JMeter Load Testing

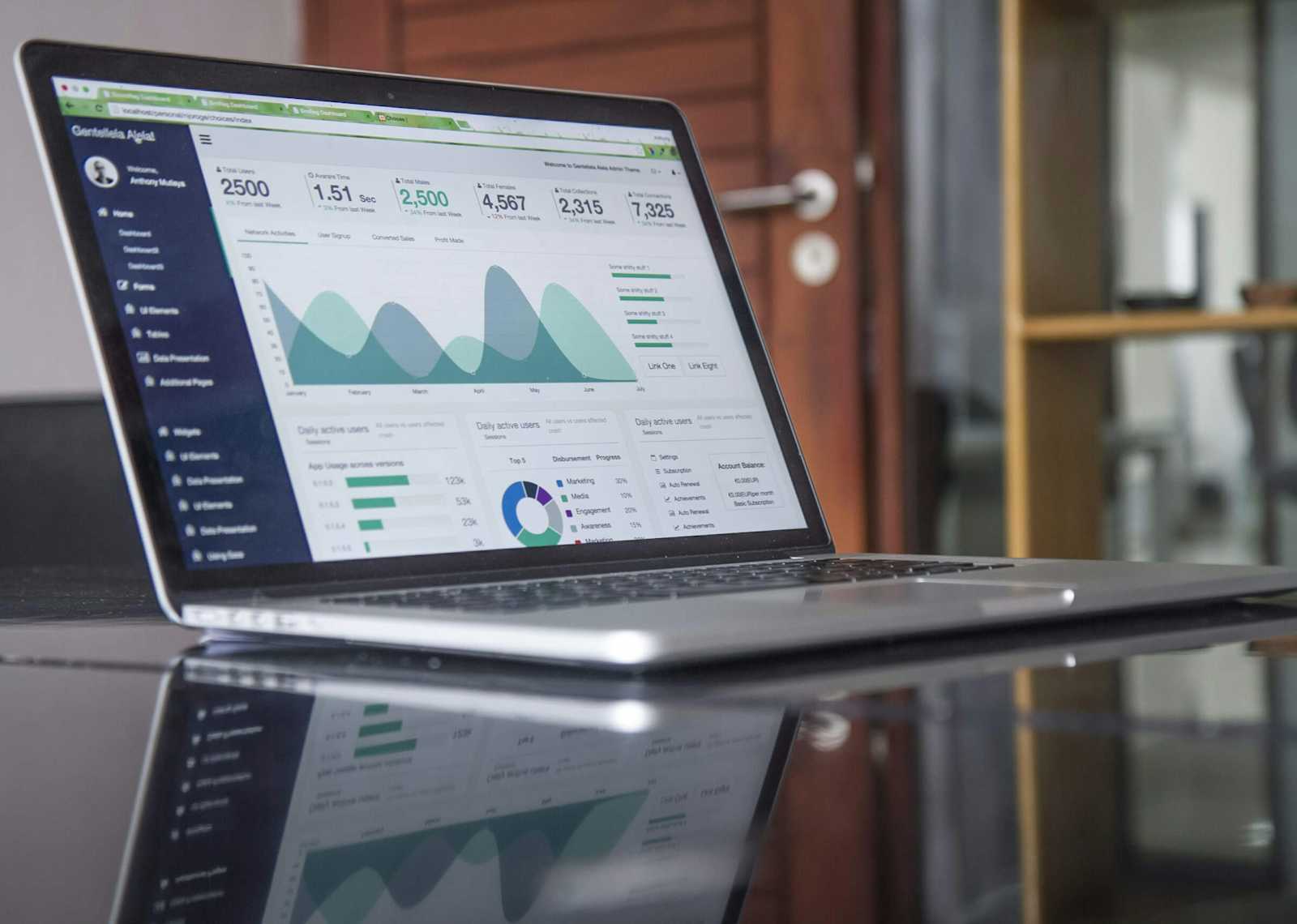
Understanding JMeter
JMeter is an open-source load testing tool that simulates user traffic to analyze application performance. It works by sending requests, measuring response times, and identifying performance bottlenecks.
