How to Get Started with Zustand for State Management

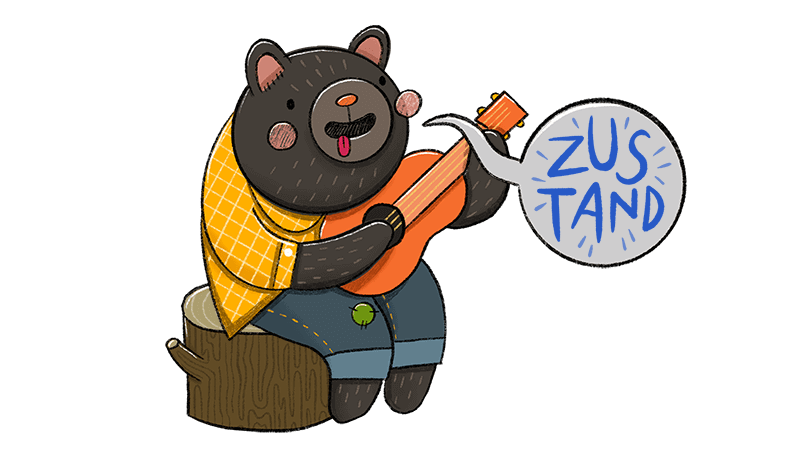
Are you a frontend developer struggling with state management in your React applications? You’re not alone! This blog is designed to help you navigate this complex but crucial aspect of web development.
Do you know what is state management ?
State management is the process of tracking, storing, and updating data throughout your application. When a user interacts with your website (clicks a button, submits a form, etc.), the state changes, and these changes are reflected in your UI.
Think of state management as the central nervous system of your application — it coordinates how data flows and how your components respond to changes.
The Challenge
While React provides built-in tools like useState
, useRef
, and useContext
for handling state, these solutions can quickly become unwieldy in larger applications. As your project grows, you might face challenges like:
Prop drilling across multiple component levels
Difficulty tracking where and how state changes occur
Inconsistent state updates leading to bugs
Performance bottlenecks with unnecessary re-renders
Solution
For complex applications, external state management libraries can bring order to chaos. These tools provide structured ways to organize, access, and update your application’s state. Some popular options include:
Zustand: Lightweight and straightforward with a minimal API
Redux: Powerful with a robust ecosystem, though with a steeper learning curve
Recoil: Facebook’s state management library designed specifically for React
Jotai: Atomic approach to state management
Hookstate: Performance-focused state management solution
In the following sections, we’ll explore how Zustand library can transform your development experience and help you build more maintainable React applications.
Today we will learn about Zustand, as a beginner.
Introduction :
What Is Zustand?
Zustand is a small, fast and scalable state-management solution for React applications. It abstracts state management away into a tiny API that has the right balance of flexibility and efficiency and importantly, cuts down on the boilerplate code. Zustand follows a simple Flux style (Flux is the application architecture Facebook uses to build JavaScript applications.) and uses hooks for state management in React. Unlike some other libraries, such as Redux, Zustand does not need you to wrap your application in providers.
Zustand, a state management library, was made by Daishi Kato(the man behind Zustand also created Jotai and Valtio) and has easily become a very popular state management library in 2023 because of its ease of use and performance stats. Zustand has a vibrant open-source community actively contributing and enhancing its functionality, making it a popular choice for state management in modern React applications.
Why Use Zustand?
- Simpler and Less Boilerplate
Compared to Redux and the other state management libraries, Zustand allows you to manage state with way less code. It does not require you to define reducers, actions, or dispatch functions — everything is managed via hooks and direct state manipulation.
- Performance Optimization
Zustand is extremely performant by design. It provides the ability selective state subscription. It means that component doesn’t re-render when another part of state that is not used directly by it gets modified. Which helps to avoid unnecessary re-renders.
- State Merging by Default
When you update an object state in Zustand, it maintains the new data with the existing state. Normally, you need to manually spread the previous state {…state,Vegetable:Spinach}
. But in Zustand if your state is {Fruit:Apple, Vegetable:Onion}
and you update it with {Vegetable:Spinach}
, Zustand ensures that {Fruit:Apple}
remains unchanged while {Vegetable:Spinach}
is updated.
- No Need for Context Providers
Zustand does not require a provider wrapping your application like React’s Context API or Redux. This helps in managing states and reduces the complexity of maintaining that piece of code, especially in bigger applications.
- Immutability by Default
The first one is that Zustand enforces immutability on state updates. This method avoids common bugs around mutable state changes and optimizes debugging and performance.
- Middle Ware and Extensibility support
A simple middleware system is available in Zustand, as well as logging, persistence, and dev tools. It provides a lot of room for flexibility and allows developers to implement state management as per their requirement.
- Local and Global State Management
Zustand works great for local and global state. It can work with component specific state while also being able to deal with shared application state without extensive setup.
- State Management Based on Subscriptions
Zustand provides fine-grained dependency tracking so a component can subscribe to just some portion of the state. It allows for selective re-rendering of only the components that rely on specific parts of the state, resulting in improved performance and responsiveness. For example : components explicitly subscribe to specific slices of the state using selectors (useCounterStore(state => state.count)
).
- Insider Information: Must Have Elements
Zustand is an open-source project with a vibrant community of contributors. It is also well maintained with over 30,000 stars on GitHub as at the time of writing.
