Spring AI Fundamentals
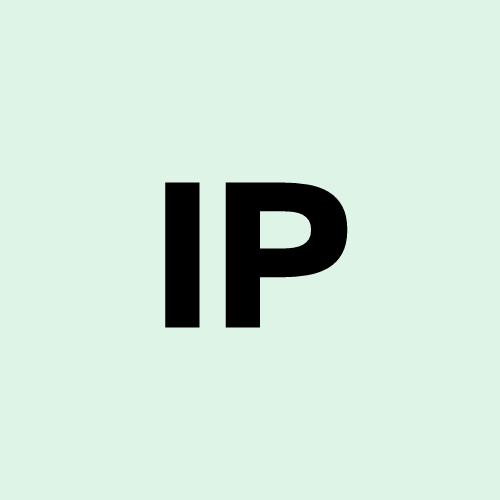
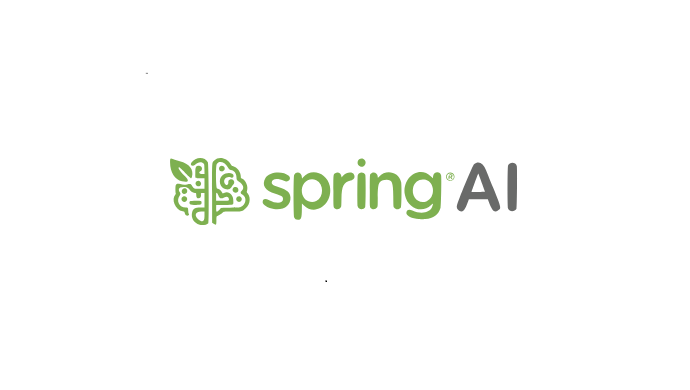
Spring AI is an emerging framework that brings Generative AI capabilities to applications developed with Spring Boot. By offering a standardized client abstraction, Spring AI enables developers to integrate various AI service providers and large language models (LLMs) into their applications with ease.
What is Spring AI?
Spring AI provides a consistent interface to interact with different AI service providers, making it easier to integrate AI-driven functionalities without being locked into a specific provider. Whether you choose OpenAI, Azure OpenAI, MistralAI, Google Vertex AI, or Amazon Bedrock, Spring AI abstracts the underlying complexities, providing a seamless development experience. Additionally, Spring AI supports locally-run LLMs through Ollama, allowing for on-premises AI inference.
Key Features of Spring AI
Unified Client Abstraction: A consistent API to interact with multiple AI providers.
Easy Integration: Quickly integrate AI-powered functionalities into Spring Boot applications.
Support for Multiple AI Providers: OpenAI, Azure OpenAI, Google Vertex AI, MistralAI, and Amazon Bedrock.
Local AI Models Support: Run models locally using Ollama for enhanced privacy and control.
Extensibility: Allows developers to customize and extend functionality based on specific use cases.
Building a Simple Spring AI Application
In this example, we have built a basic Spring AI application that sends a textual prompt to an LLM and prints the response.
Dependencies
To use Spring AI in a Spring Boot Kotlin application, add the required dependencies to your build.gradle.kts
:
dependencies {
implementation("org.springframework.boot:spring-boot-starter")
implementation("org.springframework.ai:spring-ai-core:0.6.0")
implementation("org.springframework.ai:spring-ai-openai:0.6.0")
}
Configuration
Configure your OpenAI API key in application.properties
:
spring.ai.openai.api-key=openai_api_key
Implementation
Create a simple Spring Boot Kotlin service to interact with the LLM:
import org.springframework.ai.openai.OpenAiClient
import org.springframework.stereotype.Service
@Service
class AIService(private val openAiClient: OpenAiClient) {
fun getAIResponse(prompt: String): String {
return openAiClient.getChatResponse(prompt)
}
}
Controller
Expose an endpoint to send prompts to the AI model:
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RequestParam
import org.springframework.web.bind.annotation.RestController
@RestController
class AIController(private val aiService: AIService) {
@PostMapping("/ask")
fun askAI(@RequestParam prompt: String): String {
return aiService.getAIResponse(prompt)
}
}
Running the Application
Start your Spring Boot Kotlin application and test it by sending a POST request:
curl localhost:8080/ask \
-H"Content-type: application/json" \
-d'{"question":"Why is the sky blue?"}'
{"answer":"The sky appears blue because of the way the Earth's atmosphere
scatters sunlight. When sunlight travels through the atmosphere, it collides
with molecules and particles in the air. These collisions cause the sunlight
to scatter in all directions. Blue light has a shorter wavelength and scatters
more easily than other colors, which is why we see the sky as blue during
the day."}
This should return a response generated by the AI model.
Exploring More
For a practical implementation of a Spring AI-based project, you can check out this GitHub repository: Board Game Buddy - GitHub
Conclusion
Spring AI simplifies the integration of Generative AI capabilities into Spring Boot applications by providing a unified client abstraction, supporting multiple AI providers, and enabling local AI execution. Whether you're working with cloud-based AI models or locally hosted LLMs, Spring AI offers flexibility and ease of use for developers building AI-powered applications.
Subscribe to my newsletter
Read articles from Ilkay Polat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
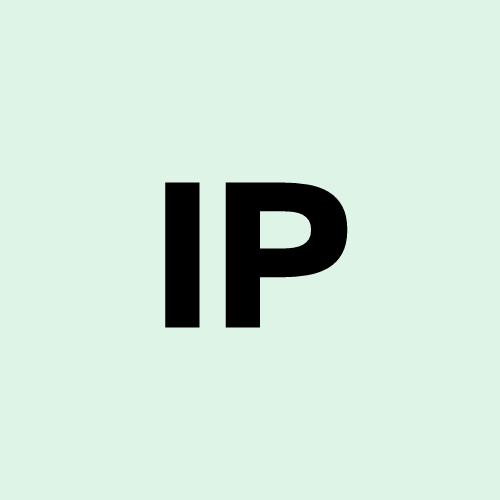