Day 4: Making Decisions with If-Else Statements
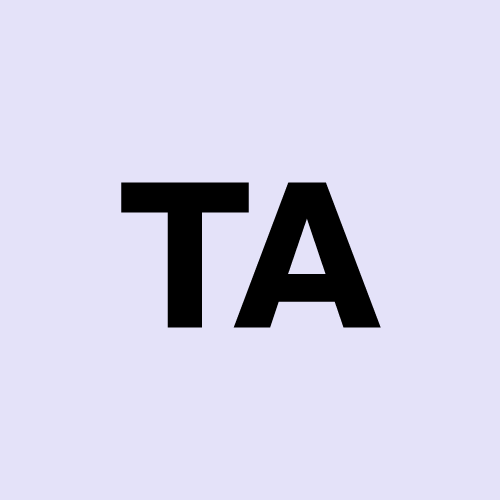
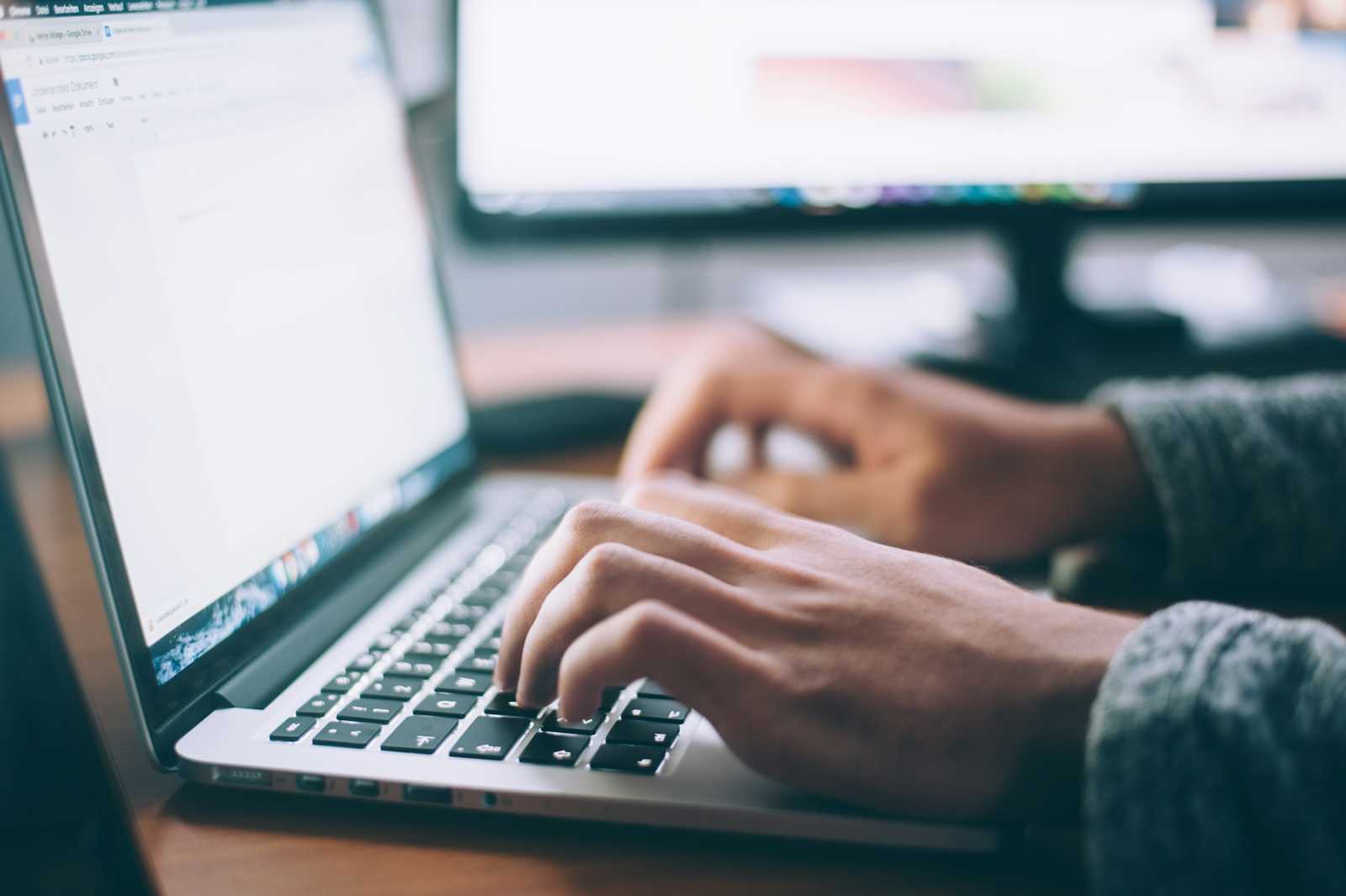
In this article, we will learn how decisions are made by our computer program using the if-else statements. Wwe will be learning:
✅ What are If-Else statements?
