Folding in Elixir

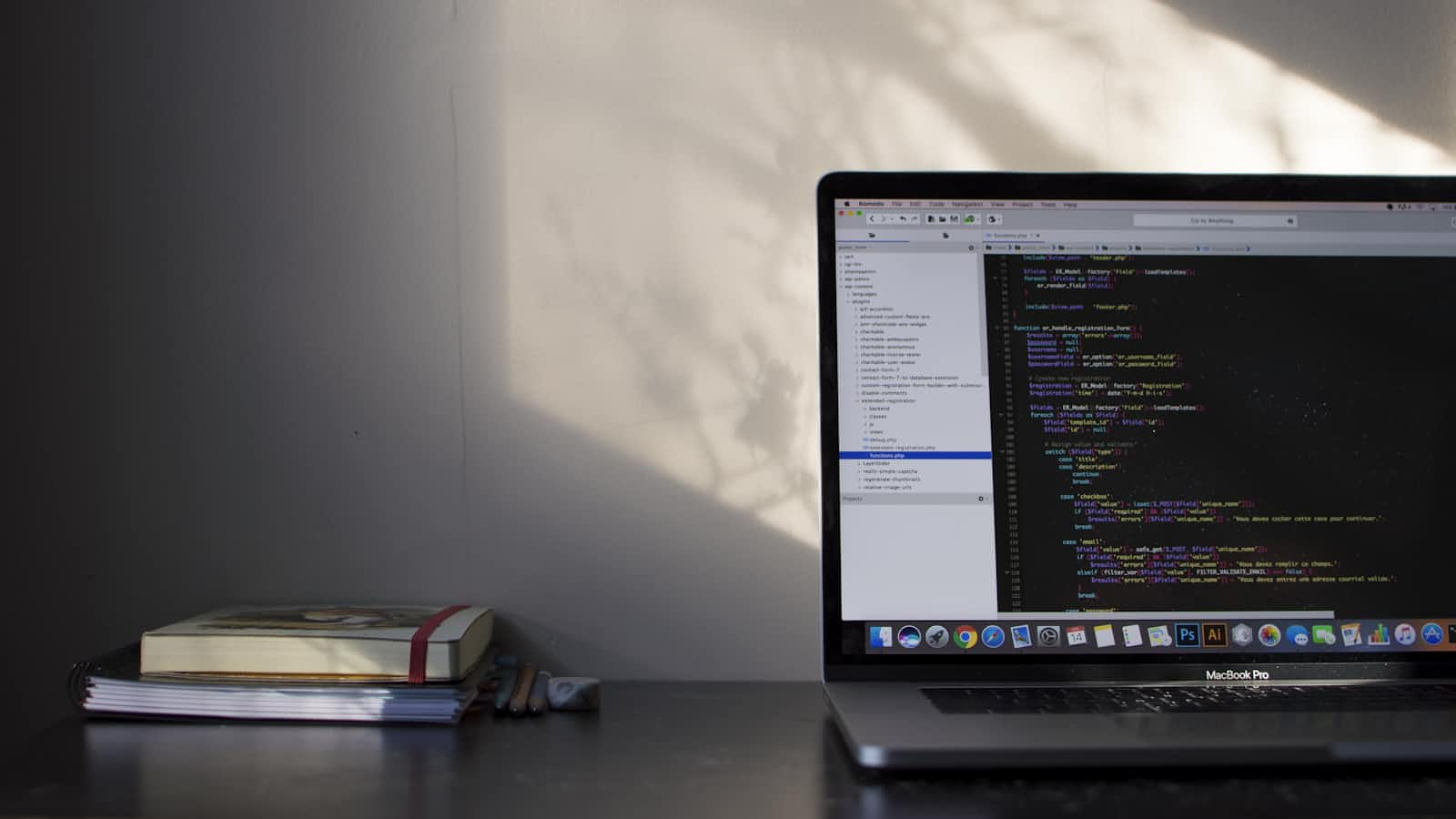
Enumerables!! We work with them all the time. In functional programming, enumerables are data structures that can be iterated (enumerated) over — meaning you can go through each element one by one and apply operations like map, filter, reduce, etc. More often than not you’ll find yourself in situations where you’ll need to produce a complex result (which can be another enumerable😆) from an enumerable. This is where folding comes in. Let’s start with the definition of a fold and why exactly you should care!