JMeter Script Architecture:
Test Plan
├── Thread Group
│ ├── Number of Threads (Users)
│ ├── Ramp-Up Period
│ ├── Loop Count
│ ├── Config Elements
│ │ ├── CSV Data Set Config
│ │ ├── HTTP Header Manager
│ ├── Pre-Processors
│ │ ├── Regular Expression Extractor
│ │ ├── JSON Extractor
│ ├── Timers
│ │ ├── Constant Timer
│ │ ├── Uniform Random Timer
│ ├── Samplers
│ │ ├── HTTP Request
│ │ ├── JDBC Request
│ ├── Post-Processors
│ │ ├── BeanShell PostProcessor
│ │ ├── JSON Path Extractor
│ ├── Assertions
│ │ ├── Response Assertion
│ │ ├── Duration Assertion
│ ├── Listeners
│ │ ├── View Results Tree
│ │ ├── Aggregate Report
│ │ ├── Simple Data Writer
Test Plan in JMeter
A Test Plan is the top-most element in a JMeter script, acting as a container for all test components. It defines the overall structure, execution flow, and configurations for a performance test.
Key Features of a Test Plan:
Holds all test elements – Thread Groups, Samplers, Listeners, etc.
Defines User Variables – Reusable variables across the test.
Configures Test Execution – Manage test-level settings like functional mode or user-defined properties.
Controls File Encoding & Data – Allows specifying CSV input files for dynamic data injection.
Non-Test Elements – Includes elements like HTTP Cache Manager or Cookie Manager.
Execution Flow:
sqlCopyEditTest Plan
→ Thread Groups
→ Config Elements
→ Timers
→ Samplers
→ Post-Processors
→ Assertions
→ Listeners
Each component inside a test plan executes in order, ensuring an organized and systematic approach to load testing.
1. Thread Group (Users & Requests)
A Thread Group is the foundation of any JMeter test plan. It defines the number of virtual users (threads) and how they interact with the application under test.
Key Features of a Thread Group:
Number of Threads (Users) – Specifies the number of concurrent users.
Ramp-Up Period – Time taken to start all users gradually.
Loop Count – Number of times each user repeats the test scenario.
Scheduler (Optional) – Defines start and end times for the test.
Thread Behavior – Customizable using logic controllers and timers.Defines the number of virtual users and test execution flow.
Example: Simulating 500 users hitting an API over 1 minute.
2. Samplers (Request Generators)
Generate different types of requests:
HTTP Request → API Testing
JDBC Request → Database Load Testing
FTP Request → File Transfer Testing
Example: Sending an HTTP POST request with JSON payload.
3. Listeners (Result Collection & Analysis)
Capture and visualize test results.
Common Listeners:
View Results Tree → Detailed response logs
Summary Report → Aggregate metrics
Aggregate Graph → Graphical performance representation
Simple Data Writer
Simple Data Writer is a JMeter listener that logs test results in a specified file format. It is useful for capturing raw test data efficiently. However, it does not provide visual analysis, making it ideal for automated processing.
Key Features:
Logs data in CSV or XML format.
Minimal performance impact compared to other listeners.
Can be integrated with external reporting tools for further analysis.Example: Displaying response times and error rates in a table.
4. Timers (Request Delay Control)
Simulate realistic user behavior by adding delays.
Common Timers:
Constant Timer → Fixed delay between requests
Gaussian Random Timer → Variable delay within a range
Example: Adding 2 seconds between login and dashboard requests.
5. Assertions (Response Validation)
Ensure correct API responses.
Common Assertions:
Response Assertion → Validate status code/content
Duration Assertion → Set response time limits
Example: Ensuring API response contains "success".
6. Config Elements (Test Parameterization)
Store reusable configurations.
Common Config Elements:
CSV Data Set Config → Parameterize with dynamic data
HTTP Header Manager → Set authentication headers
User Defined Variable
Example: Using CSV files to send multiple usernames/passwords.
7. Pre/Post-Processors (Dynamic Request Handling)
Modify requests before or after execution.
Common Processors:
Regular Expression Extractor → Capture dynamic values
JSR223 Pre/Post-Processor → Custom scripting
Example: Extracting session tokens from API responses.
Key JMeter Performance Metrics (With Examples)
Metric | Definition | Example |
Response Time | Time taken from request initiation to full response. | API response time = 1.5s |
Latency | Time to receive the first byte from the server. | Latency = 500ms |
Throughput | Number of requests handled per second/minute. | 1000 requests/min |
Error Rate | Percentage of failed requests. | 5% errors in 200 requests |
Hits per Second | Number of HTTP calls per second. | 500 hits/sec during spike |
Performance Metric Flow (Visual Representation)
[Request Sent]
--> [Latency]
--> [Processing]
--> [Response Time]
--> [Throughput & Error Rate]
Load Testing Strategies in JMeter
1. Load Testing
Simulate expected traffic to evaluate performance.
Example: 1000 users accessing an e-commerce website.
2. Stress Testing
Push system beyond capacity to test failure points.
Example: Testing a payment gateway with 5000 concurrent users.
