Unlocking Powerful Search Capabilities in Sitecore XM Cloud with Next.js

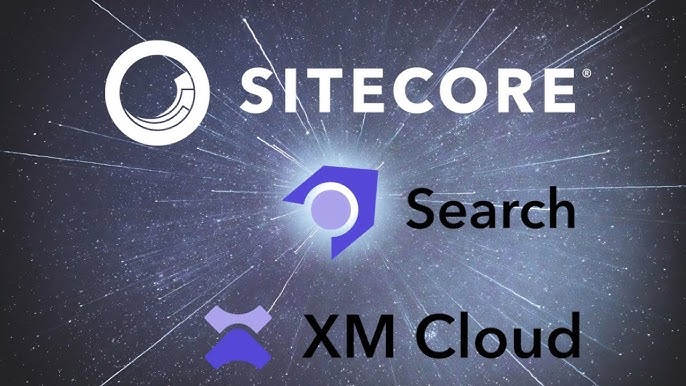
In today’s digital landscape, delivering a seamless and intuitive search experience is crucial for engaging users and driving conversions. Sitecore XM Cloud, combined with Next.js, offers a powerful platform for building modern, headless websites with robust search functionality. In this article, I’ll walk you through the steps to implement Sitecore Search in a Sitecore XM Cloud environment using Next.js, and explain why Sitecore Search is the best choice over alternatives like Coveo or SOLR.
Why Choose Sitecore Search Over Coveo or SOLR in XM Cloud?
While Coveo and SOLR are excellent search solutions, Sitecore Search offers unique advantages when used with Sitecore XM Cloud:
Native Integration: Sitecore Search is built specifically for Sitecore platforms, ensuring seamless integration with XM Cloud. This native compatibility reduces setup complexity and ensures optimal performance.
AI-Driven Personalization: Sitecore Search leverages AI and machine learning to deliver personalized search results based on user behavior, preferences, and context. This level of personalization is harder to achieve with generic solutions like SOLR.
Unified Platform: By using Sitecore Search, you stay within the Sitecore ecosystem, which simplifies maintenance, updates, and support. You don’t need to manage third-party integrations or deal with compatibility issues.
Scalability: Sitecore Search is designed to handle large-scale websites and high traffic volumes, making it ideal for enterprise-level implementations.
Why Use Sitecore Search SDKs Over RESTful APIs?
When implementing Sitecore Search in Next.js, you have two options: using RESTful APIs or leveraging the Sitecore Cloud SDKs. Here’s why the SDKs are a better choice:
Simplified Development: The Sitecore Search SDKs provide pre-built methods and utilities that abstract away the complexity of making raw API calls. This reduces boilerplate code and speeds up development.
Type Safety and IntelliSense: SDKs often come with TypeScript support, enabling better type safety and autocompletion in your IDE. This improves developer productivity and reduces errors.
Optimized Performance: SDKs are optimized for performance and include built-in features like caching, retries, and error handling, which you’d otherwise need to implement manually with RESTful APIs.
Future-Proofing: SDKs are maintained by Sitecore, ensuring compatibility with future updates and new features. With RESTful APIs, you may need to update your implementation manually when changes occur.
Steps to Implement
1. Set Up Sitecore Search in XM Cloud
Enable Sitecore Search in your XM Cloud dashboard.
Configure the search index and map content types.
Generate API keys and store them securely in .env.local.
2. Install Sitecore Cloud SDK
Add the SDK to your Next.js project:
npm install @sitecore-cloud/sdk
3. Initialize the SDK
Create a configuration file to initialize the SDK:
// lib/sitecoreClient.js
import { SitecoreClient } from '@sitecore-cloud/sdk';
const client = new SitecoreClient({
apiKey: process.env.SITECORE_SEARCH_API_KEY,
endpoint: process.env.SITECORE_SEARCH_ENDPOINT,
});
export default client;
4. Create a Search Component
Build a component to fetch and display search results:
// components/Search.js
import { useState } from 'react';
import client from '../lib/sitecoreClient';
const Search = () => {
const [query, setQuery] = useState('');
const [results, setResults] = useState([]);
const handleSearch = async () => {
const response = await client.search({ query, language: 'en', pageSize: 10 });
setResults(response.results);
};
return (
<div>
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
placeholder="Search..."
/>
<button onClick={handleSearch}>Search</button>
<ul>
{results.map((result, index) => (
<li key={index}>
<a href={result.url}>{result.title}</a>
</li>
))}
</ul>
</div>
);
};
export default Search;
5. Enhance the Search Experience
Debouncing: Delay search queries until the user stops typing.
Styling: Use CSS or Tailwind CSS to style the search UI.
Subscribe to my newsletter
Read articles from Aditya Gautam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
