Closures in JavaScript
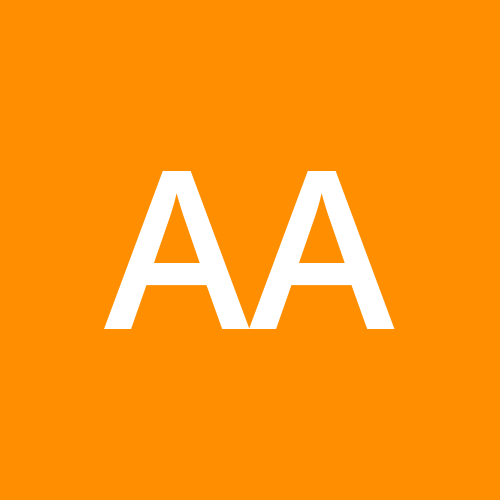
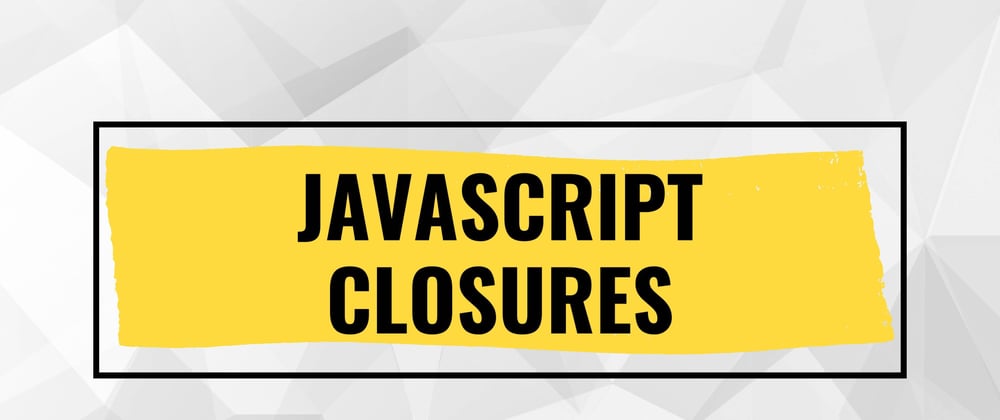
What is Closure?
A closure in JavaScript is a function that remembers its lexical scope (the environment where it was created) even after the function is executed and that scope is no longer active.
You can say it’s like a backpack - Here this function carries the variable it needs even when it’s used later.
In Simple Terms:
When a function is defined inside another function, the inner function has access to:
Its own variables
Variables of the outer function
Global variables
Closures allow you to remember and access variables even when the outer function has finished execution.
Example of a closure:
function counter() {
let count = 0; // Private variable
return function () {
count++;
console.log(`Count: ${count}`);
};
}
const increment = counter();
increment(); // Count: 1
increment(); // Count: 2
Key Properties of Closures:
Access to Outer Scope: Inner functions can access variables from their outer functions.
Persistent Data: Values are stored even after the outer function completes.
Encapsulation: Useful for hiding data (private variables).
State Management: Maintain state between function calls (e.g., counters).
Subscribe to my newsletter
Read articles from allwin ajith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
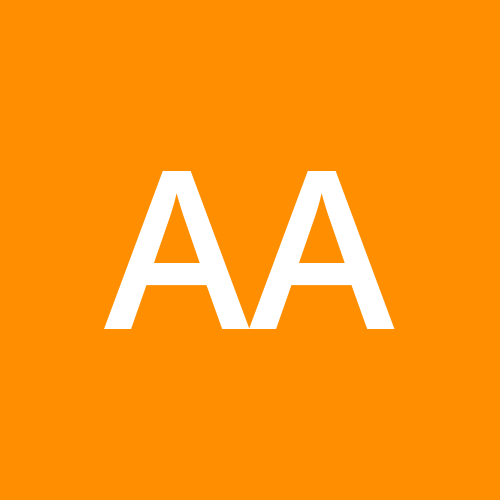