Week 2 : How Robots Remember – Storing Data with Variables! 🚀🤖

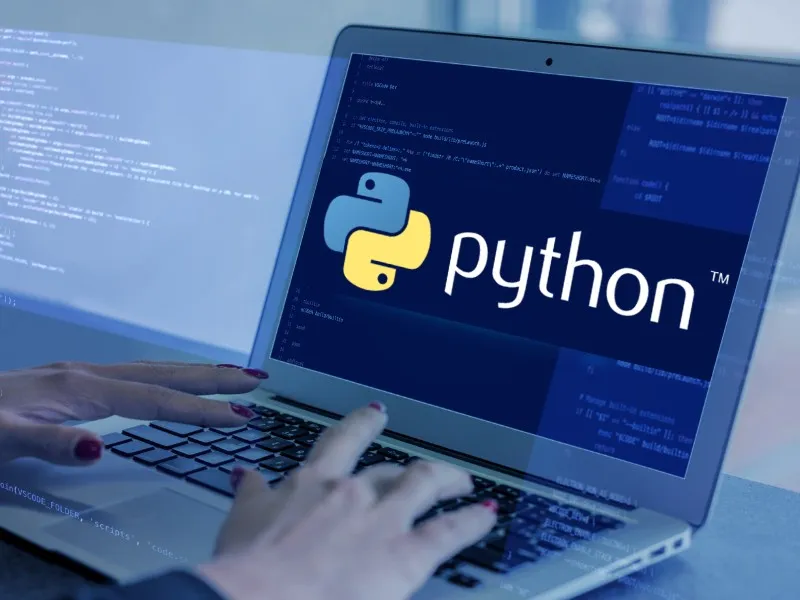
Welcome, Young Coders!
Last time, we learned how to talk to our robot friends using Python and made them say "Hello, World!". Now, imagine if our robot could remember things like its name, age, and favorite color! That’s where variables come in. Today, we’ll explore how to store information using variables and data types in Python.
🔍 What Are Variables?
Think of a variable as a special container where we can store information. It’s like a backpack where you keep your school supplies. When you need something, you take it out!
🎒 Real-Life Example:
Imagine you have a backpack labeled "Lunch". Inside, you put your favorite snack. When it’s time to eat, you open it up and take out your snack!
In Python, we store information the same way:
snack = "Apple"
print("I have a", snack, "in my backpack!")
Output:
I have a Apple in my backpack!
Cool, right? Now let’s explore what kind of things we can store!
🧮 Different Types of Data in Python
Python has different kinds of data, just like your backpack can hold books, pencils, and snacks!
1️⃣ Numbers (Integers & Floats)
Numbers help computers count things. There are two main types:
✅ Integers (int
) → Whole numbers like 5, 10, 42
✅ Floats (float
) → Decimal numbers like 3.14, 2.5, 99.99
📌 Example:
age = 10 # Integer
height = 4.5 # Float
print("I am", age, "years old and", height, "feet tall.")
💡 Try It! Change the numbers and see what happens!
2️⃣ Text (Strings - str
)
A string is just text inside quotes (" " or ' '). We use it when we want Python to remember words, names, or even sentences!
📌 Example:
robot_name = "Zeno"
print("My robot's name is", robot_name)
🤖 Try It! Change "Zeno"
to your own robot’s name!
3️⃣ True or False (Booleans - bool
)
Computers love YES or NO answers. That’s why we have Booleans:
✅ True
→ Yes
❌ False
→ No
📌 Example:
robot_can_fly = False
print("Can my robot fly?", robot_can_fly)
🔍 Recap: What Did We Learn?
✔ Variables store information in Python (like labeled boxes!)
✔ Data types help us store different kinds of information:
Numbers (
int
,float
) →10
,3.14
Strings (
str
) →"Hello!"
True/False (
bool
) →True
,False
✔ We can use print() to display our variables!
💡 Discussion: Think About This!
Imagine you are designing a super robot 🦾.
❓ What information would you store inside your robot?
💬 Share your thoughts in the comments!
📌 Next Week: We’ll learn how to interact with users by taking input! 🎤
🚀 What’s Next?
✅ Practice: Try building your own robot in Python!
✅ Homework: Answer the quiz and share your Google Colab link with me!
✅ Have Fun!
See you next class, Super Coders! 🔥💻
Subscribe to my newsletter
Read articles from Ridwan Ibidunni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ridwan Ibidunni
Ridwan Ibidunni
I'm a mathematician that loves its applications in all spheres of life, especially in the field of machine learning. I write Java, Python and Android applications