notepad. But the best way to write it is in any IDE, as it is easy to find Syntax errors or Indentation errors.
I prefer to write it in Visual Studio Code. It’s good to install an extension called “ HashiCorp Terraform ” as it shows our code in different colors to identify the mistakes easily.
Step3: Write Terraform code based on documentation
Terraform documentation link for Ec2: https://registry.terraform.io/providers/hashicorp/aws/latest/docs/resources/instance
Go to this link and we find examples on how we can create Ec2 instance using Terraform code.
With the help of documentation we can easily write Terraform code to create Ec2 instance in our AWS account.
Here is the code:
provider "aws" {
region = "us-west-2"
access_key = "AKIAQL*******WR3O4SH"
secret_key = "aRsZ2dhL+**********pV0Y0FnN"
}
resource "aws_instance" "my_first_ec2" {
ami = "ami-0b6d6dacf350ebc82"
instance_type = "t2.micro"
}
However, it's not a good practice to hardcode AWS access keys in your Terraform code. Instead, it's safer to store them in environment variables or configuration files like terraform.tfvars.
Code Explanation
• As we want to deploy our code in AWS, we have to give provider as “aws”.
We have so many providers. You can find the list of providers in this link:
https://registry.terraform.io/browse/providers
• We have to provide the region, where we want to deploy our ec2 instance. Here i provided “us-west-2” as i want to deploy here.
• Access_key and secret_key are used for authentication. These are used to securely connect Terraform to our AWS account.
Here is the link for documentation:
https://registry.terraform.io/providers/hashicorp/aws/2.70.1/docs#authentication
• aws_instance is the resource type.
• my_first_ec2 is the resource name, which can be used only within Terraform file for reference.
• AMI (Amazon Machine Image) is a pre-configured virtual machine image in Amazon Web Services (AWS) that contains the operating system and applications needed to launch and run instances on AWS EC2 (Elastic Compute Cloud). It acts as a template for creating new EC2 instances.
AMI id is different for different regions, even for the same image. We can find the AMI Id in AMI Catalog in AWS account.
• instance_type refers to the configuration of compute resources (CPU, memory, storage, and networking capacity) that you can choose when launching an EC2 instance. Each instance type is designed to meet specific workloads and performance requirements.
Based on the our requirements we have to select instance type like t2.micro, t3.medium etc.
Step4: Use Terraform commands to create resources
After writing terraform code in visual studio. Open command prompt and go to the path where our terraform files are. Now, follow the below steps to create a resource(EC2 instance) and modify it.
i) terraform init - It will download appropriate plugins associated with the provider we have defined. Our case, it is AWS.
so that Terraform will integrate and connect with our AWS account to deploy our resources that we defined.
ii) terraform plan - It will show us, what resources it will create/ destroy in AWS or any environment, based on the code we specify on our Terraform file.
Now we should verify whether everything is correct like AMI ID, Instance type etc. Once we have verified that everything is correct, then create and resources in AWS.
This command will not create any resources. This will show the list of creating or updating or deleting resources.
iii) terraform apply - It will create resources.
At the end of this list, it will provide a prompt for confirmation to proceed further to perform these actions. We have to enter “ yes ”. Then it will start creating resources.
Now, the resources have been created in AWS.
Step5: Check created resources in AWS Console
Now we can go and check in AWS console for the resources we created.
Step6: Modify EC2 Instance using Terraform
Now we want to give our EC2 instance, a name.
We can do that by adding tags in our Terraform file.
provider "aws" {
region = "us-west-2"
access_key = "AKIAQ*****GWR3O4SH"
secret_key = "aRsZ2dhL+U*********pXs6bHZ4V0Y0FnN"
}
resource "aws_instance" "my_first_ec2" {
ami = "ami-0b6d6dacf350ebc82"
instance_type = "t2.micro"
tags = {
Name = "my-first-ec2"
}
}
Now give the command terraform plan.
Here, it shows that 1 change is there in our Terraform file and the change is related to Tags.
After we review this, we can do terraform apply.
Now, we can see the name for our ec2 instance.
Conclusion:
In this blog, we covered the detailed steps to create and modify an EC2 instance using Terraform. By following these steps, you can easily automate the management of your EC2 instances, making your infrastructure more efficient and consistent.
Terraform simplifies the process by using code to define and manage resources, enabling you to quickly launch, update, or delete instances as your needs change.
With these skills, you can confidently create EC2 instances using Terraform, saving time and reducing the potential for errors.
Thanks note:
Thank you for visiting my blog! I hope you found the steps helpful. If you enjoyed this post, feel free to follow me for more interesting and useful information. All my blogs will be in simple, general English, making it easy to understand. Stay tuned for more updates!
Subscribe to my newsletter
Read articles from Surekha Kokatam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
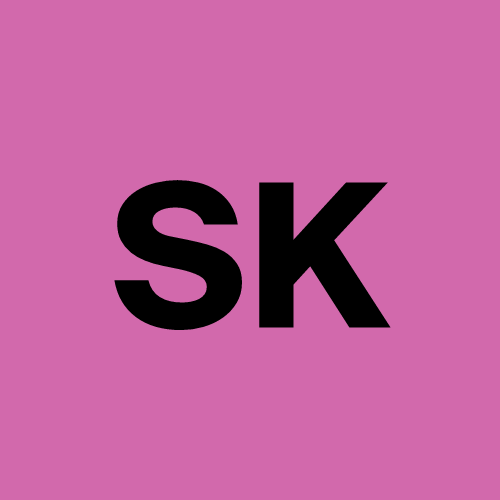