Day 20: JavaScript LocalStorage, SessionStorage & Cookiesโ-โManaging Data in the Browserย ๐ช

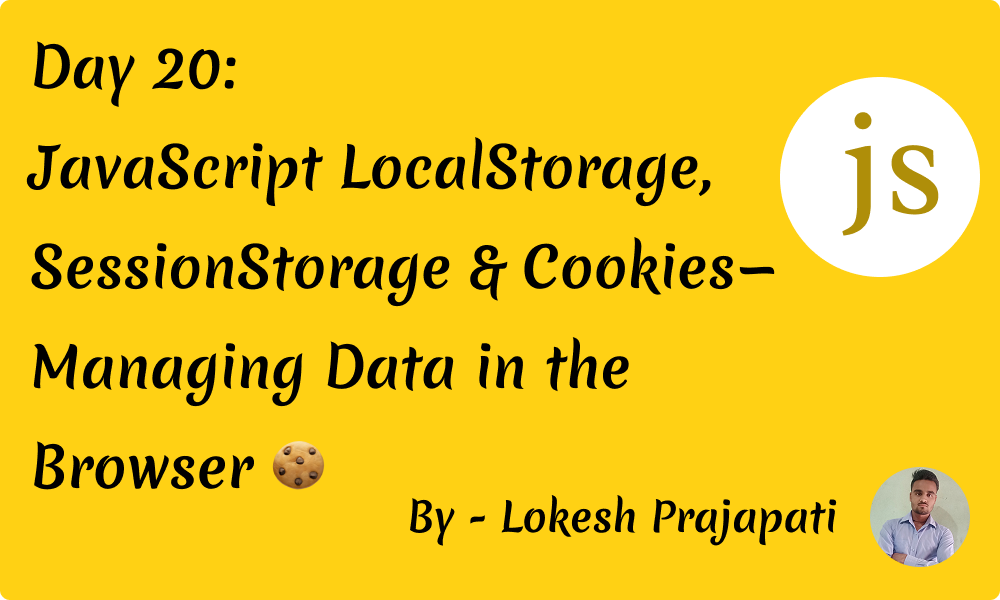
Managing data in the browser efficiently is essential for creating a smooth user experience. JavaScript provides three primary ways to store data in the browser: LocalStorage, SessionStorage, and Cookies. In this guide, weโll explore their differences, use cases, and best practices with real-life examples.
1. LocalStorage ๐๏ธ
What is it?
LocalStorage allows storing data in the browser with no expiration time.
Data persists even after the browser is closed and reopened.
Stores up to 5MB of data per origin.
Accessible only within the same origin.
โ When to Use LocalStorage?
Storing user preferences (e.g., theme selection, language settings).
Caching lightweight data that doesnโt need frequent updates.
Saving form inputs or drafts for later use.
๐ Example:
// Save data to LocalStorage
localStorage.setItem("username", "JohnDoe");
// Retrieve data
const user = localStorage.getItem("username");
console.log(user); // Output: "JohnDoe"
// Remove an item
localStorage.removeItem("username");
// Clear all storage
localStorage.clear();
2. SessionStorage ๐
What is it?
SessionStorage is similar to LocalStorage, but its data is removed when the browser session ends.
Stores up to 5MB of data per origin.
Accessible only within the same origin.
โ When to Use SessionStorage?
Storing temporary user session data.
Preserving form inputs during navigation.
Caching API responses for a single session.
๐ Example:
// Save data to SessionStorage
sessionStorage.setItem("authToken", "123abc");
// Retrieve data
const token = sessionStorage.getItem("authToken");
console.log(token); // Output: "123abc"
// Remove an item
sessionStorage.removeItem("authToken");
// Clear all session storage
sessionStorage.clear();
3. Cookies ๐ช
What is it?
Cookies store small pieces of data (max 4KB) that can be sent with every HTTP request.
Can have an expiration date or be deleted when the browser is closed.
Often used for authentication and tracking.
โ When to Use Cookies?
Storing authentication tokens (e.g., session IDs).
Tracking user activity (e.g., analytics, preferences).
Handling login sessions across different tabs.
๐ Example:
// Set a cookie with JavaScript
document.cookie = "user=JohnDoe; expires=Fri, 31 Dec 2025 23:59:59 GMT; path=/";
// Read cookies
console.log(document.cookie); // Output: "user=JohnDoe"
// Delete a cookie by setting an expired date
document.cookie = "user=; expires=Thu, 01 Jan 1970 00:00:00 GMT; path=/";
4. Key Differences ๐ง
5. Best Practices โ
๐น Use LocalStorage for non-sensitive, long-term storage.
๐น Use SessionStorage for temporary session data.
๐น Use Cookies only for authentication and tracking (consider Secure & HttpOnly flags).
๐น Avoid storing sensitive information like passwords in any client-side storage.
Conclusion ๐ฏ
Choosing the right storage mechanism in JavaScript depends on your use case. LocalStorage and SessionStorage are great for storing non-sensitive data, while Cookies are essential for authentication. Understanding these differences ensures better security and performance in your applications. ๐
What do you prefer for managing browser data? Share your thoughts in the comments! ๐
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
๐ JavaScript | React | Shopify Developer | Tech Blogger Hi, Iโm Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. Iโm currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Letโs learn and grow together. ๐ก๐ #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode