Using DataLoader to Batch and Optimize Database Queries in GraphQL β‘

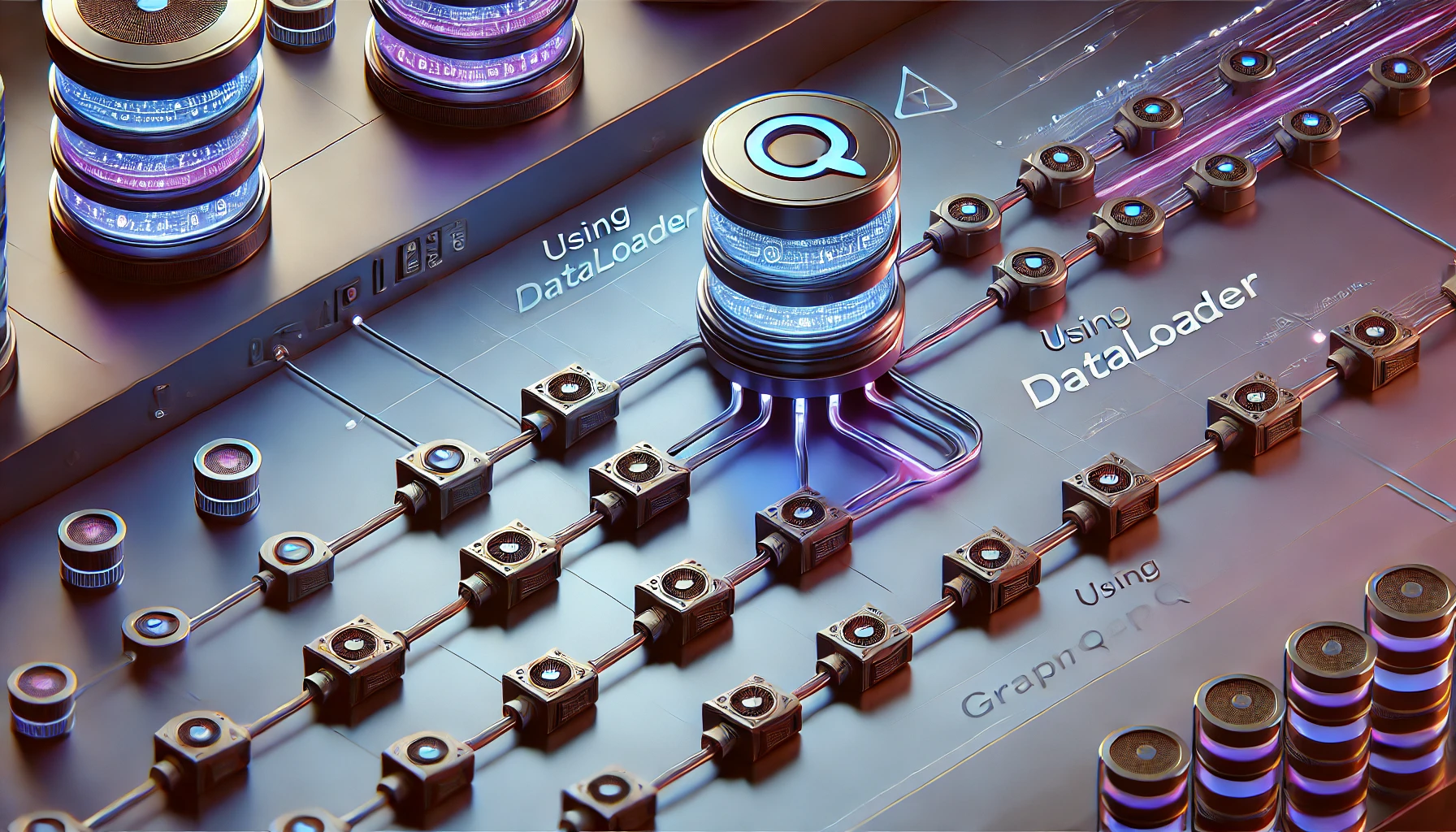
What is DataLoader?
DataLoader is a generic utility developed by Facebook for batching and caching database queries efficiently in GraphQL applications. It helps in reducing redundant queries and solving the N+1 query problem by grouping multiple queries into a single batch request.
Key Features of DataLoader:
Batching: Combines multiple database requests into a single query to optimize performance.
Caching: Stores results to prevent redundant database calls in the same request cycle.
Asynchronous Execution: Uses promises to handle multiple database requests efficiently.
By integrating DataLoader into GraphQL resolvers, we can significantly improve the efficiency and scalability of our APIs.
Why DataLoader is Necessary π§
GraphQL APIs provide flexibility in data fetching, allowing clients to request only the necessary data. However, this flexibility can lead to the N+1 query problem, a common performance issue where multiple queries to related data cause database inefficiencies.