✅ How to use If-Else statements
✅ Using Else-If for multiple conditions
✅ Building a simple decision-making program
What are If-Else Statements
Imagine you are going outside and you are stuck in making the right decision for the kind of clothing you need to have on. You have two options:
If the weather is cold, you will have to pick thick clothing
Else, if it is warm, you will pick lighter clother
That’s exactly how computer programs make decisions usinf if-else statements in c#. Another simple example is
If you have enough money, you can buy ice cream. 🍦
Else, you can't buy it. 😢
How to use If-Else Statements in your c# program
In C#, we write an if-else statement like this:
using System;
class Program
{
static void Main()
{
int age = 18;
if (age >= 18)
{
Console.WriteLine("You can vote! 🗳️");
}
else
{
Console.WriteLine("You are too young to vote. 😢");
}
}
}
What Happens?
If
age
is 18 or more, the computer prints:You can vote! 🗳️
If
age
is less than 18, the computer prints:You are too young to vote. 😢
🎉 Great! You just made the computer think and decide!
Using If-Else for multiple conditions
Sometimes, you might need your computer program to make decisions from more than two options. For example;
If it's raining, take an umbrella. ☔
Else if it's cold, wear a jacket. 🧥
Else, just go outside as you are. 😃
In C#, we can use if-else for multiple conditions:
using System;
class Program
{
static void Main()
{
int temperature = 15;
if (temperature > 30)
{
Console.WriteLine("It's really hot! Drink water. 🥵");
}
else if (temperature > 20)
{
Console.WriteLine("It's warm. Enjoy the day! 😃");
}
else if (temperature > 10)
{
Console.WriteLine("It's a bit chilly. Wear a jacket. 🧥");
}
else
{
Console.WriteLine("It's really cold! Stay warm. ❄️");
}
}
}
What Happens?
If
temperature = 35
, the output is:It's really hot! Drink water. 🥵
If
temperature = 22
, the output is:It's warm. Enjoy the day! 😃
If
temperature = 15
, the output is:It's a bit chilly. Wear a jacket. 🧥
If
temperature = 5
, the output is:It's really cold! Stay warm. ❄️
🎉 Now the computer can make multiple decisions!
Let’s try something more fun. We will take in a user input in our if-else statements. We will ask the user to input their age and make the program decide if the user can drive or not
using System;
class Program
{
static void Main()
{
Console.WriteLine("Enter your age:");
int age = Convert.ToInt32(Console.ReadLine());
if (age >= 18)
{
Console.WriteLine("You are old enough to drive! 🚗");
}
else
{
Console.WriteLine("Sorry, you need to be at least 18 to drive. 😢");
}
}
}
What Happens?
1️⃣ The program asks:
Enter your age:
2️⃣ You type 17 → The output is:
Sorry, you need to be at least 18 to drive. 😢
3️⃣ You type 20 → The output is:
You are old enough to drive! 🚗
👏 Now the computer can make decisions based on user input!
Building a simple decision-making program
Let’s create a program that takes a students score and assign a grade. This program can be used by academic institutions to grade students according to their score. This will save them the stress of having to go through a long spreadsheet of scores and manually assigning grades.
Let’s build a program that takes a student’s score and assigns a grade!
using System;
class Program
{
static void Main()
{
Console.WriteLine("Enter your score:");
int score = Convert.ToInt32(Console.ReadLine()); //This ensures our user's input is in integers
if (score >= 90)//If score is greater than or equal to 90
{
Console.WriteLine("Grade: A 🎉");
}
else if (score >= 80)//If score is greater than or equal to 80
{
Console.WriteLine("Grade: B 😊");
}
else if (score >= 70)//If score is greater than or equal to 70
{
Console.WriteLine("Grade: C 😐");
}
else if (score >= 60)//If score is greater than or equal to 60
{
Console.WriteLine("Grade: D 😕");
}
else
{
Console.WriteLine("Grade: F 😢");
}
}
}
What Happens?
If the user enters 95, the output is:
Grade: A 🎉
If the user enters 72, the output is:
Grade: C 😐
If the user enters 55, the output is:
Grade: F 😢
🎉 You just built an automatic grading system!
You can make the grading system for fun by further modifying it using all we’ve learnt so far.
Modify the grading system so that:
1️⃣ It asks for the student’s name first.
2️⃣ The final message includes their name, like:
Taiwo, your grade is: B 😊
(Hint: Use Console.ReadLine()
to get the name and store it in a string
variable!)
That’s all for this article, see you in day 5!
Subscribe to my newsletter
Read articles from Taiwo Ayorinde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
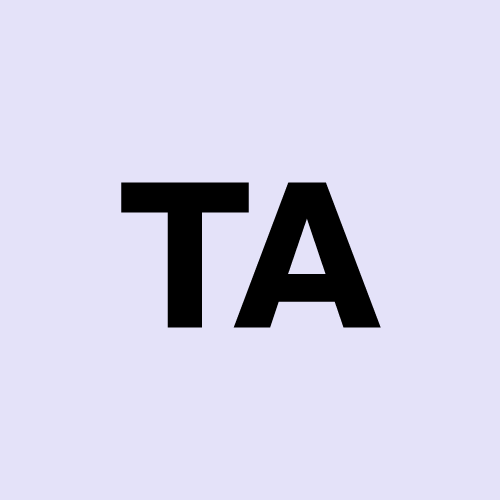