Behavioral Design Patterns

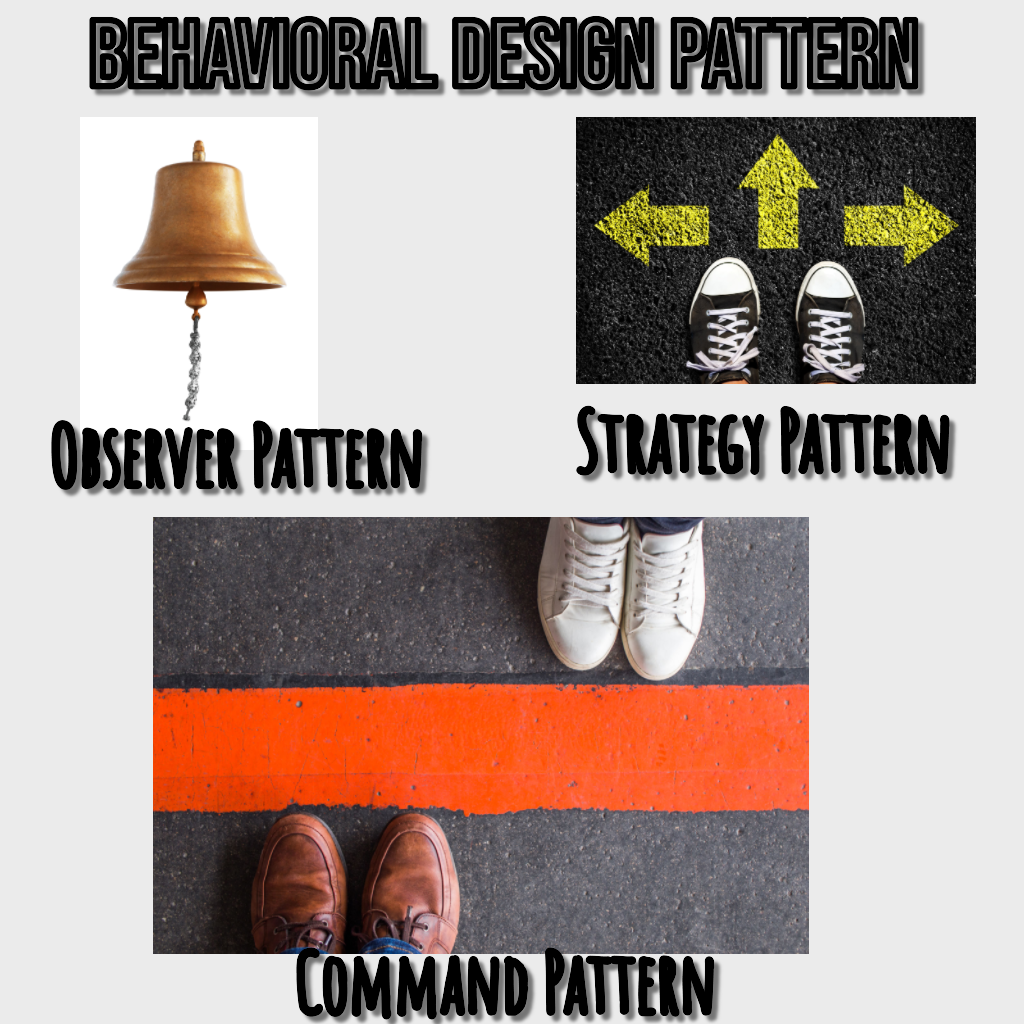
Now that your kitchen is set up (Creational Design Patterns) and well-organized (Structural Design Patterns), it's time to start cooking!
Behavioral Design Patterns define how objects communicate and interact within a system, just like how chefs, waiters, and kitchen staff coordinate to serve a perfect meal in a restaurant.
Letโs explore these patterns with real-world cooking analogies!
1. Observer Pattern โ Waiter & Customers ๐
A waiter takes orders from multiple tables. When the food is ready, the kitchen notifies the waiter, who then serves the customers. The waiter doesnโt check constantlyโthey just get notified when needed.
๐น In software: The Observer Pattern allows multiple objects (customers) to be notified automatically when the state of another object (kitchen) changes.
