100 Days of SwiftUI - Day 1

Table of contents
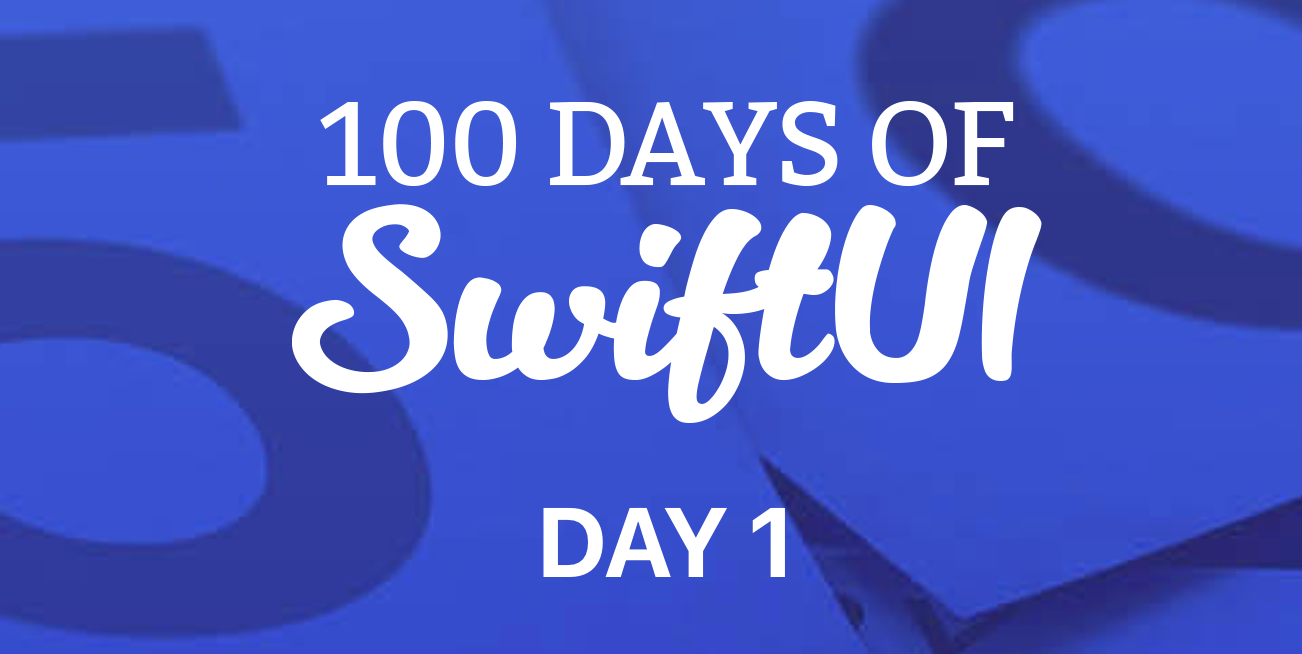
First post in my #100DaysOfSwiftUI journey. Here’s some stuff I learned (at https://www.hackingwithswift.com/100/swiftui/1):
⸻
Setting Up a Playground & Variables vs Constants
First, I learned how to set up a Playground in Xcode, which is a great way to experiment with code. I got comfortable with the basics of variables (var)
and constants (let)
.
Using constants (let)
instead of variables (var)
whenever possible helps Swift optimize the code since the value never changes. It also prevents accidental modifications, reducing the chance of bugs.
let name = "Swift"
var age = 25
⸻
Working with Strings
I got familiar with strings and some useful methods like print()
and uppercased()
. These are great for output and debugging.
print("hello".uppercased())
// Prints "HELLO"
Additionally, I learned about the method hasPrefix()
, which checks if a string starts with a certain substring.
let name = "SwiftUI"
print(name.hasPrefix("Swift"))
// Prints true
⸻
CamelCase Naming Convention
I also encountered the camelCase naming convention, which is used widely in Swift. For example, variables like dogNameLength
follow this format, where the first word is lowercase, and subsequent words start with uppercase letters. It’s a key part of maintaining readability and consistency in code.
⸻
Importing Cocoa
I learned about the import Cocoa statement, which is necessary when working with macOS apps, as it provides access to key libraries for app development.
import Cocoa
⸻
- The Difference Between Swift and SwiftUI
A crucial distinction I learned is the difference between Swift and SwiftUI. Swift is the programming language itself, while SwiftUI is a framework used to build user interfaces in a declarative manner.
⸻
Integers and Operations
I explored integers (whole numbers) and their operations.
100_000_000
is equivalent to 100,000,000, but the underscores are there to help with readability.
You can define integers as constants or variables.
let reallyBigNumber = 100_000_000
var score = 10
Operations like addition, subtraction, multiplication, and division can be performed using shorthand:
score += 5 // equivalent to score = score + 5
score -= 2 // equivalent to score = score - 2
score *= 3 // equivalent to score = score * 3
score /= 2 // equivalent to score = score / 2
print(score) // outputs the result
⸻
Multiples Check
I also played around with checking whether numbers are multiples of others using isMultiple(of:)
.
print(120.isMultiple(of: 3)) // prints true
This prints true because 120 is divisible by 3 without a remainder.
⸻
Multi-line vs Single-line Strings
I learned about multi-line strings in Swift, which are helpful for readability when working with long text. A multi-line string spans multiple lines in the code, making it easier to format.
let multiLineString = """
This is a multi-line
string that spans
multiple lines.
"""
However, single-line strings are more practical for things like error messages. When debugging, it’s easier to search for error messages if they are contained within a single line rather than broken up across multiple lines.
⸻
Double-Precision Floating-Point Numbers
The concept of double-precision floating-point numbers was introduced. “Double-precision” refers to the use of 64 bits instead of the typical 32 bits (single-precision) for storing real numbers. This allows for greater accuracy when working with floating-point numbers.
⸻
The “Floating-Point” Representation
A floating-point number allows the decimal point (or binary point) to “float” depending on the value. This makes it possible to represent very large or very small numbers, but it also introduces slight precision errors due to its limited binary representation.
⸻
Data Types and Type Safety
Swift is a type-safe language, which means once you define a variable or constant with a certain type, you can’t mix it with another type. For instance, if you try to add an Int
and a Double
, you will get an error.
let a = 1
let b = 2.0
let c = a + b // Error: Cannot mix types
To fix this, you can either convert b to an Int using:
let c = a + Int(b)
Or convert a to a Double using:
let c = Double(a) + b
Subscribe to my newsletter
Read articles from Matt B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
