An in-depth look at dynamic routing with Next.js

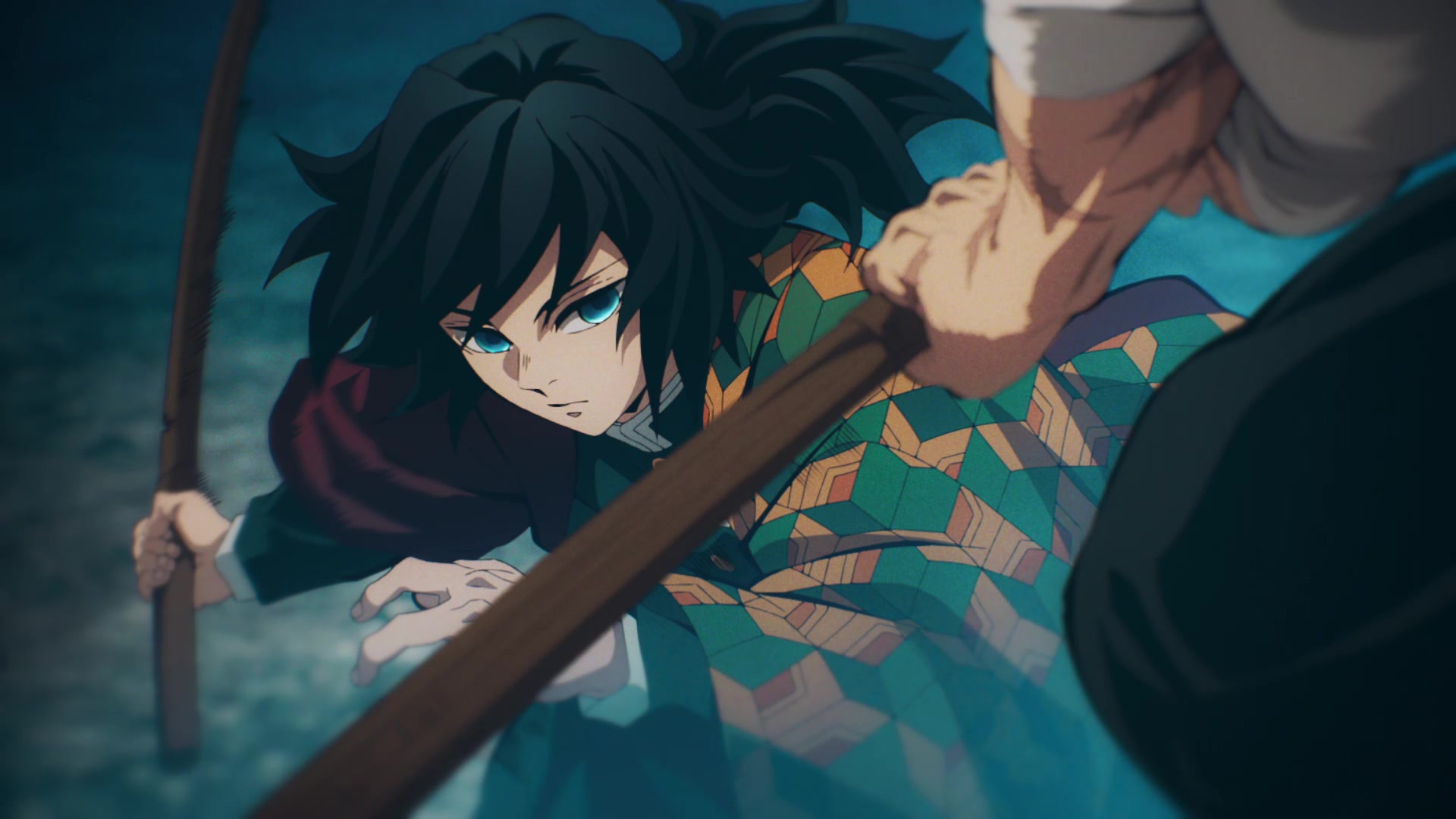
[43]
Routing in React App
To implement routing within a React application, developers typically rely on a third-party package, such as React Router, which provides the necessary tools for managing navigation. The core of the routing configuration resides in a dedicated routes.js
file. Within this file, individual routes are defined, each mapping a specific URL path to a corresponding React component. For every unique route, a separate component file is created, encapsulating the content and functionality associated with that particular view.
