Integrating HashiCorp Vault with Laravel on DigitalOcean

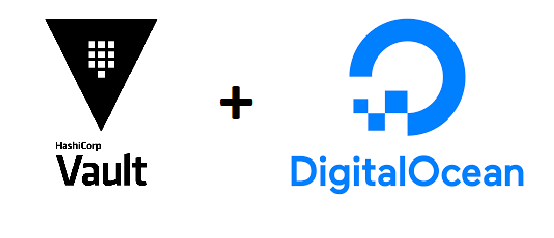
This documentation outlines the steps to integrate HashiCorp Vault into a Laravel application hosted on DigitalOcean using Laravel Forge. The goal is to securely manage secrets without hardcoding them in the codebase.
1. Understanding the Process
Laravel applications typically store sensitive credentials in an .env
file. Instead of hardcoding these secrets, we will use Vault to manage them dynamically.
Vault Address, Token, and Secret Path will be stored in the
.env
file.Laravel will fetch secrets from Vault at runtime.
The DevOps team will automate deployments to reduce manual handling of secrets.
2. Prerequisites
Before integrating Vault, ensure the following:
✅ HashiCorp Vault is installed and configured on your server.
✅ You have root access to Vault.
✅ Your Laravel application is running on DigitalOcean with Laravel Forge.
✅ Your team has access to modify the .env
file and deployment pipelines.
3. Setting Up Vault Secrets
Step 1: Store Secrets in Vault
The security team (or Vault admin) should create and store secrets in Vault:
vault kv put secret/my-app database_password=SuperSecureP@ssw0rd
To verify the stored secret:
vault kv get secret/my-app
Step 2: Configure Vault in Laravel
In the .env
file, add the Vault-related variables:
VAULT_ADDR=http://your-vault-server:8200
VAULT_TOKEN=your-root-or-app-token # it is advisable to only use the spp token and never display your root token.
VAULT_SECRET_PATH=secret/my-app
These values do not store the actual secrets but allow Laravel to authenticate and fetch secrets dynamically.
Step 3: Extract Secrets from Vault in Laravel
Modify config/services.php
to pull secrets from Vault:
return [
'database' => [
'password' => env('DATABASE_PASSWORD', null) ?? getVaultSecret('database_password'),
],
];
Now, define the getVaultSecret
function in a helper file:
function getVaultSecret($key)
{
$client = new \GuzzleHttp\Client();
$vaultAddr = env('VAULT_ADDR');
$vaultToken = env('VAULT_TOKEN');
$vaultPath = env('VAULT_SECRET_PATH');
$response = $client->get("$vaultAddr/v1/$vaultPath", [
'headers' => [
'X-Vault-Token' => $vaultToken,
],
]);
$data = json_decode($response->getBody()->getContents(), true);
return $data['data'][$key] ?? null;
}
Step 4: Test Connection to Vault
Run the following command to verify that Laravel can fetch secrets from Vault:
php artisan tinker
>>> getVaultSecret('database_password');
If configured correctly, it should return the stored password from Vault.
4. Automating Deployment (Recommended)
Instead of manually handling secrets, use Laravel Forge to automate deployments:
Enable Git Hooks to deploy on push.
Configure CI/CD Pipelines (GitHub Actions, GitLab CI/CD) to inject Vault secrets dynamically.
Example GitHub Actions workflow:
name: Deploy Laravel App
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Fetch Secrets from Vault
run: |
export VAULT_ADDR=http://your-vault-server:8200
export VAULT_TOKEN=your-root-or-app-token
export DATABASE_PASSWORD=$(vault kv get -field=database_password secret/my-app)
echo "DATABASE_PASSWORD=${DATABASE_PASSWORD}" >> .env
- name: Deploy to DigitalOcean
run: ./deploy.sh
5. Key Actions
Security Team: Sets up Vault, creates secrets, and provides authentication details.
Developer Team: Implements logic to fetch secrets dynamically in Laravel.
Developer Operations Team: Automates deployment using Git hooks or CI/CD.
w/ this setup, you ensure that secrets are never hardcoded, reducing security risks and simplifying management across environments.
Subscribe to my newsletter
Read articles from Imam Bashir Abdulwahab (Twenty4) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Imam Bashir Abdulwahab (Twenty4)
Imam Bashir Abdulwahab (Twenty4)
An open Source Security Advocate