Mastering ABAP Field Symbols #01
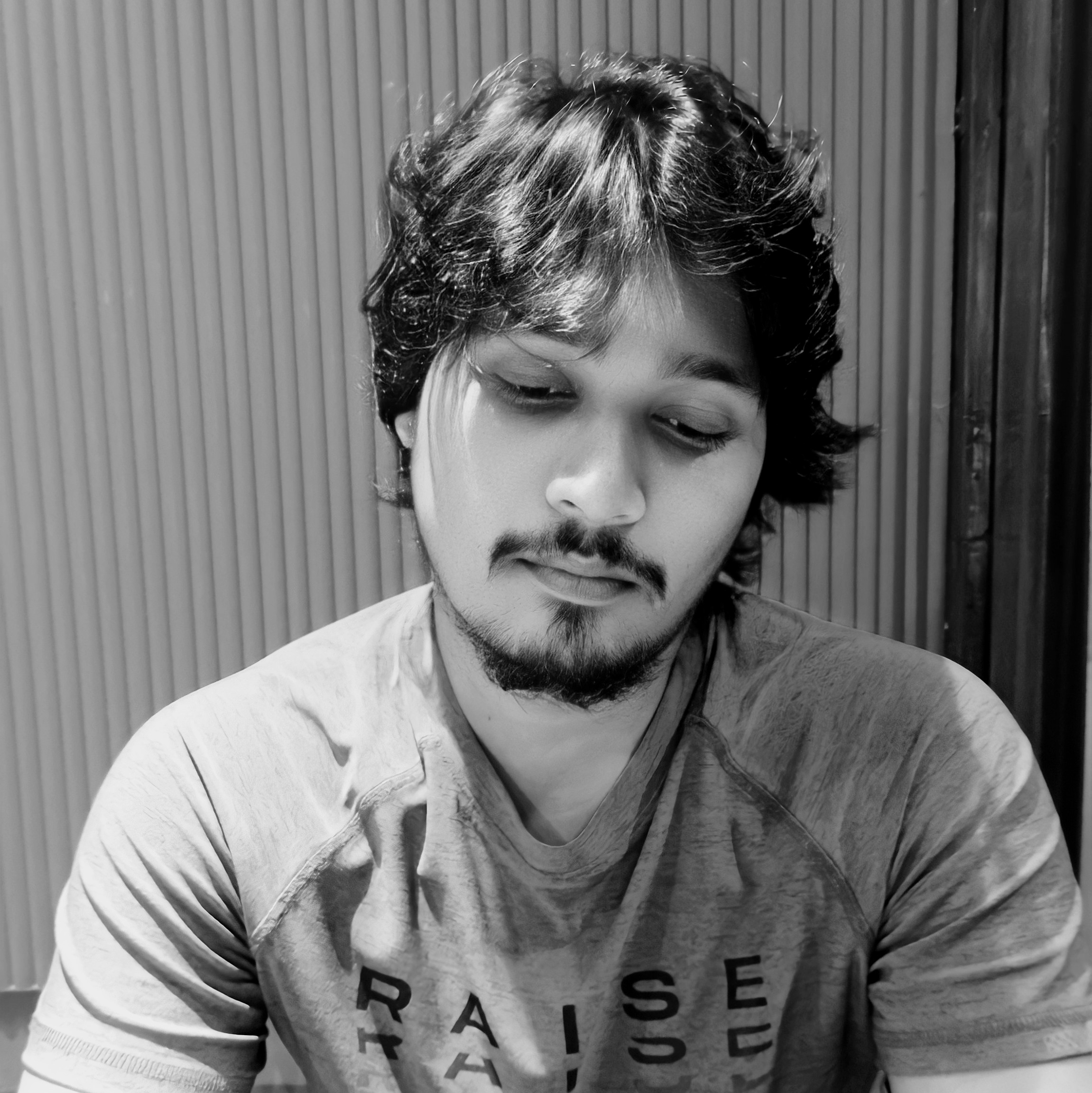
Unlock the Power of Pointers in ABAP Without the Headaches
If you’ve ever wished for a magic wand in ABAP to manipulate data without duplicating memory or writing endless MODIFY
statements, field symbols are your answer. Think of them as post-it notes that point directly to data in memory—lightweight, efficient, and dynamic. In this tutorial, we’ll demystify field symbols, explore their superpowers, and build a real-world project to cement your understanding.
What Are Field Symbols?
Imagine you’re organizing a library. Instead of photocopying every book (consuming shelf space), you place colorful post-it notes on the originals. These notes don’t hold the content—they simply point to where the book resides. Field symbols work similarly:
They don’t store data but reference memory addresses of variables, structures, or internal tables.
Changes via field symbols directly affect the original data (like editing the actual book instead of a copy).
Why Use Field Symbols?
Memory Efficiency: No redundant copies.
Dynamic Programming: Handle data types and structures determined at runtime.
Simplified Code: Modify internal tables directly without
MODIFY
statements.
Declaring Field Symbols: Typed vs. Generic
1. Typed Field Symbols
Specify the data type upfront for type safety.
