The Inner Workings of Python: A Deep Dive

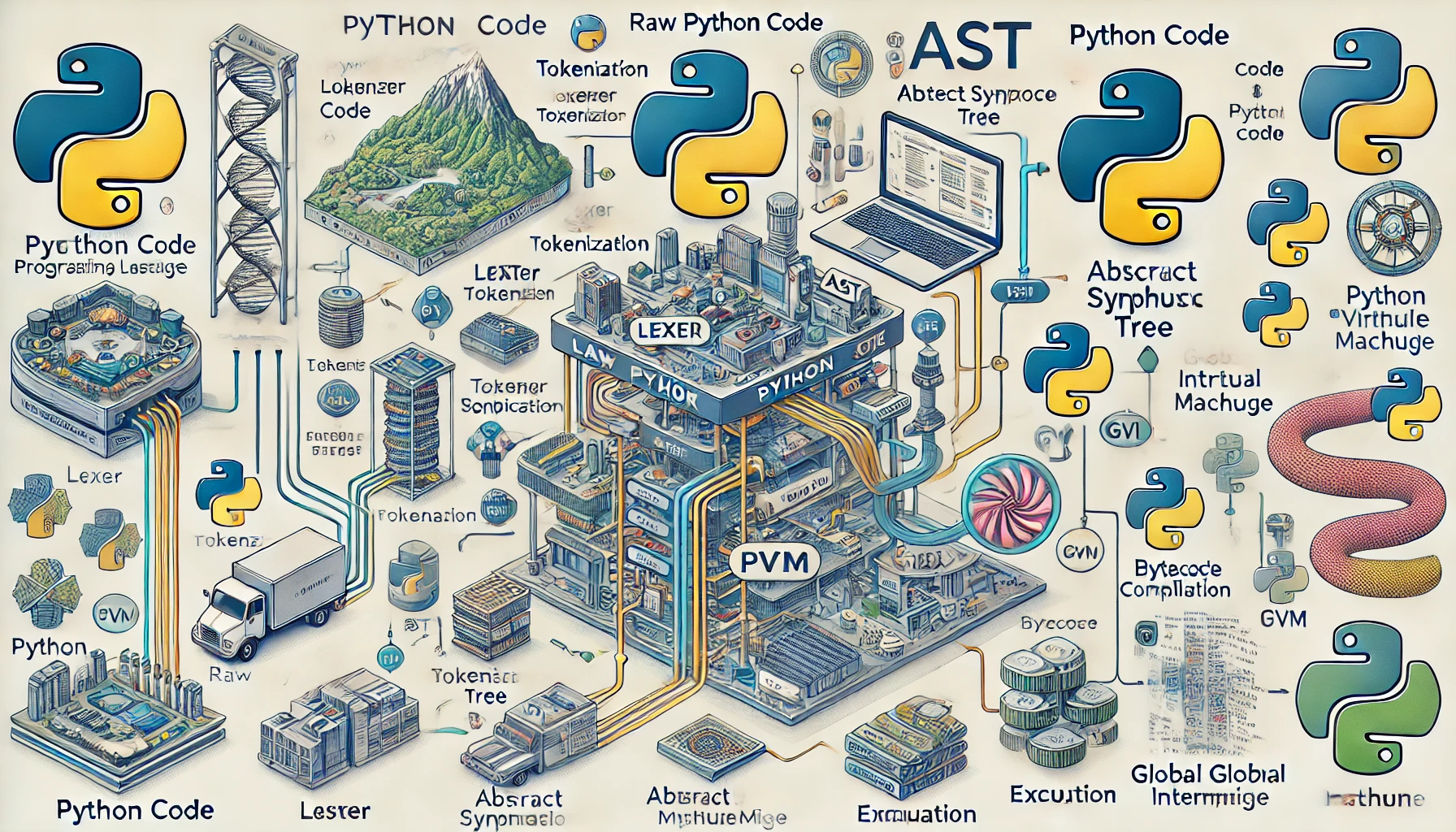
Python is one of the most popular programming languages today, known for its simplicity, readability, and extensive libraries. But what happens behind the scenes when you run a Python program? Understanding Python's inner workings can help developers write more efficient code, fix performance issues, and better use its features. In this blog, we will explore the internals of Python, including the interpreter, memory management, and the Global Interpreter Lock (GIL).
1. Python Interpreter
Python is an interpreted language, meaning the code is executed line-by-line rather than being compiled into machine code first. The standard implementation of Python is CPython, which interprets and runs Python code through these steps:
Step 1: Parsing and Tokenization
When you run a Python script, the interpreter first converts it into a series of tokens. These tokens represent different elements of the language, such as keywords, operators, and variables.
