Optimizing List Performance in .NET MAUI: Best Alternatives & Recommendations

Table of contents
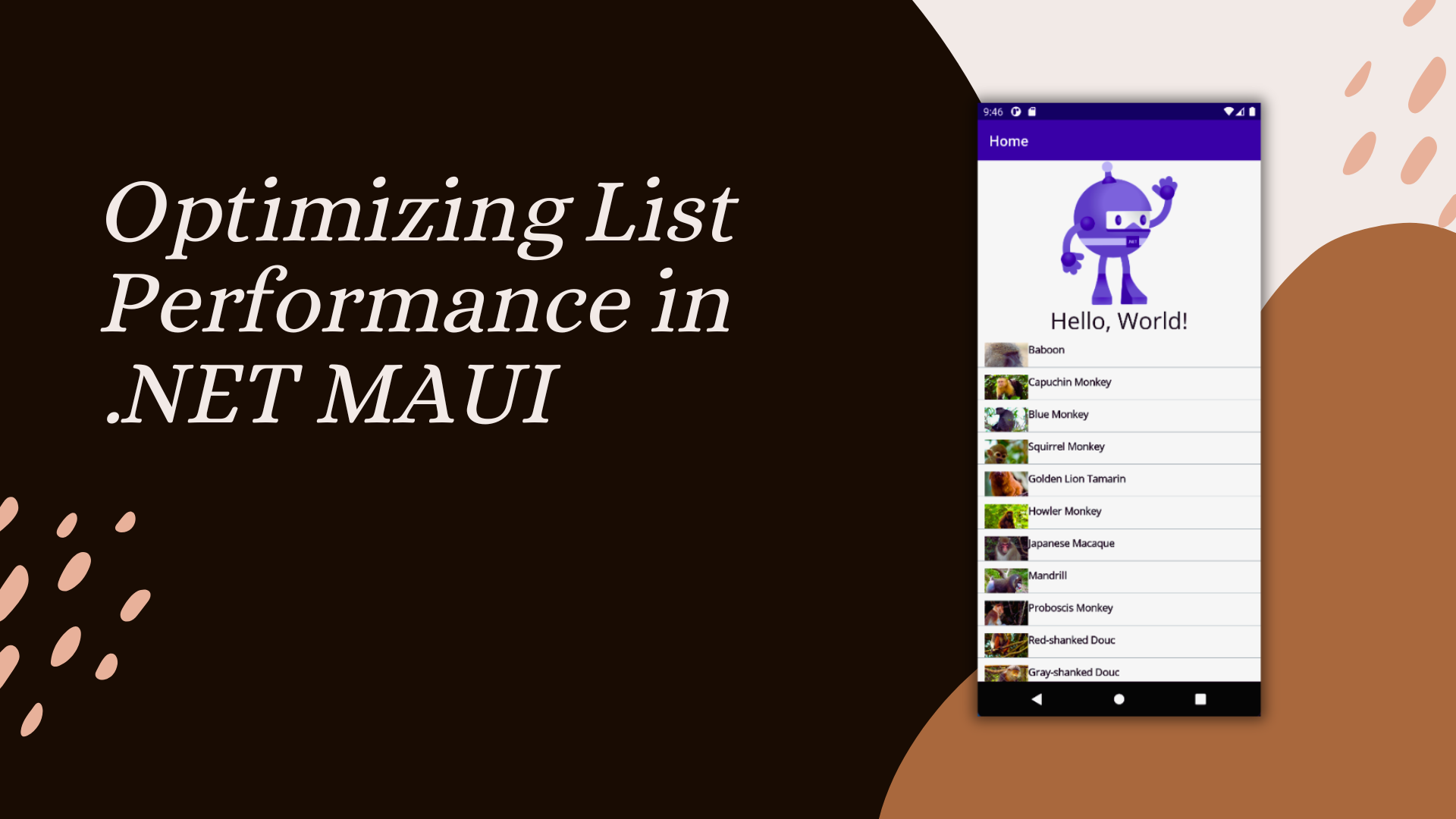
Introduction
In modern app development, displaying lists is a common requirement, whether the data set is small or large. In .NET MAUI, CollectionView
is often the solution, but many developers encounter performance challenges when working with it.
In this guide, we’ll explore effective techniques to optimize CollectionView
performance and discuss alternative solutions for scenarios where it may not be the best choice.
CollectionView in .NET MAUI
When working with lists in .NET MAUI, CollectionView
is often the first choice due to its flexibility and ease of integration. If you're new to CollectionView
, we can check out the official documentation for a detailed guide:
🔗 CollectionView Documentation
While CollectionView
is optimized for display, many developers face performance challenges—especially on Android. On platforms like Windows, iOS, and macOS, it generally performs well with minimal issues.
