Mutability vs Immutability in JavaScript: A Tale of Two Datas
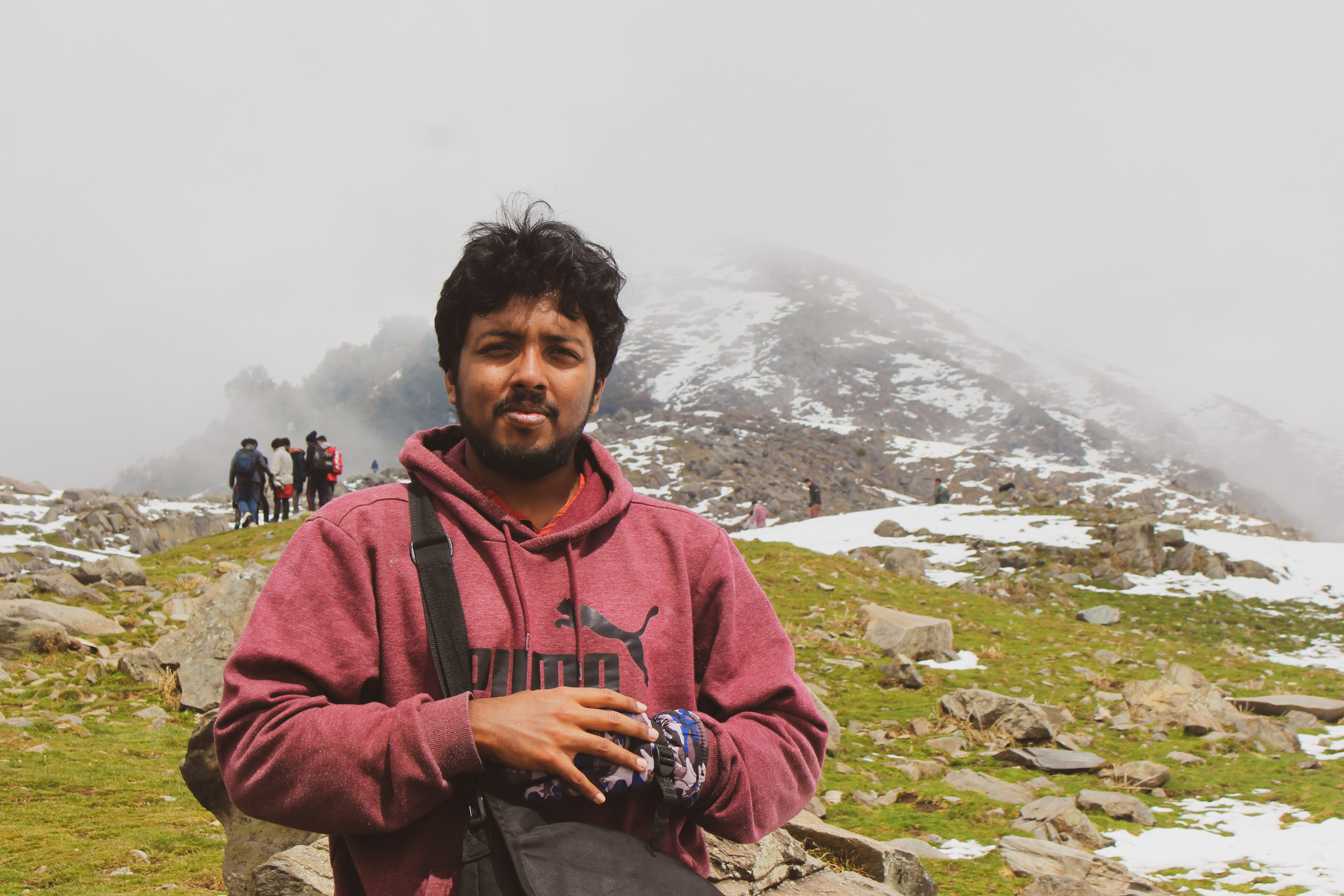
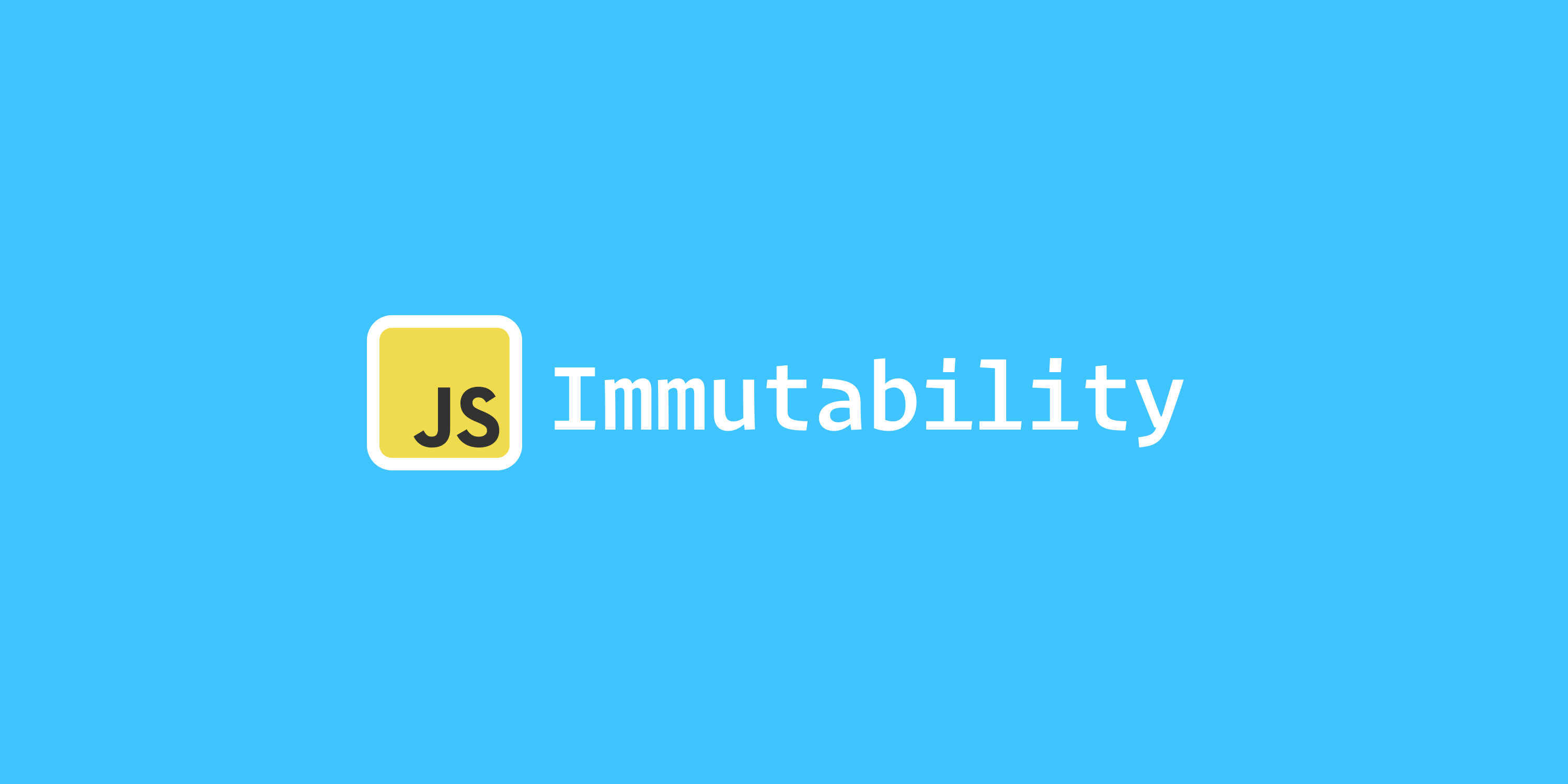
JavaScript is like a magical land where data can either be a rebel (mutating and changing all the time) or a saint (immutable and forever pure). But what does this mean for you, the developer? Let’s dive into the quirky world of mutability and immutability and uncover the secrets to writing clean, efficient, and bug-free code.
1. Mutability: The Rebel
Imagine you have an array:
javascript
Copy
let partyList = ["Alice", "Bob"];
You decide to add a new friend:
javascript
Copy
partyList.push("Charlie");
Now, partyList
is ["Alice", "Bob", "Charlie"]
. The original array has been mutated. It’s like inviting Charlie to the party and rearranging the furniture to make space. Fun, but chaotic!
Why Mutability Can Be Tricky
Side Effects: If you pass
partyList
to a function and it mutates the array, the original list changes everywhere. This can lead to bugs that are hard to track down.Unpredictability: Mutating data makes your code less predictable. You never know when someone might change your data behind your back.