Java Example: Observer Pattern
import java.util.ArrayList;
import java.util.List;
// Step 1: Define an Observer interface
interface Observer {
void update(String orderStatus);
}
// Step 2: Create a Subject (Observable)
class Kitchen {
private List<Observer> waiters = new ArrayList<>();
private String orderStatus;
public void addObserver(Observer waiter) {
waiters.add(waiter);
}
public void notifyObservers() {
for (Observer waiter : waiters) {
waiter.update(orderStatus);
}
}
public void prepareOrder(String status) {
this.orderStatus = status;
notifyObservers(); // Notify all waiters
}
}
// Step 3: Implement Concrete Observers
class Waiter implements Observer {
private String name;
public Waiter(String name) {
this.name = name;
}
public void update(String orderStatus) {
System.out.println(name + " received update: Order is " + orderStatus);
}
}
// Step 4: Usage
public class ObserverPatternDemo {
public static void main(String[] args) {
Kitchen kitchen = new Kitchen();
Waiter waiter1 = new Waiter("Waiter A");
Waiter waiter2 = new Waiter("Waiter B");
kitchen.addObserver(waiter1);
kitchen.addObserver(waiter2);
kitchen.prepareOrder("Ready to Serve!");
// Output:
// Waiter A received update: Order is Ready to Serve!
// Waiter B received update: Order is Ready to Serve!
}
}
๐ Why use the Observer Pattern?
โ
Useful when multiple objects need real-time updates
โ
Avoids constant checking (polling)
โ
Commonly used in event-driven systems
2. Strategy Pattern โ Cooking Styles ๐ฅ
Imagine you're cooking chicken. You can fry, grill, or bake itโeach method is interchangeable based on preference.
๐น In software: The Strategy Pattern allows you to switch between different algorithms dynamically at runtime.
Java Example: Strategy Pattern
// Step 1: Define a Strategy Interface
interface CookingStrategy {
void cook();
}
// Step 2: Implement Concrete Strategies
class FryingStrategy implements CookingStrategy {
public void cook() {
System.out.println("Frying the chicken ๐ณ");
}
}
class GrillingStrategy implements CookingStrategy {
public void cook() {
System.out.println("Grilling the chicken ๐ฅ");
}
}
class BakingStrategy implements CookingStrategy {
public void cook() {
System.out.println("Baking the chicken ๐");
}
}
// Step 3: Create a Context Class
class Chef {
private CookingStrategy strategy;
public void setStrategy(CookingStrategy strategy) {
this.strategy = strategy;
}
public void cookDish() {
strategy.cook();
}
}
// Step 4: Usage
public class StrategyPatternDemo {
public static void main(String[] args) {
Chef chef = new Chef();
chef.setStrategy(new FryingStrategy());
chef.cookDish(); // Output: Frying the chicken ๐ณ
chef.setStrategy(new GrillingStrategy());
chef.cookDish(); // Output: Grilling the chicken ๐ฅ
}
}
๐ Why use the Strategy Pattern?
โ
Enables dynamic switching of behaviors
โ
Promotes the Open-Closed Principle (easy to add new strategies)
โ
Avoids long conditional statements
3. Command Pattern โ Restaurant Ordering System ๐
In a restaurant, a waiter takes orders, but the chef executes them. The waiter doesnโt care how the food is preparedโjust that the order gets fulfilled.
๐น In software: The Command Pattern separates request initiation (waiter) from execution (chef), making it easy to handle undo actions, logging, and queuing.
Java Example: Command Pattern
import java.util.ArrayList;
import java.util.List;
// Step 1: Define a Command Interface
interface Order {
void execute();
}
// Step 2: Create Concrete Commands
class BurgerOrder implements Order {
public void execute() {
System.out.println("Cooking a burger ๐");
}
}
class PizzaOrder implements Order {
public void execute() {
System.out.println("Preparing a pizza ๐");
}
}
// Step 3: Create an Invoker (Waiter)
class Waiter {
private List<Order> orders = new ArrayList<>();
public void takeOrder(Order order) {
orders.add(order);
}
public void serveOrders() {
for (Order order : orders) {
order.execute();
}
orders.clear();
}
}
// Step 4: Usage
public class CommandPatternDemo {
public static void main(String[] args) {
Waiter waiter = new Waiter();
waiter.takeOrder(new BurgerOrder());
waiter.takeOrder(new PizzaOrder());
waiter.serveOrders();
// Output:
// Cooking a burger ๐
// Preparing a pizza ๐
}
}
๐ Why use the Command Pattern?
โ
Decouples request and execution
โ
Supports undo functionality
โ
Ideal for task queuing and macros
Why Behavioral Patterns Matter?
Just as a well-managed restaurant ensures smooth operations, Behavioral Design Patterns create efficient and flexible communication between objects.
When to use Behavioral Design Patterns?
โ
When objects need to react to state changes (Observer Pattern)
โ
When you need interchangeable algorithms (Strategy Pattern)
โ
When requests and execution should be decoupled (Command Pattern)
Final Thoughts
Think of Behavioral Design Patterns as streamlining the cooking processโensuring that all elements of the system communicate effectively. By mastering these patterns, you can build scalable, efficient, and maintainable software.
Would you like to explore more patterns in depth? Drop your thoughts in the comments! ๐
Subscribe to my newsletter
Read articles from Rohith Reddy Seelam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