Let’s explore it’s solution🚀
1️⃣ Reduce Hierarchy in DataTemplate
One of the biggest performance issues is a deeply nested UI hierarchy. Keeping your DataTemplate
structure as simple as possible can significantly boost performance. Let’s compare two examples:
❌ Inefficient Approach (Deep Hierarchy)
<CollectionView>
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid RowDefinitions="Auto,Auto" ColumnDefinition="*,Auto">
<StackLayout Grid.Row="0" Grid.Column="0">
<Label Text="Hello!"/>
</StackLayout>
<StackLayout Grid.Row="0" Grid.Column="1">
<Label Text="Hello!"/>
</StackLayout>
<StackLayout Grid.Row="1" Grid.ColumnSpan="2">
<Label Text="Hello!"/>
</StackLayout>
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
✅ Optimized Approach (Simplified Hierarchy)
<CollectionView>
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid RowDefinitions="Auto,Auto" ColumnDefinitions="*,Auto">
<Label Text="Hello!"/>
<Label Grid.Column="1" Text="Hello!"/>
<Label Grid.Row="1" Grid.ColumnSpan="2" Text="Hello!"/>
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
Although both examples look the same, removing unnecessary nesting improves rendering speed. The simpler the hierarchy, the better the performance.
2️⃣ Prefer Grid Over StackLayout & FlexLayout
StackLayout
is useful but recalculates child sizes dynamically, which can impact performance.FlexLayout
is powerful but often unnecessary for simple lists.✅
Grid
is the best choice forCollectionView
items because it avoids unnecessary layout recalculations and enhances efficiency.
Example: Instead of using a StackLayout
, use a Grid
to structure items efficiently.
3️⃣ Use Compiled Bindings for Faster Rendering
Microsoft recommends using compiled bindings (x:DataType
) to significantly boost performance. It enables compile-time checking and eliminates runtime reflection overhead.
✅ How to Enable Compiled Binding
xmlCopyEdit<CollectionView ItemsSource="{Binding Items}">
<CollectionView.ItemTemplate>
<DataTemplate x:DataType="{x:Type local:YourModel}">
<Grid>
<Label Text="{Binding Name}" />
<Label Text="{Binding Description}" />
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
By simply adding x:DataType
, we can achieve major performance improvements 🚀.
4️⃣ .NET 9 Performance Enhancements
With .NET 9, Microsoft introduced optimized handlers for CollectionView
, reducing UI lag and improving overall responsiveness.
✅ Enable New CollectionView Handlers
csharpCopyEdit#if IOS || MACCATALYST
builder.ConfigureMauiHandlers(handlers =>
{
handlers.AddHandler<Microsoft.Maui.Controls.CollectionView, Microsoft.Maui.Controls.Handlers.Items2.CollectionViewHandler2>();
handlers.AddHandler<Microsoft.Maui.Controls.CarouselView, Microsoft.Maui.Controls.Handlers.Items2.CarouselViewHandler2>();
});
#endif
If our app targets iOS or MacCatalyst, implementing these handlers can make a noticeable difference in performance.
5️⃣ Avoid Wrapping CollectionView in a ScrollView ❌
Many developers mistakenly wrap CollectionView
inside a ScrollView
, thinking it improves scrolling behavior. This is incorrect and harms performance because CollectionView
already has built-in scrolling.
❌ Incorrect Approach (Causes Performance Issues)
<ScrollView>
<CollectionView>
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid>
<Label Text="Hello!" />
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
</ScrollView>
✅ Correct Approach (Improves Performance)
<Grid>
<CollectionView>
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid>
<Label Text="Hello!" />
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
</Grid>
This ensures that CollectionView
handles scrolling efficiently without unnecessary nested scrollable elements.
6️⃣ Use ItemSizingStrategy for Faster Loading
If we don’t need dynamic item heights, forcing CollectionView
to measure only the first item can drastically improve performance. It may not use much in real life examples, but it is still an effective approach
✅ Optimize Item Measurement
xmlCopyEdit<CollectionView ItemSizingStrategy="MeasureFirstItem">
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid>
<Label Text="Hello!" />
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
</CollectionView>
This reduces the overhead of measuring every item individually, making the list render faster.
When NOT to Use CollectionView 🚫
While CollectionView
is powerful, it’s not always the best option. Consider alternatives in these cases:
✅ For Small Data Sets – If your list has very few items (e.g., 3–5 options), a simple StackLayout
or ListView
might be more efficient.
✅ For Static Data – In cases like settings pages, where data doesn’t change dynamically, using BindableLayout
instead of CollectionView
can be better for performance.
Alternatives
✨ Bindable Layout
Bindable Layout is one of the best options, especially in terms of smooth scrolling. It arranges elements sequentially, eliminating the need for caching, resulting in consistent performance across all platforms.
📚 More details: Microsoft Docs
🔄 Advantages
✅ Very smooth scrolling performance.
✅ Ideal for small datasets.
❌ Disadvantages
❌ Fails with large lists (10+ items).
❌ No built-in features like Load More, Span, etc.
