Rest and ...spread operator in JS

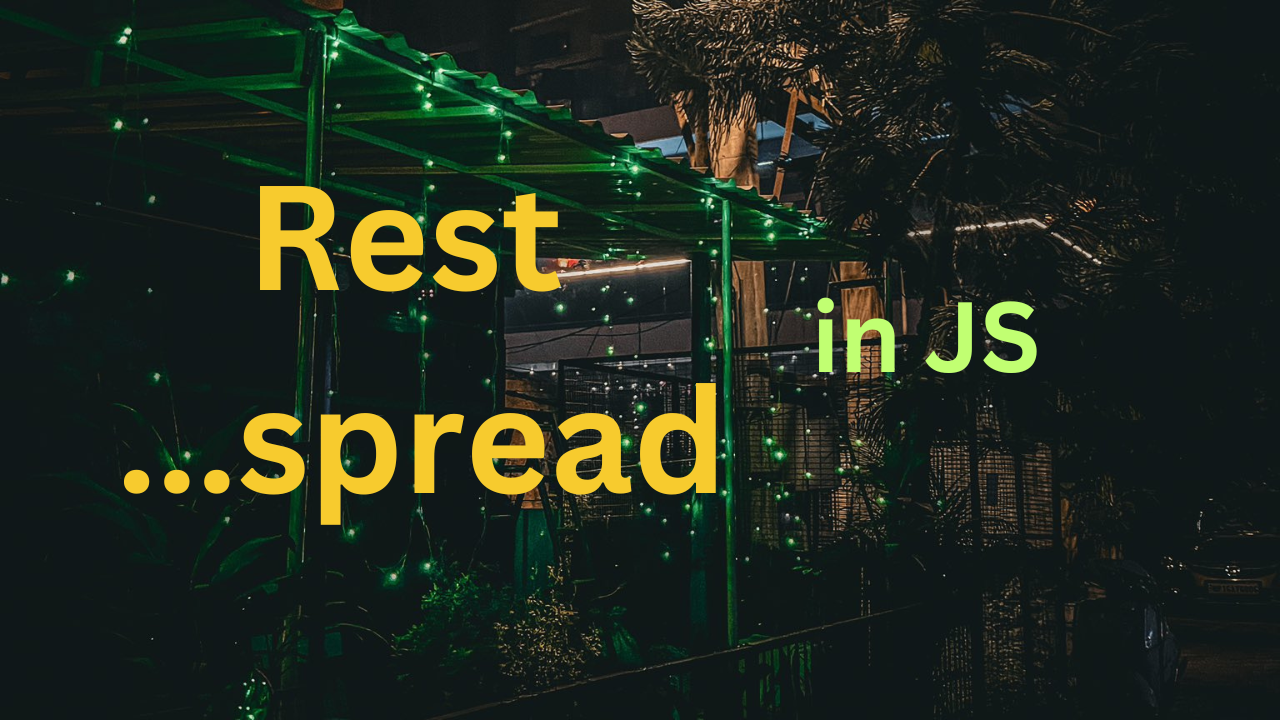
Namaste! 😁, this is Aryan Sharma's blog, and I hope you're all doing well & great.
📍You can find my blogs here↗️
Let's get started!
Rest and spread are one of the Javascript built-in methods
In this blog, we will learn to pass arrays to such functions as parameters.
Rest operator
Imagine we have an array having 5 items/elements in it.
but we need to find the sum of only the first two elements.
A function can be called with any number of arguments, and there will be no error if most arguments are unused.
function sum(a, b) {
return a + b;
}
alert( sum(1, 2, 3, 4, 5) );
The rest of the parameters can be included in the function definition by using three dots ...
followed by the name of the array containing them.
The dots mean “gather the remaining parameters into an array”.
function sumAll(...args) {
let sum = 0;
for (let x of args) sum += x;
return sum;
}
alert( sumAll(1) );
alert( sumAll(1, 2) );
alert( sumAll(1, 2, 3) );
We can get the first parameters as variables and gather only the rest.
const {a, ...rest, b} = {a: 1, b: 2, c: 3, d: 4};
//error
console.log(a);
console.log(b);
console.log(rest);
The
...rest
must always be last.
arguments variable
Arguments are variables that we provide to a function.
Most people find it difficult to learn the difference between argument
and parameters
In the past, the only method to obtain all of the function's arguments was to use arguments because.
the remainder of the parameters were not included in the language. It is still functional; the previous code included it.
However, arguments is not an array even though it is iterable and array-like. For instance, we are unable to call arguments. map(...) since it does not accept array functions.
And it contains all of the arguments. We can’t take them partially, like we can do with the rest of the parameters.
So, when we need these features, the rest of the parameters are preferred.
Now, we are moving to 2nd topic of this article.
Spread syntax
We’ve just got to know how to get an array from the list of parameters
sometimes we need to do exactly the reverse...
The spread operator (...
) in JavaScript is used to spread out elements of an iterable (like an array or object) into individual elements.
⏩ Imagine we are combining the 2 arrays
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combinedArray = [...arr1, ...arr2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
arr1
andarr2
are two arrays.Using the spread operator
...
, we create a new arraycombinedArray
that includes all elements fromarr1
andarr2
.This allows us to combine arrays without mutating the original arrays.
With Objects
const obj1 = { name: "Aryan", age: 25 };
const obj2 = { job: "Developer", city: "Mumbai" };
const combinedObject = { ...obj1, ...obj2 };
console.log(combinedObject);
// Output: { name: "Aryan", age: 25, job: "Developer", city: "Mumbai" }
Function Arguments Example
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
const result = sum(...numbers); // equivalent to sum(1, 2, 3)
console.log(result); // Output: 6
The
sum
the function takes three arguments.By using the spread operator, the
numbers
the array is expanded into individual arguments when passed to thesum
function.
See u in the next blog...Stay tuned🎶
Don't forget to connect with me on: LinkedIn
Subscribe to my newsletter
Read articles from aryan sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

aryan sharma
aryan sharma
Hey, Awesome ones! Aryan this side👋 Full-Stack Developer, Life-Long Learner, Optimistic Using this blog to help code newbies. Learn with me! :)