A fold is a way of getting a single value by operating on a list of values.
Enum.reduce/3 in Elixir is used precisely for folding and you can implement complex functions on top of it when required. You are going to see exactly why it is so effective through an example application that has practical instances where the same might be useful.
Code Samples
It will be better if you follow along by cloning this repository. Follow the instructions in the README for a quick setup.
Our app adds a customer and a list of their fruits into a Database. The main thing here is a customer can have multiple fruits added at the same time. Pretty simple, right ? Not so fast. We’ll see why the conventional form submission technique might not exactly work in this scenario and why we have to think of other ingenious methods. The program :
Quick Overview Approach
Normally when we want to get values out of forms in submission and validation callbacks, we typically use the %Phoenix.HTML.Form{} struct to capture the value in our form using the Access behaviour e.g
<.input field={@form[:name]} type="text" placeholder="Customer Name..." />
This works fine in most scenarios. But what if the form fields are dynamic? What if we do not know beforehand what the form fields are going to be? This is exactly the situation we have above. We do know that the customer has one name but we have absolutely no idea how many fruits they are going to buy (enter into the form). Lets break down the solution and see where we need to utilize folding.
Solution
Head over to lib/folding_in_elixir_web/live/fruits/index.ex
. That happens to be our parent LiveView. It is responsible for showing all the customers that have bought fruits. What we’re more interested in is the Live Components :
FoldingInElixirWeb.FruitsLive.NameComponent
FoldingInElixirWeb.FruitsLive.FruitComponent
The first live component is responsible for rendering a form that captures the customer name. I am a fan of separation of concerns which is why I ensured that the fruits would be uploaded solely in the form contained in FoldingInElixirWeb.FruitsLive.FruitComponent
. For the purposes of brevity, I will direct the remainder of this article to this.
Uploading Fruits
Go to lib/folding_in_elixir_web/live/fruits/components/fruit.component.ex
and inspect the code. By the way, I used a cool component library called Tremorx for the UI. You can check it out.
At the top you’ll find:
alias FoldingInElixir.{Helpers}
This Helpers module has a lot of functions that we’ll use.
Okay, now lets think of how we are going to solve this. First of all we have to realize that we do not want to limit the number of fruits that the user can upload. In this case a list must be involved. Each fruit field consists of the attributes name, price, quantity and total. Our main schema is the customer schema located in lib/folding_in_elixir/customer/customer.ex
but it embeds a fruit schema located in lib/folding_in_elixir/customer/fruit.ex
. I am not going to go into the nitty-gritty of embedded schemas but they are basically schemas that are not stored in their own database table, rather they are embedded within another schema's data structure — typically inside a map or JSON field.
defmodule FoldingInElixir.Market.Customer do
use Ecto.Schema
import Ecto.Changeset
@primary_key {:id, :binary_id, autogenerate: true}
@foreign_key_type :binary_id
schema "customers" do
field :name, :string
timestamps(type: :utc_datetime)
embeds_many :fruits, FoldingInElixir.Market.Fruit, on_replace: :delete
end
def changeset(customer, attrs) do
customer
|> cast(attrs, [
:name
])
|> validate_required(:name)
|> validate_name()
|> cast_embed(:fruits)
end
def name_changeset(customer, attrs) do
customer
|> cast(attrs, [
:name
])
|> validate_required(:name)
|> validate_name()
end
def validate_name(changeset) do
changeset
|> validate_length(:name,
min: 4,
max: 60,
message: "the name must be between 4 and 20 characters long"
)
end
end
We are going to use an empty map as the data structure for our form and build our own validation logic.
defp assign_form(socket) do
form = to_form(%{}, as: "fruits")
assign(socket, :form, form)
end
Initially no fruits exist and we want to track the fruits in the socket so we’ll initialize fruits to an empty list and the fruit count to 0 in the mount callback.
