π Build a Modern PDF Protector Tool Using Python and CustomTkinter (Step-by-Step Guide)
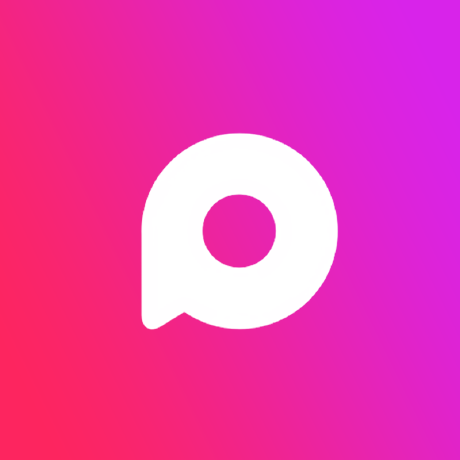
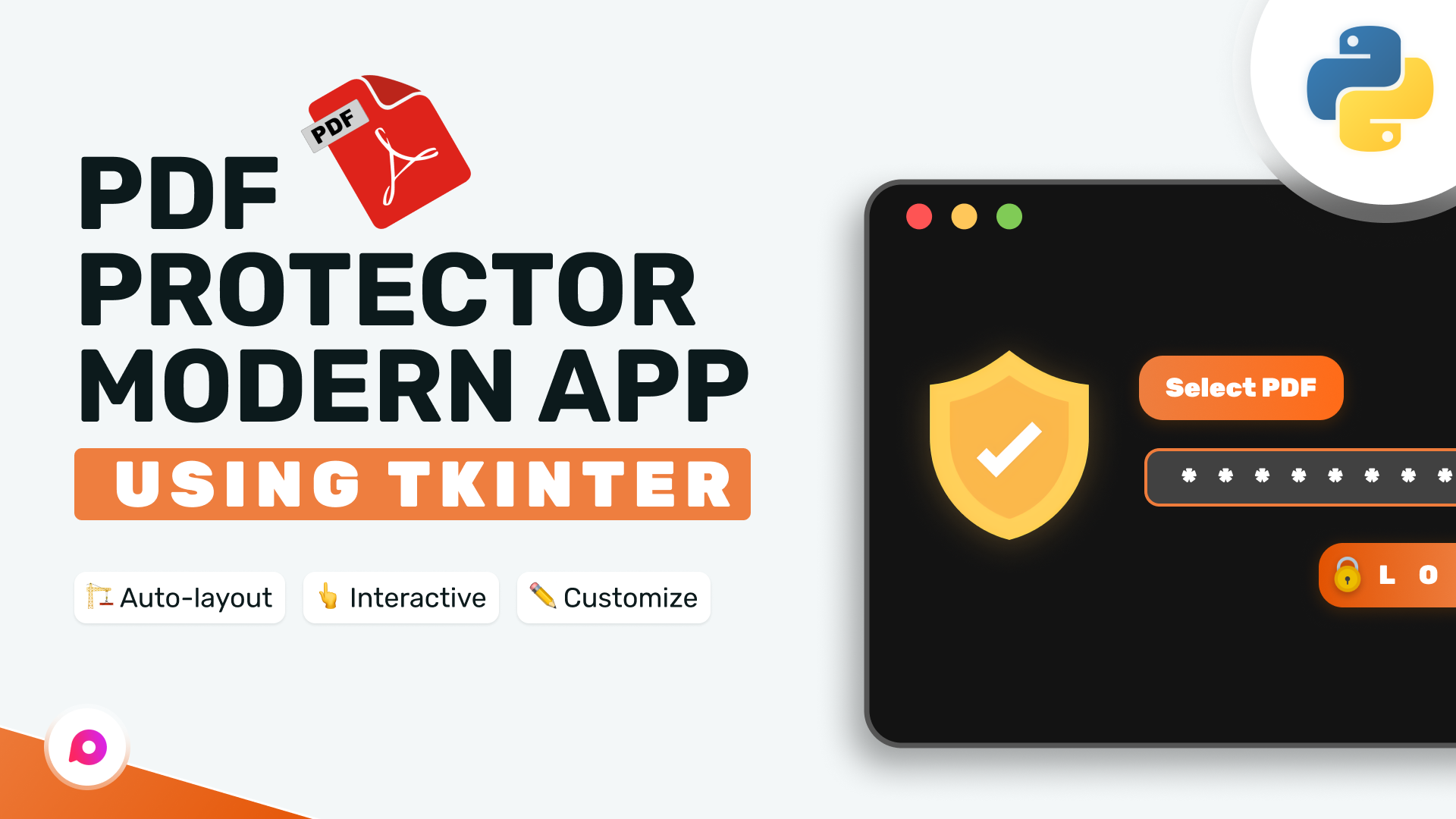
Protecting sensitive PDF documents is essential in todayβs digital world β whether it's for reports, contracts, or personal files. In this tutorial, you'll learn how to build a modern PDF password protector tool using Python with a sleek GUI made using CustomTkinter.
