Goodbye Boilerplate: Meet DataHandler for React

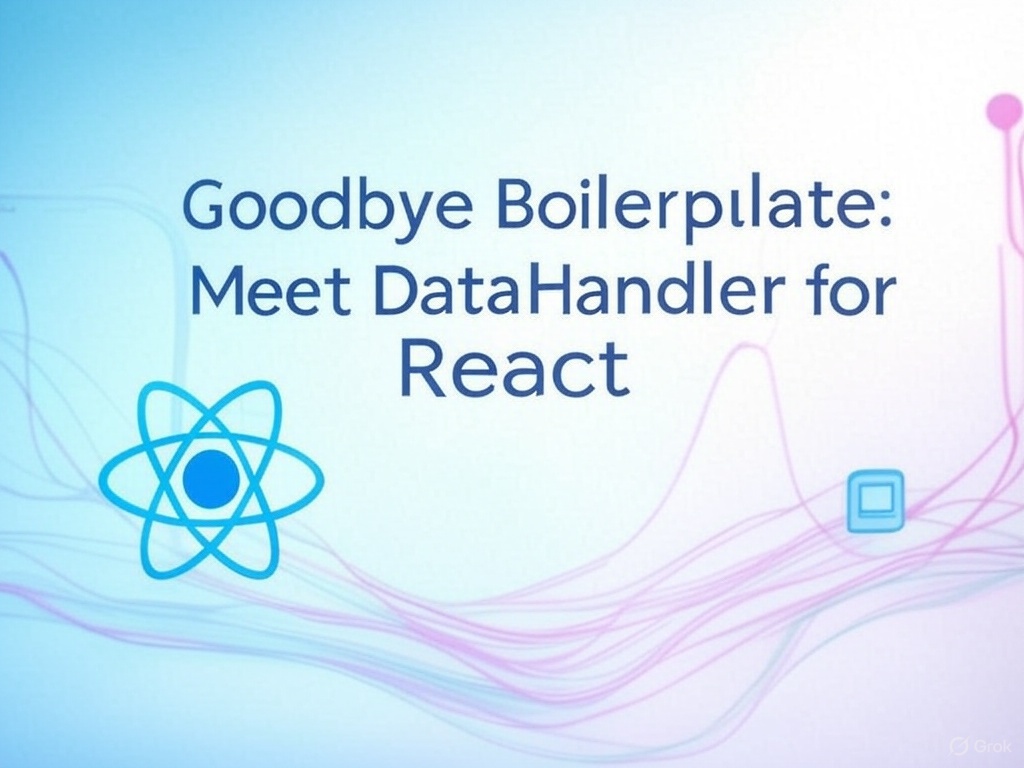
When building React applications, fetching and displaying data is a core task. However, it often comes with repetitive boilerplate: handling loading states, catching errors, and showing "no data" messages. If you’ve ever found yourself wrapping components in layers of suspense, error boundaries, and empty-state checks, you’re not alone. In this post, I’ll introduce DataHandler—a reusable React component that consolidates these concerns into a single, type-safe solution. Let’s explore why it’s useful, how it works, and how you can use it in your projects.
The Problem: Repetitive Data-Fetching Boilerplate
Imagine you’re building a menu display feature. You fetch data from an API, and you need to:
Show a loading skeleton while the data loads.
Display an error message if the fetch fails.
Render a "no items found" UI if the data is empty.
Catch runtime errors if something crashes during rendering.
Here’s a typical implementation:
tsx
const MenuFeature = ({ limit = "2", searchQuery }) => {
const { data: { data: menus } = {}, isLoading, isFetching, isError } = useGetAllMenusQuery(/* ... */);
return (
<CustomErrorBoundary error={isError}>
<CustomSuspense isLoading={isLoading || isFetching} fallback={<MenuSkeleton />}>
<NoItemFound data={menus} message="No menus found">
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-3 xl:grid-cols-4 gap-4">
{menus?.map((food) => <MenuCard key={food.id} menu={food} />)}
</div>
</NoItemFound>
</CustomSuspense>
</CustomErrorBoundary>
);
};
This works, but it’s verbose. Every time you fetch data, you repeat this nesting pattern. It’s prone to inconsistency, hard to maintain, and doesn’t catch runtime errors (e.g., if MenuCard throws an exception). What if we could simplify this into a single component?