3. Spike Testing
Sudden increase in load to check stability.
Example: Simulating Black Friday shopping traffic surge.
4. Endurance Testing
Long-duration load testing for stability assessment.
Example: Running tests for 24 hours to detect memory leaks.
JMeter Interview Questions & Answers
1. What is JMeter and what is it used for?
- JMeter is an open-source performance testing tool used to test web applications, APIs, databases, and other services by simulating multiple users and measuring system performance.
2. What are the core components of a JMeter Test Plan?
- Test Plan, Thread Group, Samplers, Config Elements, Timers, Assertions, Pre-Processors, Post-Processors, and Listeners.
3. What is a Thread Group in JMeter?
- A Thread Group defines the number of virtual users (threads), ramp-up time, and iteration count for executing test scripts.
4. What is a Sampler in JMeter?
- A Sampler generates different types of requests (e.g., HTTP, FTP, JDBC, TCP) to test the performance of services.
5. What are Timers in JMeter, and why are they used?
- Timers introduce delays between requests to simulate real-world scenarios. Examples include Constant Timer, Gaussian Random Timer, and Uniform Random Timer.
6. What are Assertions in JMeter?
Assertions validate response correctness. Examples:
Response Assertion (checks response content)
Duration Assertion (ensures response time is within a limit)
JSON Assertion (validates JSON structure)
7. What is the execution order of components in JMeter?
- Test Plan → Thread Group → Config Elements → Pre-Processors → Timers → Samplers → Post-Processors → Assertions → Listeners
8. What is Response Time vs. Latency in JMeter?
Response Time: Time from request initiation to full response.
Latency: Time from request initiation to receiving the first byte of response.
9. What are Listeners in JMeter?
Listeners collect and display test results. Examples:
View Results Tree (detailed request-response)
Summary Report (aggregated performance metrics)
Aggregate Report (throughput, error %, response time)
10. What is Correlation in JMeter, and how do you handle it?
- Correlation is extracting dynamic values (e.g., session IDs) from responses and using them in subsequent requests using Regular Expression Extractor or JSON Extractor.
11. What is Throughput in JMeter?
- Throughput is the number of requests processed per second or minute. It helps measure system performance under load.
12. How do you analyze performance results in JMeter?
- Using Aggregate Report, Summary Report, and Graph Results, or by exporting logs to external tools like Grafana or Taurus.
13. What is the Simple Data Writer in JMeter?
- A Listener that logs test results to a file (CSV/XML) with minimal performance impact, useful for automated analysis.
14. How does Distributed Load Testing work in JMeter?
- Multiple JMeter instances (slaves) generate load, controlled by a master instance using the
jmeter-server
mode.
15. What is the purpose of Config Elements in JMeter?
Config Elements set up request parameters or shared test settings. Examples:
HTTP Header Manager (adds headers)
CSV Data Set Config (parameterizes test data)
16. How can JMeter integrate with CI/CD pipelines?
- JMeter tests can be automated using Taurus, Jenkins, or GitHub Actions, generating reports for continuous performance testing.
17. What is the Taurus Framework, and why is it used with JMeter?
- Taurus simplifies JMeter execution using YAML files, provides real-time reporting, and integrates with CI/CD for automated performance testing.
18. What is Ramp-Up Time in JMeter?
- Ramp-Up Time defines how long JMeter takes to start all virtual users. A Ramp-Up of 10 seconds for 100 threads means 10 users start every second.
19. How can you parameterize test data in JMeter?
- Using CSV Data Set Config to read values from a file dynamically and use them in requests.
20. What is a Transaction Controller in JMeter?
- It groups multiple requests as a single transaction, allowing measurement of response time for an entire workflow (e.g., login → search → logout).
Mind Map Summary
[TEST PLAN}
[Thread Group] --> [Users, Ramp-up, Iterations]
[Samplers] --> [HTTP, JDBC, FTP Requests]
[Listeners] --> [Results, Logs, Graphs]
[Timers] --> [Delays, User Simulation]
[Assertions] --> [Response Validation]
[Performance Metrics] --> [Response Time, Latency, Throughput, Errors]
[Testing Types] --> [Load, Stress, Spike, Endurance]
[Interview Focus] --> [Correlation, Assertions, Result Analysis, Parameterization]
Subscribe to my newsletter
Read articles from Amit Sangwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amit Sangwan
Amit Sangwan
💼 Automation Engineer | AI Enthusiast | Tech Blogger Passionate about automation, AI agents, and testing. Exploring innovations in QA while sharing insights on technology and career growth. Always learning, always evolving. 🚀