2. Immutability: The Saint
Now, let’s say you want to keep your original party list intact. Instead of mutating it, you create a new list with the added guest:
javascript
Copy
let partyList = ["Alice", "Bob"];
let newPartyList = [...partyList, "Charlie"];
Here, partyList
remains ["Alice", "Bob"]
, and newPartyList
is ["Alice", "Bob", "Charlie"]
. It’s like throwing a second party instead of crashing the first one. Peaceful, right?
Why Immutability Rocks
Predictability: Your original data stays safe. No surprises!
Debugging: Easier to trace changes since data isn’t being modified in place.
Functional Programming: Immutability is a core principle in functional programming, making your code cleaner and more declarative.
3. Pass-by-Reference vs Pass-by-Value
Primitive Types: Pass-by-Value
Primitive types like number
, string
, and boolean
are passed by value. Think of it as handing someone a photocopy of your favorite recipe. They can scribble on it, but your original recipe stays safe.
javascript
Copy
let recipe = "Pancakes";
function changeRecipe(r) {
r = "Waffles";
}
changeRecipe(recipe);
console.log(recipe); // "Pancakes" (unchanged)
Objects and Arrays: Pass-by-Reference
Objects and arrays are passed by reference. It’s like giving someone your actual recipe book. If they spill coffee on it, your book is ruined.
javascript
Copy
let recipeBook = { breakfast: "Pancakes" };
function spillCoffee(book) {
book.breakfast = "Coffee-Stained Pancakes";
}
spillCoffee(recipeBook);
console.log(recipeBook.breakfast); // "Coffee-Stained Pancakes" (ruined!)
4. Shallow Copy vs Deep Copy
Shallow Copy: The Surface-Level Clone
A shallow copy duplicates only the top-level properties. Nested objects or arrays are still shared by reference. It’s like copying the cover of a book but not the pages inside.
javascript
Copy
let original = { food: { breakfast: "Pancakes" } };
let shallowCopy = { ...original };
shallowCopy.food.breakfast = "Waffles"; // Affects the original
console.log(original.food.breakfast); // "Waffles" (oh no!)
Deep Copy: The Full Clone
A deep copy creates a completely independent copy, including all nested objects or arrays. It’s like photocopying the entire book, page by page.
javascript
Copy
let original = { food: { breakfast: "Pancakes" } };
let deepCopy = JSON.parse(JSON.stringify(original));
deepCopy.food.breakfast = "Waffles"; // Doesn’t affect the original
console.log(original.food.breakfast); // "Pancakes" (safe!)
Pro Tip: Use libraries like lodash (_.cloneDeep
) for robust deep copying.
5. Advanced Concepts
Proxies: The Data Bodyguards
JavaScript Proxies let you intercept and customize operations on objects. They’re like bodyguards for your data, ensuring no one messes with it without your permission.
javascript
Copy
let target = { food: "Pancakes" };
let handler = {
set(obj, prop, value) {
console.log(`Setting ${prop} to ${value}`);
obj[prop] = value;
return true;
},
};
let proxy = new Proxy(target, handler);
proxy.food = "Waffles"; // Logs: "Setting food to Waffles"
Closures: The Memory Keepers
Closures capture and retain references to outer variables. If the captured variable is an object or array, changes to it persist across function calls.
javascript
Copy
function createCounter() {
let count = 0;
return function () {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
Structural Sharing: The Memory Saver
Immutable libraries like Immutable.js use structural sharing to optimize memory usage. Instead of creating a completely new copy, they reuse unchanged parts of the original data structure.
javascript
Copy
const { Map } = require("immutable");
const map1 = Map({ a: 1, b: 2 });
const map2 = map1.set("b", 3);
console.log(map1 === map2); // false (different references)
console.log(map1.get("a") === map2.get("a")); // true (shared reference for unchanged data)
6. Practical Tips for Working with Immutable Data
Use the Spread Operator (
...
)For arrays:
const newArr = [...arr, newItem];
For objects:
const newObj = { ...obj, newProp: value };
Leverage Libraries
Use Immer for simpler immutable updates.
Use lodash for deep cloning and equality checks.
Avoid Direct Mutations
- Use methods like
map
,filter
, andreduce
instead ofpush
,pop
, orsplice
.
- Use methods like
Adopt Functional Programming Principles
Write pure functions that don't modify their inputs.
Use higher-order functions like
map
andfilter
.
