๐ 7 Advanced Angular Tricks Only Experienced Developers Know (You Won't Find These in Docs)
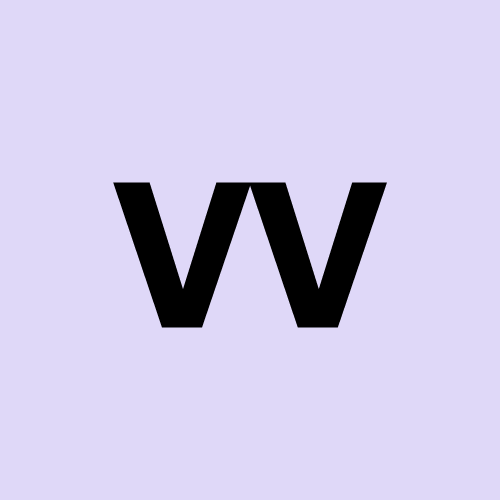
๐ฏ Introduction
As an Angular developer, you probably know the basics. But real pros know these advanced tricks that can boost your app's performance and make your code cleaner.
โ 1. Change Detection Optimization
Angular's default change detection can slow down your app. Use ChangeDetectionStrategy.OnPush
to optimize performance.
@Component({
selector: 'app-my-component',
changeDetection: ChangeDetectionStrategy.OnPush
})
๐ฅ This ensures Angular only checks for changes when the input reference changes, not on every event.
โ 2. Custom Directives in PrimeNG
You can create custom directives to modify PrimeNG components.
@Directive({ selector: '[customStyle]' })
export class CustomStyleDirective {
constructor(private el: ElementRef) {
this.el.nativeElement.style.backgroundColor = 'lightblue';
}
}
โ 3. RxJS Debou/cnce for API Calls
To prevent multiple API hits when users type in a search bar:
this.searchInput.pipe(
debounceTime(300),
distinctUntilChanged(),
switchMap(value => this.apiService.search(value))
).subscribe();
โ 4. Lazy Loading with Angular Routes
Improve loading time by lazy loading modules.
In app-routing.module.ts
:
{ path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) }
โ 5. Using Angular Signals (New Feature)
Angular Signals are the future of state management.
import { signal, computed } from '@angular/core';
const count = signal(0);
const doubleCount = computed(() => count() * 2);
โ 6. Angular State Management with NgRx
Manage complex state using NgRx Store.
store.dispatch(increment());
store.select(selectCount).subscribe(count => console.log(count));
โ 7. Building a Reusable Component Library
Create your own UI library for SaaS products.
ng generate library my-component-library
๐ฏ Conclusion
These 7 advanced Angular tricks will help you build faster, cleaner, and more scalable apps.
๐ฅ Which one are you using next? Comment below
๐ Follow me for more Angular content!
Subscribe to my newsletter
Read articles from Agni Templates directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
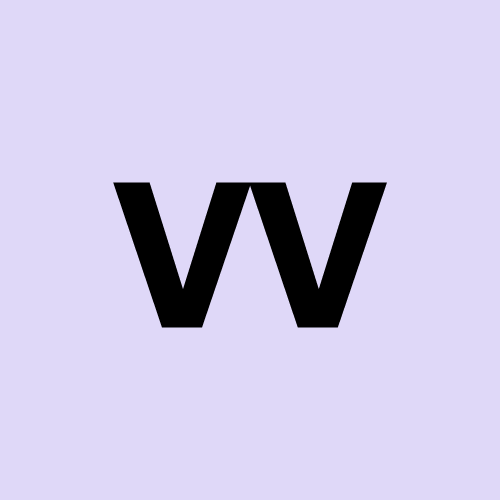