FIELD-SYMBOLS: <fs_number> TYPE i. " Points only to integers
2. Generic Field Symbols
Use TYPE any
or TYPE data
for flexibility in dynamic scenarios.
FIELD-SYMBOLS: <fs_generic> TYPE any. " Can point to any data type
Analogy: Typed symbols are like labeled folders (you know what’s inside). Generic symbols are mystery boxes (flexible but need caution).
Hands-On Example: The Two-Way Mirror
Let’s create a program where a field symbol and variable reflect each other’s changes.
Step 1: Declare and Assign
DATA: gv_number TYPE i VALUE 15.
FIELD-SYMBOLS: <fs_num> TYPE i.
ASSIGN gv_number TO <fs_num>. " Post-it note on gv_number
Step 2: Modify and Observe
WRITE: / 'Original Value:', gv_number.
IF <fs_num> IS ASSIGNED.
<fs_num> = 13. " Change via field symbol
WRITE: / 'New Value via Field Symbol:', <fs_num>,
/ 'Updated Original Variable:', gv_number.
ENDIF.
Output:
Original Value: 25
New Value via Field Symbol: 13
Updated Original Variable: 13
Key Insight: Field symbols are two-way mirrors. Change one side, and the other updates automatically.
Real-World Example: Parsing Financial Documents
In finance modules, documents like BSEG
or BKPF
often store composite keys (e.g., company code + document number). Let’s extract parts of these keys using field symbols.
Scenario:
A document key GV_KEY
stores 1000DOC1234562023
where:
Positions 0-3: Company code (
1000
)Positions 4-13: Document number (
DOC123456
)Positions 14-17: Year (
2023
)
Step 1: Declare Variables and Field Symbols
DATA: gv_key TYPE c LENGTH 18 VALUE '1000DOC1234562023'.
FIELD-SYMBOLS:
<fs_company> TYPE c,
<fs_doc> TYPE c,
<fs_year> TYPE c.
Step 2: Assign Substrings Dynamically
" Extract company code (positions 0-3)
ASSIGN gv_key+0(4) TO <fs_company>.
" Extract document number (positions 4-13)
ASSIGN gv_key+4(9) TO <fs_doc>.
" Extract year (positions 14-17)
ASSIGN gv_key+13(5) TO <fs_year>.
Step 3: Use the Values
IF <fs_company> IS ASSIGNED.
WRITE: / 'Company Code:', <fs_company>. " Output: 1000
ENDIF.
IF <fs_doc> IS ASSIGNED.
WRITE:/ 'Doc Code:', <fs_doc>. " Output: DOC123456
ENDIF.
IF <fs_year> IS ASSIGNED.
WRITE:/ 'Year:', <fs_year>. " Output: 2023
ENDIF.
Why This Matters: Avoid creating multiple intermediate variables. Directly reference substrings!
Best Practices: Don’t Get Dumped!
- Always Check Assignment
Unassigned field symbols cause runtime errors. UseIS ASSIGNED
:
IF <fs_data> IS ASSIGNED.
" Safe to use here
ENDIF.
- Unassign When Done
Free up references to prevent unintended side effects.
UNASSIGN <fs_data>.
- Prefer Typed Symbols
Use generic symbols (TYPE any
) only when necessary for dynamic logic.
Project: Build a Dynamic Internal Table Processor
Objective: Create a program that uses field symbols to:
Populate an internal table.
Modify all rows by doubling numeric fields.
Avoid
LOOP ... MODIFY
statements.
Step 1: Declare Structures and Table
TYPES: BEGIN OF ty_data,
id TYPE i,
value TYPE i,
END OF ty_data.
DATA: gt_data TYPE TABLE OF ty_data,
gs_data TYPE ty_data.
FIELD-SYMBOLS: <fs_row> TYPE ty_data.
Step 2: Populate Data
DO 5 TIMES.
gs_data-id = sy-index.
gs_data-value = sy-index * 10.
APPEND gs_data TO gt_data.
ENDDO.
Step 3: Double Values Using Field Symbols
LOOP AT gt_data ASSIGNING <fs_row>.
<fs_row>-value = <fs_row>-value * 2.
ENDLOOP.
Magic: No MODIFY
needed! Changes via <fs_row>
update gt_data
directly.
Complete Solution using modern ABAP syntax:
TYPES: BEGIN OF ty_data,
id TYPE i,
value TYPE i,
END OF ty_data.
DATA: gt_data TYPE TABLE of ty_data,
* gs_data TYPE ty_data.
FIELD-SYMBOLS: <fs_row> TYPE ty_data.
*do 5 TIMES.
* gs_data-id = sy-index.
* gs_data-value = sy-index * 10.
* APPEND gs_data to gt_data.
*ENDDO.
" Populate internal table using modern ABAP syntax
DO 5 TIMES.
APPEND VALUE #( id = sy-index value = sy-index * 10 ) TO gt_data.
ENDDO.
WRITE: / 'Before modification'.
NEW-LINE.
" Display data using field symbol (more efficient than INTO)
LOOP AT gt_data ASSIGNING <fs_row>.