✍️ Workaround for Large Lists
Even though Bindable Layout is best suited for small lists, we can extend its functionality using a Load More feature. One way to do this is by using a custom scroll view implementation:
public class LoadMoreScrollView : Microsoft.Maui.Controls.ScrollView
{
#region Constructor
public LoadMoreScrollView()
{
try
{
this.Scrolled += LoadMoreScrollView_Scrolled;
}
catch(Exception ex) { SentrySdk.CaptureException(ex); }
}
private void LoadMoreScrollView_Scrolled(object? sender, ScrolledEventArgs e)
{
try
{
var scrollView = sender as Microsoft.Maui.Controls.ScrollView;
if (scrollView == null || ItemThreshold == -1) return;
// Check if the user scrolled near the bottom
if (scrollView.ScrollY >= scrollView.ContentSize.Height - scrollView.Height - 100)
{
// Trigger the LoadMoreCommand
LoadMoreCommand?.Execute(null);
}
}
catch(Exception ex) { SentrySdk.CaptureException(ex); }
}
#endregion
#region BindableProperties
public static readonly BindableProperty ItemThresholdProperty = BindableProperty.Create(
propertyName: nameof(ItemThreshold),
returnType: typeof(int),
declaringType: typeof(LoadMoreScrollView),
defaultValue: -1,
defaultBindingMode: BindingMode.TwoWay);
public int ItemThreshold
{
get => (int)GetValue(ItemThresholdProperty);
set { SetValue(ItemThresholdProperty, value); }
}
public static readonly BindableProperty LoadMoreCommandProperty =
BindableProperty.Create(nameof(LoadMoreCommand), typeof(ICommand), typeof(LoadMoreScrollView));
public ICommand LoadMoreCommand
{
get => (ICommand)GetValue(LoadMoreCommandProperty);
set => SetValue(LoadMoreCommandProperty, value);
}
#endregion
}
🌟 ListView
ListView is an older but highly optimized option. Compared to CollectionView, its scrolling performance is better, especially for small to medium datasets. If Bindable Layout struggles with large data, and we don't want to implement custom Load More functionality, ListView is a solid choice.
📚 More details: Microsoft Docs
🔄 Advantages
✅ Highly optimized for small to medium lists.
✅ Best suited for static datasets.
❌ Disadvantages
❌ Struggles with large datasets.
❌ Lacks built-in features like Load More, Grid Span, etc.
⚠️ Important: Always set ListViewCachingStrategy
to RecycleElementAndDataTemplate
or another appropriate option to avoid performance issues.
💎 Community Packages
If the default MAUI options don’t meet your needs, several third-party libraries provide enhanced performance and features.
⚡ MPowerKit.VirtualizeListView
A highly optimized virtualized list implementation, cross-platform, and efficient. It is my favorite in terms of scrolling and cross platform consistency
📚 Integration: GitHub Repository
🔄 Advantages
✅ Supports Grid Span, Load More, Grouping, Headers, and Footers etc.
✅ Excellent for large datasets (Tested with 220+ rows and smooth scrolling).
✅ Built-in caching for improved performance.
✅ Consistent performance across platforms.
❌ When Not to Use
- If we need to display data incrementally over time, Bindable Layout may be a better choice.
🎨 DevExpress CollectionView
This alternative enhances scrolling performance by adding animations and rendering elements one by one for better efficiency.
🔄 Advantages
✅ Best option for all scenarios.
✅ Handles very complex data templates smoothly (I use in my one project where all fails because i have a large list and very complex data. But it handles very smoothly).
✅ Includes all essential list features.
❌ Disadvantages
- ❌ Limited to Android and iOS.
💻 Maui.VirtualListView
An optimized virtual list for native-level smooth scrolling. I have not used it much but it’s very optimize.
📚 Integration: GitHub Repository
🔄 Advantages
✅ Feels like a native scrolling experience.
✅ Performance is smooth and stable.
💼 Paid Libraries
For enterprise solutions, paid libraries provide advanced performance optimizations and feature-rich components.
🏢 Syncfusion ListView & Telerik CollectionView
These premium components are highly optimized, cross-platform, and provide seamless scrolling.
🔄 Advantages
✅ Fully cross-platform.
✅ Extremely smooth scrolling and animations.
✅ Comes with built-in advanced features.
❌ Disadvantages
- ❌ Requires a paid subscription.
Conclusion:
Choosing the right list control in .NET MAUI depends on your specific requirements. Bindable Layout is great for small static lists, while ListView works well for medium-sized datasets. For large and complex lists, MPowerKit.VirtualizeListView and DevExpress CollectionView provide smooth scrolling and efficient rendering. If you need enterprise-level solutions, Syncfusion and Telerik offer premium, feature-rich options. Selecting the best approach will ensure optimal performance, responsiveness, and user experience for your MAUI application! 🚀
Subscribe to my newsletter
Read articles from Haider Ali Faizi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
