๐ How I Built a Fully Responsive Dashboard in Angular Using PrimeNG and Gridster
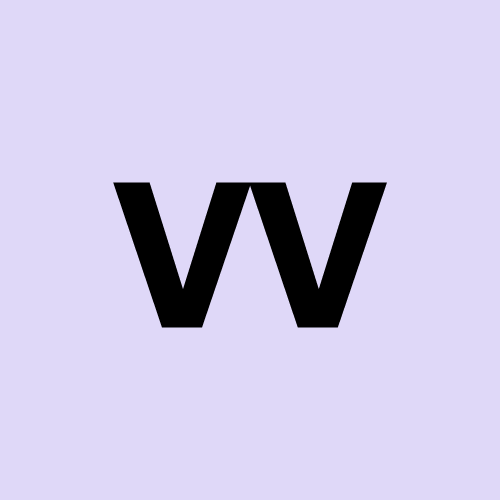
2 min read
๐ฅ Introduction
In this blog, I'll show you how I built a fully responsive, drag-and-drop dashboard in Angular using PrimeNG, Gridster, and Dragula.
If you're an Angular developer struggling with UI components and responsiveness, this guide is for you.
โ Step 1: Setting Up Angular + PrimeNG + Gridster
Install Angular Project
ng new angular-dashboard
cd angular-dashboard
Install PrimeNG + Gridster + Dragula
npm install primeng gridster2 ng2-dragula
Import Required Modules
In app.module.ts
:
import { GridsterModule } from 'angular-gridster2';
import { DragulaModule } from 'ng2-dragula';
import { DropdownModule } from 'primeng/dropdown';
โ Step 2: Gridster Configuration
In dashboard.component.html
:
<gridster [options]="gridOptions">
<gridster-item *ngFor="let item of dashboardItems" [item]="item">
<div class="widget">{{ item.name }}</div>
</gridster-item>
</gridster>
In dashboard.component.ts
:
import { GridsterConfig, GridsterItem } from 'angular-gridster2';
this.gridOptions = {
draggable: { enabled: true },
resizable: { enabled: true },
gridType: 'fit',
};
this.dashboardItems = [
{ cols: 2, rows: 1, y: 0, x: 0, name: 'Widget 1' },
{ cols: 1, rows: 1, y: 0, x: 2, name: 'Widget 2' }
];
โ Step 3: Drag-and-Drop with Dragula
In app.module.ts
:
import { DragulaModule } from 'ng2-dragula';
In HTML:
<div class="drag-container" dragula="DRAG_ITEMS">
<div *ngFor="let item of dragItems">{{ item }}</div>
</div>
In Component:
this.dragItems = ['Item 1', 'Item 2', 'Item 3'];
โ Step 4: Making it Fully Responsive
In styles.css
:
.gridster .widget {
background-color: #007bff;
color: white;
padding: 10px;
text-align: center;
border-radius: 8px;
}
@media (max-width: 768px) {
.gridster .widget {
font-size: 12px;
}
}
โ Step 5: Deploying on Vercel
npm run build
vercel
๐ฏ Conclusion
By combining PrimeNG for UI components, Gridster for layout, and Dragula for drag-and-drop, we can create a fully responsive Angular dashboard.
If you found this helpful, share it with your dev friends! ๐
0
Subscribe to my newsletter
Read articles from Agni Templates directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
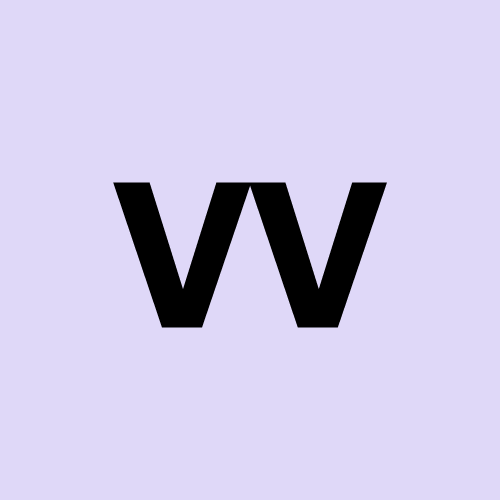