Step 2: Abstract Syntax Tree (AST) Generation
After tokenization, the interpreter generates an Abstract Syntax Tree (AST), a hierarchical structure representing the logical flow of the code.
Step 3: Bytecode Compilation
The AST is then converted into bytecode, which is a low-level set of instructions understood by the Python Virtual Machine (PVM). This bytecode is stored in .pyc
files inside the __pycache__
directory to speed up execution in future runs.
Step 4: Execution by Python Virtual Machine (PVM)
The bytecode is fed into the Python Virtual Machine (PVM), which interprets and executes the instructions. The PVM is responsible for memory management, function calls, and running the program.
2. Memory Management in Python
Python manages memory dynamically using a combination of reference counting and garbage collection.
Reference Counting
Every object in Python has a reference count, which tracks the number of times it is being used. When the reference count drops to zero, the memory occupied by the object is freed.
Example:
import sys
x = [1, 2, 3]
print(sys.getrefcount(x)) # Output: 2 (because sys.getrefcount adds an extra reference)
Garbage Collection
Python also has a garbage collector that handles cyclic references (when objects reference each other and cannot be automatically freed). The garbage collector runs periodically to detect and clean up unreferenced objects using generational garbage collection.
import gc
print(gc.get_count()) # Shows the current state of garbage collection
3. The Global Interpreter Lock (GIL)
One of the most debated aspects of Python's design is the Global Interpreter Lock (GIL). The GIL is a mutex that allows only one thread to execute Python bytecode at a time.
**Why Does Python Have a GIn
Python’s memory management (reference counting) is not thread-safe.
The GIL simplifies memory management and avoids race conditions.
Implications of the GIL
Single-threaded performance is good, but multi-threaded programs do not achieve true parallelism in CPU-bound tasks.
I/O-bound tasks (like file operations, network requests) can still benefit from multithreading.
To take advantage of multiple CPU cores, Python developers often use multiprocessing instead of threading:
from multiprocessing import Process
def worker():
print("Worker process")
if __name__ == "__main__":
p = Process(target=worker)
p.start()
p.join()
4. Python’s Just-In-Time (JIT) Compilation (PyPy)
While CPython is the standard implementation, PyPy is an alternative implementation that includes a Just-In-Time (JIT) compiler. JIT compilation translates bytecode into machine code at runtime, significantly improving execution speed.
Example:
pypy script.py # Running a Python script using PyPy instead of CPython
Conclusion
Python's internals, such as its interpreter, memory management, and the GIL, determine how Python runs code efficiently while staying user-friendly. Knowing these details helps developers improve performance, select the right tools, and write better Python programs. Whether using CPython, PyPy, or exploring concurrency models, understanding Python's inner workings is essential for advanced development.
Official Python documentation: https://docs.python.org/3/.
Subscribe to my newsletter
Read articles from Shoaib Hayat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shoaib Hayat
Shoaib Hayat
As a Software Engineer specializing in Python programming at Digital Code, I am at the forefront of digital transformation, AI, and decentralized technologies, developing and deploying next-generation solutions that redefine industries and disrupt traditional systems. With a strong foundation in Python, Machine Learning (ML), Artificial Intelligence (AI), Blockchain, and Web3, I build scalable, secure, and high-performance applications that solve complex real-world challenges. Whether it's engineering AI-driven models, creating smart contract solutions, or architecting decentralized platforms, my mission is to deliver cutting-edge innovation that drives business success and creates a lasting impact. ✔ 5+ Years of Experience: Proven expertise in software development, blockchain, and Web3, having contributed to high-impact projects and publications in these domains. ✔ AI-Powered Innovation: Developing intelligent, automated, and data-driven solutions that enhance decision-making, efficiency, and user experience. ✔ Blockchain & Web3 Specialist: Designing decentralized applications (DApps), CEX, DEX, smart contracts, and secure digital ecosystems for the future of the internet. ✔ Tech-Driven Problem Solver: Passionate about creating scalable, future-proof solutions that not only solve critical industry problems but also unlock new opportunities for businesses. ✔ Proactive, Collaborative, and Visionary: A strong advocate for excellence, diversity, and continuous learning, always seeking new challenges and groundbreaking opportunities. Beyond the code, I am a proactive thought leader, a collaborative innovator, and a relentless problem-solver who thrives on building the technologies of tomorrow. My passion lies in leveraging AI, blockchain, and decentralized computing to redefine possibilities and create transformative business solutions. Let’s connect and build the future together! If you’re looking for groundbreaking AI, blockchain, or Web3-driven innovation, I’m always open to collaborations, discussions, and strategic partnerships.