Introducing DataHandler
DataHandler is a generic, class-based React component that:
Handles loading states with a customizable fallback.
Manages both explicit errors (e.g., API failures) and runtime errors (via an error boundary).
Displays a no-data UI when data is empty, with flexible validation.
Reduces boilerplate by combining these concerns into one reusable abstraction.
Here’s the basic idea:
tsx
<DataHandler<TMenu[]>
data={menus}
isLoading={isLoading || isFetching}
isError={isError}
noDataMessage="No menus found"
>
{(menus) => (
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-3 xl:grid-cols-4 gap-4">
{menus.map((food) => <MenuCard key={food.id} menu={food} />)}
</div>
)}
</DataHandler>
No nesting, no repetition—just pass your data and states, and let DataHandler do the rest.
Implementation Details
Let’s break down how DataHandler works. Here’s the full code with key features highlighted:
tsx
import { Component, ReactNode } from 'react';
import { Skeleton } from '@/components/ui/skeleton';
import { SearchIcon } from '@/assets/icons/Icons';
type DataHandlerProps<T> = {
data: T | undefined | null;
isLoading: boolean;
isError: boolean;
children: (data: T) => ReactNode;
loadingFallback?: ReactNode;
errorFallback?: ReactNode;
noDataFallback?: ReactNode;
loadingMessage?: string;
errorMessage?: string;
noDataMessage?: string;
hasData?: (data: T | null | undefined) => data is T;
onRetry?: () => void;
onError?: (error: Error, info: { componentStack: string }) => void;
};
interface State {
hasRuntimeError: boolean;
runtimeError: Error | null;
}
/**
* DataHandler: A unified component for handling data-fetching states.
* @template T - The type of the data being handled.
* @example
* // Basic usage
* <DataHandler<Item[]>
* data={data}
* isLoading={isLoading}
* isError={isError}
* noDataMessage="No items available"
* >
* {(items) => <ul>{items.map(item => <li key={item.id}>{item.name}</li>)}</ul>}
* </DataHandler>
*/
export class DataHandler<T> extends Component<DataHandlerProps<T>, State> {
state: State = {
hasRuntimeError: false,
runtimeError: null,
};
static getDerivedStateFromError(error: Error) {
return { hasRuntimeError: true, runtimeError: error };
}
componentDidCatch(error: Error, info: { componentStack: string }) {
this.props.onError?.(error, info);
}
resetError = () => {
this.setState({ hasRuntimeError: false, runtimeError: null });
};
render() {
const {
data,
isLoading,
isError,
children,
loadingFallback,
errorFallback,
noDataFallback,
loadingMessage = 'Loading...',
errorMessage = 'An unexpected error occurred',
noDataMessage = 'No items found',
hasData = (d: T | null | undefined): d is T => (Array.isArray(d) ? d.length > 0 : !!d),
onRetry,
} = this.props;
const { hasRuntimeError, runtimeError } = this.state;
if (hasRuntimeError) {
return errorFallback ?? (
<ErrorFallback message={runtimeError?.message || errorMessage} onRetry={this.resetError} />
);
}
if (isError) {
return errorFallback ?? (
<ErrorFallback message={errorMessage} onRetry={onRetry} />
);
}
if (isLoading) {
return loadingFallback ?? <LoadingFallback message={loadingMessage} />;
}
if (!hasData(data)) {
return noDataFallback ?? <NoDataFallback message={noDataMessage} onRetry={onRetry} />;
}
return <>{children(data)}</>;
}
}
// Default Fallbacks (omitted for brevity, see full code above)
const LoadingFallback = /* ... */;
const ErrorFallback = /* ... */;
const NoDataFallback = /* ... */;
Key Features
Type Safety:
- Uses TypeScript generics (T) and a type guard (hasData) to ensure data is safely narrowed to T before passing it to children.