Conclusion
Understanding mutability and immutability in JavaScript is essential for writing clean, efficient, and bug-free code. By mastering these concepts, you'll be better equipped to work with modern JavaScript frameworks and libraries, manage state effectively, and avoid common pitfalls.The way Redux and React built upon the immutability of javascript helps you code smarter!
Whether you're working on a small project or a large-scale application, embracing immutability can lead to more predictable and maintainable code 🚀
Subscribe to my newsletter
Read articles from Vysyakh Ajith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
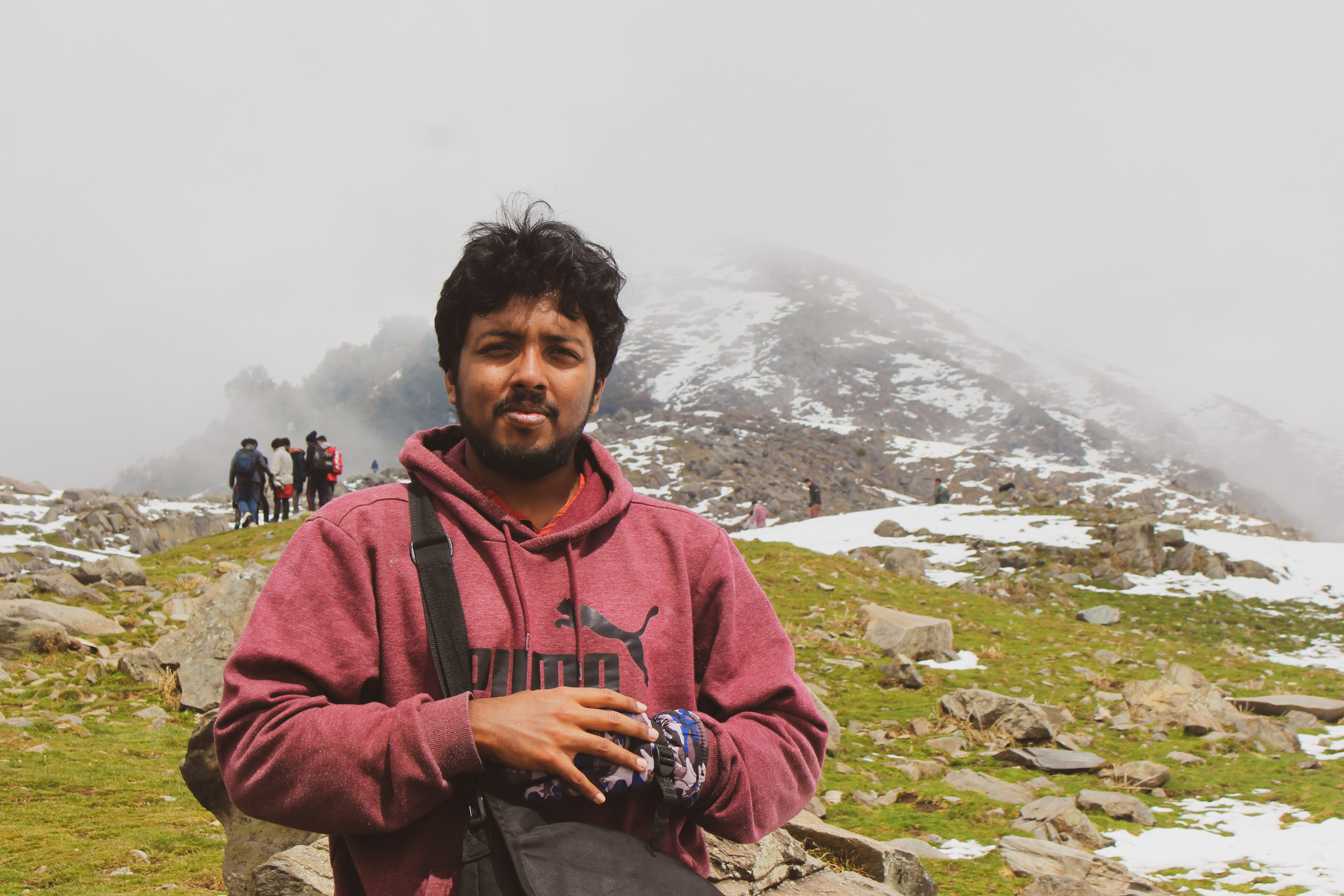
Vysyakh Ajith
Vysyakh Ajith
Aspiring full-stack developer looking forward to create end-to-end reactive web solutions with experience innovation as the motto