Unlocking Performance & The Advantages of Low Level Programming in C# .NET

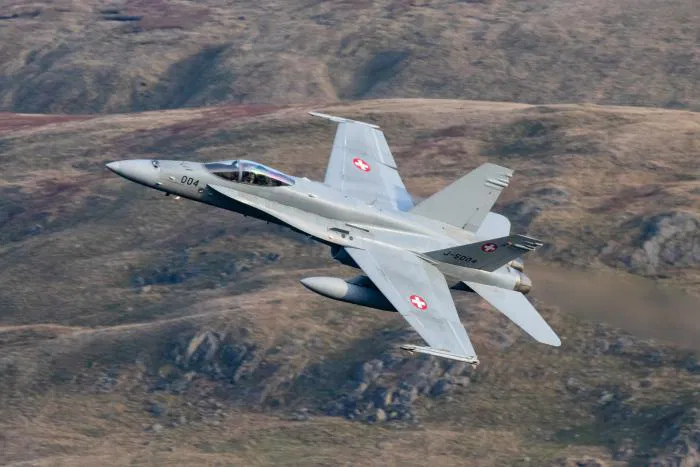
When working with C# and the .NET platform, we often default to leveraging the high level abstractions and convenient frameworks provided by Microsoft. While these frameworks are excellent for rapid development and ease of use, there are significant advantages to stepping down into lower level programming techniques. By understanding and adopting low level coding practices, we can significantly enhance application performance, achieve greater resource efficiency, and increase control over how applications interact with system hardware.
Performance is a primary advantage of low level coding in C#. Managed code in .NET generally includes features like garbage collection and bounds checking, which simplify development but can introduce overhead. Employing lower level features allows us to perform efficient, allocation free memory manipulation. This avoids unnecessary heap allocations, reducing garbage collection overhead and increasing execution speed, especially beneficial for real time or high frequency data processing tasks. Low level programming allows us to directly control memory through the use of pointers and unsafe code blocks, a valuable asset in scenarios demanding precision and speed. Although pointers introduce complexity, they enable us to bypass certain runtime checks that would otherwise introduce latency, making this approach exceptionally suited for performance critical applications like real time data processing or graphics rendering.
Low level techniques further empower us by enhancing interoperability with native code through Platform Invocation Services (P/Invoke). P/Invoke allows managed code to directly call native libraries, crucial in scenarios such as legacy integration, hardware communication, or performance intensive tasks. This direct native interaction removes performance overhead typically encountered when using wrapper libraries, offering us a powerful tool for optimisation. Adopting low level programming practices can also bring greater predictability and consistency in application behaviour. Managed code abstractions sometimes introduce latency due to operations like garbage collection pauses or runtime checks. Low level coding, such as direct memory management using stack allocations and pointers, provides deterministic performance. Consider high frequency trading applications, where latency directly translates to competitive advantage; in such scenarios, employing unmanaged memory allocations can noticeably reduce latency and increase application responsiveness.
While powerful, we must exercise caution when applying these techniques, as improper use may introduce stability or security risks. Errors such as memory leaks, buffer overruns, or security vulnerabilities require careful coding practices and thorough testing. We should focus low level techniques exclusively on critical performance paths, ensuring balance between safety, maintainability, and performance.
High Level vs. Low Level Examples
Here are five practical examples comparing high level framework code with low level implementations in C# .NET, highlighting the performance and control benefits achieved by using low level techniques.
Example 1: Array Manipulation
High Level Version:
int[] numbers = Enumerable.Range(1, 1000).ToArray();
int sum = numbers.Sum();
Low Level Version:
unsafe int SumArray(int[] array)
{
int sum = 0;
fixed (int* ptr = array)
{
for (int i = 0; i < array.Length; i++)
{
sum += *(ptr + i);
}
}
return sum;
}
Benefit: The low level implementation avoids overhead from array bounds checking, resulting in faster execution for large datasets or performance critical loops.
Example 2: String Manipulation
High Level:
string result = string.Concat("Hello", " ", "World");
Low Level Version:
Span<char> buffer = stackalloc char[11];
"Hello".CopyTo(buffer);
buffer[5] = ' ';
"World".CopyTo(buffer.Slice(6));
string result = new string(buffer);
Using stack allocations (stackalloc
) with Span<T>
avoids unnecessary heap allocations, significantly reducing garbage collection overhead and improving execution speed.
Example 3: Copying Data Quickly
High Level Version:
Array.Copy(sourceArray, destinationArray, length);
Low Level Version:
unsafe void FastCopy(int[] source, int[] destination, int length)
{
fixed (int* src = source, dest = destination)
{
Buffer.MemoryCopy(src, destination, length * sizeof(int), length * sizeof(int));
}
}
Using pointers avoids bounds checking and method overhead, providing faster execution.
Example 4: Calling Native Libraries
High Level Version: (Framework wrapper methods)
using System.Diagnostics;
var process = Process.GetCurrentProcess();
IntPtr handle = process.Handle;
Low Level Version:
using System.Runtime.InteropServices;
class NativeMethods
{
[DllImport("kernel32.dll")]
private static extern IntPtr GetCurrentThread();
public static IntPtr GetThreadHandle()
{
return GetCurrentThread();
}
}
IntPtr threadHandle = NativeMethods.GetThreadHandle();
Direct native calls provide faster and more direct interaction with underlying operating system features.
Example 5: Struct Manipulation and Memory Efficiency
High Level Version:
var point = new Point(10, 20);
Low Level Version:
Span<Point> points = stackalloc Point[1];
points[0] = new Point(10, 20);
Using stackalloc
removes heap allocation, significantly reducing memory overhead.
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
