Building Smart Multi-Agent Apps with the OpenAI Agents Python SDK
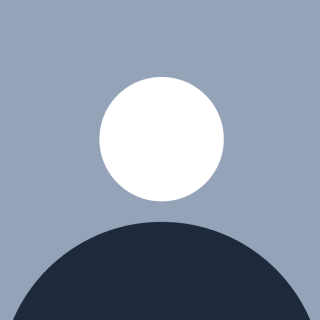
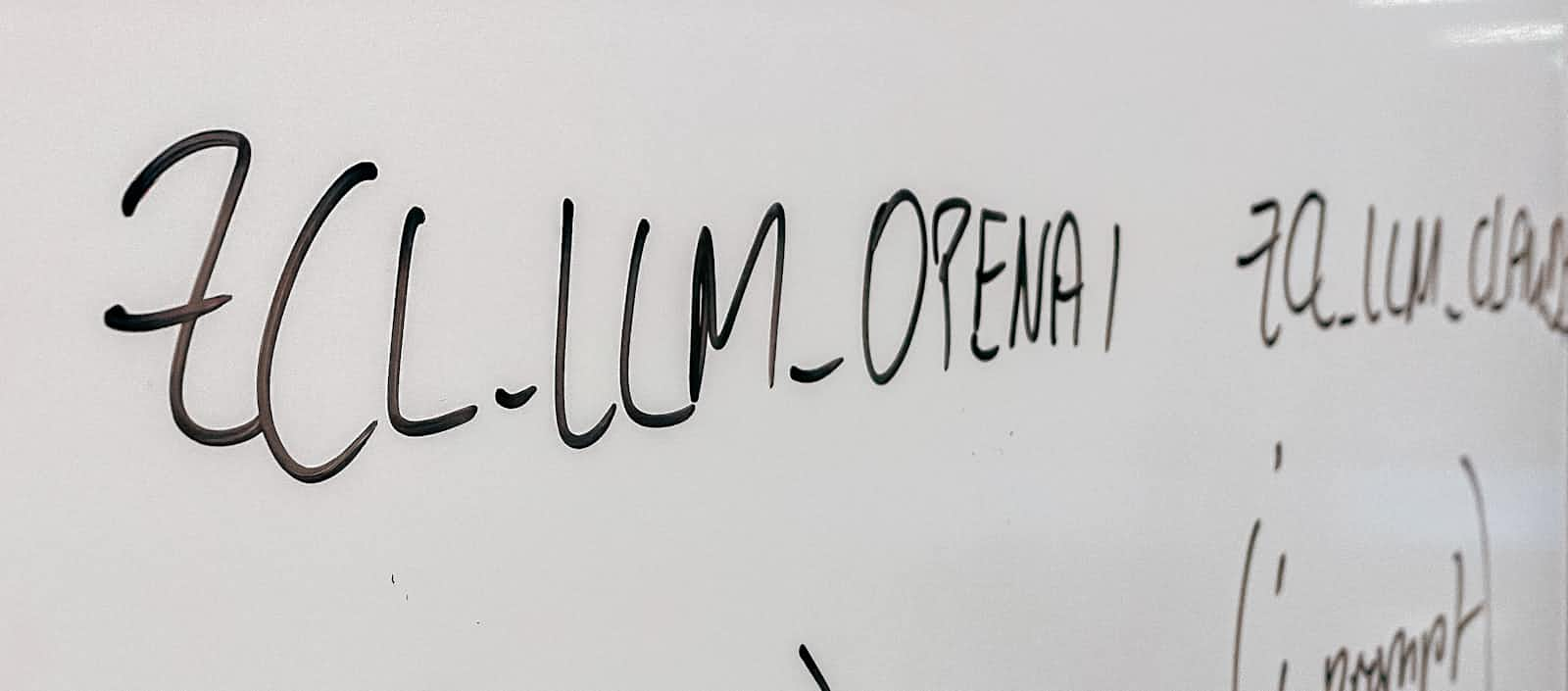
xxArtificial Intelligence (AI) is advancing rapidly, and one of the coolest developments is the idea of multi-agent systems—where multiple AI agents work together to solve complex tasks. Imagine having a team of AI assistants, each good at different things, working together to help you!
The OpenAI Agents Python SDK makes building these kinds of systems super easy. This post will show you how to use it to create smart applications with multiple agents that can work together like a team of experts.
📌 What Is the OpenAI Agents Python SDK?