The N+1 Query Problem ποΈ
In a GraphQL resolver, if fetching related data requires separate queries for each parent record, it results in an exponential increase in database calls. Consider an example:
const resolvers = {
Query: {
users: async () => await db.User.findAll(),
},
User: {
posts: async (parent) => await db.Post.findAll({ where: { userId: parent.id } })
}
};
If we fetch 10 users along with their posts, the resolver first queries for users (1 query
), then for each user, it fetches their posts (10 additional queries
). This results in 11 queries instead of an optimal 2 queries.
How DataLoader Solves This Problem
DataLoader batches multiple queries into a single request and caches results to avoid redundant calls. It allows GraphQL resolvers to efficiently fetch related data in bulk. π¦πβ‘
Step-by-Step Implementationπ
1. Install DataLoader π§
If not already installed, add DataLoader to your project:
npm install dataloader
2. Create a DataLoader for Batching Queries π
In your GraphQL setup, define a DataLoader instance to batch and cache database queries.
const DataLoader = require('dataloader');
const db = require('./models');
const userPostLoader = new DataLoader(async (userIds) => {
const posts = await db.Post.findAll({ where: { userId: userIds } });
const postsByUserId = userIds.map(id => posts.filter(post => post.userId === id));
return postsByUserId;
});
3. Integrate DataLoader in Resolvers π
Modify the resolver to use DataLoader instead of making separate queries.
const resolvers = {
Query: {
users: async () => await db.User.findAll(),
},
User: {
posts: (parent, args, context) => context.userPostLoader.load(parent.id)
}
};
4. Attach DataLoader to Context π
Ensure DataLoader is available in each request by adding it to the context in your GraphQL server setup.
const { ApolloServer } = require('apollo-server');
const server = new ApolloServer({
typeDefs,
resolvers,
context: () => ({
userPostLoader: userPostLoader
})
});
Verifying Performance Improvements π
Before implementing DataLoader, let's analyze the number of queries being made. Consider the following GraphQL query:
query {
users {
id
name
posts {
id
title
}
}
}
Without DataLoader : β
Query to fetch users (1 query)
SELECT * FROM users;
Query for each user's posts (10 queries if there are 10 users)
SELECT * FROM posts WHERE userId = 1; SELECT * FROM posts WHERE userId = 2; ... SELECT * FROM posts WHERE userId = 10;
Total queries: 11
With DataLoader : β
Query to fetch users (1 query)
SELECT * FROM users;
Single batched query to fetch all posts at once (1 query)
SELECT * FROM posts WHERE userId IN (1,2,3,4,5,6,7,8,9,10);
Total queries: 2
Performance Gains : π
By reducing the number of queries from 11 to 2, DataLoader significantly reduces database load and improves response time. The reduction in queries is more noticeable as the dataset grows, ensuring better scalability and performance efficiency. Before DataLoader, fetching posts for 10 users resulted in 11 queries. With DataLoader, it reduces to 2 queries:
Fetch all users
Fetch all posts in a single batch query
Integrating DataLoader with Prisma/Sequelize/MongoDB π οΈ
Using DataLoader with Prisma
const userPostLoader = new DataLoader(async (userIds) => {
const posts = await prisma.post.findMany({
where: { userId: { in: userIds } },
});
const postsByUserId = userIds.map(id => posts.filter(post => post.userId === id));
return postsByUserId;
});
Using DataLoader with Sequelize
const userPostLoader = new DataLoader(async (userIds) => {
const posts = await Post.findAll({
where: { userId: userIds },
});
return userIds.map(id => posts.filter(post => post.userId === id));
});
Using DataLoader with MongoDB (Mongoose)
const userPostLoader = new DataLoader(async (userIds) => {
const posts = await Post.find({ userId: { $in: userIds } });
return userIds.map(id => posts.filter(post => post.userId.toString() === id.toString()));
});
Impact of Using DataLoader
Improved Performance: Reduces database queries, significantly optimizing response times.
Better Scalability: Handles large GraphQL queries efficiently, making the API more scalable.
Reduced Database Load: Batching reduces the number of queries, minimizing database stress.
Caching Benefits: Avoids redundant database calls by caching previously fetched data.
Conclusion π‘
Using DataLoader in GraphQL APIs is essential for optimizing database queries and solving the N+1 query problem. By batching requests and caching results, it significantly enhances performance and scalability. Whether you are using Prisma, Sequelize, or MongoDB, integrating DataLoader is a best practice for efficient GraphQL APIs.
Subscribe to my newsletter
Read articles from Gaurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Kumar
Gaurav Kumar
Hey! I'm Gaurav, a tech writer who's all about MERN, Next.js, and GraphQL. I love breaking down complex concepts into easy-to-understand articles. I've got a solid grip on MongoDB, Express.js, React, Node.js, and Next.js to supercharge app performance and user experiences. And let me tell you, GraphQL is a game-changer for data retrieval and manipulation. I've had the opportunity to share my knowledge through tutorials and best practices in top tech publications. My mission? Empowering developers and building a supportive community. Let's level up together with my insightful articles that'll help you create top-notch applications!