Clicking the New Customer button opens the modal and you we see a New Fruit button which is pushes a add_new_fruit
event. Here is where we need to be more creative.
def handle_event("add_new_fruit", _params, socket) do
fruits = socket.assigns.fruits
count = socket.assigns.fruit_count
new_count = count + 1
name = "fruit_" <> Integer.to_string(new_count) <> "_name"
quantity = "fruit_" <> Integer.to_string(new_count) <> "_quantity"
price = "fruit_" <> Integer.to_string(new_count) <> "_price"
total = "fruit_" <> Integer.to_string(new_count) <> "_total"
new_fruit = %{
id: new_count,
name: String.to_atom(name),
quantity: String.to_atom(quantity),
price: String.to_atom(price),
total: String.to_atom(total)
}
# to append the new_fruit into our list
new_fruits = fruits ++ [new_fruit]
{
:noreply,
socket
|> assign(fruit_count: new_count)
|> assign(:fruits, new_fruits)
}
end
Because at any event pushed a fruit count will be a unique integer e.g 3 , we can use that count to generate unique fields for each fruit field. A field on a Phoenix form must be an atom so that its value is accessed through the @form[:field]
syntax. We are going to generate these field names by prefixing “fruit” concatenating with count for uniqueness and finally ending it with the specific attribute e.g “name”
name = "fruit_" <> Integer.to_string(new_count) <> "_name"
Because each field is an atom we have to transform them by:
name: String.to_atom(name)
Now that we have our fruit map with all its required attributes, we are going to append it to our list and then assign our new count and fruits values into the socket.
In our heex template
we enumerate over the fruits list using the for comprehension syntax and access each of the atom fields using the dot syntax since each fruit is a map.
<%= for fruit <- @fruits do %>
<Layout.flex
flex_direction="row"
align_items="start"
justify_content="between"
class="gap-6"
>
<Layout.col class="space-y-1.5">
<div class={show_errors_on_fruit_field(fruit.id, @list_of_submitted_params)}>
<p class="text-red-400">This fruit's fields contains errors</p>
</div>
</Layout.col>
<Layout.col class="space-y-1.5">
<.input field={@form[fruit.name]} type="text" placeholder="Fruit Name..." />
</Layout.col>
<Layout.col class="space-y-1.5">
<.input field={@form[fruit.quantity]} type="number" placeholder="Quantity..." />
</Layout.col>
<Layout.col class="space-y-1.5">
<.input field={@form[fruit.price]} type="number" placeholder="Price..." />
</Layout.col>
<Layout.col class="space-y-1.5">
<.input field={@form[fruit.total]} type="text" readonly placeholder="Total..." />
</Layout.col>
<Layout.col class="space-y-1.5">
<div class={show_remove_fruit_button(fruit.id, @fruit_count)}>
<Button.button
variant="secondary"
size="xl"
class="mt-2 w-min"
phx-click={JS.push("remove_fruit", value: %{id: fruit.id})}
phx-target={@myself}
>
Remove Fruit
</Button.button>
</div>
</Layout.col>
</Layout.flex>
<% end %>
Now we have another problem. We want to show the total values when a user edits the price and quantity fields. For that we’ll use our validate callback for that purpose.
If you start entering values into the form and look at the fruit_params they are going to look something like this :
params = %{
"fruit_1_name" => "orange",
"fruit_1_price" => "23",
"fruit_1_quantity" => "34",
"fruit_1_total" => ""
}
We need to take this map and return something like this :
fruit_params => %{
"fruit_1_name" => "orange",
"fruit_1_price" => "4",
"fruit_1_quantity" => "4",
"fruit_1_total" => "16"
}
which we can then show to the user. Helpers.get_total/2 will help:
fruit_params = Helpers.get_totals(fruit_params, fruit_count)
Our fruit count will help us know what unique fields we will have to manipulate by returning a list of values from one to the current count essentially the number of fruits in the socket.