WRITE: / |ID: { <fs_row>-id }, Value: { <fs_row>-value }|. " String template
ENDLOOP.
" Modify values using field symbol (direct table modification)
LOOP AT gt_data ASSIGNING <fs_row>.
<fs_row>-value = <fs_row>-value * 2.
ENDLOOP.
SKIP. " Add empty line
WRITE: / 'After modification'.
NEW-LINE.
" Display modified data
LOOP AT gt_data ASSIGNING <fs_row>.
WRITE: / |ID: { <fs_row>-id }, Value: { <fs_row>-value }|.
ENDLOOP.
Conclusion: Field Symbols Are Your ABAP Superpower
Field symbols transform how you handle data—making your code faster, cleaner, and more dynamic. Whether you’re parsing financial keys or processing massive tables, they’re indispensable.
Your Challenge:
Modify the project to handle a generic structure (e.g., add a currency
field) and use a generic field symbol to update values. Share your solution in the comments!
Ready to level up your ABAP game? Field symbols are just the beginning. 🚀
Loved this? Follow me on Hashnode for more ABAP deep-dives, and smash that like button if you’d use field symbols to parse your next document key! 💬✨
Subscribe to my newsletter
Read articles from Fahim Al Jadid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
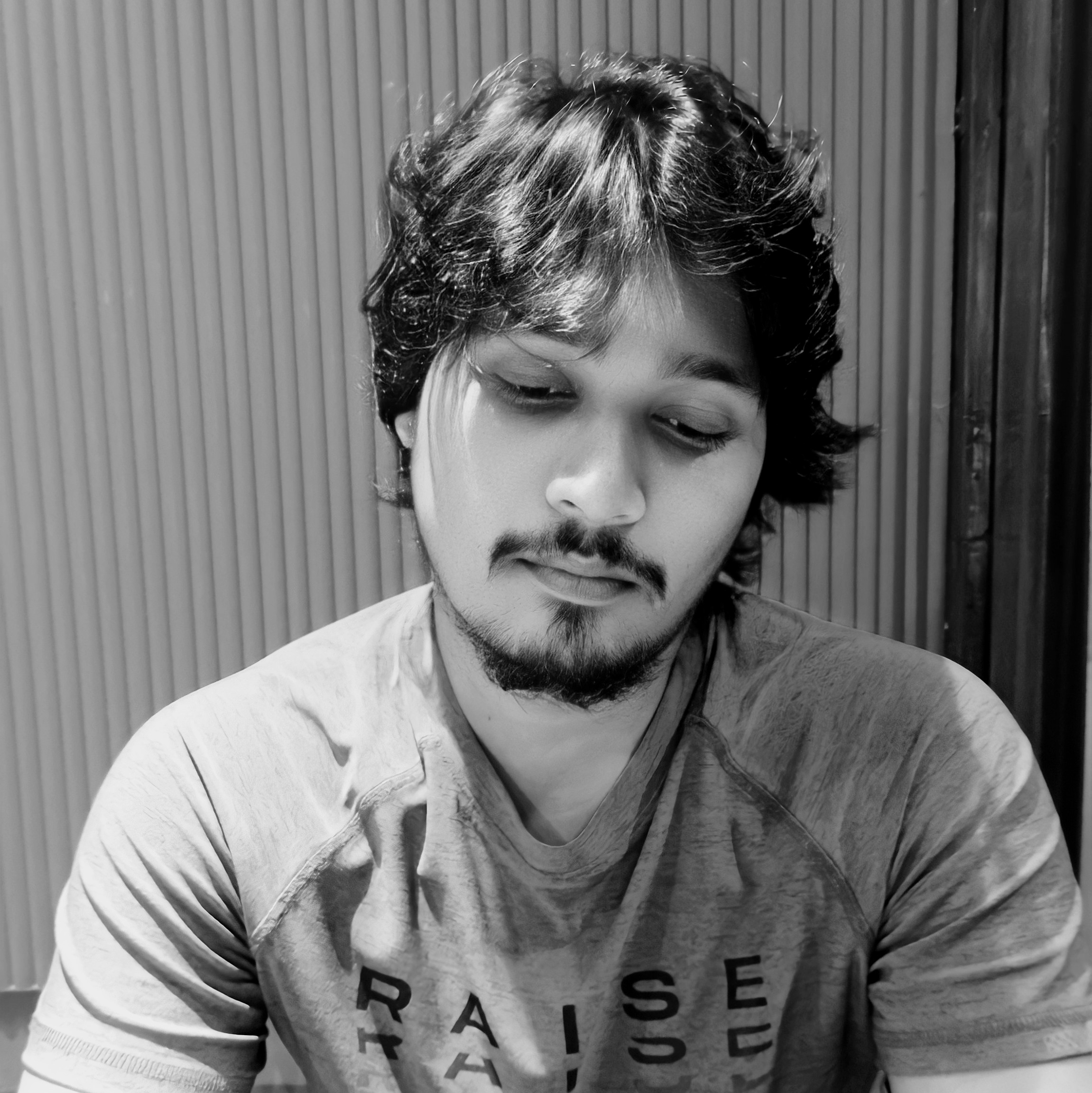