This component is then exported, making it accessible to other parts of the application. In routes.js
, these exported components are imported, and a new route object is constructed. This object includes a path
property, which specifies the URL pattern that should trigger the rendering of the associated component, effectively establishing the connection between the URL and the user interface.
Routing in Next App
Next.js employs a file-system based routing mechanism, simplifying route creation. When a new file is added to the pages
folder within a Next.js project, that file automatically becomes accessible as a route. By strategically combining file names with a nested folder structure, developers can effectively define and implement the majority of common routing patterns.
Dynamic Routes
product/index.js
function ProductList() {
return (
<>
<h2>Product 1</h2>
<h2>Product 2</h2>
<h2>Product 3</h2>
</>
)
}
export default ProductList
product/[productId].js
import { userRouter } from 'next/router'
function ProductDetail() {
const router = useRouter()
// The hook returns a router object from which we access the query parameter object
const productId = router.query.productId
// 'productId' on the query object corresponds to the dynamic segment specified with the file name
return <h1>Details about the product {productId}</h1>
}
export default ProductDetail
Output: Note: Here, productId
can be any string and not just a number.
Next.js treats '[ ]' in a file name as a dynamic segment to create a dynamic route.
Nested Dynamic Routing
How to navigate from localhost:3000/product/1
to localhost:3000/product/1/review/1
?
product/[productId]/index.js
import { useRouter } from 'next/router'
function ProductDetail() {
const router = useRouter()
const productId = router.query.productId
return <h1> Details about product {productId} </h1>
}
export default ProductDetail
```
`product/[productId]/review/[reviewId].js`
```js
import { useRouter } from 'next/router'
function Review() {
const router = useRouter()
const { productId, reviewId } = router.query
return <h1>Review {reviewId} for product {productId}</h1>
}
export default Review
Catch All Routes
docs/[...params].js
import { useRouter } from 'next/router'
function Doc() {
const router = useRouter()
const { params = [] } = router.query
console.log(params)
if(params === 2) {
return (
<h1> Viewing docs for feature {params[0]} and concept {params[1]} </h1>
)
} else if (params.length === 1) {
return <h1>Viewing docs for feature {params[0]}</h1>
}
return <h1>Docs Home Page</h1>
}
export default Doc
This page will match any URL that contains the docs segment in the path.
Link Component Navigation
It is used for client-side routing within your application. Something to keep in mind is that you shouldn't be using plain HTML anchor tag for client-side routing as it would make a new server request and any client-side request you're maintaining will be erased.
import Link from 'next/link'
function Home() {
return (
<div>
<h1>Home Page</h1>
<Link href="/blog">
<a>Blog</a>
</Link>
<Link href="/"> //Represents the root of our application
<a>Home</a>
</Link>
</div>
)
}
export default Home
By passing the productId
as a prop to the function.
import Link from 'next/link'
function ProductList( { productId = 100 }) {
return (
<>
<Link href="/product/3" replace>
<a>Product 1</a>
</Link>
<Link href={`/product/${productId}`}>
<a>Product {productId}</a>
</Link>
</>
)
}
Here, the replace
prop will replace the current history state instead of adding a new URL stack.
Subscribe to my newsletter
Read articles from Pranav Bawgikar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranav Bawgikar
Pranav Bawgikar
Hiya ๐ I'm Pranav. I'm a recent computer science grad who loves punching keys, napping while coding and lifting weights. This space is a collection of my journey of active learning from blogs, books and papers.