This tool allows users to select any PDF file, enter a password, and encrypt the file within seconds β all through a modern-looking interface that feels professional and easy to use.
π§ Why Build a PDF Protector Tool?
β Protect sensitive information with ease
β Learn GUI development with CustomTkinter
β Understand how PDF encryption works in Python
β Improve your Python skills by building a real-world project
π οΈ Tools & Libraries Youβll Use
Python β Core programming language
PyPDF2 β To encrypt the PDF files
CustomTkinter β To create a modern and beautiful GUI
Tkinter FileDialog β To select files from the system
π§ͺ How It Works
User selects a PDF file
User enters a password
Tool encrypts the PDF using PyPDF2
Encrypted file is saved with
_protected
added to the filename
π» Full Python Code with CustomTkinter GUI
pythonCopyEditimport customtkinter as ctk
from tkinter import filedialog, messagebox
from PyPDF2 import PdfReader, PdfWriter
import os
ctk.set_appearance_mode("Dark")
ctk.set_default_color_theme("blue")
app = ctk.CTk()
app.geometry("500x350")
app.title("PDF Protector Tool")
def protect_pdf():
file_path = filedialog.askopenfilename(filetypes=[("PDF Files", "*.pdf")])
if not file_path:
return
password = password_entry.get()
if not password:
messagebox.showerror("Error", "Please enter a password.")
return
try:
reader = PdfReader(file_path)
writer = PdfWriter()
for page in reader.pages:
writer.add_page(page)
writer.encrypt(password)
filename = os.path.basename(file_path).replace(".pdf", "_protected.pdf")
output_path = os.path.join(os.path.dirname(file_path), filename)
with open(output_path, "wb") as f:
writer.write(f)
messagebox.showinfo("Success", f"PDF Protected!\nSaved as: {filename}")
except Exception as e:
messagebox.showerror("Error", str(e))
# UI Elements
title_label = ctk.CTkLabel(app, text="PDF Password Protector", font=ctk.CTkFont(size=20, weight="bold"))
title_label.pack(pady=20)
password_entry = ctk.CTkEntry(app, placeholder_text="Enter password", show="*")
password_entry.pack(pady=10, ipadx=40, ipady=5)
protect_btn = ctk.CTkButton(app, text="Select PDF and Protect", command=protect_pdf)
protect_btn.pack(pady=20)
footer_label = ctk.CTkLabel(app, text="Created with β€οΈ using Python & CustomTkinter", font=ctk.CTkFont(size=12))
footer_label.pack(side="bottom", pady=10)
app.mainloop()
π¨ Why CustomTkinter?
While Tkinter is a default GUI toolkit in Python, CustomTkinter allows for modern, flat design interfaces, dark mode support, and a much better user experience.
Perfect for 2025 and beyond.
π SEO Keywords You Can Target
PDF password protection in Python
How to encrypt PDF using Python
Build GUI app using CustomTkinter
Modern Python projects for beginners
PyPDF2 tutorial for PDF encryption
πΊ Watch the Full Tutorial
Want the full breakdown with code walkthrough and design explanation? Watch the video on YouTube:
π [Insert your video link here]
π What's Next?
Now that you've built a fully working PDF protection tool, try adding:
Decryption functionality
Drag-and-drop support
Save to custom folder option
Add logging or status bar
π§΅ Final Thoughts
Learning how to build real-world tools like a PDF password protector is a great way to solidify your Python skills. Combining functional coding with beautiful UI design gives your projects that professional touch.
So what are you waiting for? Try this project, and don't forget to share your version online and tag me!
Subscribe to my newsletter
Read articles from Proxlight directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
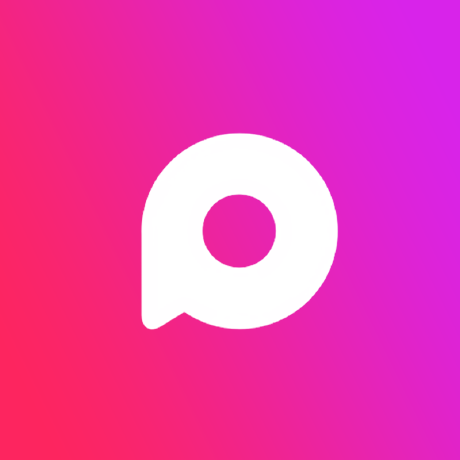
Proxlight
Proxlight
Do you need help with programming? You are not the only one. In this channel, we tackle gradual programming topics and end the videos on cool projects you can work on for practice to improve your skills on that given topic, step by step. Join us as we use fun and practical projects to grow our programming skills.ππ».