The OpenAI Agents SDK is a tool that helps you create and control multiple AI agents using Python. Each agent has its own job and can even pass tasks to other agents if needed. It’s perfect for building things like customer support systems, research assistants, or even AI-powered shopping guides.
💻 Installation and Setup
Before we can use the SDK, we need to install it. To do this, open your terminal or command prompt and type:
pip install openai-agents
Next, we need to set up our API key, which is like a password that lets you use OpenAI’s services. You can do this by typing:
export OPENAI_API_KEY=sk-...
(Replace sk-...
with your actual API key).
🔍 Understanding the Basics
The SDK has three main parts:
Agents:
Think of an agent like a smart chatbot.
Each agent can follow instructions and use tools to complete tasks.
Handoffs:
If one agent can’t handle something, it can pass the task to another agent that’s better suited for the job.
For example, if a customer support bot gets a shopping question, it can pass it to a shopping bot.
Guardrails & Tracing:
Guardrails make sure agents don’t mess up.
Tracing helps you see what the agents are doing so you can fix any mistakes.
🌟 Your First Agent: A Simple Haiku Generator
Let's create a basic agent that writes a poem (haiku) about recursion in programming.
from agents import Agent, Runner
# Create an agent with instructions
agent = Agent(
name="Assistant",
instructions="You are a helpful assistant. Write a haiku about recursion in programming."
)
# Run the agent and print the output
result = Runner.run_sync(agent, "Start")
print(result.final_output)
✅ What This Code Does:
We create an agent called Assistant and tell it to write a haiku.
Then, we run it and print the result.
The output might look something like:
Code within the code,
Functions calling themselves,
Infinite loop’s dance.
🔗 Making Agents Work Together (Handoffs)
Sometimes, one agent can’t handle everything. That’s where handoffs come in. Let’s say we have:
A support agent that handles refunds.
A shopping agent that gives fashion advice.
A triage agent that decides who should handle the task.
from agents import Agent, Runner
# Define specialized agents
support_agent = Agent(
name="Support & Returns",
instructions="You are a support agent who can process refund requests.",
)
shopping_agent = Agent(
name="Shopping Assistant",
instructions="You are a shopping assistant who can help with product searches.",
)
# Define a triage agent that routes queries
triage_agent = Agent(
name="Triage Agent",
instructions=(
"Route the user to the correct agent: "
"If it's about refunds, send it to Support & Returns. "
"If it's about shopping, send it to Shopping Assistant."
),
handoffs=[shopping_agent, support_agent],
)
# Test the system with a shopping-related question
output = Runner.run_sync(triage_agent, "What shoes should I wear with a black dress?")
print(output.final_output)
✅ What This Code Does:
We have one agent to handle refunds and another to give shopping advice.
The triage agent figures out which agent should handle the question.
This way, your AI system is smart enough to pass tasks to the right helper!
🧩 Adding Special Tools to Agents
The SDK also lets you turn Python functions into tools that your agents can use. For example, let’s create a tool that tells the weather.
from agents import function_tool, Agent, Runner
@function_tool
def get_weather(city: str) -> str:
return f"The weather in {city} is sunny."
agent = Agent(
name="Weather Agent",
instructions="You are a helpful agent who provides weather updates.",
tools=[get_weather],
)
result = Runner.run_sync(agent, "What's the weather in Tokyo?")
print(result.final_output)
✅ What This Code Does:
The
get_weather
function becomes a tool for the agent.The agent can call this tool to give weather updates.
The output could be:
The weather in Tokyo is sunny.
🔍 Tracking What’s Going On (Tracing)
To see what your agents are doing, you can use tracing. This makes debugging way easier.
from agents import trace, Runner
with trace(workflow_name="Debugging Agent Flow"):
result = Runner.run_sync(agent, "Check the weather in New York.")
print(result.final_output)
✅ What This Code Does:
It keeps a record of how the agent processes the task.
You can use this info to spot errors and make improvements.
💡 Real-Life Applications
The OpenAI Agents SDK can be used for many things:
Customer Support Bots: Handling refunds, product questions, and troubleshooting.
AI Research Assistants: Searching the internet, analyzing data, and creating reports.
Financial Advisors: Helping users with banking, loans, and investment advice.
📌 Conclusion
The OpenAI Agents Python SDK makes it super easy to build AI systems where multiple agents work together like a team. By using handoffs, tools, and tracing, you can create smart applications that handle all sorts of tasks.
So go ahead, try building your own AI team! It’s easier than you think. 😄
Subscribe to my newsletter
Read articles from Aryan Chaurasia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by