Installation & Setup:
Installing Zustand
Zustand is a light state management library and would be easily installed using npm or yarn. First, you will want to head to your terminal window and execute one of the following commands:
Using npm:
npm install zustand
Using yarn:
yarn add zustand
This will install Zustand and make it usable in your React project.
Creating a Basic Zustand Store
After installing Zustand, you need to create a store to manage your application state. Zustand provides an intuitive API to define and use state.
Installing Zustand only gets you halfway. With Zustand, you define state and use it with a very simple API. Here is a basic example of defining and using a store.
Step 1 : Store Definition Create a new file (e.g., store. js) and configure your Zustand store:
import { create } from 'zustand';
// Define the store
const useCounterStore = create((set) => ({
count: 0, // Initial state
increase: () => set((state) => ({ count: state.count + 1 })), // Action to increase count
decrease: () => set((state) => ({ count: state.count — 1 })), // Action to decrease count
reset: () => set({ count: 0 }), // Action to reset count
}));
export default useCounterStore;
Step 2 : Using the hook we created in store.js
Once the store is set up, you can use it in your React components:
import React from ‘react’;
import useCounterStore from ‘./store’;
const Counter = () => {
// Access the state and actions from the store
const { count, increase, decrease, reset } = useCounterStore();
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increase}>Increase</button>
<button onClick={decrease}>Decrease</button>
<button onClick={reset}>Reset</button>
</div>
);
};
export default Counter;
Step 3 : Add the Component to the App
Now, simply import and use the Counter component in your main application file (e.g., App.js):
import React from ‘react’;
import Counter from ‘./Counter’;
const App = () => {
return (
<div>
<h1>Zustand Counter Example</h1>
<Counter />
</div>
);
};
export default App;
Zustand eliminates the need for complex reducers and actions.
Only components that use a particular state update when it changes.
Core Concepts Actions, State Updates, and Middleware in Zustand :
- Zustand is a small state management solution for React applications which makes it easy to control and update the state in your app. First one, The core concepts of Zustand are Actions, State Updates and Middleware, Below we will talk about each of them & also show a sample that will help you use them.
Actions
In Zustand, actions are functions through which we are able to change the state. They work like “dispatch actions” in Redux, but are easier to implement. It means actions are defined inside the store and used to amend the state directly.
For instance, in a counter application we will be using actions like increase, decrease and reset to update the count state.
State Updates
- How updates work in Zustand: when we create the store, the set function is available in the callback function. With the set function, you can either pass a new state object to update the state directly, or a callback function that will return the new state based on the current state.
For example:
set({ count: 10 }) // will set count state to 10 directly.
set(state => ({ count: state.count + 1 })) // adds 1 to the count state.
Middleware
Zustand Middleware helps us extend the functionality of store. Middleware allows you to intercept actions before they reach the reducer, which you can use to log state changes, persist state to local storage, or integrate with other libraries like Redux DevTools. We can also create custom middleware, as Zustand has built-in middleware.
The devtools middleware, for example, lets you inspect the changes you make to your state in Redux DevTools.
Adding Middleware : If you wish to hook into some middleware, like logging state changes, you can add it when creating the store. For example, the devtools middleware:
import { create } from ‘zustand’;
import { devtools } from ‘zustand/middleware’;
const useCounterStore = create(
devtools((set) => ({
count: 0,
increase: () => set((state) => ({ count: state.count + 1 })),
decrease: () => set((state) => ({ count: state.count — 1 })),
reset: () => set({ count: 0 }),
}))
);
export default useCounterStore;
This will allow you to debug your state changes using Redux DevTools.
Key Benefits of Zustand :
State Management Simplified : Unlike Redux, Zustand does not require you to write complex reducers and actions to manage your application state.
Performance: Re-renders only occur in components that utilize a specific state when that state changes.
Flexibility: Zustand allows easy enhancement with middleware or custom logic.
Conclusion :
State management is one of the key aspects of building modern web applications, especially as your web applications get more complex. Although React offers some built-in solutions like useState, useContext, and useReducer, state management in large applications can be pretty dirty and hard to maintain. And this is where a state management library comes in, and in this case Zustand.
It is fast, scalable, and light weight state management solution for React Applications. Objects as sources of truth are hugely user friendly in that you can just have your app call the state object directly without complex lazy loading or placing reducers in one area or actions or providers that many libraries use such as redux. Instead, Zustand exposes a small and straightforward API, and is very fast.
Why Zustand Stands Out ?
Less boilerplate: Zustand has less boilerplate than other state management utilities. Only a few lines to define and update state.
Performance: Zustand enables fine-grained updating of components — only those which depend on specific areas of the state will re-render on that state change.
Flexibility: Compatible for both local and global state, making it ideal for small and large applications.
Middleware Support: Zustand allows you to extend its functionality with middleware, such as logging, persistence, or integration with Redux DevTools.
No Providers Needed: Unlike React Context or Redux, Zustand doesn’t require wrapping your app in providers, reducing complexity.
Why Choose Zustand?
- Zustand is an excellent choice for developers who want a simple, efficient, and flexible state management solution. It’s particularly well-suited for React applications of all sizes, from small projects to large-scale applications. With its minimal setup, performance optimizations, and extensibility, Zustand makes state management a breeze.
In summary, if you’re looking for a state management library that’s easy to learn, quick to implement, and powerful enough to handle complex applications, Zustand is a fantastic option. Give it a try, and you’ll see how it simplifies your development workflow while keeping your app performant and maintainable.
Subscribe to my newsletter
Read articles from Harshit Ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