Error Boundary:
- Implements componentDidCatch and getDerivedStateFromError to catch runtime errors, with a resetError method for recovery.
State Priority:
- Checks states in order: runtime errors > explicit errors > loading > no data > success.
Customization:
- Supports custom fallbacks, messages, and validation logic via props.
Why Use DataHandler?
Less Boilerplate: Replaces multiple nested components with one.
Consistency: Ensures uniform handling of data states across your app.
Robustness: Catches both API errors (isError) and runtime crashes.
Flexibility: Customize fallbacks and validation to fit your needs.
Usage Examples
1. Basic Usage
Display a list of items with default fallbacks:
tsx
type Item = { id: string; name: string };
const ItemList = () => {
const { data, isLoading, isError } = useQuery('items', fetchItems);
return (
<DataHandler<Item[]>
data={data}
isLoading={isLoading}
isError={isError}
noDataMessage="No items available"
>
{(items) => (
<ul>
{items.map((item) => <li key={item.id}>{item.name}</li>)}
</ul>
)}
</DataHandler>
);
};
2. Custom Fallbacks
Override the default UI:
tsx
const CustomLoading = () => <div>Loading...</div>;
const CustomError = ({ retry }) => (
<div>
<p>Oops!</p>
<button onClick={retry}>Retry</button>
</div>
);
const ItemListWithCustomUI = () => {
const { data, isLoading, isError } = useQuery('items', fetchItems);
return (
<DataHandler<Item[]>
data={data}
isLoading={isLoading}
isError={isError}
loadingFallback={<CustomLoading />}
errorFallback={<CustomError retry={() => console.log('Retrying')} />}
>
{(items) => (
<ul>
{items.map((item) => <li key={item.id}>{item.name}</li>)}
</ul>
)}
</DataHandler>
);
};
3. Custom Data Validation
Filter active users:
tsx
type User = { id: string; name: string; isActive: boolean };
const ActiveUserList = () => {
const { data, isLoading, isError } = useQuery('users', fetchUsers);
return (
<DataHandler<User[]>
data={data}
isLoading={isLoading}
isError={isError}
hasData={(users): users is User[] => !!users && users.some(user => user.isActive)}
noDataMessage="No active users found"
>
{(users) => (
<ul>
{users.filter(user => user.isActive).map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)}
</DataHandler>
);
};
4. Runtime Error Handling
Log crashes:
tsx
const ItemListWithErrorLogging = () => {
const { data, isLoading, isError } = useQuery('items', fetchItems);
return (
<DataHandler<Item[]>
data={data}
isLoading={isLoading}
isError={isError}
onError={(error, info) => console.error('Crash:', error, info)}
>
{(items) => {
if (Math.random() > 0.5) throw new Error('Random crash!');
return (
<ul>
{items.map((item) => <li key={item.id}>{item.name}</li>)}
</ul>
);
}}
</DataHandler>
);
};
Conclusion
DataHandler is a game-changer for React developers tired of writing the same data-fetching boilerplate. By combining loading states, error handling, and no-data checks into one component, it saves time, improves consistency, and handles edge cases like runtime errors. Whether you’re building a simple list or a complex dashboard, DataHandler adapts to your needs with minimal fuss.
Try it in your next project—copy the code, tweak the fallbacks, and see how it simplifies your workflow. Have questions or improvements? Share them in the comments!
Subscribe to my newsletter
Read articles from Forhad Hossain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Forhad Hossain
Forhad Hossain
Hi, I'm Farhad Hossain, a passionate web developer on a journey to build impactful software and solve real-world problems. I share coding tips, tutorials, and my growth experiences to inspire others.