The get_total_helper function will then get invoked for each number in the list.
list = Enum.to_list(1..count)
list_of_maps_of_fruits =
Enum.map(list, fn x ->
get_total_helper(params, x)
end)
We will get the integer value of price and total and return an error string as the total field if one of the two is not a number:
case price == :error do
true ->
params =
Map.merge(params, %{"fruit_#{count}_total" => "quantity and price must be numbers"})
otherwise multiply the two values together:
total = elem(price, 0) * elem(quantity, 0)
params = Map.merge(params, %{"fruit_#{count}_total" => "#{total}"})
After that we group the fields of one fruit map together :
individual_map_for_fruit =
Enum.reduce(params, %{}, fn {key, value}, accumulator_map ->
case String.starts_with?(key, "fruit_#{count}") do
true ->
# this is the accumulator value to be used in the next iteration
Map.put(accumulator_map, key, value)
false ->
# do nothing to the accumulator
accumulator_map
end
end)
# * this code transforms our map from having string keys to having atom keys
# * in preparation for the next step
individual_map_with_atom_keys =
Enum.map(individual_map_for_fruit, fn {x, y} -> {String.to_atom(x), y} end)
|> Enum.into(%{})
individual_map_with_atom_keys
Reduce(enum, acc, fun) takes the enum which in this case will be a list of all the attributes, an accumulator which essentially is what we want our outcome to be and then a function to be invoked on all elements of the enumerable. In each subsequent iteration the accumulator is changed and the value of the last accumulator becomes the return value.
In this case acc will be an empty map. Let’s say count is 1. As long as a particular field’s key starts with fruit_1 that key-value pair will be added to our map of the first fruit. That means all 4 fields (name, quantity, price and total) will be added to that map. Now after that we convert our string keys to atoms.
We again use Enum.reduce/3 to merge all maps that were produced previously:
def merge_individual_maps_to_one(list_of_maps) do
merged_map =
Enum.reduce(list_of_maps, %{}, fn map, empty_map ->
Map.merge(empty_map, map)
end)
merged_map
end
This will work absolutely fine because there are no duplicate fields.
Saving the Fruits
Now that the total field has already been populated, saving our fields will not be that hard. If we have this :
fruit_params => %{
"fruit_1_name" => "Melon",
"fruit_1_price" => "2",
"fruit_1_quantity" => "5",
"fruit_1_total" => "10",
"fruit_2_name" => "Pineapple",
"fruit_2_price" => "30",
"fruit_2_quantity" => "10",
"fruit_2_total" => "300"
}
we need to transform it to this :
list_of_fruits #=> [
%{name: "Melon", total: "10", price: "2", quantity: "5"},
%{name: "Pineapple", total: "300", price: "30", quantity: "10"}
]
Let’s look at Helpers.get_list_of_params(fruit_params, count)
list_of_tuples =
Enum.reduce(params, [], fn {key, value}, list ->
case String.starts_with?(key, "fruit_#{count}") do
true ->
prefix = "fruit_#{count}_"
key = String.replace_prefix(key, prefix, "")
[{key, value} | list]
false ->
list
end
end)
This reduce block will essentially filter all the list parameters using the unique prefix “fruit_#{count}” and remove that unique prefix. If we had “fruit_1_name” now we have “name”. We will use the last block of code to add an errors key so that we know if that fruit map has errors or not.
map_of_fruit =
case Enum.find(map_of_fruit, fn {_key, value} ->
value == "" or value == "quantity and price must be numbers"
end) do
nil ->
map_of_fruit = Map.put(map_of_fruit, :errors, false)
map_of_fruit
_ ->
map_of_fruit = Map.put(map_of_fruit, :errors, true)
map_of_fruit
end
That’s essentially it. Lastly we send the list of correct parameters to our parent LiveView using:
send(self(), {:valid_fruit_details, {customer, list_of_fruit_params}})
so that they can be submitted there.
Thanks
I hope you learnt a lot about folding and its applications. There are segments of this example app that I have not touched but I hope you will figure them out. Thanks for your time and continue programming ☺️.
Subscribe to my newsletter
Read articles from Dean Kinyua directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
